Data Structures & Algorithms
Table of content:
- What Is Data Structure?
- Key Features Of Data Structures
- Basic Terminologies Related To Data Structures
- Types Of Data Structures
- What Are Linear Data Structures & Its Types?
- What Are Non-Linear Data Structures & Its Types?
- Importance Of Data Structure In Programming
- Basic Operations On Data Structures
- Applications Of Data Structures
- Real-Life Applications Of Data Structures
- Linear Vs. Non-linear Data Structures
- What Are Algorithms? The Difference Between Data Structures & Algorithms
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Asymptotic Notation?
- How Asymptotic Notation Helps In Analyzing Performance
- Types Of Asymptotic Notation
- Big-O Notation (O)
- Omega Notation (Ω)
- Theta Notation (Θ)
- Little-O Notation (o)
- Little-Omega Notation (ω)
- Summary Of Asymptotic Notations
- Real-World Applications Of Asymptotic Notation
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Big O Notation
- Types Of Time Complexity
- Space Complexity In Big O Notation
- How To Determine Big O Complexity
- Best, Worst, And Average Case Complexity
- Applications Of Big O Notation
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Time Complexity?
- Why Do You Need To Calculate Time Complexity?
- The Time Complexity Algorithm Cases
- Time Complexity: Different Types Of Asymptotic Notations
- How To Calculate Time Complexity?
- Time Complexity Of Sorting Algorithms
- Time Complexity Of Searching Algorithms
- Writing And optimizing An algorithm
- What Is Space Complexity And Its Significance?
- Relation Between Time And Space Complexity
- Conclusion
Table of content:
- What Is Linear Data Structure?
- Key Characteristics Of Linear Data Structures
- What Are The Types Of Linear Data Structures?
- What Is An Array Linear Data Structure?
- What Are Linked Lists Linear Data Structure?
- What Is A Stack Linear Data Structure?
- What Is A Queue Linear Data Structure?
- Most Common Operations Performed in Linear Data Structures
- Advantages Of Linear Data Structures
- What Is Nonlinear Data Structure?
- Difference Between Linear & Non-Linear Data Structures
- Who Uses Linear Data Structures?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is a linear data structure?
- What is a non-linear data structure?
- Difference between linear data structure and non-linear data structure
- FAQs about linear and non-linear data structures
Table of content:
- What Is Sorting In Data Structures?
- What Is Bubble Sort?
- What Is Selection Sort?
- What Is Insertion Sort?
- What Is Merge Sort?
- What Is Quick Sort?
- What Is Heap Sort?
- What Is Radix Sort?
- What Is Bucket Sort?
- What Is Counting Sort?
- What Is Shell Sort?
- Choosing The Right Sorting Algorithm
- Applications Of Sorting
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Bubble Sort Algorithm
- Bubble Sort Algorithm
- Implementation Of Bubble Sort In C++
- Time And Space Complexity Analysis Of Bubble Sort Algorithm
- Advantages Of Bubble Sort Algorithm
- Disadvantages Of Bubble Sort Algorithm
- Applications of Bubble Sort Algorithms
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Merge Sort Algorithm
- Algorithm For Merge Sort
- Implementation Of Merge Sort In C++
- Time And Space Complexity Analysis Of Merge Sort
- Advantages And Disadvantages Of Merge Sort
- Applications Of Merge Sort
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Selection Sort Algorithm
- Algorithmic Approach To Selection Sort
- Implementation Of Selection Sort Algorithm
- Complexity Analysis Of Selection Sort
- Comparison Of Selection Sort With Other Sorting Algorithms
- Advantages And Disadvantages Of Selection Sort
- Application Of Selection Sort
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Insertion Sort Algorithm?
- How Does Insertion Sort Work? (Step-by-Step Explanation)
- Implementation of Insertion Sort in C++
- Time And Space Complexity Of Insertion Sort
- Applications Of Insertion Sort Algorithm
- Comparison With Other Sorting Algorithms
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Quick Sort Algorithm
- Step-By-Step Working Of Quick Sort
- Implementation Of Quick Sort Algorithm
- Time Complexity Analysis Of Quick Sort
- Advantages Of Quick Sort Algorithm
- Disadvantages Of Quick Sort Algorithm
- Applications Of Quick Sort Algorithm
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Heap Data Structure
- Working Of Heap Sort Algorithm
- Implementation Of Heap Sort Algorithm
- Advantages Of Heap Sort
- Disadvantages Of Heap Sort
- Real-World Applications Of Heap Sort
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Counting Sort Algorithm
- Conditions For Using Counting Sort Algorithm
- How Counting Sort Algorithm Works?
- Implementation Of Counting Sort Algorithm
- Time And Space Complexity Analysis Of Counting Sort
- Comparison Of Counting Sort With Other Sorting Algorithms
- Advantages Of Counting Sort Algorithm
- Disadvantages Of Counting Sort Algorithm
- Applications Of Counting Sort Algorithm
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Shell Sort Algorithm
- Working Of Shell Sort Algorithm
- Implementation Of Shell Sort Algorithm
- Time Complexity Analysis Of Shell Sort Algorithm
- Advantages Of Shell Sort Algorithm
- Disadvantages Of Shell Sort Algorithm
- Applications Of Shell Sort Algorithm
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Binary Search Algorithm?
- Conditions For Using Binary Search
- Steps For Implementing Binary Search
- Iterative Method For Binary Search With Implementation Examples
- Recursive Method For Binary Search
- Complexity Analysis Of Binary Search Algorithm
- Iterative Vs. Recursive Implementation Of Binary Search
- Advantages & Disadvantages Of Binary Search
- Practical Applications & Real-World Examples Of Binary Search
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Linked List In Data Structures?
- Types Of Linked Lists In Data Structures
- Linked List Operations In Data Structures
- Advantages And Disadvantages Of Linked Lists In Data Structures
- Comparison Of Linked Lists And Arrays
- Applications Of Linked Lists
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Singly Linked List In Data Structure?
- Insertion Operations On Singly Linked Lists
- Deletion Operation On Singly Linked List
- Searching For Elements In Single Linked List
- Calculating Length Of Single Linked List
- Practical Applications Of Singly Linked Lists In Data Structure
- Common Problems With Singly Linked Lists
- Conclusion
- Frequently Asked Questions (FAQ)
Table of content:
- What Is A Linked List?
- Reverse A Linked List
- How To Reverse A Linked List? (Approaches)
- Recursive Approach To Reverse A Linked List
- Iterative Approach To Reverse A Linked List
- Using Stack To Reverse A Linked List
- Complexity Analysis Of Different Approaches To Reverse A Linked List
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Graph Data Structure?
- Importance Of Graph Data Structures
- Types Of Graphs In Data Structure
- Types Of Graph Algorithms
- Application Of Graphs In Data Structures
- Challenges And Complexities In Graphs
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Tree Data Structure?
- Terminologies Of Tree Data Structure:
- Different Types Of Tree Data Structures
- Basic Operations On Tree Data Structure
- Applications Of Tree Data Structures
- Comparison Of Trees, Graphs, And Linear Data Structures
- Advantages Of Tree Data Structure
- Disadvantages Of Tree Data Structure
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Dynamic Programming?
- Real-Life Example: The Jigsaw Puzzle Analogy
- How To Solve A Problem Using Dynamic Programming?
- Dynamic Programming Algorithm Techniques
- Advantages Of Dynamic Programming
- Disadvantages Of Dynamic Programming
- Applications Of Dynamic Programming
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Sliding Window Algorithm
- How Does The Sliding Window Algorithm Works?
- How To Identify Sliding Window Problems?
- Fixed-Size Sliding Window Example: Maximum Sum Subarray Of Size k
- Variable-Size Sliding Window Example: Smallest Subarray With A Given Sum
- Advantages Of Sliding Window Technique
- Disadvantages Of Sliding Window Technique
- Conclusion
- Frequently Asked Questions
Table of content:
- Introduction To Data Structures
- Data Structures Interview Questions: Basics
- Data Structures Interview Questions: Intermediate
- Data Structures Interview Questions: Advanced
- Conclusion
Learn All About Bubble Sort Algorithm (With Code Examples)
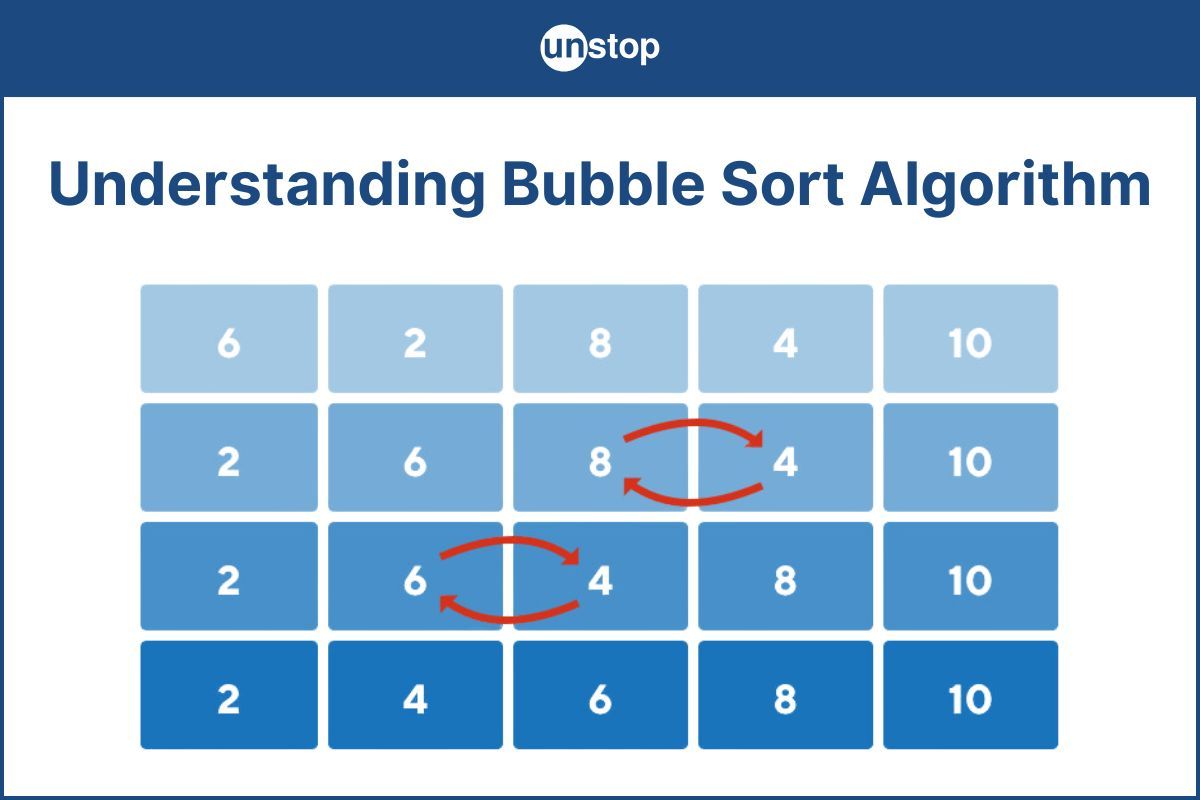
Sorting algorithms play a crucial role in organizing data efficiently, and Bubble Sort is one of the simplest techniques to achieve this. It repeatedly compares adjacent elements and swaps them if they are in the wrong order, making it a straightforward yet fundamental sorting method. While not the most efficient for large datasets, Bubble Sort is widely used for teaching purposes due to its easy-to-understand nature.
In this article, we will explore the working principle of Bubble Sort, analyze its time complexity, and discuss its advantages and limitations with an implementation in C++ and Python.
Understanding Bubble Sort Algorithm
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. This process continues until the list is completely sorted. It is called Bubble Sort because the larger elements "bubble up" to the end of the list with each pass.
How Bubble Sort Works?
- Start from the first element.
- Compare it with the next element.
- If the first element is greater than the second, swap them.
- Move to the next pair and repeat the process.
- The largest element moves to the last position in each pass.
- Repeat the process for the remaining elements until the whole list is sorted.
Real-Life Example: Arranging Books by Height
Imagine a librarian wants to arrange books on a shelf in increasing order of height.
- The librarian picks the first two books and compares their heights.
- If the first book is taller than the second, they swap them.
- Then, they move to the next pair and continue swapping until the tallest book reaches the end.
- They repeat the process for the remaining books until all are sorted.
This mimics how Bubble Sort moves larger numbers to the right while sorting the rest of the list.
Bubble Sort Algorithm
Bubble Sort is a simple comparison-based sorting algorithm that repeatedly swaps adjacent elements if they are in the wrong order.
Algorithm For Bubble Sort
- Start
- Repeat the following steps for (n-1) passes:
- Iterate from the first element to the second last element.
- Compare adjacent elements.
- Swap them if they are in the wrong order (i.e., if the left element is greater than the right one).
- Continue the process until the largest element reaches the correct position at the end.
- Continue the process for the remaining (n-1) elements.
- Stop when no more swaps are needed.
- End
Pseudocode For Bubble Sort
BubbleSort(arr, n)
for i = 0 to n-1
for j = 0 to n-i-2
if arr[j] > arr[j+1] then
swap(arr[j], arr[j+1])
end for
end for
return arr
Example:
Let's say we have an array:
arr[] = {5, 3, 8, 4, 2}
Pass 1 (Sorting the largest element to the last)
- Compare 5 & 3 → Swap → [3, 5, 8, 4, 2]
- Compare 5 & 8 → No Swap → [3, 5, 8, 4, 2]
- Compare 8 & 4 → Swap → [3, 5, 4, 8, 2]
- Compare 8 & 2 → Swap → [3, 5, 4, 2, 8]
(Largest element 8 is now at the correct position)
Pass 2
- Compare 3 & 5 → No Swap → [3, 5, 4, 2, 8]
- Compare 5 & 4 → Swap → [3, 4, 5, 2, 8]
- Compare 5 & 2 → Swap → [3, 4, 2, 5, 8]
Pass 3
- Compare 3 & 4 → No Swap → [3, 4, 2, 5, 8]
- Compare 4 & 2 → Swap → [3, 2, 4, 5, 8]
Pass 4
- Compare 3 & 2 → Swap → [2, 3, 4, 5, 8]
The array is now sorted!
Implementation Of Bubble Sort In C++
Here’s a C++ program that implements Bubble Sort and prints the sorted array.
Code Example:
#include <iostream>
using namespace std;
// Function to implement Bubble Sort
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
bool swapped = false; // Optimization: To check if any swap happens
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
swap(arr[j], arr[j + 1]);
swapped = true;
}
}
// If no elements were swapped, break early
if (!swapped) break;
}
}
// Function to print an array
void printArray(int arr[], int n) {
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
cout << endl;
}
// Driver code
int main() {
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr) / sizeof(arr[0]);
cout << "Original array: ";
printArray(arr, n);
bubbleSort(arr, n);
cout << "Sorted array: ";
printArray(arr, n);
return 0;
}
Output:
Original array: 64 34 25 12 22 11 90
Sorted array: 11 12 22 25 34 64 90
Explanation:
In the above code example-
- We start by including the <iostream> library to handle input and output operations.
- Now we use namespace std; to avoid prefixing standard library elements with std::.
- The bubbleSort() function implements the Bubble Sort algorithm to sort an array in ascending order.
- We use two nested loops:
- The outer loop iterates through the array, controlling the number of passes.
- The inner loop compares adjacent elements and swaps them if they are in the wrong order.
- We introduce a swapped flag to optimize the sorting process:
- If no swaps occur in a pass, it means the array is already sorted, so we break out of the loop early.
- The printArray() function iterates through the array and prints its elements.
- In main(), we define an integer array with some unsorted values.
- We calculate the number of elements in the array using sizeof(arr) / sizeof(arr[0]).
- We print the original array using the printArray() function.
- We call the bubbleSort() function to sort the array.
- We print the sorted array to verify the output.
- The program returns 0, indicating successful execution.
Time And Space Complexity Analysis Of Bubble Sort Algorithm
Bubble Sort is a simple sorting algorithm, but its efficiency depends on the arrangement of elements in the input array.
1. Time Complexity Analysis
Worst Case (O(n²)) - Reverse Sorted Order
- In the worst-case scenario, the array is sorted in descending order.
- Each element is compared with every other element.
- The total number of comparisons: (n−1)+(n−2)+...+2+1 = n(n−1)/2 ≈ O(n²)
- Example:
Input:{9, 8, 7, 6, 5}
Comparisons: Maximum required.
Best Case (O(n)) - Already Sorted Array
- If the array is already sorted, no swaps are needed.
- The algorithm makes one full pass to verify sorting.
- If an optimized Bubble Sort with a swapped flag is used, it exits early after one pass.
- The time complexity is: O(n)
- Example:
Input: {1, 2, 3, 4, 5}
Comparisons: n-1, and the loop breaks early.
Average Case (O(n²)) - Random Order
- In an average scenario, the array elements are randomly arranged.
- The total comparisons are still approximately: O(n²)
- Example:
Input: {5, 1, 4, 2, 8}
Comparisons: Intermediate number of swaps.
2. Space Complexity Analysis
Space Complexity: O(1)
- Bubble Sort is an in-place sorting algorithm, meaning:
- It does not use any extra space.
- Swaps occur within the array itself.
- The only extra space used is for temporary variables (i, j, and swapped flag).
Advantages Of Bubble Sort Algorithm
Some of the advantages are as follows:
- Simple and Easy to Implement → Straightforward logic, easy to code.
- In-Place Sorting (O(1) Space Complexity) → Does not require extra memory.
- Stable Sorting Algorithm → Preserves the order of equal elements.
- Best Case Performance O(n) (With Optimization) → Exits early if the array is already sorted.
Disadvantages Of Bubble Sort Algorithm
Some of the disadvantages are as follows:
- Slow for Large Data Sets (O(n²) Time Complexity) → Inefficient compared to other sorting algorithms.
- Too Many Swaps → Unnecessary swaps increase execution time.
- Not Used in Real-World Applications → Better alternatives exist (QuickSort, MergeSort).
- Inefficient for Nearly Sorted Arrays (Without Optimization) → Performs redundant passes if not optimized.
Applications of Bubble Sort Algorithms
While Bubble Sort is not the most efficient sorting algorithm for large datasets, it still has some practical applications in specific scenarios due to its simplicity and ease of understanding. Here are some of the key applications of Bubble Sort:
1. Educational Tool For Teaching Sorting Algorithms
Bubble Sort is widely used in introductory computer science courses to teach the basic concepts of sorting algorithms. Its simple logic helps students understand how comparison-based sorting works, with an emphasis on element swapping and iterative processing.
2. Small Datasets Or Nearly Sorted Data
For small datasets, the overhead of more complex algorithms may not justify their use, and Bubble Sort’s simplicity can be a good choice. If the data is nearly sorted (i.e., only a few swaps are needed), Bubble Sort can perform well and be more efficient than other algorithms due to its early stopping mechanism (when no swaps occur in a pass).
3. Sorting With Minimal Space Requirements
Bubble Sort is an in-place sorting algorithm, meaning it does not require extra memory (beyond a few variables for the loop). This makes it useful in situations where memory constraints are significant, and using additional memory for other sorting algorithms might not be feasible.
4. Stable Sorting Requirement
Bubble Sort is a stable sorting algorithm, which means that it preserves the relative order of equal elements in the dataset. This property can be valuable in cases where stability is required, such as when sorting objects based on multiple fields (e.g., sorting students by grade, but preserving their original order if they have the same grade).
5. External Sorting (In Limited Scenarios)
In very small-scale external sorting scenarios (like sorting data that cannot fit entirely in memory), Bubble Sort can sometimes be used where simplicity and low memory usage are more important than performance.
6. Simple Data Set Operations
When working with simple data structures like arrays or lists, especially in situations where the dataset is already mostly sorted or the number of elements is very small, Bubble Sort can be applied to achieve quick results without needing complex setups.
7. Comparing Algorithms In Benchmarking
Bubble Sort is often used in algorithmic benchmarking as a baseline comparison. Its poor performance against more sophisticated algorithms like Quick Sort or Merge Sort makes it an easy point of reference to demonstrate the importance of algorithm optimization.
Conclusion
Bubble Sort is a simple, comparison-based sorting algorithm that works by repeatedly swapping adjacent elements until the entire list is sorted. While it’s not the most efficient algorithm for large datasets due to its O(n²) time complexity, it serves as an excellent tool for educational purposes, helping beginners understand the fundamental concepts of sorting.
We also explored how the algorithm works, its time complexity analysis, and the possibility of optimization through early stopping. Though it is rarely used in production environments due to its inefficiency, Bubble Sort can be useful for small datasets, nearly sorted data, or situations with minimal memory requirements.
Ultimately, while more efficient sorting algorithms like Quick Sort and Merge Sort should be preferred for large-scale data, Bubble Sort remains a valuable learning tool and can still serve practical purposes in specific, simple applications.
Frequently Asked Questions
Q. What is Bubble Sort?
Bubble Sort is a simple comparison-based sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. This process is repeated until the list is sorted.
Q. How does Bubble Sort work?
In each pass through the list, Bubble Sort compares adjacent elements and swaps them if needed. The largest unsorted element "bubbles" up to its correct position at the end of the list. This process continues for each element until the entire list is sorted.
Q. What is the time complexity of Bubble Sort?
The time complexity of Bubble Sort is O(n²) in the worst and average cases, where n is the number of elements in the array. In the best case (when the array is already sorted), the time complexity can be O(n) if an optimized version with early stopping is used.
Q. What are the advantages of using Bubble Sort?
- Simple to implement and understand.
- Stable sorting algorithm, preserving the relative order of equal elements.
- Efficient for small datasets or nearly sorted data.
- In-place sorting: doesn’t require additional memory.
Q. What are the disadvantages of Bubble Sort?
- Inefficient for large datasets due to its O(n²) time complexity.
- Compared to more advanced sorting algorithms (like Quick Sort or Merge Sort), it is significantly slower for large or unsorted data.
Q. When should Bubble Sort be used?
Bubble Sort should be used when:
- The dataset is small or nearly sorted.
- Memory usage is a concern, as it is an in-place algorithm.
- Teaching or learning basic sorting techniques in educational environments.
Suggested Reads:
- Difference Between Hashing And Encryption Decoded
- 53 Frequently Asked Linked List Interview Questions With Answers 2024
- Data Structure Interview Questions For 2024 [With Detailed Answers]
- Tree Topology | Advantages & Disadvantages In Computer Network
- Decoding Data Redundancy In DBMS| Causes, Advantages, Solutions
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Kartik Deshmukh 2 days ago
Titiksha Bansal 5 days ago
Siddhi Raney 1 week ago
Archana Ba Parmar 1 week ago
Paritosh Sharma 1 week ago
Oshanki Priya 2 weeks ago