How To Create A Git Branch? 10 Ways Explained (With Examples)
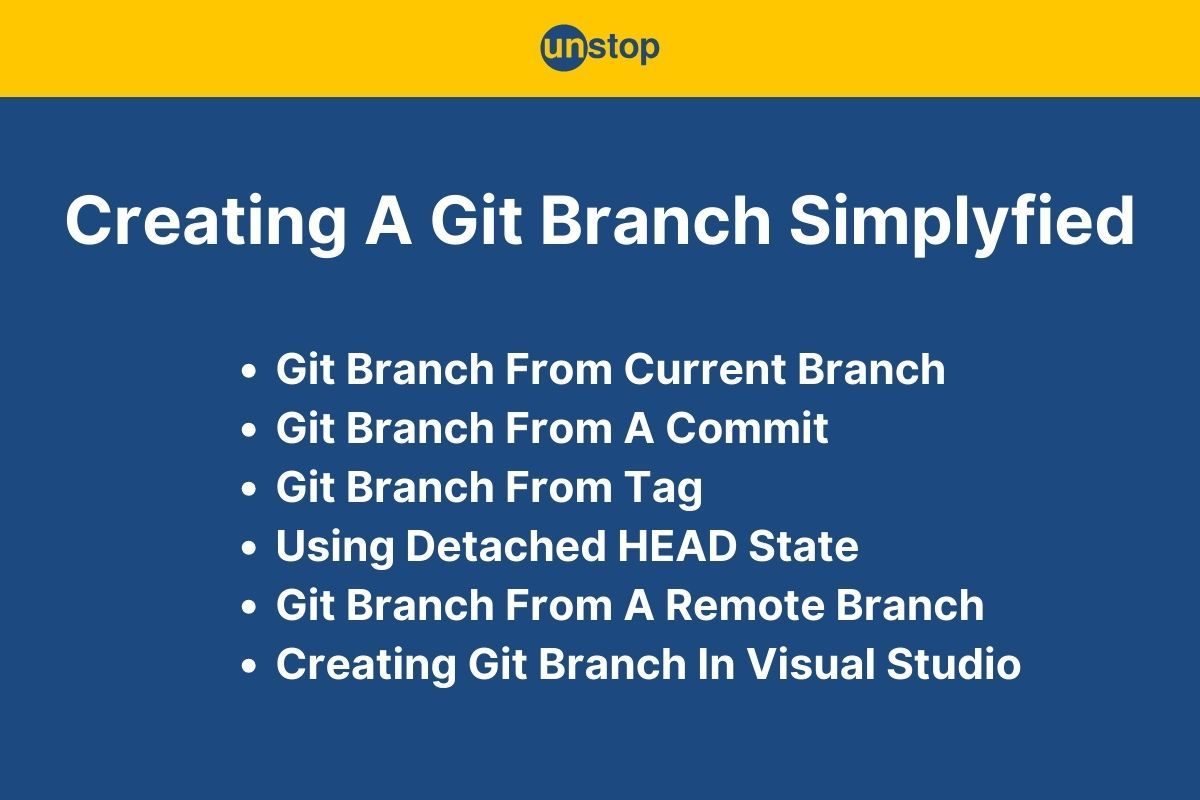
A branch in Git is a concept/ component that allows users to branch out of the current version of code or files. In other words, it facilitates separation between different versions of code or files. The creation of branches helps developers work on individual segments without affecting the whole project until the changes are finalized. In this article, we will discuss the git create branch process and various aspects of using them, and branch management with commands and GUI tools.
We'll also discuss merging changes from other branches, strategies for organizing development teams around multiple branches, and potential issues when branching. So let's get started!
Prerequisites For Git Create Branch Process
To ensure success in the process of creating and using new branches in Git version control tool, it is important to keep the following points in mind:
- Ensure you have a clean working tree without any uncommitted changes. Check with the git status command if needed.
- Get the latest version of your code from the remote repository by running the git pull request/ command or configure an upstream branch using git push -u origin master. Here, we are assuming your local branch is called master, and its corresponding remote is called origin in Git terminology.
- Create the new branch using either of the two following commands-
- Git checkout -b branch name (to create & switch to it): This method creates a copy from the currently checked-out parent commit and switches directly into this new Git branch.
- Git branches branch name(only to create it): This only creates this new remote branch without checking out, so you need to check out later on when needed explicitly
- Finally, push these changes back to the remote so others working on a project can share the same context as yours. To do so, use the git push --set-upstream origin remote name command.
This will also set up tracking progress so you can pull and push changes concerning this branch without mentioning its name every time.
How To Create A New Branch In Git?
Branching is a concept where developers can create multiple copies (or branches) of the codebase from the same source, allowing them to develop new features without disrupting their main line of development.
- This enables teams to work independently on different areas and makes it easier for control systems like Git to keep track of changes in each branch.
- Branches allow developers to work with open-source projects as well as closed-source ones.
They typically start identical and diverge over time as individual developers complete different tasks before being merged when tested and ready for release.
Here is a step-by-step explanation of how to Git create branch:
- To create a new branch, use the command git checkout -b [branch_name], where [branch_name] is your desired name for the new branch.
- It will create a copy of the codebase and put you in it so that any changes made are added to this branch instead of the master or any other existing branches.
- You can check which branch you're currently on by running git status.
- Finally, push your changes back up with git push origin [branch_name] so they can be shared with others on GitHub or wherever your repository may live!
Let's understand each step/ command, including what happens in the repository when you create a branch in Git.
- Create a new branch in the repository, where [branch_name] is your desired name for that particular branch. This copy of the codebase starts identical to the master, and it can eventually diverge as individual developers complete different tasks before being merged when tested and ready for release. The command is git checkout -b [branch_name].
- Then, use the git status command, which checks which branch you're currently on. This is to ensure that any changes made will be added to this branch instead of other existing branches or, even worse, overwriting something already done in the master.
- Finally, the git push origin [branch_name] command pushes all changes made while working on this feature onto the remote server (i.e., GitHub, BitBucket).
- Once it's there, others can review it quickly without having access to your local machine. Thus, merging into master becomes more manageable since everyone sees what was changed/added throughout its development lifetime. The syntax for this command is- git checkout -b [branch_name]. Here, the git checkout is followed by the '-b' option, which tells Git to create a branch and your desired name for that particular branch.
For example, if you want to create a new feature called add_labels, then the command will look like this- git checkout -b add_labels.
- This command will create the add_labels branch, starting identical to the master. However, it can eventually diverge as different tasks are completed over time before being merged back together with it at release time!
- The command for creating a new branch with switching is git checkout -b [branch_name]. This makes the branch and switches you into it, allowing any changes to be added directly to this feature.
- The command for creating a new branch in Git without branch switching is git branch [branch_name]. This creates the branch but does not switch you into it so that any changes are still being made in the master or whatever other existing branches may exist.
- To switch to the newly created branch called branch_name, use the command git checkout [branch_name].
Branch Naming Conventions | Git Create Branch
Branch naming conventions are essential when using Git for software development, as they provide a consistent way to group branches according to their purpose. When creating a Git branch, it is important to be cautious of the branch naming details/ conventions.
In this section, we will discuss the different types of branch names and how they should be formatted to stay organized and keep track of code changes effectively.
- Feature Branch Naming Convention: The naming convention for a feature branch should be descriptive, i.e., feature/[short-description].
For example - feature/payment_module. - Hotfix Branch Naming Convention: A hotfix branch name should include the issue tracker ID and a brief description of the fix related to that ticket number, followed by a 'hotfix' term at its end.
For example- hotfix/#1234_Fixing_filepaths. - Fixes Relevant To Release Version: When releasing fixes relevant to some version in production branches, use release-[version] as the prefix. The name should also include the dot notation (.) or hyphens (-) according to your organization's needs.
For example- release-2.4 or release-v2-4. - Maintenance Branches: These types of branches are used when bugfixes need a gradual phased rollout rather than an instant deployment on the master. The maintenance branch names should hence follow this format- maint-[issueTrackerID]-[shortDescription].
For example, maint#123456789 Fix tweaks for dashboard display issues. - Chore Branches: This branch type usually deals with updating documentation and non-functional changes like refactoring code, cleaning up dependencies, etc. To form such branch names, use the format- chore/[shortDescription].
For example - chore/add_documentation.
Also Read: Git Rename Branch | How To Rename Local & Remote Branch With Ease
Different Ways Of Creating New Git Branch
As mentioned, Git branches are a powerful tool that allows developers to work on new and existing features without disturbing the main project. They provide an isolated environment where changes can be tested and evaluated separately from other code.
Git branches allow multiple members of a team to collaborate on different tasks at the same time, as well as enable easy reverting if something goes wrong during development or testing. There are several ways you can create a git branch, including-
- Manual creation using command line tools,
- Making via Github’s web interface,
- Merging from another branch, patching with patches files, branching with forks & pull requests.
Each method has its own benefits and you must select one depending upon your need for speed or simplicity in task completion. In this section, we will discuss multiple ways to create a Git branch.
Git Create Branch From The Current Branch
Imagine you are starting with a current branch where you have an existing codebase. Now you want to create a new branch from that. This would allow for further project development while ensuring the original version remains unchanged.
The process of creating a new Git branch from a current branch involves three main steps. They are-
- First Git checkout branch operations, i.e., check out from your current working branch with the git checkout <branchname> command.
- Create the new Git branch using the git checkout -b <new_branch_name> command. It will result in two branches pointing at the same commit event (HEAD).
- Push the newly created local repository to the remote origin server with the git push --set-upstream origin <remote_repo> command or deploy it manually as needed.
These steps must be followed whenever we want to add a new feature or make changes without affecting our existing live production environment. It allows us to make any changes and test them out without affecting our live website or application.
List Of Commands To Create Git Branch From Current
- Git checkout <branch_name>: This command switches between branches.
For example, if you wanted to switch from master to a new feature branch called my-new-feature, you should run the following command: $ git checkout my-new-feature. - Git branch -a or git branch --all: This command lists all local and remote branches in a repository. For example, running this will return something similar to the output:
*master remotes/origin/HEAD -> origin/master remotes/origin/my_other_branch. - Git merge <targetBranchName>: This command merges targetBranchName into your active one. For instance, if you want to merge two branches named dev & develop, then running the following command would do that- ::$git merge dev develop.
- Git push <remoteRepo> <localBrancName>: You should use the push command to share your newly created and potentially modified code with everyone else working on this project. Let's say you want to push changes to the current development type system, and then the syntax structure looks like this- stringResult = string1 + string2:git push origin development.
- Git pull <remoteRepo> <localBrancName>: The pull request/ command pulls all the changes made to remote and brings them locally. For example, if you wanted to pull any changes that were pushed to the development branch, then this can be done by running the pull request- $ Git pull origin development.
Create Git Branch From A Different Branch
Suppose you have a project with two branches, dev and master. You are currently working on the dev branch, but now you need to create another branch from this one for additional feature development.
The Process to Create Git Branch From A Different Branch:
- To create a new Git branch from an existing one (in this case, the dev branch), check out the desired source branch that will serve as your starting point. For example, if you want to work off of the dev branch, you must use the command- git checkout dev.
- After changing to our desired source repository, you must then use the git checkout -b <target_branch> command, where target_branch is what we want to name our new branch. For instance, app-feature-4 might be used here, provided another active repository or current local branches don't already take it.
- The next step to carry out once you have switched the repository is to switch to master mode. You should switch into master mode and get detailed information regarding how many files were added, modified, or deleted directly due to the process, which took place before creating any commits associated with newly created code fragments/file structure, etc.
- This way, once all changes have been recorded on top of existing remote states, even without pushing anything, it allows us to experiment without fear of breaking anything.
- Finally, once everything looks satisfactory, the last command you must run is git push -u origin <target_branch>, which sends new commits over a remote repository named target_branch (in this case, app-feature-4) and sets it as an upstream branch for the local one. This ensures the branches stay in sync even after future pushes/pulls occur.
By doing all these steps, you should now have a successful creation of a new git branch off from your existing dev that is ready for feature development. Below is a list of commands to be used in the process of creating Git branch, along with a brief explanation.
List of the commands
- git checkout <source_branch>: This command will switch you to the branch that serves as your starting point for creating a new one.
Example: Git checkout dev, where dev is the branch you are switching to. - git checkout -b <target_branch>: This command creates the new branch from an existing source and sets it up locally on your machine. Here, target_branch refers to the name you want to give you, your repository.
Example: Git checkout -b App-Feature 4. In this case, app-feature-4 is the repository name given. - git push -u origin <target_branch>: This command sends your new commits over to the remote repository on target_branch (in our example, app-feature-4) and sets it as an upstream branch for your local one to keep them in sync after future pushes/pulls take place.
Example: Git push –U Origin AppFeature4
Create A Git Branch From A Commit
Imagine you are working on a file in your project that you track with version control using Git. After making changes, you commit the file and push it to the remote repository. The process to create a git branch from a commit is given below, followed by a list of commands to be used during this process.
The Process to Create Git Branch from Commit:
- A Git branch is a pointer or label referencing commits within a repository. When creating a new branch from an existing commit, the checkout command will take all of the changes since that commit and create a new branch off of it.
- This assists developers in isolating certain lines of development while they work on different features without impacting other branches or merging them prematurely before they're ready for use.
- To create this type of branch, one would typically run commands like git checkout -b {new_branch_name} {commit-hash}.
- Here, the {new_branch_name} is the name you'd like to give to your newly created branch, and {commit-hash} is the hash (or identifier) for whichever specific commit point at which we want our new feature/bug fix/etc. to start from.
- git clone <url> // Clones an existing repository from a provided URL into the current working directory
- git checkout -b <branchName> // Creates and checks out a new branch called branchName.
- git pull origin <branchName> // Pulls any updates to the local ref of the specified remote branch onto your machine.
- git add // Stages all changes in your current working directory for commit.
- git commit -m "<message>"// Commits all changes with an appropriate log message.
- git push origin <branchName> /// Pushes commits to the remote repo on the specified branch (or creates one if it doesn't exist).
- git checkout <commitHash> // Checks out the specified commit in your project so you can work with it.
- git branch <newBranchName> <commitHash> // Creates a new branch starting from the provided commit hash.
Create A Git Branch From Git Tag
Consider a situation where you are working on a project with multiple developers. The requirement is to create a Git tag in the master branch and then have other developers work off that tag by creating different branches.
The Process to Git Create Branch From Tag:
To create a branch from an existing tag in Git, use the git checkout command along with the -b option followed by your desired name for the new branch.
For example, the git checkout -b <new-branch> <existing-tag> command. This will check out all files associated with that tag into your newly created feature branch so you can begin editing those files as needed. Once you’re done making changes to this feature/bugfix branch, push them back up using the git push origin <new-branch> command.
List of commands:
- git checkout: This command checks out a branch or tag from your local repository. For example, git checkout <branch/tag>.
- git checkout -b: The -b flag allows you to create and switch to a new branch simultaneously using one command. For example, you would use a syntax like git checkout –b <newBranchName> [existingTag].
- git push origin <nameOfNewBranch>: Once any changes are made in your feature/bugfix branch, that needs to be reflected upstream into master (or another development environment). Just run this simple command while pointing to the created new branch with its name as a parameter for its contents, and code changes get uploaded back up again on the central repo.
Create A Git Branch Using The Detached HEAD State
A Detached HEAD state occurs when you are no longer connected to any branch in a Git repository. This can happen if you check out an old commit or switch branches without creating a new one. In this situation, all commits will be orphaned and tracked by the repository once reconnected to some existing branch or another newly created one.
The Process of Git Create branch Using The Detached HEAD
It allows developers to continue their work as usual while preserving any changes they made previously, which were temporarily disconnected from branches in Git repositories.
- To create a new branch from your detached head state, use the git checkout -b <new-branch-name> command.
- You may specify where it should begin tracking commits with the --track command like git checkout --track origin/<existing_brach>.
- With either of these commands, after entering them into the terminal window, you should have successfully reattached to version control and created your copy of an existing repository.
List of commands
- git checkout <commit ID/branch> - This command will switch to a specific commit or branch, putting your repository into a detached head state.
For example, git checkout 7d7e33. - git log --online --decorate - This command can view the commits still available after switching into the detached head state; it helps you find an existing commit or branch from which you want to restart tracking again.
For example, git log --online --decorate. - git reflog - Similar to the previous command but using reflog, this will display references only for the local repository and not the remote one, hence helping developers see all their past commits quickly as well identify those currently disconnected due to lack of tracking (i.e., in Detached Head State).
For example, git reflog. - git reset [--hard] - If needed, this allows users to undo changes made in Git repositories while they were previously in a detached HEAD state (for instance, if there was some mistake made during that period). It is important to mention that the '–hard' flag should be used with caution since its effects are irreversible! So, use it carefully when running this command.
For example, git reset --hard. - git checkout -b <new-branch-name> - This is an important step that allows developers to create a new branch from their current detached head state to get back on version control without any disruption or loss of past changes.
For example, git checkout -b my_new_feature.
Create A Git Branch From A Remote Branch
A remote branch is a version of the code that exists on a machine different from the one you are currently using. It can be accessed remotely through SSH or FTP, and its changes can be tracked in real time. Remote branches provide a separate environment for testing new features or making experimental changes without altering your local development environment until you’re comfortable with them.
The Process to Git Create Branch from a Remote Branch:
Creating a branch from a remote branch involves cloning that repository to create an exact copy on your computer.
- From there, you can open up the project files and make any necessary adjustments within them.
- After that, push those revisions back up to their original location as part of creating your distinct feature branch off of it.
- This allows developers working collaboratively on projects to quickly test their ideas before committing them to official production channels. Thus helping ensure higher quality control over product releases and bug patches.
List of commands
- git clone <remote-repository> - Clones a remote repository into your local working directory, creating an exact copy of its contents and allowing you to work with it on your computer. Example: git clone https://github.com/username/project-name.
- git checkout -b <new-branch> - Creates a new branch from the current HEAD (or specified commit) and then checks out that branch’s code so you can work on it immediately afterward. Example: git checkout -b feature_x.
- git push origin <new-branch> - Pushes any changes made within this new branch back up to the source repository as part of creating an independent feature or patch off of its base codebase for further exploration by other developers downstream if desired later on down the line.
Example: git push origin feature_x.
Create A Git Branch In The Remote Repository
Remote repositories are online storage locations that exist on remote machines or servers. They serve as a central hub for developers to work collaboratively and provide version control so changes over time can be tracked and undone if necessary. Any changes made in the repository will need to be pulled from the remote server before other collaborators can use them.
The Process to Git Create Branch in a Remote Repository
This process allows developers to create separate versions of projects without affecting anyone else's work. It is helpful when working with multiple teams who might want different features developed simultaneously but don't necessarily want their progress to conflict with each other's results yet still allow them access to one another's advances.
While all code within branches follows along its track, it is still stored under the master branch, allowing everyone involved to see what any new addition looks like when combined into one final product or realizing your project goal(s).
List of commands
- git clone: This command creates a local copy of the remote repository on the computer. Example: git clone <URL>.
- git fetch: Used to fetch and download new commits from the remote branch without merging them into your local branch. Example: git fetch origin/master.
- git pull: Fetches all changes from the remote tracking branch (e.g., master) and merges them into your local working directory so you can begin work with the latest codebase version. Example: git pull origin/master.
- git checkout -b <branch-name>: Creates a new feature or experiment in separate Git branches based on existing main project source code example, checkout & branch where it creates and switches to newly created full commit history for that specific purpose. Example: git checkout –b myfeature.
- git push --set-upstream origin <branch_name>: Pushes (uploads) all content associated with this particular set upstream onto an existing populated repository. This creates space for active collaboration among multiple users when creating features together but ensures everyone stays updated as progress continues across different platforms by notifying each other of newly pushed content. Example: git push –set-upstream origin feature.
Creating A Git Branch Using The Branch Dropdown Menu
The branch dropdown menu in a version control system is essentially a list of your project's branches or versions. It allows you to select an existing branch from the branch dropdown menu and use it as the basis for creating new changes within that particular branch.
The primary purpose behind this feature is to simplify the merging process by ensuring each change only affects one specific version (or set of code) at any given time. Coding teams can avoid manually merging multiple files together before releasing updates into production.
Process of Creating a Git Branch Using the Branch Dropdown Menu
- First, visit the beach dropdown menu and click on the 'Create New Branch' option from the list. Then providing a name and set an upstream repository if necessary.
- After naming your latest creation, you can see what has changed since then compared to other versions available on that same page.
- You can then examine whether or not these modifications are worth adding permanently by merging them into another existing release candidate within this same UI, too.
List of commands
- git init: This command initializes a local git repository in the current directory and is used when setting up version control for an existing project. Example: git init.
- git clone: This command copies all files from one remote repository to your local computer. It creates a full copy of the project on your machine that can be edited independently of its source location while remaining linked with it for future commits back upstream if needed). Example: git clone https://github/exampleuser/repo-name.
- git add: The command adds new or modified files in the working directory to the commit staging area, and they get ready to go into the next commit upon user confirmation (through 'commit' commands). Example: git add.
- git commit -m <message>: The commit takes all changes added previously and allows you to set a message explaining why these changes were made. This helps other developers understand the changes quickly and lets them know who was responsible. Examples: GIT Commit -m "Fixed bug #123.
- git push origin <branch_name>: Finally, this pushes our committed code change.
Creating A Git Branch Using The Branch Overview
The branch overview is when a user creates a repository with different branches that the organization can use. In this scenario, each branch represents an isolated workspace where teams and members can work on different tasks without affecting the main development process. Note that every Git branch functions as its independent project within the larger codebase.
The Process of Creating a Branch Using Branch Overview
It typically involves navigating to your organizational dashboard or page in GitHub and accessing your list of repositories there.
- From this point, you should see all your existing branches listed and an option for creating a New Branch at the top (or under Actions).
- After clicking this button, it will prompt you to enter some details, such as name and description, before saving it to create your new branch.
- Once saved, all changes should appear on-screen, demonstrating that they have been successfully created!
List of commands:
- git checkout: This command is used to switch between existing or create a new branch from an existing one. Example: git checkout master, which will switch you to the master branch.
- git branch: This command lists all available branches in your repository, locally and remotely. Example: git branch -a, which will display all current branches.
- git-checkout -b [branch_name]: This creates a new local working copy of your remote repository by specifying the name of the new desired local branching point after the -b flag when using this command. It checks out any changes made upstream for provided arguments before creating the newly mentioned feature named after the provided argument immediately afterward. Example: git checkout – b newBranchName will create a brand-new directory with a given name.
Git Create Branch In Visual Studio
As you know, Git is a version control system that allows developers to manage their source code and track changes over time. It can store any digital asset, such as text documents, photographs, or software projects.
Visual Studio integrated with Git makes it easy for developers to create new branches to work on different features or fix bugs without affecting the main branch (master). Creating branches allows developers to make separate commits within each branch and switch between them whenever needed.
The Process to Create A Git Branch in Visual Studio Using Git:
Step 1: Open up Visual Studio and go to the View menu option at the top left-hand side of your screen. Here, select the 'Team Explorer' option.
Example: The user has opened Visual Studio and is trying to access Team Explorer. To do so, they can open it to View->Team Explorer.
Step 2: Select the 'Branches' option under the Local Git Repositories section.
Example: The user must access a repository's branches list to view all existing local or remote branches. They can select Branches under Local Git Repositories within Team Explorer.
Step 3: Click the 'New Branch' button to open the Create New Branch Dialog box, where you need to enter the name of your newly created branch.
Example: To create a new branch with its name, the user must click on the New Branch button, which appears after clicking on 'Branches' within the team explorer window. This will open the 'Create New Branch' dialog box, wherein they must input their desired name for that particular branch.
Step 4: You also have an option if you want this newly created branch to be based on other existing local/remote branches by clicking the 'Compare with another Branch' dropdown list just below the Computer field.
Example: The user can make the recently created branch dependent on a different existing one. To do so, they can scroll down the Create New Branches Dialog window and click on the 'Compare with another Branch' dropdown list, just below the 'Computer' Field.
Step 5: Now click the Create button so that your new local git branch will be created.
Example: After specifying all requirements (name of the desired new/local Git Branch and comparison of it), the user needs to press the 'Create' button so that their request will not remain unfulfilled & Local GIT branch gets successfully generated.
How To Delete A Git Branch?
Deletion of branches is a vital process in the Git revision control workflow. You can delete a local or remote Git branch deletion is done using the commands below:
- To delete a local git branch, use the branch command:
$ git branch -d <branch_name> - To delete a remote git branch, use this branch command:
$git push origin --delete <branch_name>
For more, read: Git Delete Branch | Local & Remote Branches With Examples
Conclusion
Git branches allow developers to work on new and existing features safely. They also allow users to test changes without affecting other parts of the repository, track multiple lines of development in one project, and merge when these features are ready. There are many ways to create Git branches, such as creating a branch from another branch or tag.
You can also use commands such as git checkout -b to create Git branches. Note that while following the process to Git create branch, you must also understand the best practices for naming your branches. It will help ensure that files are tracked efficiently by version control systems. Once you are done with the branch and no longer need it, you can delete the Git branches, either local or remote. For this, you must use the command git push origin --delete command.
Frequently Asked Questions
Q. What is a base branch in Git?
A base branch in Git is the main development line from which all other branches originate and merge when specific features or updates are completed. A base branch is the primary version of a codebase and serves as the common ancestor for any other branches created in a project repository. It is a foundation for developers to create custom edits without altering or affecting existing features.
Q. How do you switch between different branches on Git?
You can switch branches on Git using the git checkout <branch name> command. This command will check out your specified branch, making it your current working branch.
The command git branch can also list and switch between all available branches. When we want to create a new branch, we can run the git checkout -b <new-branch> command, which will created from your current working branch.
Q. What is a default branch in Git?
A default branch in Git represents the main development line for any project hosted using this version control system by indicating where new contributions should be made from existing feature/bug fixing activities, etc. By convention, most repositories have created either master as their default or often also set up trunk as its trunk-line location – essentially synonymous terms here.
Q. What does hot fix mean with regard to branching strategy in GIT?
Hotfixes refer to quick patches deployed quickly onto production environments without doing much testing around these release notes. They are usually done during emergency scenarios like patch releases needed urgently due to security vulnerabilities found at run time. These usually stem from a dedicated branch created for the task and merged back into production with minimal review cycles.
Q. What is a pointer in Git?
A pointer or ref in Git represents an immutable repository state that points to commits, tags, branches, etc. It lets users quickly navigate any given commit history along its respective timeline by referring to it directly (e.g., HEAD / MASTER pointers).
Q. When do you create a branch on issues using GIT?
Branches are typically used when you want to introduce new features, other changes, or fix bugs without disrupting the existing codebase. They can also be used as temporary development sandboxes during experimentation to keep track of different versions being worked upon simultaneously. By creating separate branches related to certain tasks/issues, developers can easily collaborate while keeping their work organized and avoid conflicting with one another's progress.
Q. How does branching off from master help organize your work better?
Branching off from the master helps organize your work better by allowing you to separate areas within specific tasks. For example, tasks like feature implementations, bug fixes, etc., can be done independently, enabling focused collaboration amongst members with access rights over said directory tree structure. This allows for far better tracking of changes & ensures minimal disruption when releasing updates.
Q. What is a branch pointer in GIT?
A branch pointer or ref in Git points to either commits, tags, or branches by providing an immutable repository state for quick navigation through its respective timeline. It's been used as a shortcut mechanism that can be pointed towards specific locations within a project like master/HEAD, etc., so users don't have to look up every single commit manually while traversing down its entire history stack.
This compiles our discussion on how to create a Git branch. You might also be interested in reading the following:
- Git Hooks | Definition, Usage, Types, Workflow & More (+Examples)
- Git Cherry Pick Command | How To Use, Undo, Resolve Conflicts & More
- What Is GitHub? An Introduction, How-To Use It, Components & More
- Git Rebase | Strategies, Commands, Best Practices, Examples & More
- Git Vs. GitHub | 11 Differences, Applications, Prices & More
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment