Git Commands | Complete Guide From Basics To Advanced (+Examples)
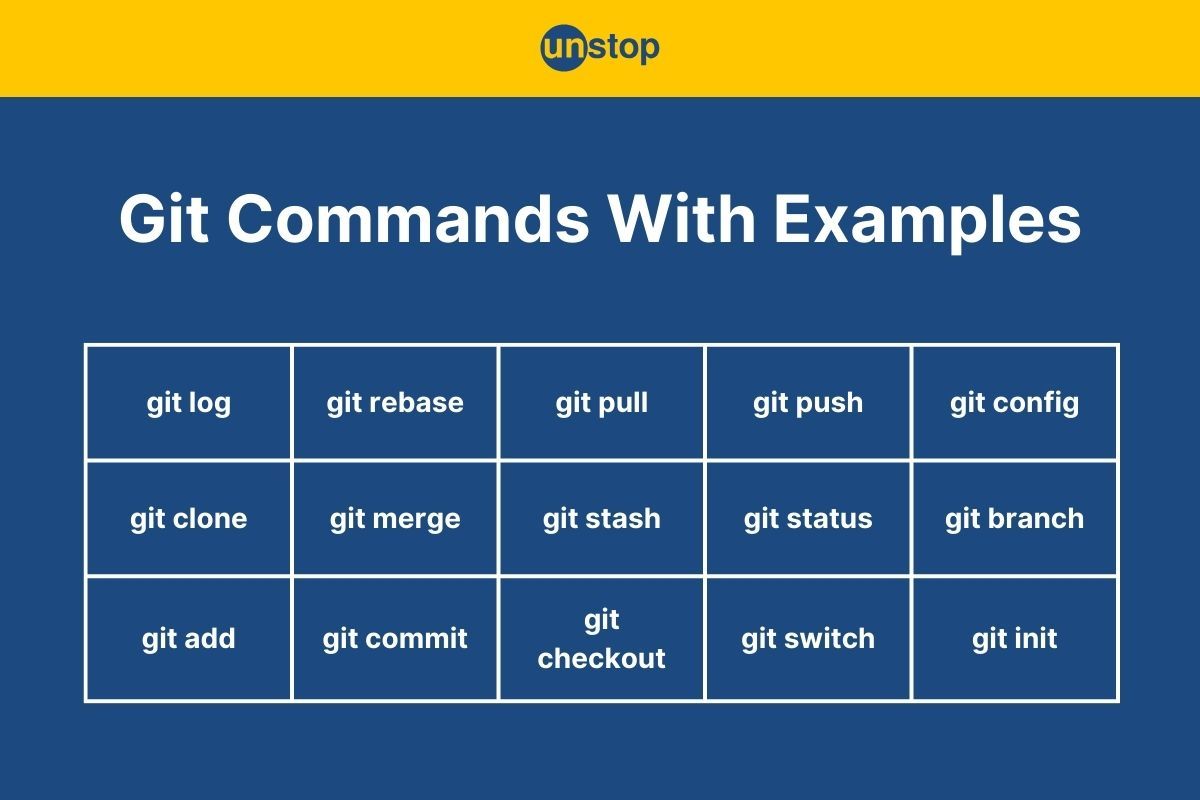
If you’re looking for a way to collaborate with other software developers remotely and want to stage the changes made to your code base, then Git would be the most practical tool for you. Git, a powerful version control system, is equipped with a plethora of Git Commands that will help you move through repositories, commit changes, origin branch out, merge code, and do much more. Git Commands can be daunting at first, but don’t worry! With the help of this article, we will guide you to transit from a complete noob to a Git Hero.
What Is Git?
Git is a free, open-source version control system widely used in software development. It is a powerful tool that operates in a distributed manner, making it easy for several developers to work on a single project simultaneously and individually.
- Each developer can copy the whole project on their local server, including its complete commit history and branches.
- Git is also easily integrated with platforms like GitHub, GitLab, and Bitbucket.
- Think of it like this- You and your friend want to write a story as a group project. Here, Git will help you maintain all the changes made to your story and revert back to any version when required.
- In other words, Git allows the developers to keep track of the changes made to the file at a specific time through Git commits.
- This enables the developers to experiment with the code base, isolate the changes, and merge them back to the main branch. The diagram below showcases how Git actually works.
We have prepared a list commands that every user must be familiar with. For example, git init, git clone, git branch, git checkout, git add, git commit, git push, git pull, etc., help manage the code repositories. But before we discuss these commonly used Git commands, you must be familiar with the two main types of repositories you will work with when using Git.
Local Repositories
The local repositories are those repositories that serve as a personal workspace. These are located on your computer and contain all the files and folders related to your work. This is very beneficial as they allow you to work on projects offline as well, which means making changes and tracking the progress without any internet connection.
Remote Repositories
The remote repositories are those repositories that do not exist on your local computer. Instead, they exist on another server elsewhere, typically hosted on platforms like GitHub, GitLab, or Bitbucket. It is also referred to as a server repository, which doubles up as a Hub for collaboration among the developers and team leaders. The developers can push the changes to the main repository and pull the latest changes from other developers.
Let’s dive deep into the important Git commands used in local and remote repositories.
Git Commands: Working With Local Repositories
First and foremost, let’s learn how to use Git Commands on your local computer. For that, go through the following steps-
- Open your terminal or command prompt.
- Navigate to the local repository directory with the help of the cd command.
- Initialize a Git repository if needed.
- Run the Git commands.
Now that you know how to open Git, let's look at some basic Git commands used in the local repositories.
- git init: The git init command is used to initialize a new empty Git repository into your system. Whenever you run the command, Git creates a new repository equipped with the necessary files and directories to track the changes in your project. The Git creates the new hidden directory as .git.
Syntax: git init
Example:
- git add: The git add <file(s)> command is used to add particular file(s) or changes in the directory. This command is used to stage changes in the code base. It can also be used to see the differences between the changes made in the working directory and the staging area.
Syntax: git add <file(s)>
Example: The command in the snippet below will add the file to the staging area. You can also add multiple files at the same time.
- git commit: In order to maintain a well-documented and organized history, it is important to commit changes to the project regularly. This can be done with the help of the git commit command, which helps create a new commit in Git with an explanatory commit message. This represents the snapshots of the changes you’ve made to your repository.
Syntax: git commit -m “<commit-message>”
Example:
- git status: This command displays the status of your Git repository at the moment. Having knowledge of the files that have been changed and edited, the files that are ready for the next commit, and the untracked files is helpful. It instantly verifies if the changes are correctly tracked and committed or not, checking the state of your repositories.
Syntax: git status
Example Of Git Status Command:
- git config: The user.name and user.email are initially set up using the git config command. This details the login and email address that will be utilized from a local repository. You can use it to configure your Git environment by setting and viewing various configuration parameters.
Syntax: git config
Example: In this example, we set the username and user email.
- git branch: This command is used to help organize branches in a repository. It helps organize branches in a repository by listing, deleting, or creating branches. It is known to be a flexible command order to help manage or remove different development stages within the Git project function.
Syntax: git branch
Some commonly used options are:
- -a or - -all: Lists both local and remote branches.
- -d or - -delete: Deletes a branch.
- -m or - -move: Renames a branch.
Example: The example below shows how to create a new branch, list all branches, and delete that branch.
- git checkout: This command is helpful while switching between the branches, creating new branches, or regaining any files from a commit. The git checkout can be useful for other reasons, such as to check out tags or a specific commit. However, one must be careful when using this command, as it can reject changes directly in your work.
Syntax: git checkout <branch-name>
Example: In this example, we check out the New-Branch and create a new branch named New-New-Branch.
- git merge: This command is used to merge changes from one branch to another (or, say, to an active branch). This helps interconnect any changes made in the current branch directly into the main branch. It's crucial to remember that joining branches may cause conflicts if the changes occur simultaneously.
Syntax: git merge <branch-name>
Example:
Git Commands: Working With Remote Repositories
Before starting with the commands used in remote repositories, let us understand how to run the Git commands on a remote repository. Follow these steps to run your remote repository:
- First, clone the remote repository on your local system.
- Now, navigate to the local repository created by cloning.
- Configure the remote repositories (This is optional).
- Fetch the latest changes onto your local repository.
- Make appropriate changes to your local repository.
- Now, push all these changes to the remote repository.
- At last, pull all the changes from the remote repository.
There are various commands used for interacting with remote repositories. Let’s look at some commonly used Git commands for working with remote repositories.
- git remote: This command helps add the new repository to your Git configuration by allowing you to establish a connection between your local repository and the remote repository. It also lets you fetch, push, and pull changes between them. In this command, we can replace <name> with a nickname for your repository and <repository-url> with the repository URL.
Syntax: git remote add <name> <repository-url>
Example:
- git clone: The basic git clone usage is creating a local replica of a remote repository onto your computer and downloading the entire project’s history, branches, and files. This enables us to work with the remote repository locally. The <repository-url> specifies the URL of the remote repository.
Syntax: git clone <repository-url>
Example:
- git pull: This command fetches the latest changes made on the remote repository and merges them into your local branch. This also updates the local branch with the recent commits from the specified <branch-name> on the <remote> repository, like the push command.
Syntax: git pull <remote> <branch-name>
Git pull usage example:
- git push: This command is used to upload and push your local commits to a remote repository. It also updates the specified <branch-name> on the <remote> repository with the local changes.
Syntax: git push <remote> <branch-name>
Example:
Branch- Git Commands
A Git branch command enables the user to create and modify branches. It prohibits switching between branches and can also be perceived as a branch management tool enabling users to create, delete, or rename branches.
Following are some commonly used Git branch commands:
- git branch: Shows a list of the local branches present in your git repository.
- git branch -a: Shows a record of local as well as remote branches present in Git repository.
- git branch -c: Copy a Git branch (Note: This command does not exist in Git).
- git branch -d <branch-name>: Remove a local Git branch. This command is rendered useless if the branch to be deleted has unmerged corrections.
- git branch -m <branch-name> <new-branch-name>: Rename a Git branch.
- git branch -r: Show a record of the remote branches present in your Git current repository.
Git Checkout Commands
The Git checkout command enables the user to shift between various branches of a project. They enable the user to explore the different branches of the project, thus improving their ability to make changes.
Some commonly used Git checkout commands:
- git checkout <branch-name>: Shift to a different Git branch from the master branch.
- git checkout -b <branch-name>: Shift to a new branch upon its creation.
An example for both is:
- git checkout -b <branch-name><remote-name>/<branch-name>: Make a local branch from the remote Git branch and explore it.
- git checkout <commit hash>: Checkout a previous Git commit.
Merge- Git Commands
Git merge helps the user to incorporate the changes made in one branch into another. It's a method of bringing different changes together, where all the corrections from different branches are combined into a single unit.
Various git merge commands include:
- git merge: Merges multiple development histories together. Usually used along with the Fetch command.
- git merge <branch-name>: Merge changes from one branch into the branch you currently checked out.
An example for both:
- git merge --abort: Abolishes the merge process and restores the project to its former state. It is used as a safety check.
- git merge --continue: Try to finish a merge that was stopped due to file conflicts post-resolution.
Clone- Git Commands
A Git clone command makes a local copy of a project from a remote repository. It's similar to xeroxing a book, enabling you to have your own version to use without affecting the original.
Some popular Git clone commands are:
- git clone <repository-url>: Replicates a specified remote repository.
- git clone <repository-url> <directory-name>: Replicates a repository and name the local directory.
- git clone <repository-url> --origin <name>: Clones a repository and name the remote (<name>).
Commit- Git Commands
Git commit command saves changes made by the user in Git by making a checkpoint in the committed project history. It can be likened to taking a snapshot of your work, enabling the user to refer back to the changes later if needed.
Some popular and helpful git commands are:
- git status: Shows a command record of files in the staging directory with corresponding file status.
- git add: Stages file changes. Running this command with the corresponding file name will stage the file changes to your staging directory.
- git commit -m: Adds a Git commit message. The message is supposed to be added in quotation marks following the command.
Config- Git Commands
Git config commands lets the user configure Git settings, like name, email, etc. It's a method of personalizing Git as per the user's needs and persona.
Some popular Git config commands are:
- git config --global: Personalized configurations stored in the user's home directory.
- git config --user.email: Sets up the email associated with various Git actions.
- git config --user.name: Sets the username associated with various Git commits and actions.
- git config --list: Provides a comprehensive view of various Git config settings like local, global, and system levels.
This example includes git config --user.email, git config --user.name, and git config --list.
- git config --local: Customizes Git repository's specific settings and overwrites Git configurations on the global and system level. The local is an option just like global.
The example looks similar to git config --global.
Pull- Git Commands
When you use git pull, you fetch the newest updates made by others in the project and merge them into your own version. It's like syncing the local copy of a document with the most recent changes your teammates or collaborators have made. This helps ensure the user has the most up-to-date version to continue working on.
Some popular git pull commands include:
- git pull: Combines fetching updates from another repository or branch and integrating them into the user's own.
- git pull --quiet: Performs the pull operation silently without displaying unnecessary text output after fetching and merging.
- git pull --verbose: Provides more detailed text output after the fetch and merge steps. Gives the user a clearer view of what changes were retrieved and integrated into the project.
For both commands, it will refuse to merge if they are unrelated in the system like in the example shown below.
Push- Git Commands
Git push commands enable user(s) to upload and share their local commits with a central repository. It takes the changes made on a computer and updates the shared version of the project, where all corrections are visible and accessible to other contributors.
Some commonly used Git push commands are:
- git push <remote> <branch>: Pushes the specified branch along with all the necessary commits to a specific remote repository.
- git push <remote> --force: Forces a Git push, even if it results in a non-fast-forward merge. Be cautious when using this option, as it can potentially overwrite and discard commits on the remote repository.
- git push <remote> --all: Pushes all your local branches to a specified remote repository.
- git push <remote> --tags: Pushes all your local tags to a specified remote repository.
Rebase- Git Commands
With git rebase, the user can reorganize and combine the changes onto a different branch, creating a clearer and more streamlined history of your work. It's akin to adjusting the pieces of a puzzle to seamlessly fit into a new picture, allowing changes to integrate smoothly with a different starting point.
Some popular Git Rebase commands are as follows:
- git rebase: Reapplies commits from a branch onto a different base branch, integrating changes in a linear sequence.
- git rebase <branch>: Reapplies commits from the current branch onto the specified branch, incorporating changes in a linear sequence.
- git rebase --interactive: Allows the user to interactively modify and rearrange commits during the rebase process.
- git rebase --abort: Cancels an ongoing rebase operation and reinstates the branch to its former state.
Stash- Git Commands
Users can temporarily set aside unfinished work in private storage using git stash commands with the freedom to switch tasks without committing incomplete changes. It's like tucking away your ongoing modifications in a personal hiding spot, allowing you to focus on other tasks while keeping your unfinished work safe and easily retrievable.
Some popular Git Stash commands are:
- git stash: Creates a stash with the local modifications made by the user and reverts back to the head commit.
- git stash -u: This will stash untracked files.
- git stash list: Displays a list of all stashes in the user's repository.
- git stash show: Views the file content of the user's most recent stash. It shows the changes stored in the stash as a diff between the stashed content and the commit when the stash was created.
Remote- Git Commands
With git remote, users can view and handle the connections to remote servers and repositories, which act as online storage spaces for their code. It's like having a map of the locations where users store their code backups, enabling collaboration and synchronization of work across different places.
Some popular Git Remote commands are given below:
- git remote: It allows users to view and manage the connections to remote repositories.
- git remote -v: Shows a list of remote repositories along with their URLs, giving the user an overview of their configured remotes.
- git remote add <name> <url>: The Git add command adds a new remote repository with a specified name and URL to the user's Git configuration. It enables the user to establish a connection to a remote repository for collaboration or code synchronization.
- git remote rename <old-name> <new-name>: Renames a remote repository from the old name to the new name in the user's Git configuration. Provides a way for the user to update the reference to a remote repository without altering its URL.
Some Advanced Git Commands
By now, you must be familiar with some of the most important Git basic commands used in local and remote repositories. Now, we will discuss some of the Git advanced commands. So, let's get started with the Git commands list that will boost your understanding and help you become the best Git Hero.
Branch Git Commands
- git push <remote> --delete <remote-branch-name>: Removes a remote Git branch.
- git push --set-upstream <remote> <branch>: Sets an upstream branch, i.e., this command sends your local branch to the new remote branch.
Checkout Git Commands
- git checkout <tag name>: Helps explore a Git tag in a detached HEAD state.
- git checkout -b <branch-name><tag-name>: Helps explore a Git tag in the form of a branch.
Merge Git Commands
- git merge --squash: Combines all changes being merged in a branch into a single commit.
- git merge --no-commit: Combines branches into the current branch without creating a new commit.
- git merge --no-ff: Creates a merge commit rather than attempting a fast-forward.
Clone Git Commands
- git clone <repository-url> --branch <branch-name>: Replicates a repository and helps explore the specific branch.
- git clone <repository-url> --depth <depth>: Replicates a repository with a specified number of commits (<depth>).
- git clone <repository-url> --no-tags: Replicates a repository without copying the repo’s tags.
Commit Git Commands
- git commit: Saves changes to your Git repository. Running this command with the corresponding file name will save the file changes to your repo.
- git commit -a: Adds all modified and removed files in the working directory to the current commit.
- git commit --amend: Amends a Git commit.
Pull Git Commands (Related to Merge)
- git pull --squash: Combines branch changes into a single commit.
- git pull --no-commit: Merges branches without automatically committing.
- git pull --no-ff: Always creates a merge commit, even for fast-forward merges.
Pull Git Commands (Related to Fetch)
- git pull --all: Fetches all remote branches.
- git pull --depth=<depth>: Retrieves a limited number of commits.
- git pull --dry-run: Simulates the process without modifying the repository.
- git pull --prune: Removes references to deactivated remote branches.
- git pull --no-tags: Excludes fetching of tags.
Rebase Git Commands
- git rebase --onto <newbase>: Reapplies commits onto a new base, disregarding the previous base branch. It is important to remember that one should never rebase commits once they are pushed to the public repository.
Stash Git Commands
- git stash drop <stash>: Removes a specific stash from the user's list of stashes in the repository.
- git stash pop <stash>: Applies a stash to the top of the current working tree and removes it from the user's list of stashes.
- git stash apply <stash>: Applies a stash on top of the current working tree. The stash is not removed from the user's list of stashes.
- git stash clear: Removes all stashes from the user's repository.
Remote Git Commands
- git remote remove <name>: Removes the specified remote repository from the user's Git configuration. It allows the user to disconnect from a remote repository that is no longer needed.
- git remote show <name>: Displays detailed information about a specific remote repository, including the remote branches and configuration details. Gives the user insights into the remote repository, such as the branches available and additional settings.
- git remote prune <name>: Cleans up stale references to remote branches that have been deleted or are no longer available in the specified remote repository.
This advanced Git command list will provide you with powerful functionality for managing your codebase, collaborating with others, and maintaining a clean and efficient version control centralized workflow.
Conclusion
We can conclude that Git is a powerful and advanced tool to manage all changes that might happen in a project. It helps users scale projects and allows a work environment that is suitable for multiple developers. While using Git, the Git commands are essential and revolutionary tools that facilitate easy access to repositories and the code base, help make necessary changes, maintain a version history of all those changes, structural branching of the repositories, and leverage the efficiency of the developers and operations.
The Git commands list for both basic and advanced commands given above will help you manage all the tasks seamlessly and effectively, thus saving time and effort. We are sure that by the end of this, you must have developed an understanding of all the basic Git commands you would require while working on your project, whether on remote repositories or your local repository. And gained comprehensive knowledge of some of the advanced Git commands that every developer must know.
Frequently Asked Questions (FAQs)
Q. How to merge two branches using Git?
To merge two branches in Git, follow the steps listed below:
- First, switch to the feature branch.
- Then, execute the command git merge <branch-name> command to initiate the merge with the desired branch.
- Git will handle the automatic merging of changes. If conflicts arise, you will have to resolve them manually.
- After resolving conflicts, commit the changes to complete the merge, integrating both branches' changes into the target branch.
Q. What are the popular Git hosting services?
Git hosting services are platforms that facilitate the storage and management of code online, ensuring easy collaboration with others in a user-friendly environment. Users can benefit from using such platforms as they can easily connect and collaborate with fellow developers per their respective projects' requirements.
GitHub, SourceForge, GitLab and Bitbucket are some popular Git hosting services used across the globe by developers wishing to collaborate with other users and in need of accessing various repositories.
Q. What do you understand by the term Git repository?
A Git repository is essentially a directory where project files are stored, in addition to metadata and version control information. It enables developers to track changes, manage branches efficiently, and collaborate easily with other users. It contains all data on the project’s evolution, which makes it easier for the user to access information regarding the project as per his/her wish.
Q. How do you add a message to a Git commit?
Messages added to Git commits act as descriptions for the corrections made in the source code, providing a clear and concise summary that helps in understanding the purpose and context of the commit. The correct syntax you should follow to pass a message to a Git commit is:
git commit -m "this is the message".
Q. How is the Git Fetch command different from the Git Pull command?
The Git Fetch command retrieves the latest changes from a remote repository, while automatically updating your local repository without merging them. This allows you to review the changes before deciding how to integrate them. In contrast, the Git Pull command combines the fetch operation with an immediate merge of the retrieved changes into your current feature branch.
Q. How does Git merge conflict occur?
A Git merge conflict happens when the same part of a file is modified by different people or branches, due to which Git can’t combine them automatically. In such a situation, we need to manually decide how to merge the changes together. Such scenarios arise when the commits are on the same line or branch. One of the simplest ways to address a merge conflict is by opening the file and making the required changes.
Q. Why do we need Git commands?
Git commands are essential for effective version control and collaborative software development. They simplify tasks like creating branches, improving user collaboration, conflict resolution, code manipulation, and code backup. Git commands thus help better manage code bases, synchronize data, and maintain an organized work environment.
By now, you must be familiar with most Git commands. Here are a few other topics you must know about:
- Git Log | A Comprehensive Guide To Using Git Log Command
- Git Vs. GitHub | 11 Differences, Applications, Prices & More
- Git Cherry Pick Command | How To Use, Undo, Resolve Conflicts & More
- Git Submodule: Add, Remove, Pull Changes & More (With Examples)
- What Is Bash? Features, Major Concepts, Commands, & More
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment