Git Submodule: Add, Remove, Pull Changes & More (With Examples)
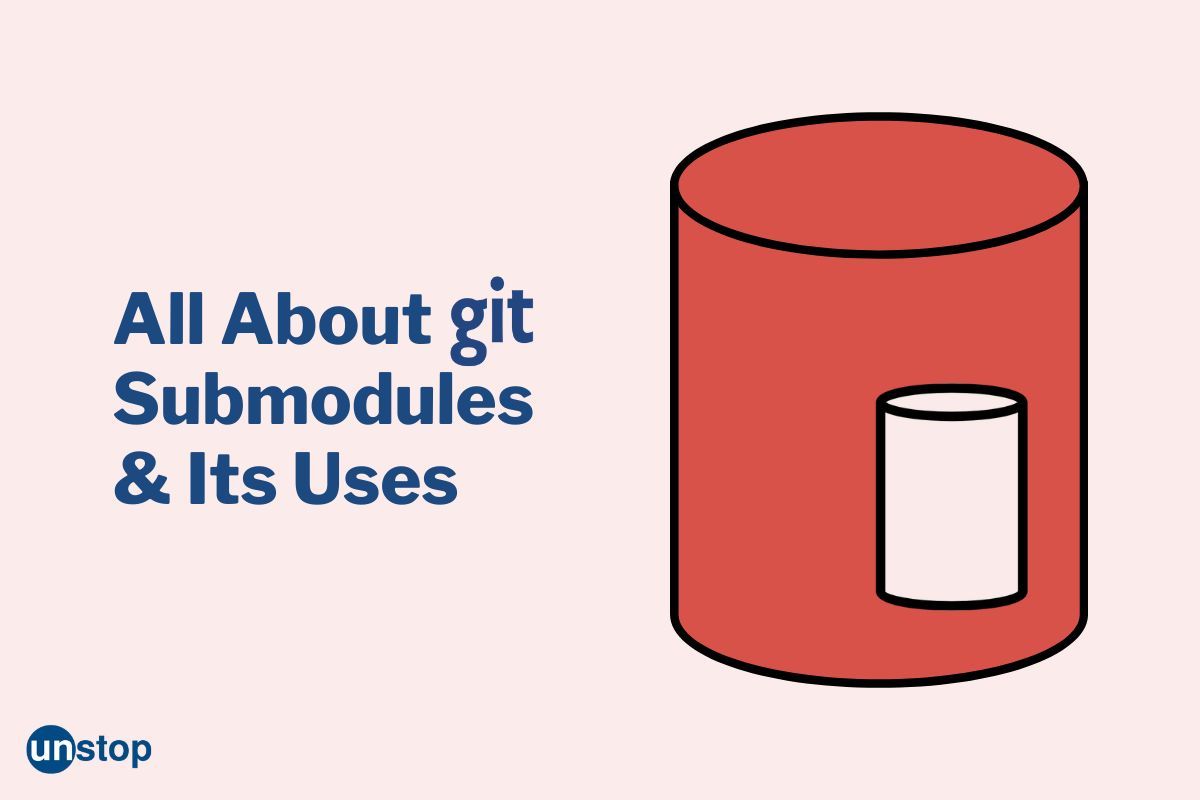
Git is a widely-used version control system that allows developers to track and manage changes to their codebase. One feature that sets Git apart from other version control systems is its ability to handle submodules. Git submodules are a powerful tool that can help you manage dependencies and keep your source code organized. In this article, we will discuss what Git submodules are, how they work, and how you can use them effectively.
What Are Git Submodules?
Git submodules are a feature of Git that allows you to include one Git repository as a subdirectory within another Git repository. This can be useful if you want to use code files from another repository in your project without actually copying them into your repository.
When you add a Git submodule path to your repository, Git creates a special entry in your repository's .gitmodules
config file that points to the submodule's URL and the location where it should be cloned. When you clone the main repository, Git will automatically clone the submodules as well, and initialize them as separate external repositories within your main repository's directory tree or project tree.
Submodules can be updated independently of the main repository, which means that you can keep the code in the submodule up-to-date with the upstream repository without having to manually copy and paste changes into your main repository. However, working with submodules can be complex, especially when it comes to managing changes and merging code between repositories.
How Git Submodules Work?
Git submodule is a way to include a Git repository as a subdirectory within another Git repository. It allows you to keep a separate repository for a specific component or library that you want to include in your project.
Here's how it works:
-
First, you need to add a submodule to your repository. This can be done using the
git submodule add
command, followed by the URL of the repository you want to include and the path where you want it to be located within your project. -
Git will create a new file called
.gitmodules
in the root directory of your repository. This file contains information about the submodule, including the URL, the path, and the commit SHA of the specific version of the submodule that is included. -
When you commit and push changes to your main repository, the submodule will not be included in the commit. Instead, the commit will include a reference to the specific commit SHA of the submodule that is included.
-
To update the submodule to a new version, you can use the
git submodule update
command. This will fetch the latest changes from the submodule repository and update the reference to the new commit SHA in your main repository. -
To make changes to the committed submodule itself, you need to navigate to the submodule directory and work on it like any other Git repository. When you commit and push changes to the submodule repository, you will also need to update the reference to the new commit SHA in your main repository.
How To Add Git Submodule?
The git add submodule
command is used to add a submodule to your repository. The syntax for the command is as follows:
git submodule add <repository URL> <path>
Here, <repository URL>
is the URL of the repository that you want to add as a submodule and <path>
is the path where you want the submodule to be located within your repository.
For example, if you wanted to add a submodule for the my-library
repository located at https://github.com/my-account/my-library.git
, and you wanted the submodule to be located in a directory called lib
within your repository, you would run the following command:
git submodule add https://github.com/my-account/my-library.git lib
This would create a new directory called lib
within your repository, and clone the my-library
repository into that directory as a submodule. Git would also create a new entry in your repository's .gitmodules
file to track the submodule.
Once you have added a submodule to your repository, you can use the git submodule update
command to clone the submodule and initialize it within your repository.
How To Remove Git Submodule?
To remove a submodule in Git, follow these steps:
-
Navigate to the root directory of your Git repository in your terminal to check the version history of the current Git branch.
-
Use the following command to list all the submodules present in your repository contents:
git submodule
-
Identify the submodule you want to remove from the list of submodules.
-
Use the following command to remove the submodule:
git submodule deinit <path_to_submodule>
Replace <path_to_submodule>
with the relative path to the submodule, you want to remove. For example, if your submodule is located at path/to/submodule
, you would run:
git submodule deinit path/to/submodule
-
Use the following command to remove the submodule from the Git index:
git rm <path_to_submodule>
Again, replace <path_to_submodule>
with the relative path to the submodule, you want to remove.
-
Use the following command to remove any leftover Git references to the submodule:
rm -rf .git/modules/<path_to_submodule>
Replace <path_to_submodule>
with the same path used in step 4.
-
Commit your changes using the following command:
git commit -m "Removed submodule <path_to_submodule>"
-
Replace
<path_to_submodule>
with the same path used in steps 4 and 6.
That's it! The submodule should now be completely removed from your Git repository.
Pulling In Upstream Changes From The Submodule Remote
When working with submodules in Git, it is common to have a submodule that tracks a remote repository. You can pull in changes from the submodule's remote repository to update your local repository.
To pull in upstream changes from the submodule remote, you need to perform the following steps:
- Change to the submodule directory:
cd submodule_directory
- Fetch changes from the upstream remote:
git fetch origin
- Checkout the branch you want to update:
git checkout branch_name
- Merge the changes from the upstream remote into your local branch:
git merge origin/branch_name
- Commit the changes:
git commit -m "Pull in upstream changes"
- Push the changes to the submodule's remote:
git push origin branch_name
After completing these steps, your local submodule repository will be updated with the changes from the upstream remote repository.
It's important to note that pulling in upstream changes from a submodule remote does not automatically update the parent repository. You'll need to commit the changes in the submodule and push them to the parent repository to update it.
Git Submodule Commands
Here are some of the most commonly used submodule commands:
-
git submodule init
: Git submodule init command initializes all the submodules in your repository. The Git submodule init command clones the submodule repositories into the.git/modules
directory and checks out the submodule commits. -
git submodule update
: Updates all the original submodule repositories to their latest commits. -
git status command
: The git status command is a commonly used command in Git that allows you to view the current state of your local repository. -
git submodule update --init
: Initializes and updates all the submodules in your repository. -
git checkout command
: The git checked-out submodule commit is a fundamental command in Git that allows you to switch between different default branches or commits in the parent module in Git repository. -
git submodule update --recursive
: Updates all the submodules in your repository and their submodules (if any) recursively. -
git submodule foreach <command>
: Runs a Git command on each submodule. For example,git submodule foreach git pull
will rungit pull
on each submodule. -
git submodule status
: Shows the status of each submodule in your repository, including the commit hash and whether the submodule is up-to-date. -
git submodule sync
: Synchronizes the URL of each submodule with the URL listed in the.gitmodules
file. -
git submodule deinit <path>
: Removes the submodule at the specified path from your repository. This command does not delete the submodule repository itself. -
git submodule update --remote
: Updates each submodule to the latest commit on its remote master branch. -
git clone command
: The clone command is used to clone a single repository that contains one or more submodules. Submodules are separate Git code repositories that are embedded inside another Git repository.
Uses Of Git Submodules
Git submodules are used in large projects to incorporate external repositories as dependencies. This allows developers to reuse code across submodule projects, manage external dependencies, and keep the codebase and package management organized.
Here are some common use cases for Git submodules:
-
Incorporating third-party libraries: You can use submodules to include external libraries into your submodule project as dependencies. This makes it easier to manage updates and keep the codebase organized.
-
Sharing external code between projects: Submodules allow you to share the actual code across different projects. You can have a common submodule code that is shared across multiple projects with submodules and configuration files, and any changes made to the common codebase will be reflected in the project repository.
-
Managing complex projects: Git submodules are useful for managing complex projects with multiple repositories. By using submodules, you can manage the dependencies between different repositories and keep the codebase organized.
-
Versioning control: Submodules allow you to track the version of a specific repository at a specific point in time. This can be useful when you need to ensure that a specific version of a dependency is used.
Overall, Git submodules provide a way to manage external dependencies and share external code across projects while keeping the codebase organized and maintainable. However, it's important to understand how the concept of submodules works and how to properly manage them to avoid potential issues.
You might also be interested in reading the following:
- What Is GitHub? An Introduction, How-To Use It, Components & More!
- Introduction To Git Stash | How To Use Git Stash Commands
- What Is Bash? Features, Major Concepts, Commands, & More!
- What Is Linux Shell? Definition, Features, Types & More!
- What Is C++? An Introduction To C++ Programming Like No Other!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment