Python Programming Table of content:
Print A Calendar In Python - Detailed Steps With Code Examples
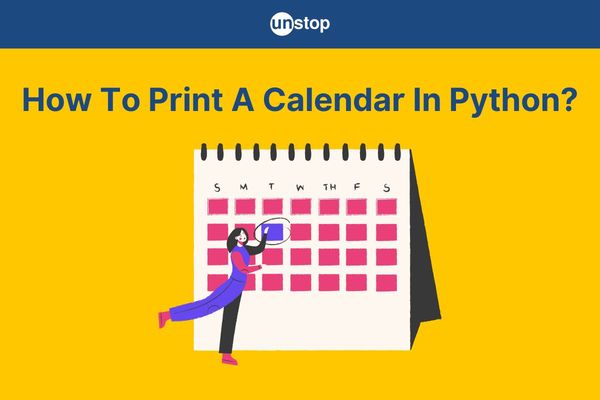
In our fast-paced digital world, managing time effectively has become more crucial than ever. Whether for personal organization, project management, or event planning, a well-structured calendar can be a game-changer. Python, with its versatility and ease of use, offers a powerful platform for creating custom calendar applications that can cater to a wide range of needs.
In this article, we will focus on how to create a simple Python program that displays a calendar for any month and year, using Python's built-in libraries to facilitate the task.
The Calendar Module In Python
The calendar module in Python programming provides functions and classes that allow users to work with dates and calendars in a straightforward and intuitive manner. It is a built-in module, meaning it comes pre-installed with Python, so there’s no need for additional installation.
The syntax of the calendar module is as follows:
import calendar
Key Features Of The Calendar Module
Some of the important features of the Python calendar module are as follows:
- Displaying Calendars: The calendar Python module allows you to generate and display calendars for specific months and years in a user-friendly format.
- Manipulating Dates: It provides functions to calculate and manipulate dates, such as determining the number of leap years, finding out which day of the week a specific date falls on, etc.
- Customizable Output: Users can format the output of calendars in various ways, including the layout and the starting day of the week.
- Support for Different Calendars: The module supports various calendar formats, including the Gregorian calendar, which is the calendar system used by most of the world today.
Explore this amazing course and master all the key concepts of Python programming effortlessly!
Classes And Methods In The Calendar Module
The built-in Python calendar module contains several classes that provide additional functionality for handling calendar-related tasks. Here are the primary classes and their respective methods available in the calendar module:
Class Name | Description | Calendar Module Methods |
---|---|---|
Calendar | Provides core functionalities for creating and managing calendar instances. |
monthcalendar(year, month) |
TextCalendar | Generates plain text calendars for display in text-based environments (like consoles). |
formatmonth(year, month) |
HTMLCalendar | Generates calendars in HTML format for use in web applications. |
formatmonth(year, month) |
LocaleTextCalendar | A subclass of TextCalendar that supports localization features for generating text calendars according to locale. | Inherits methods from TextCalendar, with localization enhancements. |
LocaleHTMLCalendar | A subclass of HTMLCalendar that supports localization features for generating HTML calendars according to locale. | Inherits methods from HTMLCalendar, with localization enhancements. |
Prerequisites For Writing A Calendar Program In Python
Before we start with creating an interactive calendar application, there are some prerequisites that one must ensure:
- Basic Knowledge of Python: Familiarity with Python syntax, functions, and data types will help you understand the code more easily.
- Python Installed: Make sure Python (version 3.6 or higher) is installed on your system. You can download it from the official Python website.
- Integrated Development Environment (IDE): Use any IDE or text editor of your choice, such as PyCharm, Visual Studio Code, or even a simple text editor like Notepad.
- Familiarity with the Command Line: Basic knowledge of using the command line or terminal will be helpful for running your Python scripts.
- Understanding of Python Libraries: We will utilize the calendar module, which is a built-in library in Python, so no additional installation is required.
How To Write And Print A Calendar Program In Python
In this section, we will outline the general steps to create a simple calendar program using Python. Each step includes an explanation along with the necessary code snippets.
Step 1: Import The Calendar Module
To work with calendars in Python, we first need to import the calendar module. This built-in module provides functions to handle various calendar-related tasks.
import calendar
After this, create a function (e.g., display_calendar()) where the main logic of the program will reside.
Step 2: Get User Input For Year And Month
Next, we need to prompt the user to input the year and the month for which they want to display the calendar. We'll use the input() function to gather this information.
year = int(input("Enter the year (e.g., 2024): "))
month = int(input("Enter the month (1-12): "))
Step 3: Validate User Input
It's important to ensure that the user inputs valid values for both the year and the month. We can do this by checking if the month is between 1 and 12.
if month < 1 or month > 12:
print("Invalid month! Please enter a number between 1 and 12.")
Step 4: Display The Calendar
Once we have validated the inputs, we can use the built-in function month() from the calendar module to display the calendar for the specified month and year.
print(calendar.month(year, month))
Step 5: (Optional) Display The Whole Year Calendar
If you want to give the user the option to display the entire year's calendar, you can add a conditional check to ask if they would like to see it.
show_full_year = input("Would you like to see the full year calendar? (yes/no): ").lower()
if show_full_year == 'yes':
print(calendar.calendar(year))
Step 6: Add Error Handling
Implement a try-except block to handle potential errors, such as invalid input types (non-integers).
try:
# Input and validation code here
except ValueError:
print("Invalid input! Please enter numeric values for year and month.")
Step 7: Run The Program
Once you have all the components in place, you can invoke the main() function and run the program. Make sure to handle any exceptions that may arise, such as entering non-integer values for year and month.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Calendar Program In Python To Display A Month
To display a specific month of a calendar in Python, we will utilize the built-in calendar module, which provides functions to work with dates and calendars.
Code Example:
Output:
Explanation:
In the above Python code example-
- We start by importing the calendar module, which provides various functions to work with calendars in Python.
- Next, we define a function called display_month_calendar() that will handle the process of displaying a specific month’s calendar.
- Inside the function, we use a try block to attempt to get input from the user for the year and month.
- We prompt the user to enter the year, converting their input into an integer.
- Then, we prompt the user to enter the month, also converting this input into an integer.
- We then validate the month input by checking if it falls within the range of 1 to 12. If the input is outside this range, we print an error message and exit the function.
- If the input is valid, we use the calendar.month(year, month) function to generate and display the calendar for the specified month and year.
- If a ValueError occurs (e.g., if the user inputs a non-numeric value), we catch this exception and inform the user that they need to enter numeric values.
- Finally, we call the display_month_calendar() function to execute the code and display the calendar.
Calendar Program In Python To Display A Year
To display the entire calendar for a specific year in Python, we will make use of the built-in calendar module, which provides useful functions to manage and display calendars.
Code Example:
Output:
Explanation:
In the above Python code example-
- We start by importing the calendar module, which allows us to work with various calendar-related functions in Python.
- Next, we define a function called display_year_calendar() that will display the entire calendar for a specified year.
- Inside the function, we use a try block to safely attempt to retrieve user input for the year.
- We prompt the user to enter the year, converting their input to an integer.
- After getting the year, we call the calendar.calendar(year) function to generate the complete calendar for that year and print it to the console.
- If the user inputs a non-numeric value, a ValueError will be raised. We catch this exception and inform the user that they should enter a numeric value.
- Finally, we call the display_year_calendar function() to execute the code and display the yearly calendar for the specified year.
Searching for someone to answer your programming-related queries? Find the perfect mentor here.
Conclusion
Developing a calendar program in Python not only demonstrates the practical applications of the language but also highlights the versatility and ease of use provided by the built-in calendar module. Through our exploration, we learned how to display individual months and entire years, showcasing the module's ability to manage date-related functionalities efficiently. The step-by-step approach, from gathering user input to error handling, equips you with the essential skills to create robust applications. With this knowledge, you're well-equipped to confidently integrate calendar functionalities into your Python projects.
Frequently Asked Questions
Q. What is the purpose of the Python calendar module?
The calendar module in Python provides functions and classes to work with dates and calendars. It allows users to display formatted calendars, manipulate dates, and handle various calendar-related tasks efficiently, such as determining leap years and calculating the day of the week.
Q. How can I display a specific month using the calendar module?
To display a specific month, you can use the calendar.month(year, month) function. This function takes the desired year and month as parameters and returns the calendar for that month as a formatted string.
Q. Can I customize the starting day of the week in my calendar program?
Yes, you can customize the starting day of the week by using the setfirstweekday() method available in the calendar module. This method allows you to specify which weekday name(0 for Monday, 6 for Sunday) should be considered the first day of the week.
Here's how you can implement this in your cal program:
- Import the Calendar Module: Make sure to import the calendar module as usual.
- Set the First Weekday: Use the setfirstweekday() method to set your preferred starting day.
- Display the Calendar: After setting the first weekday, display the calendar using the relevant functions.
Code Example:
Output:
Enter the first day of the week (0=Monday, 6=Sunday): 6
Enter the year (e.g., 2024): 2024
Enter the month (1-12): 4
April 2024
Su Mo Tu We Th Fr Sa
1 2 3 4 5 6
7 8 9 10 11 12 13
14 15 16 17 18 19 20
21 22 23 24 25 26 27
28 29 30
Explanation:
In the above source code-
- The user is first prompted to enter a number between 0 and 6 to specify the first day of the week.
- The setfirstweekday() function is then called to set this preference.
- When we display the month using calendar.month(year, month), the output calendar will reflect the chosen starting day.
Q. Is it possible to display the entire year's calendar?
Yes, you can display the entire year's calendar using the calendar.calendar(year) function. This function returns a multi-line string that represents the complete calendar for the specified year.
Q. How can I handle user input errors when entering a year or month?
To handle user input errors, you can use a try-except block to catch ValueError exceptions that occur when the user enters non-integer values. Additionally, you can implement validation checks to ensure that the month is between 1 and 12, providing informative messages for any invalid inputs.
Q. Is it possible to save the calendar output to a file?
Yes, you can save the calendar output to a file by redirecting the printed output or writing the string returned by the calendar functions to a text or HTML file. For example, you can use Python's built-in file handling to write the output to a .txt or .html file.
Calendar Program In Python – Quiz Time!
QUIZZ SNIPPET IS HERE
QUIZZ SNIPPET IS HERE
QUIZZ SNIPPET IS HERE
This concludes our discussion on how to write a calendar program in Python. Here are a few other topics that you might be interested in reading:
- How To Reverse A String In Python? 10 Easy Ways With Examples!
- Relational Operators In Python | 6 Types, Usage, Examples & More!
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)