Git Tag | Easily Create, List, Remove, Push Tags & More!
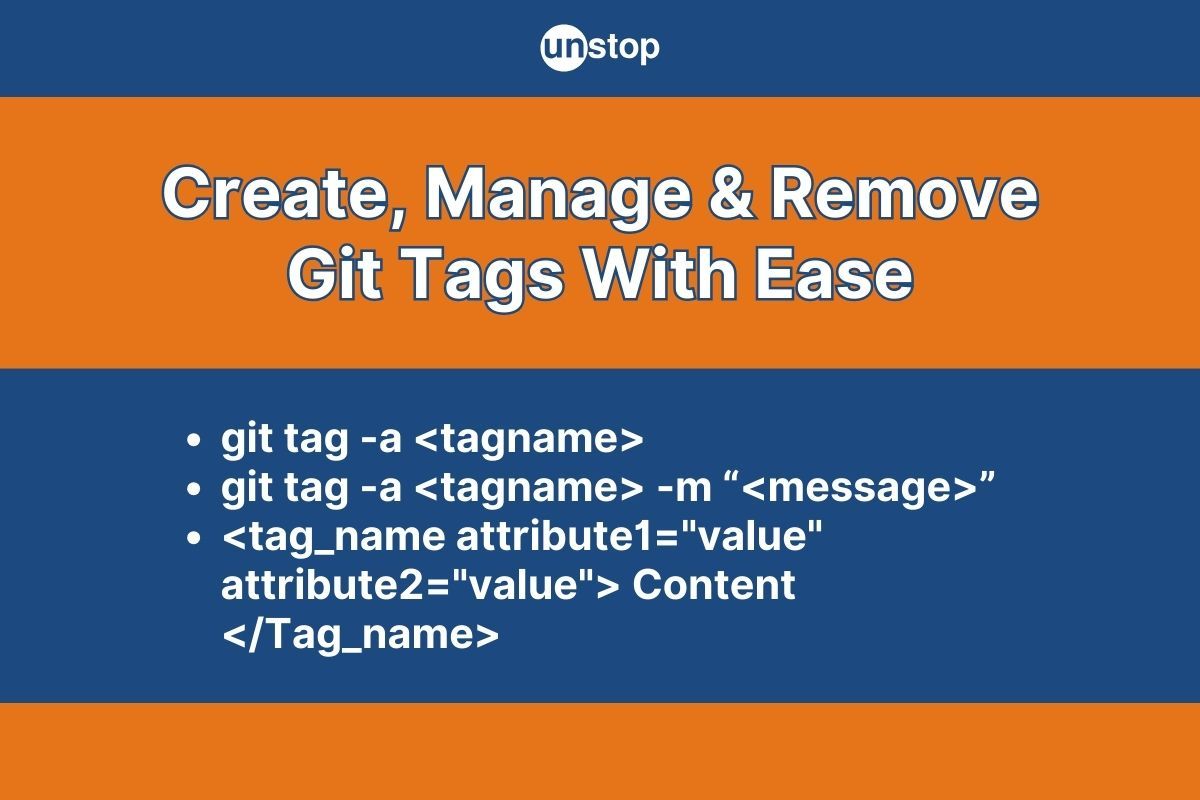
Git tags are a powerful tool/ feature that mark or point to specific points in time within your repository/ history of Git repository. They allow you to track codebase versions, quickly access important commits, and provide labels for easy reference. Tags have two parts, i.e., the tag name (which should be short but descriptive) and an associated commit SHA hash, which marks the point in the tagged history.
You can create annotated or lightweight tags depending on how much information you need about each tag. However, annotation is generally recommended as it provides more detailed information, such as author details and dates. Once created, these immutable markers will follow all future changes made by others branching from that tagged commit, making them very useful for tracking progress across Git branches over time.
When To Use Git Tags?
Git tags are used to mark significant points (or commits) in the project history. They typically indicate a release or certain iteration of a project and allow developers to access that specific version at any time.
Some use cases for Git tags include:
- Marking bug fixes or important patches
- Creating versions for software releases
- Denoting the current development state of an otherwise chaotic repository
- Grouping several commits for easier organization.
Creating A Git Tag
The process to create a Git tag is as follows:
- Ensure that you have an existing Git repository by using the git init command in the root directory of your project.
- Then, use the git tag -a <tagname> command to create a new tag. This will open the text editor for you to enter a message associated with this version. Save it it when done. Make sure you include some information about what was changed or added since the last release/commit, such as bug fixes, updated features, etc.,
- Alternatively, if you don't want to write any longer messages, then use the git tag -am" <message>" <tagname> command.
- After creating a tag, it is important to push up the tags using the git push --tags command so everyone can access them. The flag lets Git know to only send tags instead of sending everything else along with them, like commits (which can get messy).
- Finally, verify that all went well with listing out currently available tags using the git-show command, which shows both local and remote tags. Run this through the $ git show ––list command.
The Syntax To Create A Git Tag
git tag -a <tagname>
Types Of Git Tags
There are two primary types of git tags, as shown in the image below. We will discuss both of these in detail, along with syntax and examples. In the next section, we will learn about an additional type of Git tag, i.e., listing tags and their subtypes.
Annotated Tags
An annotated tag is a permanent label developers apply to specific points in the project's history. It is similar to an ordinary tag, except it contains extra information about the changes applied when the tag was created. This information usually includes a brief message describing what has changed since previous versions, who made those changes, etc. Such tags provide additional context for understanding how different versions fit together and why certain decisions were taken during development. Annotated tags are commonly used with version control systems such as git, GNU privacy guard, or Subversion (SVN).
The syntax of Annotated Git Tag:
git tag -a <tagname> -m “<message>”
An annotated tag is created using the -a ("annotation") flag with the git tag command.
Code Example:
git tag -a v1.0 -m
git push origin v1.0
# Navigate to your Git repository's root directory
cd /path/to/your/repository# Create an annotated tag with a message
git tag -a v1.0 -m "version 1.0 is released"# Push the tag to the remote repository
git push origin v1.0
Lightweight Git Tags
Lightweight tags are HTML elements that do not require an end or closing tag. They consist of single opening (<>) and closing (/>) tags, such as <br /> for line breaks or <img /> to embed images.
- Instead of having both an opening and a closing tag, they stand alone with just one set of symbols at either end.
- Lightweight tags were introduced in XHTML 1.0 to make code easier to read by creating fewer lines within documents than traditional HTML with its full range of open and closed tags per element.
- Lightweight elements will typically contain self-contained data, such as attributes specific to the type/class/ID value from which it is derived.
- Other lightweight content may include short bits of inline text or media like audio clips on webpages outside their host document's DOM hierarchy structure. This is due to the inability to exist only inside regular container-type elements like list items or table cells etc.
Syntax of Lightweight Git Tags:
<tag_name attribute1="value" attribute2="value"> Content </Tag_name>
The process of creating lightweight Git tags is as follows:
- Start by opening a text-editing program and create an HTML document or open one you already have saved to your computer.
- Write the code for the lightweight tags in between <> and /> symbols. For example- <img src=" image_url" alt=" description of image"/>
- Add any additional information with attributes inside quotes (when required) such as width=" #value" height=" #value" border= "0|1″ or other details depending on the Git tag type being used. For example- if using Link Tag: "<a href=' http://www....'>linktext</a>"
- That's it! You've successfully implemented Lightweight Git tags into your HTML document. Now save changes made before viewing them live via the web browser preview window.
Listing Git Tags
A listing tag is a way to identify items in an online marketplace. Sellers use it to categorize their products so buyers can easily find the items they seek when searching through listings.
- Listing tags also provide useful information about each item, such as brand, material type, and condition.
- These Git tags help create accurate search results, which makes it easier for customers to quickly sort through hundreds of potential purchases before making their decision.
- Some popular e-commerce sites like eBay use automated tagging systems that generate relevant keywords based on product titles or descriptions provided by the seller.
- These keywords often appear toward the bottom of each listing page and serve as additional pieces of classification data that shoppers can use to refine searches even further.
Syntax for Listing Git Tags:
Tag name: Tag Description (example - Type: Shirt)
Here is how you can create a Listing Git tag:
Step 1: Add an array of listing tags to the item. The items in this example are shirts, so let's create the following tags-
'Type: Shirt', 'Brand: XYZ Brand', and 'Material: Cotton';
Step 2: Loop through each tag and assign it to your item. In JavaScript, you can do that with a simple for loop like this:
//Declare an empty string variable where we'll store all our listing tags
var listTags = "";//Loop through each tag & concatenate them together
for (var i = 0; i < 3; i++) { listTags += myListingtags[i] + '<br/>'; } console.log(listTags);
Step 3: Once done looping through all available listing tags, they can be displayed on the page or saved as part of the database record associated with your product information.
List Local Tags
Local Git tags are a way to label or mark specific points in the history of your repository. They can identify release versions, significant changes, and other checkpoints along the development timeline. Local Git tags allow you to create multiple labels that point to the same commit so that they can easily be referred back to at any time.
- To list the local Git tags in a repository, use the git tag command from within the repository's directory in your Command Line Interface (CLI).
This will generate a list of your repository's local tags. - If you want to see more details about the tags, then use the -n flag to view tag messages. The command will then be like this- git tag -n.
- You can also use the --tags option to show a list of tags associated with your repository.
- If you want to limit the number of tags displayed, use the -n <number> flag. For example, the git tag -n 10 command will display the last 10 tags and their respective messages.
- To use the commands listed, ensure you are in your repository's root directory and run them from there.
List Remote Tags
A remote list Git tag is a special label that you can attach to a particular point in the history of your project. Identifying specific versions of your work is easy, which may be helpful for collaboration or other purposes.
- You can create multiple tags with different names and refer back to them later on if necessary.
- When pushing changes into remote repositories, it's crucial to remember tagging so others will have access to the same version of code that you do.
Git is a decentralized versioning system. To list remote Git tags, you must use the git ls-remote command with the tags and the name remote repository.
In this case, the command syntax will be as follows:
$ git ls-remote --tags <remote>
Now, say, for example, your remote name is 'origin', then you will execute the command given below to show the tags using the refs syntax as output.
$ git ls-remote --tags origin
Fetching Remote Tags Easily
To fetch tags from a remote repository, use the command with the all and the tags options:
$ git fetch --all --tags
Fetching origin
From git-repository
53a7dc..7a9ad7 master -> origin/master
You can List them using the command-
$ git tag
Tagging Old Commits
Commit history helps track source code changes. It stores all the changes developers make, like adding, editing, or deleting files, and allows them to revert to any change done using the Git system. Tagging old commits means assigning relevant tags (such as 'release') on previously committed versions of your source code within git repositories.
- This makes it easier for you to quickly find out specific points when important decisions were made regarding your software development progress, regardless of how many subsequent commits have been created since that point in time.
- With tagging, one can easily identify what features were added and when and trace any bugs that may be linked with such tagged release versions.
- In addition, it offers an overview for a team about their previous efforts/progress without going through each commit separately at great length, making collaboration between teams much more efficient and organized!
The syntax for tagging commits is-
git tag <tagname>
ReTagging Or Replacing Old Git Tags
Re-tagging or replacing old git tags is a process where you can assign new IDs to existing elements in your system.
- It’s similar to tagging, except instead of assigning labels and keywords describing the element, you are changing its unique identifier.
- This enables quicker search results, better data organization across systems (e.g., syncing multiple databases), and improved scalability when adding more elements into the mix over time.
- With re-tagging or replacing old tags comes an increased risk for errors due to manual labor involved in tracking changes. However, it pays off by ensuring all relevant parties stay informed throughout this process and taking proper backups before implementation.
Syntax:
element.setId(new_id);
Sharing: Pushing Git Tags To Remote
The steps involved in pushing tags to remote are as follows:
- First, retrieve the list of remote Git tags available by using the git fetch --tags command.
- Next, select a tag to push to the remote repository. Say, for example, you want to push the 'v0.7' tag. Then, the command will be git push origin v0.7 (where 'origin' is the placeholder for the remote name).
- After that, you must ensure that the tag was successfully pushed to the repository. For this, use the git ls-remote --tags or git show-ref --tags commands on your local repository root directory.
- Alternatively, you can also execute the command in Git Bash/Terminal, where the command will transform to- git show-ref –d –t refs/remotes/. This will list all existing references from local and remote repositories, including branches and tags.
- Now search for your desired tag, then confirm it was correctly pushed onto the remote repository by executing the following commands inside Git bash / Terminal-
# List all references stored in our Local Repo
$ git show-ref
# List only Tags stored in our Local repo
$ git show-ref -t refs/Tags/ - Execute similar commands like above to find out when the Git tag exists remotely. The command will be as follows:
#List Tags References present
$Git ls–remote — Refs/Tag/remotely - You can also run the git tag command to list all local tags available in the current repository and check if the desired one is present by using the $ git tag –l command. This will also list all available local tags.
Deleting Git Tags
Deleting a git tag refers to the process of removing an existing HTML or XML element from a web page, document, or other electronic media.
- Git tags are added to HTML documents to identify specific elements for styling purposes.
- When these tags become outdated and are no longer necessary, they can be deleted from the code using an editor such as Notepad or TextEdit on Windows/Macs, respectively.
- To delete a tag, you must first locate it within the code before right-clicking (or pressing ‘Ctrl + X’) on it.
- This will remove the entire line containing that particular tag.
- Once deleted, the page will no longer display whatever content was associated with that tag.
Example:
Say you want to remove a <h2> tag from an HTML document. So you first locate the code for that particular element and then right-click (or press ‘Ctrl + X’) to delete it. Once deleted, the associated content within that heading will no longer be visible on the page.
Delete A Remote Git Tag
This refers to the act of deleting an existing Git tag from a remote repository. A tag is used to label versions of your files or project releases, which can be tracked and identified by other developers. Deleting a remote tag allows you to clean up any unnecessary tags that are not needed in the repository.
- To delete a specific remote tag, users must have sufficient access privileges within their version control system (GitHub) for the command to complete successfully.
- Anyone with access must use the git push command origin: <tagname>. This command will remove the specified tag from their remote repository.
Example:
git push origin : v1.2 // to delete the tag `v1.2` from the remote repository
Delete Multiple Git Tags
HTML and other coding languages allow users to delete multiple tags at once quickly. This feature of deleting multiple Git tags can also be used to remove invalid or redundant tags and streamline the code by removing unnecessary lines of markup.
This process typically involves selecting all the desired tag names, then deleting them with one click or command. In some cases, it may also allow for grouping and nested deletions if supported by the editor.
Example:
<div>
<p>This is a sample text.</p>
<h1></h1> //this tag needs to be deleted.
</div>
To delete multiple tags using this feature, select the h1 tag and hit the 'delete' button or command (such as Ctrl+D). The result would look like this-
<div>< p > This is a sample text. </ p > </ div >
Checking Out Git Tags
In software development, Git tags usually identify releases and other significant points in the codebase history. When you 'check out' a Git tag, you're downloading the specific version of the associated codebase so you can inspect it or use it as part of your project. This allows developers to keep track of which versions they can access and ensure they always have up-to-date copies available when needed.
Example:
We already know that if you have a project that is maintained using the Git version control software, you can create tags for each version of your software. Anytime during the process, if any of the team members need to check out an older codebase version, they must find and select the appropriate tag to download all associated files. This makes it much easier for developers who are working on multiple versions simultaneously or want their current work to be clear from updates from others.
Git Tags: Manage & Add Versions To Project History
Git tags mark specific points in a project's history and help keep track of different releases or versions. A tag is like a bookmark that can be added at any commit or branch in the Git repository. Creating tags makes it easier to refer back to exact commits, such as bug fixes or hotfix deployments.
- To add and manage Git tags, you need access permissions on the remote server hosting your Git project tree (e.g., GIT-Tag-EX/).
- The simplest way of adding tags is by using the git tag command with the -a option followed by an annotation message for annotation. This can optionally be followed by -m, which provides lengthier custom messages suitable for release notes, etc., before pushing them through a simple git push --tags command.
- You may specify certain options while tagging with this command, such as Push Rules and Push Tag Options, via the .git config file stored locally after running the git init command.
Here is the breakdown of how to manage a project using Git and Git tags:
- First, navigate to the respective local folder that contains the project repositories.
- Next, stage the repositories locally, i.e., select and prepare the changes you want to commit.
- Then, deploy these commits to the respective remote servers. This process facilitates the management and synchronization of all files & directories corresponding to the project.
- It is also useful to add annotated messages that describe the changes being made in the commit. You can then access all the valid information when needed swiftly, given Git's ability to provide commit history.
You can customize this process as per your preferences and the requirements of the project. It is also possible to specify the output you expect from the process, such as a specific version or deployment configuration.
Your remote server project will store all tags in a Tags directory. You can view all existing tags from inside Git by using the git tag command with the --list option to specify certain options for sidebar display, such as level-wise sorting, through suitable settings.
Git Tags & History of Commits
Git tags are labels that, when applied to certain commits, enable users to locate those specific commits in a particular repository quickly. They allow developers to organize their projects more efficiently and make navigating the source code easier for other contributors.
- Commits are recorded snapshots of changes made within the version control system over a period of time. All these changes create different versions of your project or application. They can also be referred to as Git commit history, where each entry records metadata like information about who committed what change, when they did so, etc.
- A commit consists of two essential elements: the revision number/version identifier (often called ‘Hash’) and a tagging message similar to an email subject line that clarifies why this particular change was done, i.e., what problem it solves, the feature is added, etc.
Git tags are often used in Git release processes to mark specific points in the repository's history.
- A Git release such as Release Candidate is created in a Git repository based on specific commits, usually the last stable version.
- Additional fixes and features might also be needed before creating a public release.
- A new branch must be created to incorporate these changes before joining it with the main branch. This leads to the creation of a new commit merge branch.
- then, you must complete the verification process to ensure only authorized members can push changes.
- Team members define the rules for verification, which may vary depending on the specific repository under consideration. The release process considers the previous commits and the range of combined commits that have been included in the release.
The aim is to ensure that only authorized and well-documented changes (implicit pushes) are accepted into the release, leading to the desired outcome.
Conclusion
Git tags provide a powerful way to track progress across branches, quickly access essential commits, and label versions for easy reference. They have two parts, i.e., the tag name (which is short but descriptive) and an associated commit SHA hash, which marks the point in time of tagging.
There are primarily two different types of tags, i.e., annotated Git tags and lightweight Git tags. Users can decide which of these to use depending on how much information is needed about each one. The annotated Git tags are often recommended as they provide a wider range of details, such as author details and dates, etc. Once created, these immutable markers will follow all future changes made by others branching from that tagged commit, making them very useful when tracking codebase versions over time. The Git tags features also come with a variety of other uses, such as listing local/remote Git tags, fetching remote ones easily, re-tagging or replacing old ones, and pushing up new shared copies to a repository, which help users organize version history no matter how complex the project gets!
Common Git Tags Questions/FAQs
Q. What is a Tag in Git?
A Git tag is an object that references or points to a previous commit in the repository history and attaches additional information, such as a commit with a release version number or release note. Once created, Git tags cannot be modified and remain on the current commit they refer to when checked out. Tagging helps with collaboration by allowing other developers to refer back to past codebase versions easily. Tag names can be anything the user wishes. However, they name must be concise and meaningful to allow other developers to understand what each tag refers to easily.
Q. How do I create a Git Tag?
To create a tag, you need to use the git tag command with -a for annotation, followed by your commit message. An example of the command is given below:
git tag -a <tagname> –m 'message'
Q. How do I push Git tags to the origin?
To push Git tags to the origin, you must first create a tag in your Git repository. For this, use the git tag <tag_name> command. After creating a Git tag in your local repository, you must run the git push --tags command, which will push all of the tags from your local repository up to the remote repo (in this case - origin).
This process is very important for releasing versions and tracking changes between them. It helps teams ensure they're on track with developing new features. And also helps maintain existing ones by having different versions of code tagged with their appropriate release name or version number so that everyone knows what has changed since the last time they checked out a version from the source control repo (e.g., GitHub).
Q. What is the difference between a Tag and a Commit in Git?
The three primary differences between the Git tags and commits are listed in the table below:
Git Tags | Git Commits |
They mark specific points in the version history. | They save changes to files permanently in the version control system. |
The primary use is for release version history. | It can be used to make changes and create new branches |
They stay permanent until changed. | Changes are updated when a new list of commits is made. |
Q. Can we store extra information using Git tags?
Yes, we can store extra information using Hit tags like author's name, platform info, etc., along with actual code content inside Git repositories through a tagging mechanism at any time accessible from the Git project tree.
Q. What is the purpose of tagging commits in the Git repositories?
Tagging allows us to label certain groups (or versions) of work and then easily reference them later. This eliminates the need to search through thousands upon thousands of lines manually.
Q. Are there any rules while creating tag messages for release versions inside the Git repository?
Yes, users must follow certain rules while creating Git tag messages for release versions inside the repository. They are as follows:
- The Git tag message must begin with a capital letter and end with a period or a question mark.
- One must use the present simple or imperative mood in the Git tag message. For example, "Release version 1.0.0", not "Releasing version 1.0.0".
- The message must have concise language and should be short (i.e., one line).
- Whenever applicable, reference the issue that this release resolves.
- If the changes are too detailed or too long, include a link to a document containing the details of the changes.
- Always include information on the version number, release date, modified files, or any other relevant information.
Q. How can we search relevant Git tags from the project tree?
You can search relevant Git tags from the Git project tree in two ways-
- Use the git tag --list <tagname> command to retrieve a list of all tags matching a specific pattern.
- Use a graphical user interface in Git projects that will show up as sidebars on your screen with Git tags sorted by various levels and store extra information about components and code changes done over time for different releases.
Here are a few other articles that will help you gain a better understanding of programming concepts:
- Introduction To Git Stash | How To Use Git Stash Commands
- Git Submodule: Add, Remove, Pull Changes & More (With Examples)
- Most Important Linux Commands And Their Syntax (With Examples)
- How To Kill Process In Linux | Commands To Locate & Kill
- What Is C++? An Introduction To C++ Programming Like No Other!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment