Java Programming Language Table of content:
Dynamic Array In Java | Working, Uses & More (+Code Examples)
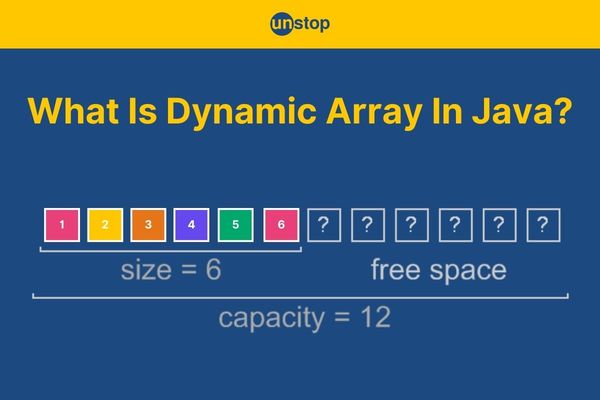
In the world of programming, data structures play a pivotal role in organizing and managing data efficiently. Among these, arrays are a fundamental structure, allowing us to store multiple elements of the same type. However, traditional arrays in Java come with a limitation—they have a fixed size. What happens when we don’t know the exact size of data in advance or need to grow the storage dynamically? This is where dynamic arrays come to the rescue.
In this article, we’ll explore the concept of dynamic arrays in Java, how they work, and their advantages over fixed-size arrays. We’ll also dive into popular implementations like ArrayList and discuss practical use cases with examples to help you leverage their potential effectively.
What Is A Dynamic Array In Java?
A dynamic array in Java programming can change its size during runtime, unlike static arrays, which are fixed in size when created. While arrays in Java are typically fixed in size, there are classes and mechanisms that provide dynamic resizing. The most common approach is to use classes from the Java Collections Framework, such as ArrayList.
Key Points:
- Dynamic Sizing: Unlike static arrays, dynamic arrays can grow or shrink as needed based on the data they hold.
- Underlying Structure: While a dynamic array may look like a traditional array, internally, it is often implemented with an array that resizes when necessary. If the array runs out of space, it can be resized, typically by doubling its size.
Example of Use Case: Imagine you are working with a list of users in a system. You start with 10 users, but later you find you need to handle 100,000 users. Using a dynamic array allows you to easily expand your storage as your requirements grow without worrying about the initial size.
Why Use Dynamic Array In Java?
Dynamic arrays, like ArrayList, offer several advantages over traditional static arrays. Here are the key reasons:
1. Flexible Size
- Fixed arrays require specifying the size upfront, which can be limiting if you're unsure about the number of elements to store. Dynamic arrays can grow and shrink as needed.
- Example: With dynamic arrays, you don't need to know how many items you'll need at the start of your program.
2. Ease of Use
- Dynamic arrays in Java, such as ArrayList, come with built-in methods for adding, removing, and accessing elements, making them much easier to work with than traditional arrays.
- No need for manual resizing or array manipulation.
3. Automatic Resizing
- When you add an element to a dynamic array and it reaches its capacity, the array automatically resizes (usually by doubling its size) to accommodate more elements.
- This automatic resizing saves you from needing to manually resize the array when it’s full, making the code cleaner and more efficient.
4. Efficient Memory Usage
- Dynamic arrays typically allocate extra memory when they resize to ensure there’s room for future additions without resizing too often. This reduces the number of resizes and ensures that memory is used efficiently.
5. Built-in Methods
- Classes like ArrayList come with a wide variety of useful methods (e.g., add(), remove(), contains(), size(), etc.) that allow you to perform operations on the array easily, without having to implement them manually.
- Example: You can quickly add, remove, or access elements with a simple method call.
6. Improved Performance for Some Operations
- While traditional arrays might offer a performance advantage in specific cases due to their fixed nature, dynamic arrays provide better performance in scenarios where the array size can vary, especially if you constantly add and remove elements.
7. Better Integration with Java Collections Framework
- Dynamic arrays like ArrayList integrate seamlessly with Java's Collections Framework, which includes additional functionality for sorting, searching, and filtering data, making them suitable for a wide range of tasks.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
What Is The Size And Capacity Of A Dynamic Array In Java?
In Java, the size and capacity of a dynamic array (such as an ArrayList) are important concepts for understanding how data is managed and stored. Let's break them down:
1. Size of a Dynamic Array:
- The size refers to the number of elements currently present in the dynamic array.
- It is the actual number of items you’ve added to the array.
- In an ArrayList, you can get the size using the size() method.
For Example:
ArrayList<Integer> dynamicArray = new ArrayList<>();
dynamicArray.add(10);
dynamicArray.add(20);
dynamicArray.add(30);
System.out.println("Size: " + dynamicArray.size()); // Output: 3
Here, the size of the array is 3 because there are three elements.
2. Capacity of a Dynamic Array:
- The capacity refers to the total amount of space allocated for the array. It’s the maximum number of elements the array can hold before it needs to resize.
- The capacity is not directly exposed by the ArrayList class. However, it increases automatically when the array exceeds its current capacity.
- By default, an ArrayList starts with a capacity of 10 and increases in size (usually by doubling) when the number of elements exceeds the current capacity.
For Example:
ArrayList<Integer> dynamicArray = new ArrayList<>(5); // Initial capacity of 5
dynamicArray.add(10);
dynamicArray.add(20);
dynamicArray.add(30);
System.out.println("Size: " + dynamicArray.size()); // Output: 3
// Capacity is internal and not directly accessible, but it will automatically grow as needed.
When you add more elements than the current capacity, the ArrayList automatically resizes itself, typically doubling the current capacity.
How To Create A Dynamic Array In Java?
Creating a dynamic array in Java is straightforward, especially with the help of the ArrayList class from the Java Collections Framework. Here's a step-by-step guide:
- Import the ArrayList Class: To use ArrayList, you need to import it from the java.util package.
- Create an Instance of ArrayList: Instantiate an ArrayList object. You can specify the type of data it will store (e.g., Integer, String, etc.). Optionally, you can specify an initial capacity for the array.
- Add Elements: Use the add() method to insert elements into the array.
- Access and Manipulate Elements: You can access elements using the get() method. Other methods like remove(), size(), and set() allow you to manipulate the array.
- Print the Array: Use System.out.println() to print the array.
Code Example:
Output:
Dynamic Array: [10, 20, 30]
Size: 3
After Adding More Elements: [10, 20, 30, 40, 50]
After Removal: [10, 20, 40, 50]
Element at Index 1: 20
Explanation:
In the above code example-
- We begin by importing the ArrayList class from Java's util package to enable the creation of a dynamic array.
- In the main method, we create an ArrayList named dynamicArray that will store integers dynamically without a predefined size.
- We add elements 10, 20, and 30 to the array using the add() method, which appends elements to the end of the list.
- To view the contents of our dynamic array and its current size, we print the array and use the size() method. Initially, the output shows [10, 20, 30] and size 3.
- We continue by adding two more elements, 40 and 50, expanding the array. Printing again shows the updated array [10, 20, 30, 40, 50].
- Next, we remove the element at index 2 (value 30) using the remove() method. After removal, the updated array is [10, 20, 40, 50].
- Finally, we access the element at index 1 (value 20) using the get() method and print it. This demonstrates how we can retrieve specific elements from the dynamic array.
Let’s now look at some practical use cases of dynamic arrays in Java-
Managing Dynamic Data Input In Java
Dynamic arrays are ideal when the size of the data is unknown at compile time. For instance, when you need to store user input or process data from an API, the array size can grow dynamically as new data is added. Example Scenario: A program that takes user input of integers and stores them in a dynamic array until the user stops.
Code Example:
Output:
Enter numbers (type -1 to stop):
5
10
15
-1
You entered: [5, 10, 15]
Total numbers: 3
Explanation:
In the above code example-
- We start by importing the ArrayList class for dynamic arrays and the Scanner class to handle user input.
- In the main method, we create a Scanner object to read inputs from the user and an ArrayList named numbers to store integers dynamically.
- We prompt the user to enter numbers, instructing them to type -1 to stop the input process.
- Using a while loop, we continuously read integers entered by the user. If the input is -1, we break out of the loop. Otherwise, we add the input to the dynamic array using the add() method.
- After the loop ends, we print the contents of the numbers array to display all the integers entered by the user.
- Finally, we use the size() method to determine and print the total number of integers stored in the array, excluding the terminating -1.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Storing And Processing Real-Time Data In Java
Dynamic arrays are perfect for applications where data is fetched or processed in real time, such as logging data, collecting messages, or storing search results. Example Scenario: Storing and displaying real-time chat messages.
Code Example:
Output:
Chat Messages:
User1: Hello!
User2: Hi there!
User1: How are you?
Explanation:
In the above code example-
- We begin by importing the ArrayList class to use a dynamic array for storing chat messages.
- In the main method, we create an ArrayList named chatMessages to hold strings representing messages in a chat.
- Using the add() method, we simulate incoming messages by adding three messages to the chatMessages array: "User1: Hello!", "User2: Hi there!", and "User1: How are you?".
- To display all the stored messages, we print a header "Chat Messages:" and use a for-each loop to iterate through the chatMessages array. Each message is printed on a new line, representing a real-time chat display.
Use Cases Of Dynamic Arrays In Java
Dynamic arrays, such as ArrayList in Java, offer flexible and efficient ways to handle data that changes in size during runtime. Here are some common use cases:
- Handling Dynamic User Input: Ideal for collecting user input when the number of entries is unknown at runtime. Example: Storing user-entered integers until they signal to stop.
- Managing Dynamic Collections: Used in applications like inventories, shopping carts, or to-do lists where items are frequently added or removed. Example: Managing a shopping cart with add and remove operations.
- Storing Real-Time Data: Perfect for real-time scenarios such as logging events, storing messages, or managing search results dynamically. Example: Storing chat messages in a messaging application.
- Processing Variable Data from APIs: Useful for storing data retrieved from APIs with unpredictable sizes. Example: Storing API responses for processing or display.
- Temporary Data Storage: Acts as a temporary buffer for data before processing or transferring. Example: Collecting file data before saving to a database.
- Dynamic Game Mechanics: Manage game inventories, leaderboards, or player stats that change dynamically. Example: Tracking players joining or leaving a game in real time.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
Dynamic arrays in Java, such as ArrayList, provide a powerful and flexible way to handle data that changes in size during runtime. Unlike traditional arrays, they automatically resize themselves, offering the perfect balance between simplicity and functionality. Whether you're managing user inputs, storing real-time data, or implementing dynamic collections, dynamic arrays make the process seamless and efficient. By leveraging their capabilities, developers can create adaptable and robust applications without worrying about predefined sizes or memory management. Dynamic arrays truly highlight Java's ability to cater to both flexibility and performance in modern programming.
Frequently Asked Questions
Q. What is the difference between a dynamic array and a regular array in Java?
- Regular Array: Regular arrays in Java have a fixed size, defined at the time of creation. Once initialized, their size cannot change. Example:
int[] arr = new int[5];
- Dynamic Array: Dynamic arrays, such as ArrayList, can grow or shrink as needed. Their size adjusts automatically when elements are added or removed. Example:
ArrayList<Integer> list = new ArrayList<>();
list.add(10); // Automatically increases capacity
Q. How does a dynamic array resize itself in Java?
Dynamic arrays in Java (like ArrayList) manage resizing internally. When the current capacity is full, the array creates a new larger array (typically 1.5–2 times the previous size), copies the elements into it, and replaces the old array.
Example: Suppose the current capacity is 10. When the 11th element is added, a new array of size 20 is created, and the elements from the old array are copied to the new one.
Q. What are the advantages of using dynamic arrays in Java?
- Flexibility: Automatically resizes as elements are added or removed.
- Ease of Use: Built-in methods like add(), remove(), and get() make operations simple.
- Dynamic Memory Allocation: Allocates memory dynamically, optimizing space usage.
- Versatility: Suitable for various use cases, from managing user input to real-time applications.
Q. What is the default capacity of an ArrayList in Java?
The default initial capacity of an ArrayList in Java is 10. When elements are added beyond this capacity, the array resizes itself, typically doubling its previous capacity.
Example: If you create an ArrayList without specifying the capacity:
ArrayList<Integer> list = new ArrayList<>();
The initial capacity is 10. Adding the 11th element triggers resizing.
Q. What are some common methods of ArrayList used for dynamic arrays?
Here are a few commonly used methods of ArrayList in Java:
- add(E element): Adds an element to the array.
- remove(int index): Removes the element at the specified index.
- get(int index): Retrieves the element at the specified index.
- size(): Returns the current number of elements in the array.
- clear(): Removes all elements from the array.
- contains(Object o): Checks if the array contains a specific element.
Q. Are dynamic arrays thread-safe in Java?
No, dynamic arrays like ArrayList in Java are not thread-safe. If multiple threads access an ArrayList concurrently and modify it, such as adding or removing elements, it can lead to inconsistent behavior and data corruption.
To ensure thread safety, you can use synchronized collections like Collections.synchronizedList(new ArrayList<>()), which wraps the ArrayList in a synchronized layer, or opt for alternatives like CopyOnWriteArrayList from the java.util.concurrent package, which is specifically designed for concurrent access.
However, these solutions may impact performance due to synchronization overhead. For highly concurrent scenarios, it's essential to carefully choose the right approach based on the application's requirements.
With this, we conclude our discussion on dynamic arrays in Java. Here are a few other topics that you might be interested in reading:
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- Throws Keyword In Java | Syntax, Working, Uses & More (+Examples)
- 10 Best Books On Java In 2024 For Successful Coders
- Difference Between Java And JavaScript Explained In Detail
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)