C++ Programming Language Table of content:
Defining Constant In C++ | Literals, Objects, Functions & More
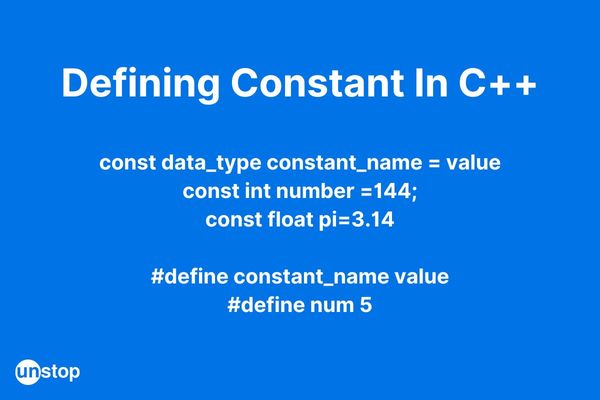
C++ is a powerful and versatile programming language that allows developers to work with various data types, enabling them to build complex and efficient applications. One of the key features of C++ language is the concept of constants. Constants are variables whose values remain fixed throughout the program's execution. In this article, we will explore the significance of constants in C++, their various uses, and how they contribute to code safety, readability, and performance optimizations. We will also get to know about literals, types of literals, and their use in a detailed manner.
What Is A Constant In C++?
In C++, a constant variable (const variable) is one whose value cannot be modified after initialization. In other words, these are values that cannot be changed during the program's execution. Constants are essential when we want to represent fixed or unchanging values in the program. And they can be of any basic data type depending upon the requirement of the program.
Unlike regular variables, the value of a constant remains the same throughout its lifetime. And any attempt to modify it will result in a compilation error. All in all, constants in C++ help improve code readability, maintainability and make it easier to update values in a centralized manner.
Ways To Define Constant In C++
There are multiple ways to define constants in C++ programming language. However, there are two most commonly used ones. One of these is by declaring a constant variable using the const keyword, followed by the data type and the variable name. And the other is by using the #define preprocessor.
In this section, we will look at these two methods of defining a constant in C++ and gather an understanding of the same with the help of examples.
Defining Constant In C++ Using The const Keyword
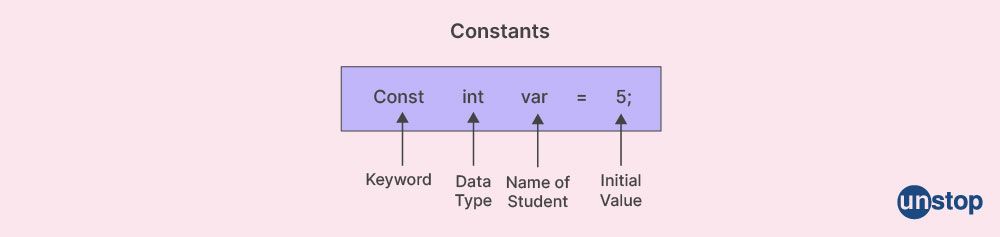
Using the const keyword is an extremely common method to declare a constant in C++. This keyword indicates that a variable's value cannot be changed once it is initialized. We can declare constants both locally as well as globally using the method. Given below is the syntax for defining a constant in C++ using the const keyword, followed by an example of the same.
Syntax:
const data_type constant_name = value
Here,
- const: It is the keyword (reserved name) used to define a constant in C++.
- data_type: It represents the data type of variable constant, either user-defined or primitive, such as int, float, char, double, long, string, etc.
- constant_name: It is the name given to the constant. Note that choosing a meaningful, constant name improves code readability and helps avoids unambiguity
- value: It is the constant's assigned value. The value has to work with the designated data type. Once allocated, it cannot be changed.
Example:
Output:
Integer number: 144
Floating point number: 3.14
Character: C
Explanation:
In the simple program example above,
- We declare and initialize three constant variables of different data types inside the main function.
- Here, the variable number is an integer constant with a value of 100, the variable pi is a floating-point constant with a value of 3.14159, and the variable ch is a character constant with the value of C.
- We then print these values on the console using the cout command.
Note that the value of the constant should be assigned at the time of its declaration failing so it produces an error. Also, attempting to modify a constant value later in the program will give you a compilation error.
Defining Constant In C++ With #define Preprocessor Directive
The #define is a preprocessor directive that is used to define constants in C++. It enables you to first give a name or alias to a value or a section of code. The preprocessor then replaces this with the defined value or section of code before the actual compilation of the program. We can define a constant in C++ globally via this approach.
Syntax:
#define Constant_name value
Here,
- #define: It is the preprocessor directive that indicates the definition of a macro or constant in C++.
- Constant_name: It is the name given to the constant or macro. It follows the same rules as naming variables, such as starting with a letter or underscore and consisting of letters, digits, or underscores.
- value: It is the value or code that will be substituted for the constant name wherever it appears in the program.
Example:
Output:
Area of the circle is: 78.5
Explanation:
- In the above example, we first declare and initialize a constant variable PI to the value of 3.14 using the #define directive.
- Now, whenever the preprocessor encounters PI in the code, it substitutes the value 3.14 in place of it.
- Next, we define a function to calculate the area of a circle called area_circle. This returns a floating type value, which is the product of PI with the square of the radius.
- In the main function, we then declare and initialize a floating type variable, radius, to the value of 5.
- The area_circle function is then called, and the output is printed using cout.
Notes:
- We do not use the assignment operator when using #define to initialize, as in the case of the const keyword. Nor does the preprocessor directive method need a specific data type.
- As per the comment in the above example, we could also use this method to name a section of code like ‘MAX’ to find the Maximum value between two numbers.
- Typically, the const keyword is recommended than the preprocessor directive as it offers better reliability, safety, and maintainability.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
What Are Literals In C++?
Literals are representations of values that are used directly in the code. They allow you to represent fixed values without the need for variables or computation. Literals give programmers a mechanism to directly express constant values. They are classified based on the data types.
Integer Literals
When integer variables are represented using a literal, they are known as integer literals. These are further divided into prefixes and suffixes.
Prefixes: Prefixes are used to specify the base or data type of the integer literal. For example, 0x or 0X for hexadecimal, 0 for octal, 0b or 0B for binary, and nothing for decimal. The common prefixes are:
- Decimal Literal: These Literals don’t have any prefix for representation, it has base 10 and contains values in the range from 0 to 9
- Octal Literal: The prefix 0 (zero) designates an octal literal. It has base 8, and the numbers that come after the zero are in the range 0–7
- Hexa Decimal Literal: The prefix 0x or 0X designates a literal in hexadecimal notation. The prefix can be followed by any number between 0 and 9, including A through F (or a-F). The base of the Hexa decimal literal is 16.
- Binary Literal: The prefix 0b or 0B designates a binary literal. Only 0 or 1 may follow the prefix in the numerals. The base of the binary literal is 2.
Example:
Output:
Decimal Literal: 143
Octal Literal: 11
Hexa decimal Literal: 31
Binary Literal: 10
Explanation:
The code example above demonstrates different types of integer literals in C++, i.e., decimal, octal, hexadecimal, and binary.
- Constants decimal, octal, hexadecimal, and binary are declared and initialized with integer literals: 143, 013, 0x1F, and 0b1010, respectively.
- Integer literals are representations of integers in different number bases: decimal (base-10), octal (base-8), hexadecimal (base-16), and binary (base-2).
- The code prints the values of these constants to the console, showing their corresponding literal evaluations using cout.
- The output displays the values: 143, 11, 31, and 10, representing the decimal, octal, hexadecimal, and binary evaluations, respectively.
Suffixes: Suffixes are used to specifically identify the data type of the integer literal. The literal size and type are affected by them. Common suffixes include the following:
Suffix | Description | Range |
int |
The default integer type takes both positive and negative values. | -2147483648 to 2147483647 |
unsigned in Suffix: u or U |
The unsigned integer takes only positive values | 0 to 4294967295 |
long int Suffix: l or L |
This represents a larger/ longer signed integer type in comparison to int. |
-2,147,483,648 to |
unsigned long int Suffix: ul or UL |
It expresses larger non-negative integers in comparison to an unsigned int. Takes only positive values. | 0 to 4,294,967,295. |
long long int |
Store signed integers that are even larger than long Int. | -(2^63) to (2^63)-1 |
unsigned long long int |
This represents non-negative integers larger in comparison to the unsigned long int type. |
0 to |
Example:
Output:
int: 10
unsigned int: 20
long int: 1000000000
unsigned long int: 5000000000
long long int: 9000000000000000000
unsigned long long int: 15000000000000000000
Explanation:
This example code demonstrates the usage of different integer data types and their respective integer literals in C++.
- Constants a, b, c, d, e, and f are declared and initialized with different integer literals of various types.
- Here, a is an int, b is an unsigned int, c is a long int, d is an unsigned long int, e is a long long int, and f is an unsigned long long int.
- The code prints the values of these constants to the console, along with their corresponding integer literals, using cout.
- The output displays the values of the constants printed on separate lines.
Floating Point Literals
These are used for the representation of decimal numbers with fractional portions. That is, they let you express any number with a decimal component and have basic forms- decimal and exponential.
Decimal Floating Point Literals:
- They represent numbers in the standard decimal format, consisting of digits, decimal points, and optional fractional parts. For example, 3.14, 0.5, 123.456.
- The decimal point separates the whole part from the fractional part. It is optional to include the leading zero before the decimal point. Also, the fractional part can be omitted if the number is an integer.
Exponential Floating point literals:
- They represent numbers in the form of a mantissa and an exponent in scientific notation. Exponential literals are useful when expressing extremely large/ small values.
- They are made up of a mantissa, an exponent indicator (e or E), and an exponent value. Examples include 1.23e-4 and 6.022e23.
- The exponent designates the power of 10 by which the mantissa should be multiplied. The e/ E symbol separates the mantissa and exponent.
Example:
Output:
Value of pi: 3.14
Value of an exponential number: 6.02e+20
Explanation:
We begin the example by including the entire C++ Standard Library for convenience.
- The program then defines a main() function as the entry point of the program.
- Next, a variable pi is declared and initialized with the value 3.14, using a decimal floating-point literal of type double.
- Another variable, exp_num, is declared and initialized with the value 6.02e20 using an exponential floating-point literal of type double.
- The program then prints the value of pi and exp_num along with a descriptive string to the console, using cout.
- The program ends by returning 0, indicating successful execution.
Boolean Literals
Boolean literals represent the truth values that are often used in conditional statements, logical expressions, and Boolean variables to control the flow of execution of a program.
- True: Represents the true state represented by any non-zero number in C++.
- False: Represents the false state, presented by zero number.
Example:
Output:
Unstop is Unstoppable
Unstop is efficient
Explanation:
- In the above example, two variables, var1, and var2, are initialized with constant boolean literals, true and false.
- We then start two if-else statements. For the first of these, since Var1 is true, the corresponding if statement is executed.
- For the second one, since var2 is false, the else statement is executed.
- Here since both var1 and var2 are constant boolean literals, the output will always be the same.
Character Literals
The character literals are used to represent individual characters in the programming languages. In C++, these consist of single characters enclosed in single quotes (' '). It represents a specific character from the character set, such as a letter, digit, symbol, or special character.
Character literals can be used to assign values to variables of type char, wchar_t, or other character-related types. They are commonly used when working with individual characters, strings, and character-based operations.
Narrow character literals (char): It represents the normal character and can take 0 to 256 values which correspond to the ASCII table. For example, the character ‘A’ has an ASCII value of 65.
Wide character Literals (wchar_t): It is the same as narrow character literals but requires twice the size. Hence, it can hold much larger values up to 65536, which corresponds to the New International Standard UNICODE table. They are followed by L and represent all types of languages. Its size is either 2 bytes or 4 bytes, depending on the compiler.
Example:
Output:
Letter: C
Digit: 9
Newline:
Wide character: 67
Explanation:
The code example uses the <bits/stdc++.h> library, which includes all standard C++ libraries. Next, using namespace std; allows the use of standard library functions without the std:: prefix.
- The main function is the starting point. Inside this, we declare and initialize four character variables.
- The character variable letter, digit, newline, and w, are assigned values of C, 9, \n, and L'C', respectively.
- The cout statements print the values (i.e., character literals) of the character variables.
- Finally, the program terminates with a return 0 statement.
String Literals
String literals are used to represent sequences of characters enclosed in double quotes. They allow you to express text, words, sentences, or any combination of characters as a single entity. Each character in string literal is stored in a consecutive memory location.
Example:
Output:
Hello, world!
Explanation:
The piece of code begins by including the <iostream> library for input/output operations.
- The main function is the starting point, just like in any other normal code/ program.
- Inside, we declare and initialize a string literal or character type using the const keyword.
- The string named greet is initialized by the value of "Hello, World!".
- Next, we use the cout object to print the value of the greet string to the console.
- The endl manipulator adds a new line after the output. The return 0; statement indicates successful program execution.
Now that we have explained how to define constants and the various types of literals, we will move on to explain the other uses of the const keyword in C++.
Pointer To A Constant In C++
To begin with, pointers are the variables that hold the memory location of another variable or constant.
- A pointer to a constant variable is when the data pointed to by the pointer is constant and cannot be altered through that pointer.
- However, the pointer is just a variable, so we can change the pointer to point different memory location
Syntax:
const data_type * ptr
Here,
- const is the keyword, and data_type refers to the type of constant variable.
- ptr is the name of the pointer
Example:
Output:
The value of the pointer is 20
Explanation:
- In the above example, we first declare in initialize two constant variables, num, and num1, to the values of 10 and 20, respectively. We use the const keyword here.
- Next, we declare an integer pointer, ptr, which points to the address of num, which has the value 10.
- Note any attempt to modify the value of num through the ptr will result in the compilation error, as mentioned in the comments in the program.
- We can, however, change the variable location to which it is pointing to. So we reinitialize the pointer and make it point to the location of num1.
- Since ptr now holds the location of num1, dereferencing it will give you the value of num1, i.e., 20. We print this value to the console using cout.
Also read- Dynamic Memory Allocation In C++ Explained In Detail (With Examples)
Constant Function Arguments In C++
Function parameters that are defined as constants and cannot be changed inside the function are known as constant function arguments. When a function parameter is marked as a constant, the function swears not to change the parameter's value.
These are especially useful when you want to ensure the caller of the function doesn’t change the original values of the arguments. It also helps avoid unnecessary copying of variables since constant variables are typically passed by reference.
Syntax:
return_type function_name(const type_name argument_name) {
// Function body
}
Here,
- The function_name refers to the name of the function.
- Terms return_type and type_name refer to the data types of the function's return value and constant argument, respectively.
- The const keyword specifies that the function argument is constant.
- The argument_name refers to the name of the function argument.
Example:
Output:
The number is: 15
Explanation:
- We begin by defining a function called printNumber, which takes the constant integer variable and prints its value using cout.
- Next, a constant variable value is declared inside the main function.
- This value is passed to printNumber function as a const argument, and the output is printed on the console.
Note that any attempt to change the value of num in the printNumber function will give you a compilation error.
Constant Member Function Of Class In C++
We say a class's member function is constant in C++ when it makes the commitment not to change the state of the object it is called on. When a member function is marked as const, it means that it won't change any of the class's non-static data members.
- Nonconstant functions are called with the help of non-constant objects only.
- And constant functions are called with any type of object.
Syntax:
data_type function_name() const
Here,
- The data_type refers t the type of the function's return value
- The name of the function is represented by function_name().
- And the const keyword specifies that the function is constant.
Example:
Output:
Radius: 5
Area: 78.5
Explanation:
The code first includes standard library headers using#include <bits/stdc++.h>
.
- It defines a C++ class called Circle to represent circles. The class has a private non-constant radius member variable to store the circle's radius.
- The class constructor takes a double parameter and initializes the radius member.
- Public member functions of the class include-
getRadius()
: Returns the circle's radius. Marked as const, indicating it doesn't modify the object's state.calculateArea()
: Calculates and returns the area of the circle using the formula3.14 * radius * radius
. Also marked as const.
- In the main() function, the program declares a constant class object c with a radius of 5.0.
- We then retrieve the circle's radius using
getRadius()
and store it in the radius variable. - Next, we calculate the circle's area using
calculateArea()
and store it in the area variable. Due to c being a constant object, attempting to modify its state (e.g.,c.radius = 10.0;
) will result in a compilation error. - Lastly, we print the circle's radius and area to the console using cout.
- The program returns 0 to indicate successful execution.
Constant Data Members In C++
A constant data member of a class is a member variable that is declared as const and cannot be modified once it is initialized. The value of a constant data member remains the same throughout the lifetime of the object. This helps maintain consistency and the integrity of an object.
Syntax:
const data_type name;
Here,
- The const keyword is used to specify that the data member is constant.
- The data_type and name refer to the member's type and its name.
Example:
Output:
Area: 78.5397
Radius: 5
Explanation:
- The code defines a C++ class called Circle to work with circles.
- The class has a private constant PI with a value of 3.14159 and a private constant radius.
- The class constructor accepts a double parameter for the circle's radius and initializes the radius member.
- Public member functions:
calculateArea()
: Calculates and returns the area of the circle usingPI * radius * radius
.getRadius()
: Returns the circle's radius.
- In the main() function, we create a circle class object c with a radius of 5.0.
- Next, the area of the circle is calculated using
calculateArea()
and stored in thearea
variable. - Then the program retrieves the circle's radius using
getRadius()
and stores it in the radius variable. - The output of the calculated area and radius are printed to the console using cout.
- The program returns 0 to indicate successful execution.
Object Constant In C++
Just like in the case of C++ constant variables, we can also declare a constant object of a class using the const keyword. But note that objects that are constant can only be used to call constant member functions. However, the non-constant object can be used to call both const member functions and non-const member functions.
Syntax:
const type_name object_name;
Here,
- Const signifies that any object created with this keyword remains constant.
- Terms type_name and object_name refer to the name of the class, and the constant object, respectively.
Example:
Output:
The area is 15
Explanation:
- The constructor of the Rectangle class in the above example accepts two integer inputs, l and w, which are used to initialize the length and width variables, respectively.
- Using the const keyword, the calculateArea() function is designated as const. This means that it can be safely called on const objects because it does not change the object's state.
- As mentioned in the comments, compilation issues will occur if the non-const member functions setLength() and setWidth() are used on the const object r.
- This is so that they can't be called on const objects because they change the state of the object.
- CalculateArea() can be called on const objects without any problems because it is tagged as const.
- In the main function, we create a const variable/ constant class object, r, with parameters 3 and 5.
- The program then calls the calculateArea() function, and the output it displayed using cout.
Conclusion
Constant in C++ are values that cannot be modified once assigned, providing immutability and boosting code readability, maintainability, and safety.
- Constant in C++ help to ensure program integrity by preventing accidental modification of values. Also, constants with explicit data types provide type safety, preventing unnecessary type conversions or assignments.
- There are two ways to declare a constant in C++. One is the use of the const keyword, and the other is the #define directive.
- The latter is used to define a constant which is then replaced by the preprocessor. However, the keyword approach is usually preferred over this approach.
- A literal (constant literal) refers to the value stored in a variable (constant variable). Literals allow you to represent values directly in your code. They thus help eliminate the need for complex expressions or intermediate variables.
There are many other uses of the keyword constant in C++, like for declaring constant objects, member functions, data members, and more.
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Quiz Time!!!
QUIZZ SNIPPET IS HERE
QUIZZ SNIPPET IS HERE
QUIZZ SNIPPET IS HERE
Frequently Asked Questions(FAQ)
Q. Can constants be initialized with runtime values?
No. In constant in C++ must be initialized with values known at compile-time only. In other words, the value assigned to a constant variable must be a constant expression to be evaluated by the compiler during the compilation process.
A constant expression, in this sense, refers to an expression that consists of literals, other constants, and operations that can be resolved at compile-time. For example, arithmetic operations involving only literals or other constants, bitwise operations, etc.
Example of constant expression:
2+3 (arithmetic)
(5|2)&3 (bitwise)
“abc”+”def” (string concatenation)
Q. How are constant expressions evaluated?
Constant expressions in C++ are expressions that can be evaluated at compile-time rather than runtime. These expressions are determined entirely by their values and do not depend on any runtime information. Constant expressions are used in various contexts, such as initializing constants, specifying array sizes, and more, to ensure compile-time optimization and safety.
Constant expressions are evaluated using a combination of the following rules:
- Literals: Literal values like integers, floating-point numbers, and characters are themselves constant expressions.
- Arithmetic Operations: Basic arithmetic operations (+, -, *, /) involving constant values are evaluated at compile-time. For example, 3 + 4 is a constant expression.
- Logical and Bitwise Operations: Logical (&&, ||) and bitwise (&, |, ^) operations involving constant operands are evaluated at compile-time. For example, true && false is a constant expression.
- Comparisons: Comparison operations (==, !=, <, <=, >, >=) between constants are evaluated at compile time. For example, 5 < 10 is a constant expression.
- Conditional Expressions (Ternary Operator): Conditional expressions (ternary operator) involving constant conditions are evaluated at compile-time. For example, true ? 1 : 0 is a constant expression.
- The sizeof Operator: This is applied to a type or object as a constant expression. For example, the sizeof(int) is a constant expression.
- Enum Values: Enum values are constants and can be used in constant expressions.
- Compile-Time Constants: Constants defined with const or constexpr qualifiers are evaluated at compile-time when used in constant expressions.
- Function Call: If a function is declared as constexpr and meets certain criteria, its calls with constant arguments can be evaluated at compile-time.
- Templates: Template metaprogramming techniques can allow for compile-time evaluation of expressions.
Q. Difference between constant and variable in C++?
Aspect | Constant | Variable |
---|---|---|
Declaration | Declared using const keyword. | Declared without const keyword. |
Value | Value cannot be changed once initialized. | Value can be changed after initialization. |
Initialization | It must be initialized at the time of declaration. | It can be initialized at the time of declaration or later. |
Memory Allocation | It can be stored in read-only memory (e.g., ROM). | Stored in regular memory (e.g., RAM). |
Usage | Useful for representing fixed or unchanging values. | Useful for storing data that can change during program execution. |
Syntax | Example: const int myConstant = 10; |
Example: int myVariable = 5; |
Pointer to | A pointer to a constant variable (e.g., const int* ptr; ) can be used. |
A regular pointer (e.g., int* ptr; ) can be used. |
Modification | It cannot be modified through the constant identifier. | It can be modified through the variable identifier. |
Q. How do you declare a constant using #define?
The preprocessor directive, #define, is one of the two ways to declare constant values in C++. We can also provide a macro for a block of code by using this preprocessor command
Syntax:
#define Name value
Example:
#define num 5
In this example, during the preprocessing stage, the preprocessor replaces each instance of num in the code with the value 5. In this sense, #define simply performs textual substitution, and it is important to note that it lacks a specified data type.
Q. Can literals be assigned to constant variables?
Yes, literals can be assigned to constant variables. Constant in C++ ensures that the assigned value remains unchanged throughout the program execution. For example, const int num = 99; assigns the integer literal 99 to the constant variable num.
Q. How do you write a constant in C++?
Constant in C++ can be defined in two ways, as shown in the table below:
Using Const keyword | Using #define Preprocessor |
The use of the constant keyword is the primarily used method of defining a constant in C++. Once a value is assigned, it cannot be altered during execution. | The preprocessor #define directive is another way to define constants in C++. It performs textual substitution, replacing occurrences of the defined identifier with the specified value during the preprocessing stage. |
Syntax: |
Syntax: #define Name value |
Q. Why do we need literals?
Literals are crucial in programming since they are a means to represent the values assigned to a constant in C++. Here are some advantages of literal:
- Direct value expression: Literals let you write integers, characters, or strings to directly express values in your code.
- Easy initialization of variables: Literals make it simple to initialize variables with constant values without the need for intricate calculations or expressions. Your code becomes more understandable as a result.
- Constant definition: Literal constants are frequently used in your code to declare constants. You can guarantee a program's integrity by explicitly stating that a constant variable's value should never change by giving a literal value to it.
- Making your code readable: Using literals improves the readability and clarity of your code. The values you use don't need to be deduced from complicated expressions or calculations for anyone viewing your code.
Q. Can literals be used in expressions?
Yes, literals can definitely be used in expressions in programming languages. In programming, literals are fixed values that are directly written into the code. They represent constants of various types, such as integers, floating-point numbers, characters, strings, and more. These literals can be used in various expressions to perform operations, comparisons, assignments, and other computations.
Here are a few examples of how literals can be used in expressions:
-
Arithmetic Expressions:
-
int sum = 5 + 3;
Here,5
and3
are literals used in an addition expression. -
double result = 10.0 * 2.5;
In this case,10.0
and2.5
are literals used in a multiplication expression.
-
-
Comparison Expressions:
-
bool isGreater = 7 > 4;
Here,7
and4
are literals used in a greater-than-comparison expression. -
bool isEqual = 'A' == 65;
The character literal'A'
and the integer literal65
are used in an equality comparison expression.
-
-
String Concatenation:
-
string fullName = "John" + " " + "Doe";
The string literals"John"
," "
, and"Doe"
are used in a concatenation expression.
-
-
Array Initialization:
-
int numbers[] = {1, 2, 3, 4, 5};
Integer literals are used to initialize the elements of an array.
-
-
Character Operations:
-
char firstLetter = 'A';
The character literal'A'
is assigned to a variable. -
char nextLetter = firstLetter + 1;
The value offirstLetter
(which is'A'
) is used in an arithmetic expression.
-
You might also be interested in reading the following:
- New Operator In C++ | Syntax, Usage, Working & More (With Examples)
- Guide To Switch Case In C++ & Important Keywords (With Examples)
- Storage Classes In C++ & Its Types Explained (With Examples)
- 2D Vector In C++ | Declare, Initialize & Operations (+ Examples)
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)