- What Is Data Structure?
- Key Features Of Data Structures
- Basic Terminologies Related To Data Structures
- Types Of Data Structures
- What Are Linear Data Structures & Its Types?
- What Are Non-Linear Data Structures & Its Types?
- Importance Of Data Structure In Programming
- Basic Operations On Data Structures
- Applications Of Data Structures
- Real-Life Applications Of Data Structures
- Linear Vs. Non-linear Data Structures
- What Are Algorithms? The Difference Between Data Structures & Algorithms
- Conclusion
- Frequently Asked Questions
- What Is Asymptotic Notation?
- How Asymptotic Notation Helps In Analyzing Performance
- Types Of Asymptotic Notation
- Big-O Notation (O)
- Omega Notation (Ω)
- Theta Notation (Θ)
- Little-O Notation (o)
- Little-Omega Notation (ω)
- Summary Of Asymptotic Notations
- Real-World Applications Of Asymptotic Notation
- Conclusion
- Frequently Asked Questions
- Understanding Big O Notation
- Types Of Time Complexity
- Space Complexity In Big O Notation
- How To Determine Big O Complexity
- Best, Worst, And Average Case Complexity
- Applications Of Big O Notation
- Conclusion
- Frequently Asked Questions
- What Is Time Complexity?
- Why Do You Need To Calculate Time Complexity?
- The Time Complexity Algorithm Cases
- Time Complexity: Different Types Of Asymptotic Notations
- How To Calculate Time Complexity?
- Time Complexity Of Sorting Algorithms
- Time Complexity Of Searching Algorithms
- Writing And optimizing An algorithm
- What Is Space Complexity And Its Significance?
- Relation Between Time And Space Complexity
- Conclusion
- What Is Linear Data Structure?
- Key Characteristics Of Linear Data Structures
- What Are The Types Of Linear Data Structures?
- What Is An Array Linear Data Structure?
- What Are Linked Lists Linear Data Structure?
- What Is A Stack Linear Data Structure?
- What Is A Queue Linear Data Structure?
- Most Common Operations Performed in Linear Data Structures
- Advantages Of Linear Data Structures
- What Is Nonlinear Data Structure?
- Difference Between Linear & Non-Linear Data Structures
- Who Uses Linear Data Structures?
- Conclusion
- Frequently Asked Questions
- What is a linear data structure?
- What is a non-linear data structure?
- Difference between linear data structure and non-linear data structure
- FAQs about linear and non-linear data structures
- What Is Search?
- What Is Linear Search In Data Structure?
- What Is Linear Search Algorithm?
- Working Of Linear Search Algorithm
- Complexity Of Linear Search Algorithm In Data Structures
- Implementations Of Linear Search Algorithm In Different Programming Languages
- Real-World Applications Of Linear Search In Data Structure
- Advantages & Disadvantages Of Linear Search
- Best Practices For Using Linear Search Algorithm
- Conclusion
- Frequently Asked Questions
- What Is The Binary Search Algorithm?
- Conditions For Using Binary Search
- Steps For Implementing Binary Search
- Iterative Method For Binary Search With Implementation Examples
- Recursive Method For Binary Search
- Complexity Analysis Of Binary Search Algorithm
- Iterative Vs. Recursive Implementation Of Binary Search
- Advantages & Disadvantages Of Binary Search
- Practical Applications & Real-World Examples Of Binary Search
- Conclusion
- Frequently Asked Questions
- Understanding The Jump Search Algorithm
- How Jump Search Works?
- Code Implementation Of Jump Search Algorithm
- Time And Space Complexity Analysis
- Advantages Of Jump Search
- Disadvantages Of Jump Search
- Applications Of Jump Search
- Conclusion
- Frequently Asked Questions
- What Is Sorting In Data Structures?
- What Is Bubble Sort?
- What Is Selection Sort?
- What Is Insertion Sort?
- What Is Merge Sort?
- What Is Quick Sort?
- What Is Heap Sort?
- What Is Radix Sort?
- What Is Bucket Sort?
- What Is Counting Sort?
- What Is Shell Sort?
- Choosing The Right Sorting Algorithm
- Applications Of Sorting
- Conclusion
- Frequently Asked Questions
- Understanding Bubble Sort Algorithm
- Bubble Sort Algorithm
- Implementation Of Bubble Sort In C++
- Time And Space Complexity Analysis Of Bubble Sort Algorithm
- Advantages Of Bubble Sort Algorithm
- Disadvantages Of Bubble Sort Algorithm
- Applications of Bubble Sort Algorithms
- Conclusion
- Frequently Asked Questions
- Understanding The Merge Sort Algorithm
- Algorithm For Merge Sort
- Implementation Of Merge Sort In C++
- Time And Space Complexity Analysis Of Merge Sort
- Advantages And Disadvantages Of Merge Sort
- Applications Of Merge Sort
- Conclusion
- Frequently Asked Questions
- Understanding The Selection Sort Algorithm
- Algorithmic Approach To Selection Sort
- Implementation Of Selection Sort Algorithm
- Complexity Analysis Of Selection Sort
- Comparison Of Selection Sort With Other Sorting Algorithms
- Advantages And Disadvantages Of Selection Sort
- Application Of Selection Sort
- Conclusion
- Frequently Asked Questions
- What Is Insertion Sort Algorithm?
- How Does Insertion Sort Work? (Step-by-Step Explanation)
- Implementation of Insertion Sort in C++
- Time And Space Complexity Of Insertion Sort
- Applications Of Insertion Sort Algorithm
- Comparison With Other Sorting Algorithms
- Conclusion
- Frequently Asked Questions
- Understanding Quick Sort Algorithm
- Step-By-Step Working Of Quick Sort
- Implementation Of Quick Sort Algorithm
- Time Complexity Analysis Of Quick Sort
- Advantages Of Quick Sort Algorithm
- Disadvantages Of Quick Sort Algorithm
- Applications Of Quick Sort Algorithm
- Conclusion
- Frequently Asked Questions
- Understanding The Heap Data Structure
- Working Of Heap Sort Algorithm
- Implementation Of Heap Sort Algorithm
- Advantages Of Heap Sort
- Disadvantages Of Heap Sort
- Real-World Applications Of Heap Sort
- Conclusion
- Frequently Asked Questions
- Understanding The Counting Sort Algorithm
- Conditions For Using Counting Sort Algorithm
- How Counting Sort Algorithm Works?
- Implementation Of Counting Sort Algorithm
- Time And Space Complexity Analysis Of Counting Sort
- Comparison Of Counting Sort With Other Sorting Algorithms
- Advantages Of Counting Sort Algorithm
- Disadvantages Of Counting Sort Algorithm
- Applications Of Counting Sort Algorithm
- Conclusion
- Frequently Asked Questions
- Understanding Shell Sort Algorithm
- Working Of Shell Sort Algorithm
- Implementation Of Shell Sort Algorithm
- Time Complexity Analysis Of Shell Sort Algorithm
- Advantages Of Shell Sort Algorithm
- Disadvantages Of Shell Sort Algorithm
- Applications Of Shell Sort Algorithm
- Conclusion
- Frequently Asked Questions
- What Is Linked List In Data Structures?
- Types Of Linked Lists In Data Structures
- Linked List Operations In Data Structures
- Advantages And Disadvantages Of Linked Lists In Data Structures
- Comparison Of Linked Lists And Arrays
- Applications Of Linked Lists
- Conclusion
- Frequently Asked Questions
- What Is A Singly Linked List In Data Structure?
- Insertion Operations On Singly Linked Lists
- Deletion Operation On Singly Linked List
- Searching For Elements In Single Linked List
- Calculating Length Of Single Linked List
- Practical Applications Of Singly Linked Lists In Data Structure
- Common Problems With Singly Linked Lists
- Conclusion
- Frequently Asked Questions (FAQ)
- What Is A Linked List?
- Reverse A Linked List
- How To Reverse A Linked List? (Approaches)
- Recursive Approach To Reverse A Linked List
- Iterative Approach To Reverse A Linked List
- Using Stack To Reverse A Linked List
- Complexity Analysis Of Different Approaches To Reverse A Linked List
- Conclusion
- Frequently Asked Questions
- What Is A Stack In Data Structure?
- Understanding Stack Operations
- Stack Implementation In Data Structures
- Stack Implementation Using Arrays
- Stack Implementation Using Linked Lists
- Comparison: Array vs. Linked List Implementation
- Applications Of Stack In Data Structures
- Advantages And Disadvantages Of Stack Data Structure
- Conclusion
- Frequently Asked Questions
- What Is A Graph Data Structure?
- Importance Of Graph Data Structures
- Types Of Graphs In Data Structure
- Types Of Graph Algorithms
- Application Of Graphs In Data Structures
- Challenges And Complexities In Graphs
- Conclusion
- Frequently Asked Questions
- What Is Tree Data Structure?
- Terminologies Of Tree Data Structure:
- Different Types Of Tree Data Structures
- Basic Operations On Tree Data Structure
- Applications Of Tree Data Structures
- Comparison Of Trees, Graphs, And Linear Data Structures
- Advantages Of Tree Data Structure
- Disadvantages Of Tree Data Structure
- Conclusion
- Frequently Asked Questions
- What Is Dynamic Programming?
- Real-Life Example: The Jigsaw Puzzle Analogy
- How To Solve A Problem Using Dynamic Programming?
- Dynamic Programming Algorithm Techniques
- Advantages Of Dynamic Programming
- Disadvantages Of Dynamic Programming
- Applications Of Dynamic Programming
- Conclusion
- Frequently Asked Questions
- Understanding The Sliding Window Algorithm
- How Does The Sliding Window Algorithm Works?
- How To Identify Sliding Window Problems?
- Fixed-Size Sliding Window Example: Maximum Sum Subarray Of Size k
- Variable-Size Sliding Window Example: Smallest Subarray With A Given Sum
- Advantages Of Sliding Window Technique
- Disadvantages Of Sliding Window Technique
- Conclusion
- Frequently Asked Questions
- Introduction To Data Structures
- Data Structures Interview Questions: Basics
- Data Structures Interview Questions: Intermediate
- Data Structures Interview Questions: Advanced
- Conclusion
Insertion Sort Algorithm - Working Explained (+Code Examples)
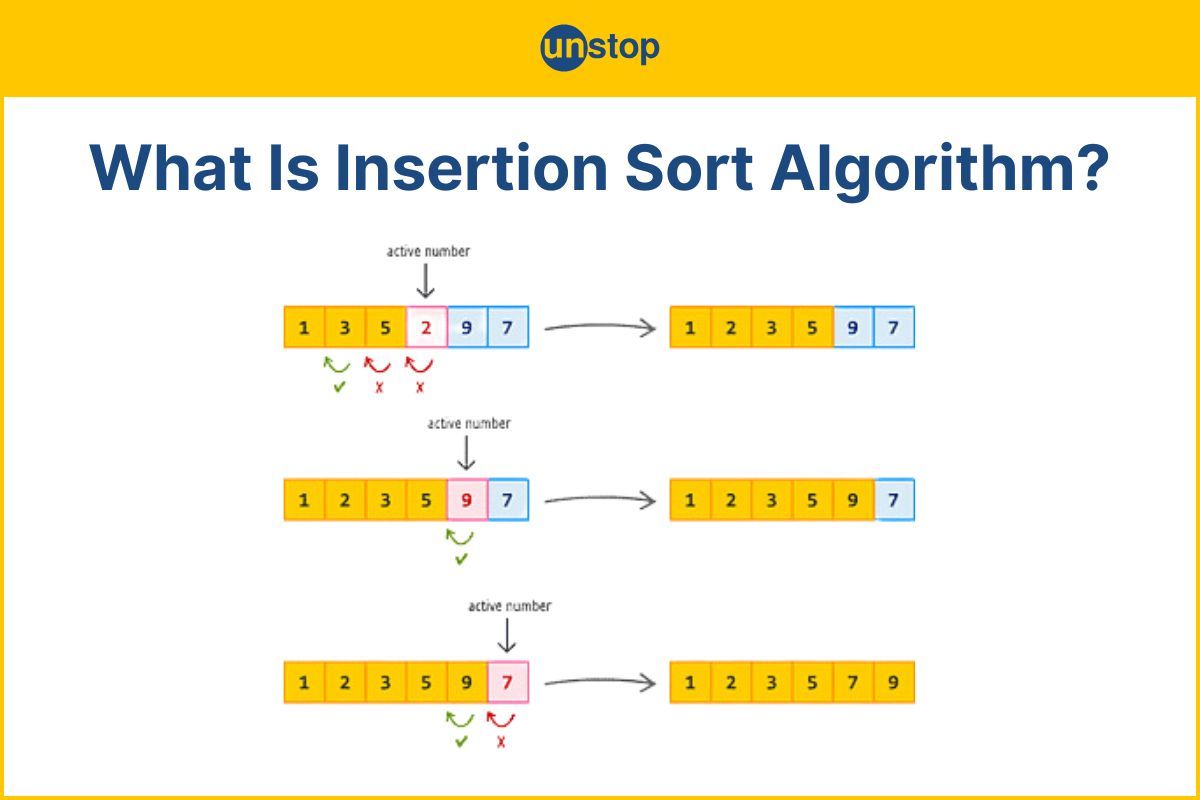
Sorting algorithms play a crucial role in computer science, enabling efficient organization and retrieval of data. One such fundamental algorithm is Insertion Sort, which works similarly to how we arrange playing cards in our hands. It builds the sorted list one element at a time by comparing and placing each new element in its correct position.
In this article, we will explore the Insertion Sort algorithm, its working mechanism, time complexity, and implementation in different programming languages. Whether you're a beginner or an experienced programmer, understanding this simple yet effective sorting technique will enhance your grasp of algorithmic problem-solving.
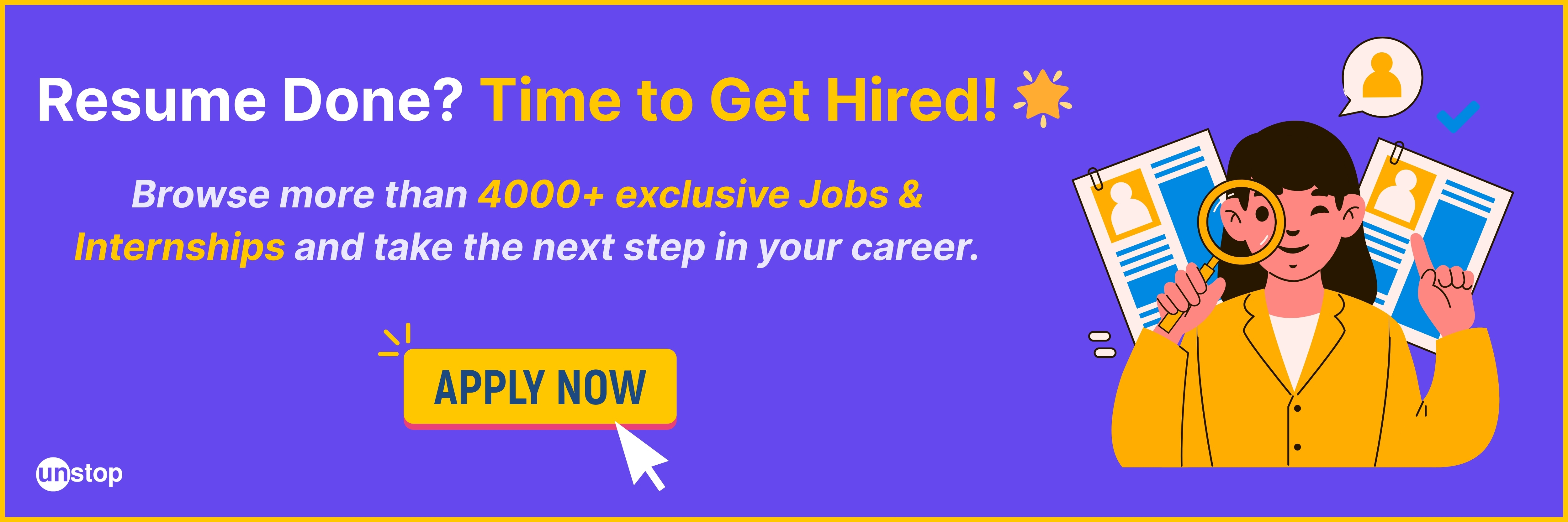
What Is Insertion Sort Algorithm?
Insertion Sort is a simple and efficient sorting algorithm that works similarly to how we sort playing cards in our hands. It builds the sorted array one element at a time by taking each element and placing it in its correct position.
Working Principle Of Insertion Sort
Insertion Sort works as follows:
- Assume the first element is already sorted.
- Pick the next element and compare it with the elements in the sorted section (left side).
- Shift the larger elements one position to the right to make space for the new element.
- Insert the picked element at its correct position.
- Repeat steps 2-4 for all elements in the array.
This process continues until the entire array is sorted.
Real-Life Analogy
Imagine you are sorting a deck of playing cards in your hand:
- You pick a card and assume it’s in its correct place.
- You take the next card and insert it into the right position by shifting the other cards if necessary.
- You continue this process, placing each new card in the correct order relative to the ones already sorted.
This is exactly how Insertion Sort works—placing each element in the right position one at a time!
How Does Insertion Sort Work? (Step-by-Step Explanation)
Let's take an example to understand the step-by-step working of Insertion Sort algorithm in data structures.
Example: Sort the array [6, 3, 8, 5, 2] using Insertion Sort.
Step 1: Consider the First Element as Sorted
- The first element 6 is already considered sorted.
- Array state: [6, 3, 8, 5, 2]
Step 2: Pick the Next Element (3) and Insert It in the Sorted Part
- Compare 3 with 6 → Since 3 < 6, shift 6 to the right.
- Insert 3 in the correct position.
- Array state: [3, 6, 8, 5, 2]
Step 3: Pick the Next Element (8) and Insert It in the Sorted Part
- Compare 8 with 6 → 8 > 6, so no shifting is required.
- Array state remains: [3, 6, 8, 5, 2]
Step 4: Pick the Next Element (5) and Insert It in the Sorted Part
- Compare 5 with 8 → Shift 8 to the right.
- Compare 5 with 6 → Shift 6 to the right.
- Insert 5 in the correct position.
- Array state: [3, 5, 6, 8, 2]
Step 5: Pick the Next Element (2) and Insert It in the Sorted Part
- Compare 2 with 8 → Shift 8 to the right.
- Compare 2 with 6 → Shift 6 to the right.
- Compare 2 with 5 → Shift 5 to the right.
- Compare 2 with 3 → Shift 3 to the right.
- Insert 2 in the correct position.
- Array state: [2, 3, 5, 6, 8]
Final Sorted Array: [2, 3, 5, 6, 8]
Pseudocode For Insertion Sort
Here’s a simple pseudocode to understand the logic of Insertion Sort:
InsertionSort(arr, n):
for i from 1 to n-1:
key = arr[i]
j = i - 1
while j >= 0 and arr[j] > key:
arr[j + 1] = arr[j] // Shift elements to the right
j = j - 1
arr[j + 1] = key // Insert the key in the correct position
Implementation of Insertion Sort in C++
Let’s now look at a simple C++ program to implement Insertion Sort-
Code Example:
#include <iostream>
using namespace std;
// Function to perform Insertion Sort
void insertionSort(int arr[], int n) {
for (int i = 1; i < n; i++) {
int key = arr[i]; // Pick the current element
int j = i - 1;
// Shift elements that are greater than key
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j = j - 1;
}
// Insert the key in the correct position
arr[j + 1] = key;
}
}
// Function to print the array
void printArray(int arr[], int n) {
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
cout << endl;
}
// Main function
int main() {
int arr[] = {6, 3, 8, 5, 2};
int n = sizeof(arr) / sizeof(arr[0]);
cout << "Original array: ";
printArray(arr, n);
insertionSort(arr, n);
cout << "Sorted array: ";
printArray(arr, n);
return 0;
}
Output:
Original array: 6 3 8 5 2
Sorted array: 2 3 5 6 8
Explanation:
In the above code example-
- We start by including the <iostream> header, which allows us to use input and output operations.
- The using namespace std; directive enables us to use standard library elements without prefixing them with std::.
- We define the insertionSort() function, which takes an array and its size as arguments.
- The sorting process begins with the second element (index 1) since a single-element array is already sorted.
- For each element, we store its value in key and compare it with the elements before it.
- If an element is greater than key, we shift it one position to the right to make space for insertion.
- Once we find the correct position, we insert the key value.
- The process repeats for all elements, gradually building a sorted section of the array.
- The printArray() function helps us display the array elements, separated by spaces.
- In main(), we define an integer array {6, 3, 8, 5, 2} and determine its size using sizeof(arr) / sizeof(arr[0]).
- We print the original array before sorting.
- The insertionSort() function is called to sort the array.
- After sorting, we print the updated array to show the sorted order.
- The program returns 0, indicating successful execution.
Time And Space Complexity Of Insertion Sort
Let’s now have a look at these aspects of the insertion sort algorithm:
1. Time Complexity
Case |
Complexity |
Explanation |
Best Case |
O(n) |
The array is already sorted. Only one comparison per element, no shifting. |
Worst Case |
O(n²) |
The array is in reverse order. Each element needs to be compared and shifted. |
Average Case |
O(n²) |
On average, elements are randomly placed, leading to about n²/4 shifts. |
2. Space Complexity
- Space Complexity: O(1) (In-place sorting, no extra memory used except a few variables).
- Stable Sort? Yes (Preserves the relative order of equal elements).
Advantages Of Insertion Sort Algorithm
Some common advantages of insertion sort are:
- Simple and Easy to Implement: The algorithm is straightforward and easy to code.
- Efficient for Small or Nearly Sorted Data: Performs well when the dataset is already partially sorted.
- Stable Sorting Algorithm: Maintains the relative order of equal elements.
- In-Place Sorting: Requires only O(1) extra space (no additional arrays needed).
- Adaptive Nature: Runs in O(n) time if the array is already sorted, making it faster than other O(n²) algorithms in such cases.
Disadvantages Of Insertion Sort Algorithm
Some common disadvantages of insertion sort are:
- Inefficient for Large Datasets: Has a worst-case time complexity of O(n²), making it slow for large inputs.
- Not Suitable for Highly Unsorted Data: Requires many comparisons and shifts in the worst case (reverse-sorted data).
- Slower Compared to Advanced Sorting Algorithms: Merge Sort (O(n log n)) and Quick Sort (O(n log n)) perform better for large datasets.
Applications Of Insertion Sort Algorithm
Some of the most common applications of insertion sort are as follows:
- Small Data Sets: Insertion Sort performs well on small datasets due to its simple implementation and low overhead. For small lists, its simplicity makes it faster than more complex algorithms like Merge Sort or Quick Sort.
- Nearly Sorted Data: If the input list is already partially sorted or nearly sorted, Insertion Sort performs very efficiently. In such cases, it can be more efficient than algorithms like Merge Sort, which may still take time for splitting and merging.
- Online Sorting: Insertion Sort is useful in applications where data is received in a continuous stream, and elements need to be inserted in sorted order as they arrive. This is often referred to as online sorting, and Insertion Sort handles such cases very well because it can insert new elements into an already sorted list incrementally.
- Adaptive Sorting: In scenarios where data is frequently updated (e.g., inserting new records into a sorted list), Insertion Sort can adapt well by adding new elements in their correct positions without re-sorting the entire list.
- Low Memory Usage: Since Insertion Sort works in-place and requires no extra memory other than a few variables for tracking elements, it is well-suited for environments where memory is limited, such as embedded systems or devices with restricted resources.
- Educational and Demonstration Purposes: Insertion Sort is often used in educational settings to teach algorithm design and sorting techniques due to its simplicity and intuitive approach. It helps beginners understand how algorithms work by focusing on fundamental concepts like comparisons and element swapping.
- Data Stream Processing: Insertion Sort can be applied in situations where we need to sort incoming data (e.g., streaming data from sensors or real-time systems) incrementally as each data point arrives, especially when it’s necessary to maintain an always-sorted list.
- Implementing Other Algorithms: Insertion Sort can be used as a part of more complex algorithms, such as Shell Sort, where the initial sorting steps are performed with a smaller gap and then refined using Insertion Sort for the final ordering.
Comparison With Other Sorting Algorithms
Here's a comparison between Insertion Sort and other popular sorting algorithms like Bubble Sort, Selection Sort, and Merge Sort in terms of efficiency, complexity, and use cases.
1. Insertion Sort Vs Bubble Sort
Aspect |
Insertion Sort |
Bubble Sort |
Working Principle |
Builds a sorted array one element at a time by inserting each element in its correct position. |
Repeatedly swaps adjacent elements if they are in the wrong order, "bubbling" the largest element to the end. |
Best Case Complexity |
O(n) (when the list is already sorted or nearly sorted) |
O(n) (when the list is already sorted) |
Worst Case Complexity |
O(n²) (when the list is reversed) |
O(n²) (same as Insertion Sort in worst case) |
Average Case Complexity |
O(n²) |
O(n²) |
Space Complexity |
O(1) (in-place sorting) |
O(1) (in-place sorting) |
Stability |
Stable (does not change the relative order of equal elements) |
Stable |
Use Cases |
Small datasets, partially sorted data, online sorting |
Small datasets, educational purposes, small-scale sorting |
Key Difference: Insertion Sort is generally faster than Bubble Sort due to fewer comparisons and fewer swaps. Insertion Sort often outperforms Bubble Sort, especially with partially sorted data.
2. Insertion Sort Vs Selection Sort
Aspect |
Insertion Sort |
Selection Sort |
Working Principle |
Builds a sorted array one element at a time by inserting each element in its correct position. |
Finds the smallest (or largest) element in the unsorted part of the array and swaps it with the first unsorted element. |
Best Case Complexity |
O(n) (when the list is already sorted or nearly sorted) |
O(n²) (doesn't improve in the best case) |
Worst Case Complexity |
O(n²) (when the list is reversed) |
O(n²) (same as Insertion Sort in worst case) |
Average Case Complexity |
O(n²) |
O(n²) |
Space Complexity |
O(1) (in-place sorting) |
O(1) (in-place sorting) |
Stability |
Stable (does not change the relative order of equal elements) |
Not stable (can change the relative order of equal elements) |
Use Cases |
Small datasets, partially sorted data, online sorting |
Simple to implement, small datasets, educational purposes |
Key Difference: Insertion Sort is generally more efficient than Selection Sort in terms of the number of swaps required. Selection Sort makes a fixed number of swaps (n-1 swaps for n elements), while Insertion Sort makes fewer swaps when the data is nearly sorted.
3. Insertion Sort Vs Merge Sort
Aspect |
Insertion Sort |
Merge Sort |
Working Principle |
Builds a sorted array one element at a time by inserting each element in its correct position. |
Divides the array into halves, recursively sorts them, and merges them to form the sorted array. |
Best Case Complexity |
O(n) (when the list is already sorted or nearly sorted) |
O(n log n) (always, as it splits and merges the array) |
Worst Case Complexity |
O(n²) (when the list is reversed) |
O(n log n) (same for all cases) |
Average Case Complexity |
O(n²) |
O(n log n) |
Space Complexity |
O(1) (in-place sorting) |
O(n) (requires additional space for merging) |
Stability |
Stable (does not change the relative order of equal elements) |
Stable (maintains the order of equal elements) |
Use Cases |
Small datasets, partially sorted data, online sorting |
Large datasets, external sorting, divide-and-conquer applications |
Key Difference: Merge Sort is much more efficient than Insertion Sort for large datasets due to its O(n log n) time complexity. However, Insertion Sort has the advantage of being in-place (no extra memory needed), while Merge Sort requires additional memory for merging.
Conclusion
Insertion Sort is a simple and intuitive sorting algorithm that works well for small datasets or when the data is already nearly sorted. Its in-place and stable nature makes it suitable for specific applications where space is limited or maintaining the relative order of equal elements is important. However, due to its O(n²) time complexity, it is not the most efficient choice for large datasets compared to more advanced algorithms like Quick Sort or Merge Sort.
Despite its limitations, Insertion Sort’s adaptive nature, ease of implementation, and real-world usability in certain scenarios ensure its relevance, especially in scenarios involving dynamic data or small collections. By understanding its strengths and weaknesses, we can apply Insertion Sort effectively in situations where it shines, offering a balance of simplicity and efficiency.
Frequently Asked Questions
Q. How does the Insertion Sort algorithm work?
The Insertion Sort algorithm sorts an array by repeatedly picking the next element and inserting it into its correct position in the already sorted portion of the array. It begins with the second element and compares it to the elements before it, shifting elements as needed to make space for the new element. This process is repeated for each element until the entire array is sorted.
Step-by-Step Process:
- Start with the second element (since the first element is trivially sorted).
- Compare the current element with the one before it.
- If the current element is smaller, shift the previous element to the right.
- Continue shifting elements until the current element is greater than or equal to the shifted element, then place it in its correct position.
- Move to the next element and repeat the process.
The sorting is done in-place, meaning no additional memory is required apart from a few variables for tracking elements.
Q. What is the time complexity of Insertion Sort?
The time complexity of Insertion Sort depends on the state of the input data.
- Best Case (O(n)): When the array is already sorted or nearly sorted, the algorithm only performs a linear scan to insert each element. No shifting occurs in this case, making it a highly efficient operation.
- Worst Case (O(n²)): When the array is sorted in reverse order, each element must be compared and shifted past every other element, resulting in quadratic time complexity.
- Average Case (O(n²)): Typically, the algorithm performs a number of comparisons and shifts proportional to n² for a randomly ordered list.
Although the worst and average case time complexities are O(n²), Insertion Sort’s best-case performance makes it a good choice for small or nearly sorted datasets.
Q. Is Insertion Sort stable?
Yes, Insertion Sort is a stable sorting algorithm. A stable sorting algorithm preserves the relative order of elements with equal values. For instance, if two elements have the same value, Insertion Sort ensures that their relative order remains unchanged after sorting. This property is important in scenarios where the relative order of identical elements carries significance.
For example, consider an array of tuples where each tuple contains a number and a string:
[(1, 'A'), (1, 'B'), (2, 'C')]. After sorting, the relative order of (1, 'A') and (1, 'B') remains intact because of the stability of Insertion Sort.
Q. How does Insertion Sort compare to other sorting algorithms in terms of memory usage?
One of the key advantages of Insertion Sort is its in-place sorting capability, meaning it requires O(1) extra space. Unlike algorithms such as Merge Sort, which require additional space for merging subarrays, Insertion Sort only requires a constant amount of extra memory for temporary variables during element comparisons and shifts.
In scenarios where memory is constrained (e.g., embedded systems or small devices), Insertion Sort is a preferable choice because of its minimal memory overhead.
Q. When is Insertion Sort the best sorting algorithm to use?
Insertion Sort is ideal in the following scenarios:
- Small Datasets: For small arrays or lists, Insertion Sort is efficient due to its low overhead and simplicity. In fact, for very small datasets, it may outperform more complex algorithms like Merge Sort or Quick Sort.
- Nearly Sorted Data: When the data is already partially sorted, Insertion Sort can quickly complete the sorting process in linear time (O(n)) with minimal shifts and comparisons.
- Real-Time Applications (Online Sorting): Insertion Sort is used in applications where new data is continuously received and needs to be inserted into an already sorted list, such as in live data streams or online sorting problems.
In these cases, Insertion Sort offers an efficient solution with less memory usage and faster sorting times for smaller or incrementally updated data.
Q. Can Insertion Sort be improved or optimized?
While the basic Insertion Sort algorithm is relatively simple and effective, there are a few optimizations that can be applied to improve its efficiency:
- Binary Insertion Sort: Instead of performing a linear search to find the correct position of an element, a binary search can be used to locate the appropriate insertion point more quickly (in O(log n) time). This reduces the number of comparisons but does not affect the shifting of elements, so the overall time complexity remains O(n²).
- Hybrid Algorithms: In practice, Insertion Sort is often combined with other sorting algorithms in hybrid approaches. For example, Timsort (used in Python’s sorting algorithm) and IntroSort (used in C++’s STL sort) use Insertion Sort for smaller subarrays during recursive sorting because of its efficiency with small datasets.
While these optimizations can make Insertion Sort more efficient in certain situations, the fundamental time complexity remains O(n²) for the worst and average cases.
Suggested Reads:
- Learn About Asymptotic Notations (+ Graphs & Real-Life Examples)
- Difference Between Hashing And Encryption Decoded
- 53 Frequently Asked Linked List Interview Questions With Answers 2024
- Data Structure Interview Questions For 2024 [With Detailed Answers]
- Tree Topology | Advantages & Disadvantages In Computer Network
- Decoding Data Redundancy In DBMS| Causes, Advantages, Solutions
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Kartik Deshmukh 1 month ago
SHABARI VIGNESH U 1 month ago
Divyansh Shrivastava 1 month ago
Archana Ba Parmar 1 month ago
Mihir Kumar Ranjan 1 month ago