- What Is Data Structure?
- Key Features Of Data Structures
- Basic Terminologies Related To Data Structures
- Types Of Data Structures
- What Are Linear Data Structures & Its Types?
- What Are Non-Linear Data Structures & Its Types?
- Importance Of Data Structure In Programming
- Basic Operations On Data Structures
- Applications Of Data Structures
- Real-Life Applications Of Data Structures
- Linear Vs. Non-linear Data Structures
- What Are Algorithms? The Difference Between Data Structures & Algorithms
- Conclusion
- Frequently Asked Questions
- What Is Asymptotic Notation?
- How Asymptotic Notation Helps In Analyzing Performance
- Types Of Asymptotic Notation
- Big-O Notation (O)
- Omega Notation (Ω)
- Theta Notation (Θ)
- Little-O Notation (o)
- Little-Omega Notation (ω)
- Summary Of Asymptotic Notations
- Real-World Applications Of Asymptotic Notation
- Conclusion
- Frequently Asked Questions
- Understanding Big O Notation
- Types Of Time Complexity
- Space Complexity In Big O Notation
- How To Determine Big O Complexity
- Best, Worst, And Average Case Complexity
- Applications Of Big O Notation
- Conclusion
- Frequently Asked Questions
- What Is Time Complexity?
- Why Do You Need To Calculate Time Complexity?
- The Time Complexity Algorithm Cases
- Time Complexity: Different Types Of Asymptotic Notations
- How To Calculate Time Complexity?
- Time Complexity Of Sorting Algorithms
- Time Complexity Of Searching Algorithms
- Writing And optimizing An algorithm
- What Is Space Complexity And Its Significance?
- Relation Between Time And Space Complexity
- Conclusion
- What Is Linear Data Structure?
- Key Characteristics Of Linear Data Structures
- What Are The Types Of Linear Data Structures?
- What Is An Array Linear Data Structure?
- What Are Linked Lists Linear Data Structure?
- What Is A Stack Linear Data Structure?
- What Is A Queue Linear Data Structure?
- Most Common Operations Performed in Linear Data Structures
- Advantages Of Linear Data Structures
- What Is Nonlinear Data Structure?
- Difference Between Linear & Non-Linear Data Structures
- Who Uses Linear Data Structures?
- Conclusion
- Frequently Asked Questions
- What is a linear data structure?
- What is a non-linear data structure?
- Difference between linear data structure and non-linear data structure
- FAQs about linear and non-linear data structures
- What Is Search?
- What Is Linear Search In Data Structure?
- What Is Linear Search Algorithm?
- Working Of Linear Search Algorithm
- Complexity Of Linear Search Algorithm In Data Structures
- Implementations Of Linear Search Algorithm In Different Programming Languages
- Real-World Applications Of Linear Search In Data Structure
- Advantages & Disadvantages Of Linear Search
- Best Practices For Using Linear Search Algorithm
- Conclusion
- Frequently Asked Questions
- What Is The Binary Search Algorithm?
- Conditions For Using Binary Search
- Steps For Implementing Binary Search
- Iterative Method For Binary Search With Implementation Examples
- Recursive Method For Binary Search
- Complexity Analysis Of Binary Search Algorithm
- Iterative Vs. Recursive Implementation Of Binary Search
- Advantages & Disadvantages Of Binary Search
- Practical Applications & Real-World Examples Of Binary Search
- Conclusion
- Frequently Asked Questions
- Understanding The Jump Search Algorithm
- How Jump Search Works?
- Code Implementation Of Jump Search Algorithm
- Time And Space Complexity Analysis
- Advantages Of Jump Search
- Disadvantages Of Jump Search
- Applications Of Jump Search
- Conclusion
- Frequently Asked Questions
- What Is Sorting In Data Structures?
- What Is Bubble Sort?
- What Is Selection Sort?
- What Is Insertion Sort?
- What Is Merge Sort?
- What Is Quick Sort?
- What Is Heap Sort?
- What Is Radix Sort?
- What Is Bucket Sort?
- What Is Counting Sort?
- What Is Shell Sort?
- Choosing The Right Sorting Algorithm
- Applications Of Sorting
- Conclusion
- Frequently Asked Questions
- Understanding Bubble Sort Algorithm
- Bubble Sort Algorithm
- Implementation Of Bubble Sort In C++
- Time And Space Complexity Analysis Of Bubble Sort Algorithm
- Advantages Of Bubble Sort Algorithm
- Disadvantages Of Bubble Sort Algorithm
- Applications of Bubble Sort Algorithms
- Conclusion
- Frequently Asked Questions
- Understanding The Merge Sort Algorithm
- Algorithm For Merge Sort
- Implementation Of Merge Sort In C++
- Time And Space Complexity Analysis Of Merge Sort
- Advantages And Disadvantages Of Merge Sort
- Applications Of Merge Sort
- Conclusion
- Frequently Asked Questions
- Understanding The Selection Sort Algorithm
- Algorithmic Approach To Selection Sort
- Implementation Of Selection Sort Algorithm
- Complexity Analysis Of Selection Sort
- Comparison Of Selection Sort With Other Sorting Algorithms
- Advantages And Disadvantages Of Selection Sort
- Application Of Selection Sort
- Conclusion
- Frequently Asked Questions
- What Is Insertion Sort Algorithm?
- How Does Insertion Sort Work? (Step-by-Step Explanation)
- Implementation of Insertion Sort in C++
- Time And Space Complexity Of Insertion Sort
- Applications Of Insertion Sort Algorithm
- Comparison With Other Sorting Algorithms
- Conclusion
- Frequently Asked Questions
- Understanding Quick Sort Algorithm
- Step-By-Step Working Of Quick Sort
- Implementation Of Quick Sort Algorithm
- Time Complexity Analysis Of Quick Sort
- Advantages Of Quick Sort Algorithm
- Disadvantages Of Quick Sort Algorithm
- Applications Of Quick Sort Algorithm
- Conclusion
- Frequently Asked Questions
- Understanding The Heap Data Structure
- Working Of Heap Sort Algorithm
- Implementation Of Heap Sort Algorithm
- Advantages Of Heap Sort
- Disadvantages Of Heap Sort
- Real-World Applications Of Heap Sort
- Conclusion
- Frequently Asked Questions
- Understanding The Counting Sort Algorithm
- Conditions For Using Counting Sort Algorithm
- How Counting Sort Algorithm Works?
- Implementation Of Counting Sort Algorithm
- Time And Space Complexity Analysis Of Counting Sort
- Comparison Of Counting Sort With Other Sorting Algorithms
- Advantages Of Counting Sort Algorithm
- Disadvantages Of Counting Sort Algorithm
- Applications Of Counting Sort Algorithm
- Conclusion
- Frequently Asked Questions
- Understanding Shell Sort Algorithm
- Working Of Shell Sort Algorithm
- Implementation Of Shell Sort Algorithm
- Time Complexity Analysis Of Shell Sort Algorithm
- Advantages Of Shell Sort Algorithm
- Disadvantages Of Shell Sort Algorithm
- Applications Of Shell Sort Algorithm
- Conclusion
- Frequently Asked Questions
- What Is Linked List In Data Structures?
- Types Of Linked Lists In Data Structures
- Linked List Operations In Data Structures
- Advantages And Disadvantages Of Linked Lists In Data Structures
- Comparison Of Linked Lists And Arrays
- Applications Of Linked Lists
- Conclusion
- Frequently Asked Questions
- What Is A Singly Linked List In Data Structure?
- Insertion Operations On Singly Linked Lists
- Deletion Operation On Singly Linked List
- Searching For Elements In Single Linked List
- Calculating Length Of Single Linked List
- Practical Applications Of Singly Linked Lists In Data Structure
- Common Problems With Singly Linked Lists
- Conclusion
- Frequently Asked Questions (FAQ)
- What Is A Linked List?
- Reverse A Linked List
- How To Reverse A Linked List? (Approaches)
- Recursive Approach To Reverse A Linked List
- Iterative Approach To Reverse A Linked List
- Using Stack To Reverse A Linked List
- Complexity Analysis Of Different Approaches To Reverse A Linked List
- Conclusion
- Frequently Asked Questions
- What Is A Stack In Data Structure?
- Understanding Stack Operations
- Stack Implementation In Data Structures
- Stack Implementation Using Arrays
- Stack Implementation Using Linked Lists
- Comparison: Array vs. Linked List Implementation
- Applications Of Stack In Data Structures
- Advantages And Disadvantages Of Stack Data Structure
- Conclusion
- Frequently Asked Questions
- What Is A Graph Data Structure?
- Importance Of Graph Data Structures
- Types Of Graphs In Data Structure
- Types Of Graph Algorithms
- Application Of Graphs In Data Structures
- Challenges And Complexities In Graphs
- Conclusion
- Frequently Asked Questions
- What Is Tree Data Structure?
- Terminologies Of Tree Data Structure:
- Different Types Of Tree Data Structures
- Basic Operations On Tree Data Structure
- Applications Of Tree Data Structures
- Comparison Of Trees, Graphs, And Linear Data Structures
- Advantages Of Tree Data Structure
- Disadvantages Of Tree Data Structure
- Conclusion
- Frequently Asked Questions
- What Is Dynamic Programming?
- Real-Life Example: The Jigsaw Puzzle Analogy
- How To Solve A Problem Using Dynamic Programming?
- Dynamic Programming Algorithm Techniques
- Advantages Of Dynamic Programming
- Disadvantages Of Dynamic Programming
- Applications Of Dynamic Programming
- Conclusion
- Frequently Asked Questions
- Understanding The Sliding Window Algorithm
- How Does The Sliding Window Algorithm Works?
- How To Identify Sliding Window Problems?
- Fixed-Size Sliding Window Example: Maximum Sum Subarray Of Size k
- Variable-Size Sliding Window Example: Smallest Subarray With A Given Sum
- Advantages Of Sliding Window Technique
- Disadvantages Of Sliding Window Technique
- Conclusion
- Frequently Asked Questions
- Introduction To Data Structures
- Data Structures Interview Questions: Basics
- Data Structures Interview Questions: Intermediate
- Data Structures Interview Questions: Advanced
- Conclusion
What Is Linear Search? Algorithm, Working, Complexity & Examples
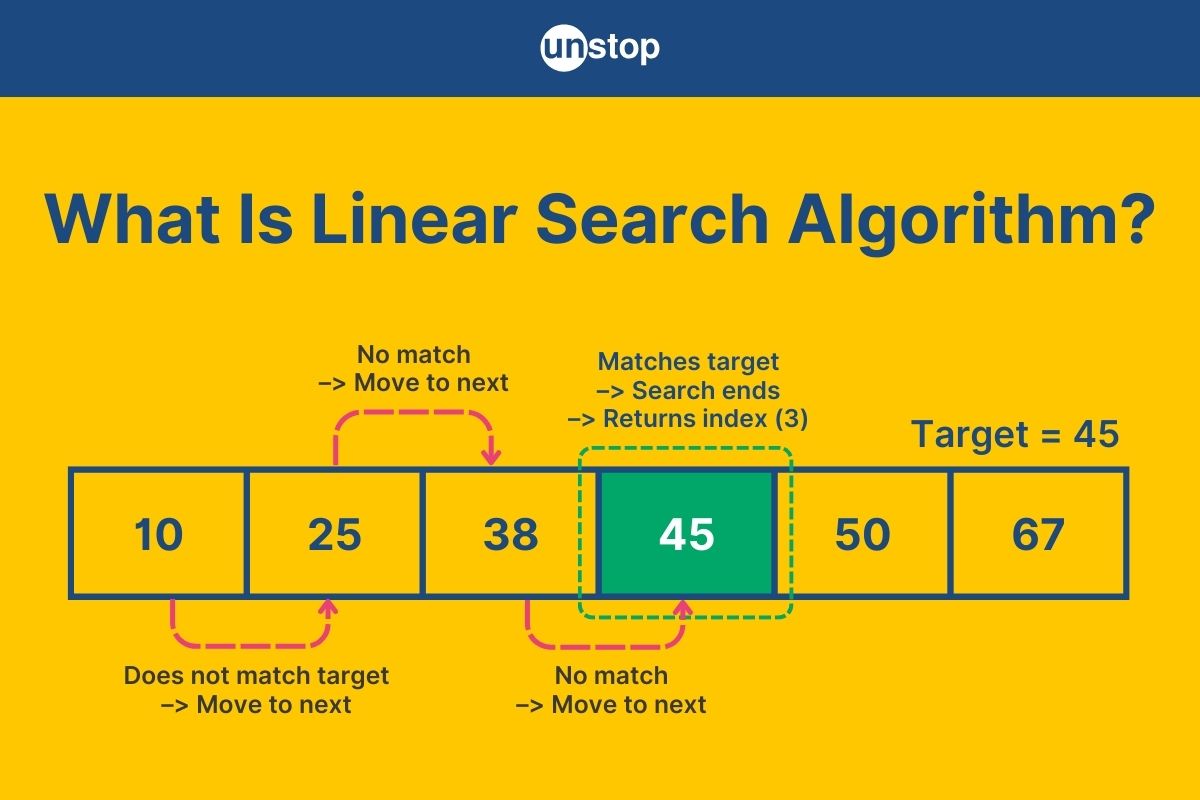
In today’s data-driven world, the ability to quickly and efficiently locate information is paramount. Whether you're sifting through a small dataset or scouring vast arrays of information, search algorithms are at the heart of these operations. In this article, we'll explore one of the most fundamental search techniques–Linear Search, breaking down its mechanics, applications, and advantages.
We have provided clear explanations and practical code examples to help you understand and implement linear search algorithms in your projects.
What Is Search?
At its core, search/searching is the process of finding a specific element or a group of elements within a collection of data. Search plays a crucial role in data structures by enabling quick access to required information from data sets that may vary widely in size and complexity.
- Searching involves scanning through a data structure–such as an array, list, or database–to locate an item that meets a specific criterion. This could be anything from finding a particular number in a list to retrieving a record from a database.
- Efficient searching is vital because it directly impacts the performance of software applications. Whether you're working on simple scripts or complex systems, the ability to search effectively can determine how fast your application responds and how well it scales.
- There are several methods for searching, each with its own use cases and performance characteristics. Linear search, which we are going to discuss in detail here, is one of the simplest forms and serves as a stepping stone to understanding more advanced search algorithms.
In the sections that follow, we’ll dive deep into the specifics of linear search in data structure–what it is, how it works, and where it’s best applied–providing you with a clear and actionable understanding of this fundamental algorithm.
What Is Linear Search In Data Structure?
Linear search, sometimes referred to as sequential search, is one of the simplest search methods used in computer science. This method works by iterating through each element in a data structure–such as an array or list–until the target element is found or the entire data structure has been examined.
Key Characteristics Of Linear Search
- Sequential Process: Linear search goes through the data structure one element at a time. It starts at the beginning and moves sequentially to the end, checking each element against the target value.
- Simplicity: Due to its straightforward approach, linear search is extremely easy to implement. This makes it an excellent choice for beginners learning about search algorithms and for scenarios where the dataset is relatively small or unsorted.
- Versatility: Unlike more complex algorithms, linear search does not require the data to be sorted. This flexibility means it can be applied to a variety of data structures without the need for pre-processing or additional constraints.
Practical Considerations When Using Linear Search In Data Structure
- Efficiency: While linear search is easy to understand and implement, its time complexity is O(n), meaning that in the worst case, it might need to inspect every element in the dataset. This makes it less efficient for large datasets compared to more advanced algorithms like binary search, which require sorted data.
- Memory Usage: One of the advantages of linear search is its minimal memory requirement–it typically only uses a constant amount of extra space (O(1)).
In summary, linear search is a fundamental technique that offers an excellent introduction to searching algorithms. Its ease of use and adaptability make it a valuable tool, especially when dealing with small or unsorted datasets where the overhead of more complex algorithms might not be justified.
What Is Linear Search Algorithm?
The Linear Search Algorithm is a method for finding a target value within a list by sequentially checking each element until the desired element is found or the list is exhausted. This algorithm is straightforward and intuitive, making it a great starting point for understanding more complex searching techniques. Here is a step-by-step breakdown of a linear search algorithm and its working:
- Initialize the Search: Start from the first element of the data structure.
- Compare Each Element: For each element in the data structure, compare it with the target value. If the element matches the target, record its index (or return it immediately). The search ends and returns the index.
- Continue Until the End: If the current element does not match the target, move to the next element in the data structure.
- Return the Result: If the target is found, return its position (index). If the search reaches the end of the list without finding the target, return a special value (e.g., -1) indicating that the target is not present.
Pseudocode For Linear Search Algorithm
FUNCTION linearSearch(array, target):
FOR index FROM 0 TO LENGTH(array) - 1:
IF array[index] == target:
RETURN index // Target found, return the index
END FOR
RETURN -1 // Target not found in the array
Explanation:
- Initialization: The algorithm begins at index 0 of the array.
- Iteration: It then iterates over each element in the array, comparing it with the target value.
- Conditional Check: If an element equals the target, the function immediately returns the index of that element.
- Completion: If no match is found after checking all elements, the function returns -1 to indicate the target does not exist within the array.
This step-by-step approach ensures that every element is examined, making the linear search a reliable, albeit not always the most efficient, method for finding an element in a list.
Working Of Linear Search Algorithm
To understand how the linear search algorithm works, let's break it down step by step with a visual representation. Consider an array of integers and a target value to be searched:
arr = [10, 25, 38, 45, 50, 67]
Target: 45
Step 1: Start from the first element
- Compare arr[0] (10) with 45.
- Since 10 ≠ 45, move to the next element.
Step 2: Compare the second element
- Compare arr[1] (25) with 45.
- Since 25 ≠ 45, move to the next element.
Step 3: Compare the third element
- Compare arr[2] (38) with 45.
- Since 38 ≠ 45, move to the next element.
Step 4: Compare the fourth element
- Compare arr[3] (45) with 45.
- Since 45 = 45, the target is found at index 3.
- The search stops, and the index 3 is recorded.
At this point, since the target element 45 is found at index 3, the function returns 3.
What Happens If the Target Is Not Found?
If the search reaches the end of the array without finding the target, it returns -1 to indicate that the element is not present. For example, when searching for 99 in the same array, the algorithm will check each element, but since 99 is not in the array, it will return -1.
This step-by-step approach ensures a clear understanding of how linear search operates.
Complexity Of Linear Search Algorithm In Data Structures
The efficiency of an algorithm is measured in terms of time complexity and space complexity. Since linear search is a simple sequential search method, its performance depends on the size of the dataset. Let’s analyze it in detail.
Time Complexity Of Linear Search
The time complexity of linear search is determined by the number of comparisons it makes to find the target element. The table below describes the time complexity for various cases:
Case |
Scenario |
Time Complexity |
Best Case |
Target is found at the first index |
O(1) |
Average Case |
Target is found somewhere in the middle |
O(n/2) ≈ O(n) |
Worst Case |
Target is at the last index or not present |
O(n) |
As evident in the table above, the key takeaways for time complexity :
- Best Case (O(1)) → If the target element is at the very first position, the algorithm stops immediately, making it a constant-time operation.
- Average Case (O(n)) → On average, the target will be found somewhere around the middle of the dataset, requiring approximately n/2 comparisons (but still expressed as O(n) in Big-O notation).
- Worst Case (O(n)) → If the target is at the last position or not in the list at all, the algorithm must traverse the entire dataset, making n comparisons.
Space Complexity Of Linear Search
Linear search operates in place, meaning it does not require extra memory apart from a few variables.
- Space Complexity: O(1)
- Why? The algorithm only uses a few variables (such as loop counters and the target value) regardless of the input size, keeping space consumption constant.
Key Takeaways:
- Time Complexity: Linear in the worst case (O(n)), but can be very fast (O(1)) if the target is found early.
Space Complexity: Constant (O(1)), making it memory-efficient.
Linear search is useful for small or unsorted datasets, but for larger data sets, more efficient algorithms like binary search (for sorted data) are preferred.
Implementations Of Linear Search Algorithm In Different Programming Languages
The linear search algorithm can be implemented in various programming languages. Below, we provide implementations in C, C++, Python, and Java, including the code, output, and explanation.
Linear Search Program In C
#include <stdio.h>
int linearSearch(int arr[], int size, int target) {
for (int i = 0; i < size; i++) {
if (arr[i] == target)
return i; // Return index if found
}
return -1; // Return -1 if not found
}
int main() {
int arr[] = {10, 25, 38, 45, 50, 67};
int target = 45;
int size = sizeof(arr) / sizeof(arr[0]);
int result = linearSearch(arr, size, target);
if (result != -1){
printf("Element found at index %d\n", result);
}else{
printf("Element not found\n");
}
return 0;
}
Output:
Element found at index 3
Code Explanation:
We begin by including the header file <stdio.h> for input/output operations.
- Function Definition: We define a function linearSearch(), which iterates through the array using a for loop.
- Inside the loop, we have an if statement which checks if the current array element is equal to the target.
- If the target element is found, it returns the index.
- If the loop completes without finding the element, it returns -1.
- Main Function Execution: We create an array, arr, containing 6 integer data type elements.
- Then, we declare and initialize a variable target with the value 45.
- Next, we calculate the size of the array using the sizeof the operator.
- We then call the function linearSearch(), passing the array, size, and target as arguments. The outcome the function returns is stored in the result variable.
- Result Handling: We use an if-else statement to check if the result is not -1 using the not equal to relational operator.
- If the condition is true, we print the index value with a string message. If the condition i false, then the else block executes printing the string message– “Element not found”.
Linear Search Program In C++
#include <iostream>
using namespace std;
int linearSearch(int arr[], int size, int target) {
for (int i = 0; i < size; i++) {
if (arr[i] == target)
return i; // Return index if found
}
return -1; // Return -1 if not found
}
int main() {
int arr[] = {10, 25, 38, 45, 50, 67};
int target = 45;
int size = sizeof(arr) / sizeof(arr[0]);
int result = linearSearch(arr, size, target);
if (result != -1) {
cout << "Element found at index " << result << endl;
} else {
cout << "Element not found" << endl;
}
return 0;
}
Output:
Element found at index 3
Code Explanation:
- Header File: We include <iostream> for input and output operations.
- Function Definition: We define the C++ function linearSearch() that uses a for loop to iterate through the input array.
- In every iteration, it uses an if-statement to check if the element matches the target.
- If an element matches the target, the function returns its index.
- If no match is found, -1 is returned.
- Main Function Execution: We declare an integer array arr with six elements of integer data type.
- We then declare a variable target and assign the integer value 45 to it.
- The array size is computed using sizeof(arr) / sizeof(arr[0]).
- We then call the function linearSearch() and store the returned value in the result.
- Result Handling: We check the result using an if-else statement.
- If result is not -1, we print the index where the element was found.
- Otherwise, we print "Element not found".
Linear Search Program In Python
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i # Return index if found
return -1 # Return -1 if not found
arr = [10, 25, 38, 45, 50, 67]
target = 45
result = linear_search(arr, target)
if result != -1:
print(f"Element found at index {result}")
else:
print("Element not found")
Output:
Element found at index 3
Code Explanation:
In the simple Python program example:
- Function Definition: We define a Python function linear_search() that iterates through the list using a for loop with the range() function, i.e., range(len(arr)).
- The range of the for loop iterations is set to the length of the array, calculated using the len() function.
- In every iteration, we check if the current array element equals the target using the equality relational operator.
- If an element matches the target, the function returns the index.
- If no match is found, the function returns -1.
- Main Execution: We create a list, arr with six elements.
- Then, we declare and initialize a variable target with the value 45.
- Next, we call the linear_search() function and store the outcome in the variable result.
- Result Handling: We use an if-else statement to check if the result is not -1.
- If the result is not -1, we use the print() function to display the index where the element is found.
- Otherwise, we print the string message– "Element not found".
Linear Search Program In Java
public class Main {
public static int linearSearch(int arr[], int target) {
for (int i = 0; i < arr.length; i++) {
if (arr[i] == target)
return i; // Return index if found
}
return -1; // Return -1 if not found
}
public static void main(String[] args) {
int arr[] = {10, 25, 38, 45, 50, 67};
int target = 45;
int result = linearSearch(arr, target);
if (result != -1) {
System.out.println("Element found at index " + result);
} else {
System.out.println("Element not found");
}
}
}
Output:
Element found at index 3
Code Explanation:
- Class & Method Definition: We define a class LinearSearch containing a method linearSearch().
- The method iterates through the array using a for loop control statement.
- If an element matches the target, it returns the index; otherwise, it returns -1.
- Main Execution: We declare an integer array arr with six elements.
- The target variable is set to 45.
- We call linearSearch() and store the result.
- Result Handling:
- If the result is not -1, the index is printed.
- Otherwise, "Element not found" is displayed.
Real-World Applications Of Linear Search In Data Structure
Linear search is simple yet useful in scenarios where other complex search algorithms might be unnecessary or impractical. Here are some real-world applications:
- Finding a contact in a small, unsorted list– If you scroll through a phone contact list that isn’t sorted, you're essentially using linear search.
- Searching for an item in an inventory– Retail systems often scan small product lists sequentially when no indexing or sorting is available.
- Looking up a student's record in an attendance sheet– If student names are listed in order of entry rather than alphabetical order, a manual search follows the linear search principle.
- Detecting a specific keyword in a document– In text editors without advanced search indexing, checking word by word mimics linear search.
- Checking for a missing component in a toolkit– If you go through tools one by one to check if something is missing, you’re applying a linear search method.
Advantages & Disadvantages Of Linear Search
Linear search is straightforward but not always the most efficient method. Here’s a comparison of its pros and cons:
Advantages |
Disadvantages |
|
|
Best Practices For Using Linear Search Algorithm
To make the most of linear search, follow these best practices:
- Use it for small datasets– Linear search is efficient when dealing with small lists, where its simplicity outweighs the inefficiency of O(n) time complexity.
- Prefer it for unsorted data– When sorting isn’t feasible, linear search can be a good choice for quick lookups.
- Optimize with early termination– If you only need to check for existence (not count occurrences), return as soon as the element is found to avoid unnecessary iterations.
- Consider alternative searches for large datasets– For large collections, use binary search (if sorted) or hash-based searching (if frequent lookups are needed) to improve efficiency.
- Use linear search when dealing with linked lists– Since linked lists don’t allow direct access by index, linear search is often the simplest way to find elements.
Conclusion
Linear search is one of the simplest search algorithms, making it a great starting point for beginners in data structures and algorithms. While its O(n) time complexity makes it inefficient for large datasets, it remains useful for small, unsorted lists and certain real-world applications like searching in text documents or looking up records in an attendance sheet.
By understanding how linear search works, its advantages, limitations, and best use cases, you can make informed decisions on when to apply it or switch to more efficient search techniques like binary search or hash-based searching. Whether you're working with arrays, linked lists, or real-world data, knowing when and where to use linear search will strengthen your problem-solving skills.
Frequently Asked Questions
Q1. What are the scenarios where linear search is most effective?
Linear search is best for small, unsorted datasets where the overhead of sorting or using advanced search structures isn't justified. It's also useful for linked lists and when searching for the first occurrence of an element in an unsorted list.
Q2. How does linear search compare to other searching algorithms?
Linear search is simpler but slower than algorithms like binary search (O(log n)) or hash-based searches (O(1) in best cases). However, unlike binary search, it does not require sorted data.
Q3. What is the worst-case time complexity of linear search?
The worst-case time complexity is O(n), which happens when the element is at the end of the list or not present at all.
Q4. Can linear search be used for searching in linked lists?
Yes, linear search is commonly used in linked lists since they do not allow direct access to elements by index.
Q5. Is there any way to optimize linear search?
Optimizations include early termination (stopping the search once the element is found) and parallel searching (splitting the dataset into parts for faster searching). However, for large datasets, binary search or hash-based search techniques are usually better.
Q6. Can linear search be used in non-linear data structures?
Yes, linear search is not only for linear data structures. It can be applied to non-linear structures like linked lists, trees, and graphs, but its efficiency varies. It works well for linked lists since they require sequential access. In trees and graphs, more efficient traversal techniques like BFS (Breadth-First Search) and DFS (Depth-First Search) are usually preferred.
This compiles our discussion on linear search. Here are a few more blogs you must explore:
- DSA Cheatsheet Unlocked: Roadmap, Boot Camp & Must-Know Resources
- 55+ Data Structure Interview Questions For 2025 (Detailed Answers)
- Time Complexity Of Algorithms: Types, Notations, Cases, and More
- Difference Between Linear Search And Binary Search (+Code Example)
- These Coding Algorithms Will Help You Crack Any Interview
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment