Java Programming Language Table of content:
Break Statement In Java | Working, Uses And More (+Code Examples)
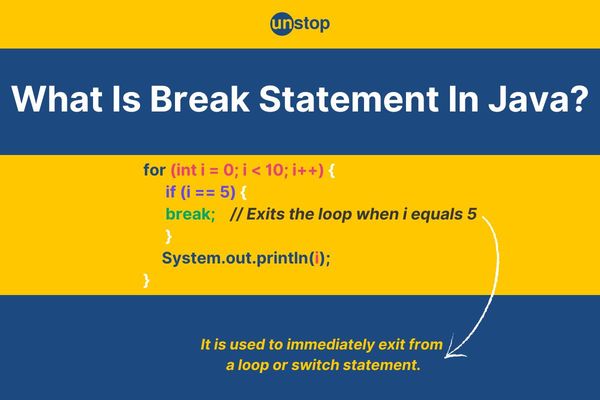
In Java, the break statement is a powerful control flow tool that allows us to exit from loops or switch statements prematurely. By using break, we can stop the execution of a loop or switch case before its natural termination condition is met. This can be particularly useful in scenarios where we want to terminate a process based on a certain condition, enhancing the efficiency and readability of the code. In this article, we will explore how the break statement works and its usage in different control structures, as well as provide examples to demonstrate its functionality.
What Is The Break Statement In Java?
The break statement in Java programming is used to immediately exit from a loop or switch statement when a certain condition is met. This allows you to stop the execution of the loop or switch early, without waiting for it to finish naturally. It is commonly used in for, while, and switch statements to improve efficiency or to handle specific scenarios where continuing the loop or switch is no longer necessary.
Syntax Of Break:
break;
Here, the Java keyword break is a command that tells the program to exit the loop or switch.
Working Of The Break Statement In Java

The break statement in Java is used to terminate the execution of loops or switch statements prematurely. Below is a breakdown of how the break statement works, followed by a flowchart for better visualization.
- Purpose: It is used to exit from a loop (like for, while, or do-while) or a switch block before their natural termination condition is met.
- Inside Loops: When the break statement is encountered inside a loop (like for, while, or do-while), it causes the loop to terminate immediately, and the program control moves to the next statement following the loop.
- Inside Switch: In a switch statement, the break ensures that only the matching case is executed, and the program exits the switch block without executing subsequent cases.
- Impact on Execution: When break is encountered, it terminates only the innermost loop or switch statement and does not affect other loops or switches outside of it.
- Common Usage: Typically used when a condition is met inside the loop or switch, and further execution is unnecessary or undesirable.
- Flow Control: The program’s flow moves immediately to the statement following the loop or switch after the break is executed.
Using Java Break Statement With Loops

The break statement is commonly used in loops to immediately exit the loop when a specific condition is met. This can be helpful when you want to stop further iterations based on some logic or when you no longer need to process additional elements. When the break statement is executed, the program control moves to the next statement after the loop, effectively terminating the loop prematurely.
Code Example:
Output (set code file name as BreakInAllLoops.java):
For Loop:
1
2
3
4
While Loop:
1
2
3
4
Do-While Loop:
1
2
3
4
Explanation:
In the above code example-
- We start by defining the class BreakInAllLoops with the main() method where all code execution begins.
- First, we print "For Loop:" to indicate that we are starting the for loop section.
- In the for loop, we initialize a variable i to 1 and set the loop to run as long as i is less than or equal to 10.
- Inside the loop, we use an if statement to check if i is 5. If true, we use break statement to exit the loop immediately.
- If i is not 5, we print the current value of i and proceed to the next iteration by incrementing i.
- Next, we print "While Loop:" to start the while loop section.
- We initialize a variable j to 1 and set the loop to run as long as j is less than or equal to 10.
- Similar to the for loop, we check if j is 5. If it is, the loop is exited using the break statement.
- If j is not 5, we print j and then increment j by 1 to continue the loop.
- Finally, we print "Do-While Loop:" to start the do-while loop section.
- In the do-while loop, we initialize a variable k to 1. The loop runs at least once, and the condition is checked after each iteration.
- Inside the loop, we check if k is 5, and if true, the loop is exited using a break statement.
- If k is not 5, we print k and increment it by 1. The loop continues as long as k is less than or equal to 10.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Using Java Break Statement With Switch Statement
The break statement is commonly used in Java's switch statement to terminate a particular case and exit the switch block. Without the break statement, the program would continue executing subsequent cases, even if they don't match the condition—this behavior is known as "fall-through."
The break ensures that the program exits the switch block after executing the matched case, preventing it from running the code for the following cases unintentionally.
Code Example:
Output (set code file name as BreakInSwitch.java):
Wednesday
Explanation:
In the above code example-
- We start by defining the class BreakInSwitch with the main() method, where the execution begins.
- A variable day is initialized with the value 3, representing a specific day of the week.
- The switch statement is used to check the value of day and execute the corresponding block of code for that case.
- In the first case, if day is 1, the program prints "Monday" and then exits the switch statement using the break statement.
- Similarly, for day values of 2, 3, 4, 5, 6, and 7, the program prints the respective day (e.g., "Tuesday" for 2, "Wednesday" for 3, and so on) and exits the switch statement after each case with a break.
- If day does not match any of the cases (i.e., the value of day is not between 1 and 7), the default case is triggered, and "Invalid day" is printed.
- The break statement in each case ensures that only the matching case is executed, and the switch statement is exited immediately after that, preventing any fall-through to the subsequent cases.
Using Java Break Statement With Infinite Loops
An infinite loop occurs when the loop condition is always true, meaning the loop runs indefinitely. In Java, we can create an infinite loop using a while(true) or for(;;) statement. The break statement can be used in these infinite loops to stop the execution when a specific condition is met. This allows the program to exit the loop safely and continue with the rest of the code, instead of running forever.
In practice, an infinite loop combined with a break statement is useful in situations where you need the program to continuously perform a task (such as waiting for user input) but need a condition to break out of the loop (like entering a specific command or receiving an input signal).
Code Example:
Output (set code file name as InfiniteLoopWithBreak.java):
Enter a number (enter 0 to stop): 5
You entered: 5
Enter a number (enter 0 to stop): 10
You entered: 10
Enter a number (enter 0 to stop): 0
Exiting the loop...
Explanation:
In the above code example-
- We start by importing the Scanner class to read user input from the console.
- The main() method is defined, where a Scanner object named scanner is created to read inputs from the user.
- We use a while (true) loop to create an infinite loop, which will keep running until a specific condition is met.
- Inside the loop, we prompt the user to enter a number by displaying the message "Enter a number (enter 0 to stop): ".
- The user’s input is captured using scanner.nextInt(), and the entered number is stored in the variable num.
- We then check if the entered number is 0. If it is, the program prints "Exiting the loop..." and the break statement is used to exit the infinite loop.
- If the entered number is not 0, the program prints the message "You entered: " followed by the entered number, and the loop continues to prompt the user for another input.
- Once the loop is exited (when the user enters 0), we close the scanner to free up resources.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Common Pitfalls While Using Break Statements In Java
While the break statement in Java can be a useful tool to exit loops or switch blocks prematurely, it can lead to certain pitfalls if not used carefully. Here are some common issues that developers might encounter:
- Forgetting to use break in a switch statement: This can lead to unintended fall-through to the next case.
- Using break too early in loops: This causes the loop to terminate prematurely, leading to incomplete processing.
- In nested loops, break only exits the innermost loop: It does not affect outer loops, which may cause confusion if both loops need to be exited.
- Using break inside a switch statement within loops: It only breaks out of the switch, not the loop itself, which can lead to confusion.
- Overusing break without clear logic: This can clutter the code and make it harder to understand and maintain.
- Relying on break to fix complex logic issues: Rather than restructuring the logic, relying on break may mask deeper problems in the code.
Best Practices For Using The Break Statement In Java
- Use break Sparingly: Avoid overusing break as it can make the code harder to read and maintain. Instead, structure your logic to handle termination conditions clearly and naturally without relying too much on break.
- Place break in the Right Context: Ensure that the break statement is logically placed where it is easy to understand. In loops, break should be used to exit early when a condition is met, and in switch statements, it should be used to prevent fall-through to other cases.
- Avoid Nested break: Avoid placing break statements in deeply nested loops, as it can make the flow of control difficult to follow. If necessary, consider refactoring the code or using flags to break out of multiple levels.
- Use break to Exit Loops When Needed: If you're searching through a collection (such as an array or list) and have found the required element, using break helps avoid unnecessary iterations and improves performance.
- Prefer Return Statements in Functions: In functions, use a return statement to exit early rather than relying on break. This improves code clarity and avoids unnecessary loops.
- Document When and Why break is Used: If break is used in complex scenarios, especially inside loops or switch statements, ensure that its purpose is well-documented with comments to help others understand its role.
- Be Mindful of Infinite Loops: When using break in infinite loops (e.g., while(true)), ensure that the condition to break the loop is clearly defined and that the program will not get stuck in the loop indefinitely.
- Use break in switch Cases: In switch statements, always use break unless you intentionally want fall-through behavior. This ensures that only the matched case is executed, and control does not continue to subsequent cases.
- Use break for Improved Readability: Use break to make your code more readable when an exit condition is simple. For example, breaking out of a loop once a specific element is found helps make your code more intuitive and avoids unnecessary iterations.
- Ensure Control Flow Is Clear: Ensure that the use of break doesn't lead to unintended behavior, especially in complex control structures like nested loops. It's often better to refactor the code to avoid confusing control flow.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Conclusion
The break statement in Java is a powerful tool that enhances control flow by allowing early termination of loops and switch statements. It provides flexibility to exit from loops once a specific condition is met, thereby improving performance and reducing unnecessary iterations. However, its overuse or improper placement can lead to confusing or hard-to-maintain code. By following best practices—such as using break sparingly, placing it logically within the flow of control, and documenting its purpose—developers can write clear, efficient, and maintainable code. Ultimately, understanding and applying the break statement correctly ensures that your program behaves as expected while avoiding potential pitfalls.
Frequently Asked Questions
Q. What is the purpose of the break statement in Java?
The break statement in Java is used to immediately terminate the execution of a loop or switch statement. It allows the program to exit a loop or switch case prematurely based on a specific condition, saving unnecessary iterations or checks. This can be particularly useful in scenarios where you only need to find the first occurrence of a value, or when continuing a switch statement would cause unintended fall-through behavior. For example, inside a loop, you can break once a target element is found, avoiding further checks.
Q. Can the break statement be used to exit multiple loops at once?
No, the break statement only exits the innermost loop or switch statement. If you need to exit multiple loops, you cannot directly use break for each loop. However, you can use a flag variable to signal the outer loop to exit or consider using labeled break statements. Alternatively, you can refactor the code into methods and return early from the method to exit all loops.
Q. Is it possible to use break in a try-catch block?
Yes, the break statement can be used inside a try, catch, or finally block to exit a loop. However, it cannot be used to exit the try-catch block itself; it will only affect loops that are in the same scope. The break will act as it would in any other block and will only exit the loop or switch statement, not the catch or try block. For example-
for (int i = 0; i < 5; i++) {
try {
if (i == 3) {
System.out.println("Found i = 3, breaking out of loop");
break; // Exits the loop
}
} catch (Exception e) {
// Catch any exceptions
}
}
Q. Is it necessary to use break in a switch statement?
While it’s not always mandatory, using break in a switch statement is highly recommended to prevent fall-through behavior. In Java, if you don’t use break at the end of a case, the program will continue to execute the next case statement, even if it doesn't match the condition. This can lead to unintended behavior. Using break ensures that only the matched case block is executed.
Q. Can break be used with labeled statements in Java?
Yes, Java allows the use of labeled break statements to exit from outer loops, especially in the case of nested loops. When dealing with multiple nested loops, a labeled break allows you to break out of a specific loop, not just the innermost one. This is particularly useful when you want to terminate a higher-level loop directly, based on a condition inside a nested loop.
Q. What happens if the break statement is used in an infinite loop?
In an infinite loop (such as while(true)), the break statement will exit the loop when the specified condition is met. Without the break, the loop would run indefinitely, but with the break, the program can stop the loop based on certain conditions (e.g., user input or a particular value being found). It is commonly used in scenarios like reading user input or continuously checking for conditions without overloading the system.
Q. How does break work with continue in loops?
The break and continue statements serve different purposes in loops. While break exits the loop entirely, continue skips the current iteration and proceeds to the next iteration of the loop. They can be used together but are typically used for different control flow purposes. Using them in combination requires careful consideration to ensure the flow of control is logical and easy to follow. For example-
for (int i = 0; i < 10; i++) {
if (i == 5) {
continue; // Skip iteration when i equals 5
}
if (i == 8) {
break; // Exit loop when i equals 8
}
System.out.println(i);
}
With this, we conclude our discussion on the break statement in Java. Here are a few other topics that you might be interested in reading:
- Thread In Java | Lifecycle, Methods, Priority & More (+Examples)
- Multithreading In Java - Complete Guide With Uses & Code Examples
- Daemon Thread In Java | Creation, Applications & More (+Examples)
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples