Java Programming Language Table of content:
Thread Priority In Java | Constants, Limitations & More (+Examples)
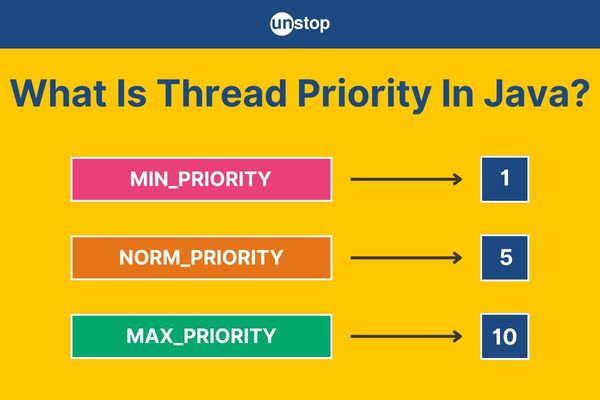
In Java, threads are the backbone of multitasking, enabling programs to execute multiple tasks simultaneously. But what happens when certain tasks need to be prioritized over others? That's where thread priority comes into play. Thread priority helps us decide the relative importance of threads during execution. In this article, we will explore what thread priority is, how it works, and how to use it effectively in Java to manage multitasking efficiently.
What Is Thread Priority In Java?
A thread is a lightweight subprocess in a program, allowing tasks to be performed concurrently. When multiple threads are in a runnable state, the system's thread scheduler decides which thread gets the CPU time. This decision is influenced by each thread's priority.
- Thread priority is a numeric value assigned to a thread to indicate its relative importance compared to other threads.
- Range: The thread priority values range from 1 (MIN_PRIORITY) to 10 (MAX_PRIORITY), with 5 (NORM_PRIORITY) as the default.
- It helps the thread scheduler decide which thread should run next when multiple threads are ready to execute.
Why Is Thread Priority Needed?
Thread priority in Java programming is important for the following reasons:
- Managing Critical Tasks: By assigning higher priority to certain threads, you can ensure that critical tasks (like real-time processing or urgent computations) are executed before less important tasks.
- Optimizing Performance: Thread priority can help optimize the performance of applications by allowing time-sensitive tasks to get more CPU time, thus improving efficiency.
- Controlling Resource Allocation: It helps in controlling the allocation of system resources, ensuring that high-priority threads get access to resources faster than lower-priority threads.
- Improving Responsiveness: Assigning appropriate priorities helps maintain the responsiveness of applications, especially in multi-threaded environments where multiple tasks are running concurrently.
- Enabling Real-time Behavior: For real-time applications, thread priority is crucial to ensure time-critical tasks are given precedence over regular tasks.
Explore this amazing course and master all the key concepts of Java programming effortlessly!
Built-In Thread Priority Constants In Java
Thread priorities in Java define the relative importance of a thread and guide the thread scheduler in determining which thread to run first when multiple threads are in a runnable state. Java provides three predefined thread priority constants:
1. Thread.MIN_PRIORITY (1)
This is the lowest priority a thread can have. Threads with this priority are treated as the least important by the scheduler. They are typically used for non-urgent tasks or background operations.
- Use Case: Performing low-priority tasks such as logging, cleanup operations, or background data syncing.
- For Example:
Thread t1 = new Thread();
t1.setPriority(Thread.MIN_PRIORITY); // Setting the lowest priority
2. Thread.NORM_PRIORITY (5)
This is the default priority for all threads unless explicitly changed. Threads with normal priority have equal importance and are treated fairly by the scheduler. Most application tasks should run with this priority to maintain balance.
- Use Case: General-purpose tasks, such as processing user input or handling regular computations.
- For Example:
Thread t2 = new Thread();
System.out.println(t2.getPriority()); // Prints 5 (default)
3. Thread.MAX_PRIORITY (10)
This is the highest priority a thread can have. Threads with this priority are considered the most important and are given preference over lower-priority threads. However, excessive use of high-priority threads can lead to starvation of lower-priority threads.
- Use Case: Critical or time-sensitive tasks, such as real-time data processing or emergency actions.
- For Example:
Thread t3 = new Thread();
t3.setPriority(Thread.MAX_PRIORITY); // Setting the highest priority
Thus, to summarize:
Priority Constant |
Value |
Purpose |
Example Use |
Thread.MIN_PRIORITY |
1 |
Least important thread |
Background tasks, logging |
Thread.NORM_PRIORITY |
5 |
Default priority for threads |
Regular tasks, user interactions |
Thread.MAX_PRIORITY |
10 |
Most important thread |
Critical, time-sensitive operations |
By assigning appropriate priorities, we can optimize thread execution and ensure that critical tasks are handled with the right level of importance.
Let’s have a look at a code example that demonstrates how to access and print the thread priority constants in Java-
Code Example:
Output (set code file name as MyThread.java):
Thread Running…
Max thread priority: 10
Min thread priority: 1
Normal thread priority: 5
Explanation:
In the above code example-
- We define a class MyThread that extends Thread and overrides the run() method to print "Thread Running..." when executed.
- In the main() method, we create an instance of the MyThread class called p1.
- We start the thread by calling p1.start(), which triggers the run method to execute.
- After starting the thread, we print the constants for thread priorities:
- Thread.MAX_PRIORITY represents the maximum priority (10).
- Thread.MIN_PRIORITY represents the minimum priority (1).
- Thread.NORM_PRIORITY represents the normal priority (5).
- The thread execution prints "Thread Running..." to the console, but the order of execution and printing the priority constants may vary depending on the thread scheduler.
Note: Other programming languages and operating systems may define priority ranges differently. Threads with higher priority are more likely to get CPU time before threads with lower priority. However, thread priority is not a guarantee; it is only a suggestion to the scheduler. Factors such as OS implementation and thread scheduling policies (e.g., preemptive or time-slicing) affect the execution order.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Thread Priority: Setter & Getter Methods
In Java, thread priority can be managed using two methods provided by the Thread class:
1. The setPriority(int priority) Method
This method is used to assign a priority to a thread. The priority parameter should be an integer between Thread.MIN_PRIORITY (1) and Thread.MAX_PRIORITY (10). If an invalid value is provided, the method throws an IllegalArgumentException.
Syntax:
public final void setPriority(int newPriority)
Here:
- public: Access modifier that allows the method to be called from any class.
- final: Modifier that prevents the method from being overridden by subclasses.
- void: Return type indicating that the method does not return any value.
- setPriority: The method name used to assign a priority to a thread.
- int newPriority: Parameter that specifies the new priority of the thread. Acceptable range: 1 (Thread.MIN_PRIORITY) to 10 (Thread.MAX_PRIORITY). Throws an exception if the value is invalid.
2. The getPriority() Method
This method retrieves the priority of a thread. It returns an integer value representing the priority of the thread.
Syntax:
public final int getPriority()
Here:
- public: Access modifier that allows the method to be called from any class.
- final: Modifier that prevents the method from being overridden by subclasses.
- int: Return type indicating the method returns an integer value (the thread's priority).
- getPriority: Method name used to retrieve the current priority of a thread.
Let’s look at a code example to understand how these methods work in Java-
Code Example:
Output (set code file name as ThreadPriorityExample.java):
Thread-0 running with priority: 1
Thread-2 running with priority: 10
Thread-1 running with priority: 5
Priority of Thread-0: 1
Priority of Thread-1: 5
Priority of Thread-2: 10
Explanation:
In the above code example-
- We define a class PriorityDemo that extends Thread, overriding the run() method to print the name and priority of the current thread.
- In the run() method, we use Thread.currentThread() to fetch the current thread object and print its name and priority.
- In the main() method of the ThreadPriorityExample class, we create three thread objects: t1, t2, and t3.
- We then set the priorities for each thread using the setPriority() method: t1 gets the minimum priority (1), t2 gets the normal priority (5), and t3 gets the maximum priority (10).
- We start the threads using the start() method, which triggers the run method execution for each thread.
- Finally, we use getPriority() to retrieve and display the assigned priorities of t1, t2, and t3.
- Thread priorities may influence the order of execution but do not guarantee it, as thread scheduling depends on the JVM and the underlying operating system.
Limitations Of Thread Priority In Java
Thread priority provides a mechanism to influence thread scheduling, but it comes with limitations that can affect consistency and reliability. Here are some of the common limitations of thread priority in Java:
- Platform Dependency: Thread priority handling is dependent on the underlying operating system. Some OS schedulers may ignore Java thread priorities entirely.
- No Guarantee of Execution Order: Setting a higher priority does not guarantee that the thread will execute before lower-priority threads.
- Ineffective in Certain Scheduling Policies: On systems using time-slicing or fair scheduling policies, all threads may get equal CPU time regardless of their priority.
- Risk of Starvation: Overuse of high-priority threads can lead to starvation of lower-priority threads if the scheduler heavily favors higher-priority threads.
- Complex Debugging: Prioritizing threads can make the program’s behavior unpredictable and challenging to debug, especially in multi-threaded environments.
- Not Suitable for Fine-Grained Control: Thread priorities are coarse and may not provide the level of control needed for real-time or highly concurrent applications.
- Inconsistent Behavior Across JVMs: Different JVM implementations might handle thread priorities differently, leading to inconsistent behavior on different platforms.
Are you looking for someone to answer all your programming-related queries? Let's find the perfect mentor here.
Best Practices For Using Thread Priority In Java
You can optimize thread performance by following these best practices for using thread priority in Java effectively:
- Stick to Default Priority: Use the default Thread.NORM_PRIORITY (5) for most threads to maintain fairness and avoid unnecessary complexity.
- Reserve High Priority for Critical Tasks: Assign Thread.MAX_PRIORITY (10) only to threads performing time-sensitive or mission-critical operations.
- Avoid Starvation: Ensure that lower-priority threads are not starved by limiting the number of high-priority threads.
- Test Across Platforms: Since thread priority handling is platform-dependent, test your application on all target environments.
- Combine with Proper Synchronization: Use synchronization mechanisms alongside priorities to avoid race conditions and ensure thread safety.
- Minimize Priority Changes: Avoid frequently altering thread priorities during runtime to maintain predictable behavior.
- Document Priority Usage: Clearly document why certain threads are assigned specific priorities for better code maintainability.
- Use Priority as a Hint: Treat thread priority as a scheduler suggestion, not a strict execution order guarantee.
Conclusion
Thread priority in Java plays a vital role in controlling the execution order of threads, offering a way to manage critical tasks and optimize resource allocation. While it can help improve performance and responsiveness, it's important to remember that thread priority is a suggestion to the thread scheduler and not a guarantee, as its behavior is platform-dependent.
By using thread priority thoughtfully and adhering to best practices, you can ensure that your multi-threaded applications run efficiently without causing issues like thread starvation. Always consider testing across different environments and using thread priority alongside other synchronization techniques for optimal results.
Frequently Asked Questions
Q. What is the default thread priority in Java?
The default thread priority in Java is Thread.NORM_PRIORITY, which is set to a value of 5. If no priority is explicitly set, threads will have this default priority.
Q. Can the thread priority guarantee the order of thread execution?
No, thread priority does not guarantee the order of execution. It is simply a suggestion to the thread scheduler, which may or may not honor it depending on the underlying operating system's scheduling policy. The thread with higher priority is generally favored, but it is not assured that it will always run before lower-priority threads.
Q. How can I set a thread’s priority in Java?
You can set a thread's priority using the setPriority(int priority) method of the Thread class. The priority value should be between Thread.MIN_PRIORITY (1) and Thread.MAX_PRIORITY (10). For example-
Thread t = new Thread();
t.setPriority(Thread.MAX_PRIORITY);
Q. What happens if I set a thread priority outside the valid range (1-10)?
If you set a thread's priority outside the valid range, Java will throw an IllegalArgumentException. You must ensure the priority value is between Thread.MIN_PRIORITY (1) and Thread.MAX_PRIORITY (10).
Q. Does the Java Virtual Machine (JVM) strictly follow thread priorities?
No, the JVM does not guarantee strict adherence to thread priorities. The actual behavior of thread scheduling is highly dependent on the underlying operating system’s thread scheduler, which may choose to ignore thread priorities entirely or use them as a hint for scheduling.
Q. Can thread priorities cause starvation in Java?
Yes, thread priorities can lead to starvation. If there are many high-priority threads running, low-priority threads may never get a chance to execute, as the system favors higher-priority tasks. This can be mitigated by carefully managing the number of high-priority threads or adjusting their priorities.
Q. How can I avoid issues with thread priority in Java?
To avoid issues like starvation or unfair scheduling, follow best practices such as:
- Use thread priorities only when necessary, and reserve high priority for critical tasks.
- Ensure that low-priority threads still get enough CPU time.
- Test your application across different operating systems to account for platform-dependent thread scheduling.
- Use proper synchronization techniques to ensure thread safety, regardless of thread priorities.
With this, we conclude our discussion on the thread priority in Java. Here are a few other topics that you might be interested in reading:
- Final, Finally & Finalize In Java | 15+ Differences With Examples
- Super Keyword In Java | Definition, Applications & More (+Examples)
- How To Find LCM Of Two Numbers In Java? Simplified With Examples
- How To Find GCD Of Two Numbers In Java? All Methods With Examples
- 10 Best Books On Java In 2024 For Successful Coders
- Difference Between Java And JavaScript Explained In Detail
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)