- What Is Data Structure?
- Key Features Of Data Structures
- Basic Terminologies Related To Data Structures
- Types Of Data Structures
- What Are Linear Data Structures & Its Types?
- What Are Non-Linear Data Structures & Its Types?
- Importance Of Data Structure In Programming
- Basic Operations On Data Structures
- Applications Of Data Structures
- Real-Life Applications Of Data Structures
- Linear Vs. Non-linear Data Structures
- What Are Algorithms? The Difference Between Data Structures & Algorithms
- Conclusion
- Frequently Asked Questions
- What Is Asymptotic Notation?
- How Asymptotic Notation Helps In Analyzing Performance
- Types Of Asymptotic Notation
- Big-O Notation (O)
- Omega Notation (Ω)
- Theta Notation (Θ)
- Little-O Notation (o)
- Little-Omega Notation (ω)
- Summary Of Asymptotic Notations
- Real-World Applications Of Asymptotic Notation
- Conclusion
- Frequently Asked Questions
- Understanding Big O Notation
- Types Of Time Complexity
- Space Complexity In Big O Notation
- How To Determine Big O Complexity
- Best, Worst, And Average Case Complexity
- Applications Of Big O Notation
- Conclusion
- Frequently Asked Questions
- What Is Time Complexity?
- Why Do You Need To Calculate Time Complexity?
- The Time Complexity Algorithm Cases
- Time Complexity: Different Types Of Asymptotic Notations
- How To Calculate Time Complexity?
- Time Complexity Of Sorting Algorithms
- Time Complexity Of Searching Algorithms
- Writing And optimizing An algorithm
- What Is Space Complexity And Its Significance?
- Relation Between Time And Space Complexity
- Conclusion
- What Is Linear Data Structure?
- Key Characteristics Of Linear Data Structures
- What Are The Types Of Linear Data Structures?
- What Is An Array Linear Data Structure?
- What Are Linked Lists Linear Data Structure?
- What Is A Stack Linear Data Structure?
- What Is A Queue Linear Data Structure?
- Most Common Operations Performed in Linear Data Structures
- Advantages Of Linear Data Structures
- What Is Nonlinear Data Structure?
- Difference Between Linear & Non-Linear Data Structures
- Who Uses Linear Data Structures?
- Conclusion
- Frequently Asked Questions
- What is a linear data structure?
- What is a non-linear data structure?
- Difference between linear data structure and non-linear data structure
- FAQs about linear and non-linear data structures
- What Is Search?
- What Is Linear Search In Data Structure?
- What Is Linear Search Algorithm?
- Working Of Linear Search Algorithm
- Complexity Of Linear Search Algorithm In Data Structures
- Implementations Of Linear Search Algorithm In Different Programming Languages
- Real-World Applications Of Linear Search In Data Structure
- Advantages & Disadvantages Of Linear Search
- Best Practices For Using Linear Search Algorithm
- Conclusion
- Frequently Asked Questions
- What Is The Binary Search Algorithm?
- Conditions For Using Binary Search
- Steps For Implementing Binary Search
- Iterative Method For Binary Search With Implementation Examples
- Recursive Method For Binary Search
- Complexity Analysis Of Binary Search Algorithm
- Iterative Vs. Recursive Implementation Of Binary Search
- Advantages & Disadvantages Of Binary Search
- Practical Applications & Real-World Examples Of Binary Search
- Conclusion
- Frequently Asked Questions
- Understanding The Jump Search Algorithm
- How Jump Search Works?
- Code Implementation Of Jump Search Algorithm
- Time And Space Complexity Analysis
- Advantages Of Jump Search
- Disadvantages Of Jump Search
- Applications Of Jump Search
- Conclusion
- Frequently Asked Questions
- What Is Sorting In Data Structures?
- What Is Bubble Sort?
- What Is Selection Sort?
- What Is Insertion Sort?
- What Is Merge Sort?
- What Is Quick Sort?
- What Is Heap Sort?
- What Is Radix Sort?
- What Is Bucket Sort?
- What Is Counting Sort?
- What Is Shell Sort?
- Choosing The Right Sorting Algorithm
- Applications Of Sorting
- Conclusion
- Frequently Asked Questions
- Understanding Bubble Sort Algorithm
- Bubble Sort Algorithm
- Implementation Of Bubble Sort In C++
- Time And Space Complexity Analysis Of Bubble Sort Algorithm
- Advantages Of Bubble Sort Algorithm
- Disadvantages Of Bubble Sort Algorithm
- Applications of Bubble Sort Algorithms
- Conclusion
- Frequently Asked Questions
- Understanding The Merge Sort Algorithm
- Algorithm For Merge Sort
- Implementation Of Merge Sort In C++
- Time And Space Complexity Analysis Of Merge Sort
- Advantages And Disadvantages Of Merge Sort
- Applications Of Merge Sort
- Conclusion
- Frequently Asked Questions
- Understanding The Selection Sort Algorithm
- Algorithmic Approach To Selection Sort
- Implementation Of Selection Sort Algorithm
- Complexity Analysis Of Selection Sort
- Comparison Of Selection Sort With Other Sorting Algorithms
- Advantages And Disadvantages Of Selection Sort
- Application Of Selection Sort
- Conclusion
- Frequently Asked Questions
- What Is Insertion Sort Algorithm?
- How Does Insertion Sort Work? (Step-by-Step Explanation)
- Implementation of Insertion Sort in C++
- Time And Space Complexity Of Insertion Sort
- Applications Of Insertion Sort Algorithm
- Comparison With Other Sorting Algorithms
- Conclusion
- Frequently Asked Questions
- Understanding Quick Sort Algorithm
- Step-By-Step Working Of Quick Sort
- Implementation Of Quick Sort Algorithm
- Time Complexity Analysis Of Quick Sort
- Advantages Of Quick Sort Algorithm
- Disadvantages Of Quick Sort Algorithm
- Applications Of Quick Sort Algorithm
- Conclusion
- Frequently Asked Questions
- Understanding The Heap Data Structure
- Working Of Heap Sort Algorithm
- Implementation Of Heap Sort Algorithm
- Advantages Of Heap Sort
- Disadvantages Of Heap Sort
- Real-World Applications Of Heap Sort
- Conclusion
- Frequently Asked Questions
- Understanding The Counting Sort Algorithm
- Conditions For Using Counting Sort Algorithm
- How Counting Sort Algorithm Works?
- Implementation Of Counting Sort Algorithm
- Time And Space Complexity Analysis Of Counting Sort
- Comparison Of Counting Sort With Other Sorting Algorithms
- Advantages Of Counting Sort Algorithm
- Disadvantages Of Counting Sort Algorithm
- Applications Of Counting Sort Algorithm
- Conclusion
- Frequently Asked Questions
- Understanding Shell Sort Algorithm
- Working Of Shell Sort Algorithm
- Implementation Of Shell Sort Algorithm
- Time Complexity Analysis Of Shell Sort Algorithm
- Advantages Of Shell Sort Algorithm
- Disadvantages Of Shell Sort Algorithm
- Applications Of Shell Sort Algorithm
- Conclusion
- Frequently Asked Questions
- What Is Linked List In Data Structures?
- Types Of Linked Lists In Data Structures
- Linked List Operations In Data Structures
- Advantages And Disadvantages Of Linked Lists In Data Structures
- Comparison Of Linked Lists And Arrays
- Applications Of Linked Lists
- Conclusion
- Frequently Asked Questions
- What Is A Singly Linked List In Data Structure?
- Insertion Operations On Singly Linked Lists
- Deletion Operation On Singly Linked List
- Searching For Elements In Single Linked List
- Calculating Length Of Single Linked List
- Practical Applications Of Singly Linked Lists In Data Structure
- Common Problems With Singly Linked Lists
- Conclusion
- Frequently Asked Questions (FAQ)
- What Is A Linked List?
- Reverse A Linked List
- How To Reverse A Linked List? (Approaches)
- Recursive Approach To Reverse A Linked List
- Iterative Approach To Reverse A Linked List
- Using Stack To Reverse A Linked List
- Complexity Analysis Of Different Approaches To Reverse A Linked List
- Conclusion
- Frequently Asked Questions
- What Is A Stack In Data Structure?
- Understanding Stack Operations
- Stack Implementation In Data Structures
- Stack Implementation Using Arrays
- Stack Implementation Using Linked Lists
- Comparison: Array vs. Linked List Implementation
- Applications Of Stack In Data Structures
- Advantages And Disadvantages Of Stack Data Structure
- Conclusion
- Frequently Asked Questions
- What Is A Graph Data Structure?
- Importance Of Graph Data Structures
- Types Of Graphs In Data Structure
- Types Of Graph Algorithms
- Application Of Graphs In Data Structures
- Challenges And Complexities In Graphs
- Conclusion
- Frequently Asked Questions
- What Is Tree Data Structure?
- Terminologies Of Tree Data Structure:
- Different Types Of Tree Data Structures
- Basic Operations On Tree Data Structure
- Applications Of Tree Data Structures
- Comparison Of Trees, Graphs, And Linear Data Structures
- Advantages Of Tree Data Structure
- Disadvantages Of Tree Data Structure
- Conclusion
- Frequently Asked Questions
- What Is Dynamic Programming?
- Real-Life Example: The Jigsaw Puzzle Analogy
- How To Solve A Problem Using Dynamic Programming?
- Dynamic Programming Algorithm Techniques
- Advantages Of Dynamic Programming
- Disadvantages Of Dynamic Programming
- Applications Of Dynamic Programming
- Conclusion
- Frequently Asked Questions
- Understanding The Sliding Window Algorithm
- How Does The Sliding Window Algorithm Works?
- How To Identify Sliding Window Problems?
- Fixed-Size Sliding Window Example: Maximum Sum Subarray Of Size k
- Variable-Size Sliding Window Example: Smallest Subarray With A Given Sum
- Advantages Of Sliding Window Technique
- Disadvantages Of Sliding Window Technique
- Conclusion
- Frequently Asked Questions
- Introduction To Data Structures
- Data Structures Interview Questions: Basics
- Data Structures Interview Questions: Intermediate
- Data Structures Interview Questions: Advanced
- Conclusion
Jump Search Algorithm | Working, Applications & More (+Examples)
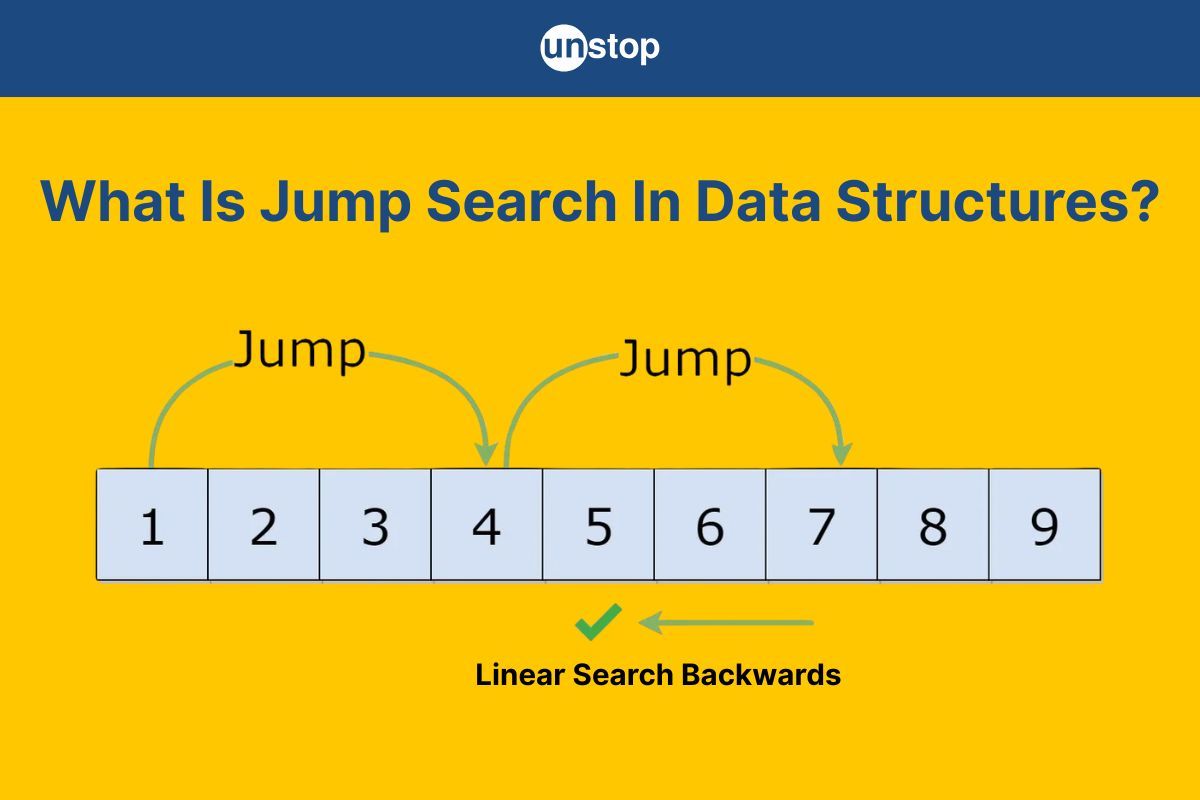
Jump Search is an efficient searching algorithm designed to strike a balance between Linear Search and Binary Search. It works by jumping ahead in fixed steps and then performing a linear search within a smaller range, reducing the number of comparisons. This algorithm is particularly useful for searching in sorted arrays, offering better performance than Linear Search while being easier to implement than Binary Search.
In this article, we will explore how Jump Search works, its step-by-step implementation, time complexity analysis, and how it compares to other search algorithms.
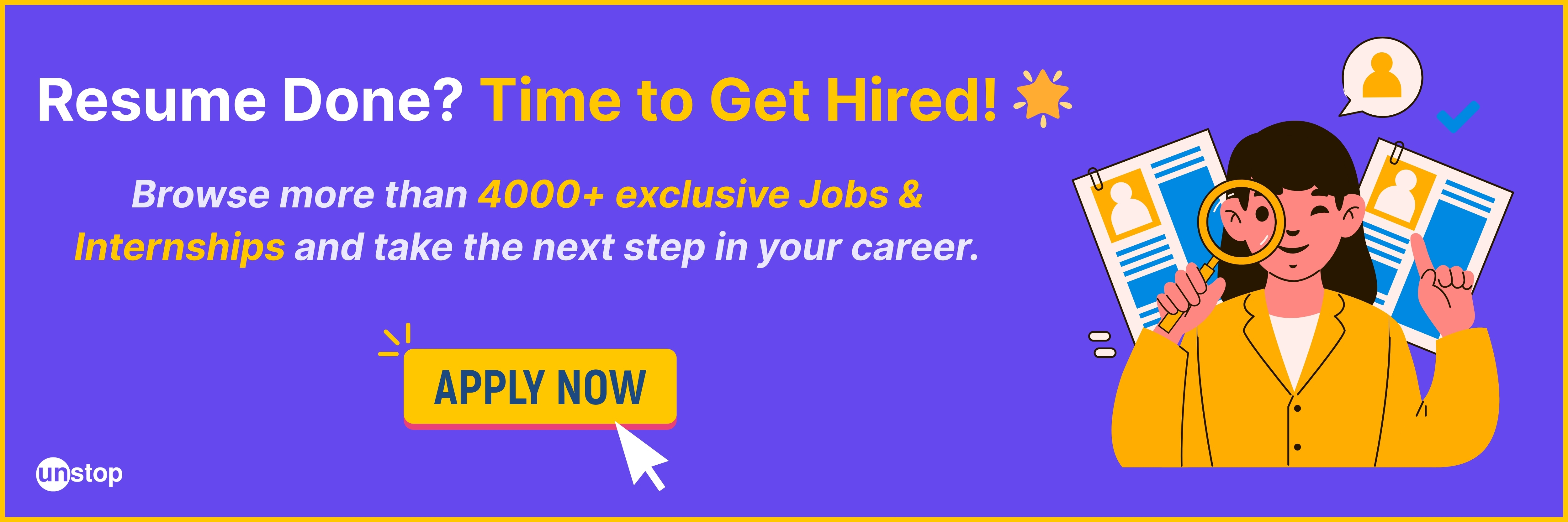
Understanding The Jump Search Algorithm
Jump Search is an efficient searching algorithm in data structures designed for sorted arrays. It works by skipping a fixed number of elements (block size) at each step instead of checking elements one by one like Linear Search. If the target element is found within a block, a linear search is performed within that block.
Working Principle:
- Choose a block size (usually √N, where N is the total number of elements).
- Jump ahead by this block size until you find a value greater than or equal to the target.
- Perform a linear search within the identified block.
- If the element is found, return its index; otherwise, return -1 (not found).
This algorithm is a trade-off between Linear Search (which checks every element) and Binary Search (which divides the array into halves).
Comparison Of Jump Search With Linear Search And Binary Search
Feature |
Jump Search |
Linear Search |
Binary Search |
Best Used For |
Large sorted arrays |
Small or unsorted arrays |
Large sorted arrays |
Time Complexity (Worst Case) |
O(√N) |
O(N) |
O(log N) |
Time Complexity (Best Case) |
O(1) (if the first element is the target) |
O(1) (if the first element is the target) |
O(1) (if the middle element is the target) |
Space Complexity |
O(1) |
O(1) |
O(1) (iterative) / O(log N) (recursive) |
Search Approach |
Skips blocks and then performs a linear search |
Checks elements one by one |
Divides array into halves |
Precondition |
Array must be sorted |
Array must be sorted |
|
Performance in Large Datasets |
Faster than Linear Search, but slower than Binary Search |
Slowest for large datasets |
Fastest among the three |
Implementation Complexity |
Moderate |
Simple |
Complex (requires recursion or iterative approach) |
Key Takeaways
- Jump Search is faster than Linear Search but not as efficient as Binary Search.
- It is useful when the dataset is sorted and Binary Search is too complex to implement in some cases.
- If frequent searching is needed, Binary Search is the best choice due to its O(log N) efficiency.
- If the dataset is small or unsorted, Linear Search may be the simplest and most practical approach.
How Jump Search Works?
Jump Search is designed for sorted arrays and works by jumping ahead in fixed steps (instead of checking elements one by one). If the target element is within a block, a linear search is performed in that block.
Let's break down the process step by step.
Step 1: Choose a Step Size
- The ideal step size is √N (square root of the array size).
- This helps balance skipping elements while not missing the target.
Step 2: Jump in Blocks
- Start from index 0 and jump ahead by step size.
- Keep jumping until you find a value greater than or equal to the target or reach the end of the array.
Step 3: Perform Linear Search in the Block
- Once you find the correct block, perform a linear search within this block.
- Compare each element in the block one by one until you find the target.
Step 4: Return the Index
- If the element is found, return its index.
- If the search reaches the end of the block without finding the element, return -1.
Example: Given sorted array-
Index: 0 1 2 3 4 5 6 7 8 9
Array: [10, 22, 30, 40, 50, 55, 60, 70, 80, 90]
- Target: 55
- Step Size: √10 ≈ 3 (rounded to 3)
Execution Steps:
- Start at index 0 → 10 (target is greater, jump ahead).
- Jump to index 3 → 40 (target is greater, jump again).
- Jump to index 6 → 60 (too large, go back to previous block).
- Perform linear search from index 4:
- Index 4 → 50 (not found).
- Index 5 → 55 (Found!).
- Return index 5 as the position of 55.
Pseudocode Of Jump Search
Function JumpSearch(arr, target, size):
step = sqrt(size) # Calculate jump size
prev = 0# Step 1: Jump through the array
while arr[min(step, size) - 1] < target:
prev = step
step += sqrt(size)
if prev >= size:
return -1 # Target not found# Step 2: Perform linear search in the block
while arr[prev] < target:
prev += 1
if prev == min(step, size):
return -1 # Target not found# Step 3: Check if the element is found
if arr[prev] == target:
return prev # Return the indexreturn -1 # Target not found
Code Implementation Of Jump Search Algorithm
Here is a C++ implementation of the Jump Search algorithm-
Code Example:
#include <iostream>
#include <cmath> // For sqrt()
using namespace std;
// Function to perform Jump Search
int jumpSearch(int arr[], int size, int target) {
int step = sqrt(size); // Block size
int prev = 0; // Previous block start index
// Jump in steps until we find a block where the target might exist
while (arr[min(step, size) - 1] < target) {
prev = step;
step += sqrt(size);
if (prev >= size) // If we exceed array size, target is not present
return -1;
}
// Perform linear search within the identified block
while (arr[prev] < target) {
prev++;
if (prev == min(step, size)) // If we reach end of the block
return -1;
}
// If target is found, return its index
if (arr[prev] == target)
return prev;
return -1; // Element not found
}
int main() {
int arr[] = {10, 22, 30, 40, 50, 55, 60, 70, 80, 90};
int size = sizeof(arr) / sizeof(arr[0]);
int target = 55;
int result = jumpSearch(arr, size, target);
if (result != -1)
cout << "Element found at index " << result << endl;
else
cout << "Element not found!" << endl;
return 0;
}
Output:
Element found at index 5
Explanation:
In the above code example-
- We include <iostream> for input-output operations and <cmath> to use the sqrt() function.
- The jumpSearch() function implements Jump Search, an efficient searching algorithm for sorted arrays.
- We determine the optimal block size as sqrt(size), which helps us skip unnecessary comparisons.
- We initialize prev to 0, representing the start of the block.
- We jump ahead in steps of sqrt(size) until we find a block where the target could exist or exceed the array bounds.
- If prev reaches or exceeds size, we return -1 since the target is not present.
- Once a potential block is identified, we perform a linear search within that block.
- If we reach the end of the block without finding the target, we return -1.
- If we find the target, we return its index.
- In main(), we define a sorted array and determine its size.
- We set the target value to 55 and call jumpSearch.
- If the target is found, we print its index; otherwise, we print "Element not found!"
- The program demonstrates an efficient way to search sorted arrays, especially for large datasets.
Time And Space Complexity Analysis
Here’s a time and space complexity analysis of Jump Search compared to Linear Search and Binary Search:
Algorithm |
Best Case (Ω) |
Average Case (Θ) |
Worst Case (O) |
Space Complexity |
Sorted Array Required? |
Jump Search |
O(1) (First element) |
O(√N) |
O(√N) |
O(1) (In-place) |
Yes |
Linear Search |
O(1) (First element) |
O(N) |
O(N) |
O(1) (In-place) |
No |
Binary Search |
O(1) (Middle element) |
O(log N) |
O(log N) |
O(1) (In-place) |
Yes |
Advantages Of Jump Search
- Faster than Linear Search – Reduces comparisons from O(N) to O(√N).
- Efficient for Large Sorted Arrays – Performs better than Linear Search in large datasets.
- Does Not Require Recursion – Works iteratively, avoiding extra function calls.
- In-Place Algorithm – Requires no extra memory (O(1) space complexity).
Disadvantages Of Jump Search
- Requires a Sorted Array – Cannot be used on unsorted data.
- Slower than Binary Search – O(√N) is worse than O(log N).
- Not Optimal for Small Arrays – Linear Search might be just as fast for small datasets.
- Finding the Optimal Step Size Can Be Tricky – The best step size is √N, but tuning it dynamically can be difficult.
Applications Of Jump Search
Here are the key areas of applications of Jump Search:
1. Searching in Large Sorted Datasets
- When dealing with large, sorted datasets, Jump Search provides an efficient alternative to Linear Search.
- It is useful when Binary Search is hard to implement, such as in linked lists, where Binary Search cannot be applied efficiently due to non-contiguous memory allocation.
2. Database Indexing
- Jump Search is used in index-based database searching, where records are sorted.
- Instead of scanning each record sequentially, it jumps through index blocks and then performs a linear search within the block containing the target record.
- Example: Searching for an entry in a B-tree index structure in databases.
3. File System Searching
- Modern file systems organize files in sorted directories.
- Jump Search helps in locating a particular file quickly by skipping sections of the directory and narrowing the search range efficiently.
- Example: Searching for a specific filename in a sorted directory of thousands of files.
4. Sparse Data Searching
- When datasets have a lot of missing or sparsely populated elements, Jump Search helps in quickly finding non-empty elements.
- Used in applications like graph algorithms, where adjacency lists are often sparse.
- Example: Searching for a non-zero element in a sparse matrix representation.
5. Optimizing Search in Memory-Limited Environments
- Since Jump Search requires O(1) extra space, it is preferred in memory-constrained environments.
- Works well in embedded systems where memory allocation is critical.
- Example: Used in microcontroller-based applications for searching sorted data efficiently.
Conclusion
Jump Search is an efficient searching algorithm that bridges the gap between Linear Search and Binary Search. By skipping ahead in fixed steps and then performing a linear search within a smaller range, it significantly reduces search time compared to Linear Search while maintaining simplicity in implementation.
While it performs well for large sorted datasets with O(√N) time complexity, it still falls behind Binary Search (O(log N)). However, its advantage lies in its low memory usage (O(1) space complexity) and iterative nature, making it suitable for indexed databases, file systems, and memory-constrained applications.
In summary, Jump Search is a great choice when Binary Search is difficult to implement and Linear Search is too slow. Understanding its strengths and limitations allows us to apply it effectively in real-world scenarios where searching efficiency is crucial.
Frequently Asked Questions
Q. What is Jump Search?
Jump Search is a searching algorithm designed for sorted arrays. Instead of checking each element one by one like Linear Search, it jumps ahead by a fixed number of steps (√N). If the element at the jump position is greater than the target value, a linear search is performed within the smaller block where the target is expected to be. This approach significantly reduces the number of comparisons compared to Linear Search.
Q. How does Jump Search differ from Binary Search?
Jump Search and Binary Search both work on sorted data, but they differ in their approach:
- Jump Search skips elements in fixed steps and then performs a linear search within a limited range. Its time complexity is O(√N).
- Binary Search continuously divides the search space into halves, reducing the number of elements at each step. Its time complexity is O(log N), making it faster than Jump Search.
- Jump Search is preferred in cases where Binary Search is difficult to implement, such as in linked lists, where elements are not stored in contiguous memory.
Q. What are the advantages of Jump Search?
- Faster than Linear Search: Instead of scanning every element, Jump Search reduces the number of comparisons significantly.
- Memory Efficient: It requires only O(1) space, meaning it does not need additional memory apart from the given input array.
- Iterative Implementation: Unlike Binary Search, which may require recursion in some cases, Jump Search works iteratively, avoiding recursive function overhead.
- Useful for Large Sorted Datasets: When data is sorted but large, and Binary Search is not feasible, Jump Search provides a better alternative to Linear Search.
Q. What are the limitations of Jump Search?
- Requires a Sorted Array: Unlike Linear Search, Jump Search cannot be applied to unsorted data.
- Slower than Binary Search: The time complexity O(√N) is higher than the O(log N) complexity of Binary Search.
- Not Ideal for Small Arrays: When working with small datasets, Linear Search can be just as fast due to lower overhead.
- Step Size Selection: Choosing the correct jump size (√N) can be challenging, especially when dealing with dynamically changing data.
Q. When should Jump Search be used?
Jump Search is useful in scenarios where:
- Binary Search is difficult to implement, such as in linked lists where direct middle access is not possible.
- The dataset is large and sorted, making Linear Search inefficient.
- Memory constraints are a concern, as Jump Search requires only O(1) extra space.
- Searching in indexed databases and file systems, where indexed blocks can be jumped over before performing a detailed search within a specific range.
Suggested Reads:
- What Is Selection Sort Algorithm? Explained With Code Examples
- Learn All About Bubble Sort Algorithm (With Code Examples)
- Merge Sort Algorithm | Working, Applications & More (+Examples)
- Quick Sort Algorithm | Working, Applications & More (+Examples)
- Heap Sort Algorithm - Working And Applications (+ Code Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Ragini yadav 1 month ago
Anish Malik 1 month ago
Ujjwal Sharma 1 month ago