Data Structures & Algorithms
Table of content:
- What Is Data Structure?
- Key Features Of Data Structures
- Basic Terminologies Related To Data Structures
- Types Of Data Structures
- What Are Linear Data Structures & Its Types?
- What Are Non-Linear Data Structures & Its Types?
- Importance Of Data Structure In Programming
- Basic Operations On Data Structures
- Applications Of Data Structures
- Real-Life Applications Of Data Structures
- Linear Vs. Non-linear Data Structures
- What Are Algorithms? The Difference Between Data Structures & Algorithms
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Asymptotic Notation?
- How Asymptotic Notation Helps In Analyzing Performance
- Types Of Asymptotic Notation
- Big-O Notation (O)
- Omega Notation (Ω)
- Theta Notation (Θ)
- Little-O Notation (o)
- Little-Omega Notation (ω)
- Summary Of Asymptotic Notations
- Real-World Applications Of Asymptotic Notation
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Big O Notation
- Types Of Time Complexity
- Space Complexity In Big O Notation
- How To Determine Big O Complexity
- Best, Worst, And Average Case Complexity
- Applications Of Big O Notation
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Time Complexity?
- Why Do You Need To Calculate Time Complexity?
- The Time Complexity Algorithm Cases
- Time Complexity: Different Types Of Asymptotic Notations
- How To Calculate Time Complexity?
- Time Complexity Of Sorting Algorithms
- Time Complexity Of Searching Algorithms
- Writing And optimizing An algorithm
- What Is Space Complexity And Its Significance?
- Relation Between Time And Space Complexity
- Conclusion
Table of content:
- What Is Linear Data Structure?
- Key Characteristics Of Linear Data Structures
- What Are The Types Of Linear Data Structures?
- What Is An Array Linear Data Structure?
- What Are Linked Lists Linear Data Structure?
- What Is A Stack Linear Data Structure?
- What Is A Queue Linear Data Structure?
- Most Common Operations Performed in Linear Data Structures
- Advantages Of Linear Data Structures
- What Is Nonlinear Data Structure?
- Difference Between Linear & Non-Linear Data Structures
- Who Uses Linear Data Structures?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is a linear data structure?
- What is a non-linear data structure?
- Difference between linear data structure and non-linear data structure
- FAQs about linear and non-linear data structures
Table of content:
- What Is Sorting In Data Structures?
- What Is Bubble Sort?
- What Is Selection Sort?
- What Is Insertion Sort?
- What Is Merge Sort?
- What Is Quick Sort?
- What Is Heap Sort?
- What Is Radix Sort?
- What Is Bucket Sort?
- What Is Counting Sort?
- What Is Shell Sort?
- Choosing The Right Sorting Algorithm
- Applications Of Sorting
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Bubble Sort Algorithm
- Bubble Sort Algorithm
- Implementation Of Bubble Sort In C++
- Time And Space Complexity Analysis Of Bubble Sort Algorithm
- Advantages Of Bubble Sort Algorithm
- Disadvantages Of Bubble Sort Algorithm
- Applications of Bubble Sort Algorithms
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Merge Sort Algorithm
- Algorithm For Merge Sort
- Implementation Of Merge Sort In C++
- Time And Space Complexity Analysis Of Merge Sort
- Advantages And Disadvantages Of Merge Sort
- Applications Of Merge Sort
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Selection Sort Algorithm
- Algorithmic Approach To Selection Sort
- Implementation Of Selection Sort Algorithm
- Complexity Analysis Of Selection Sort
- Comparison Of Selection Sort With Other Sorting Algorithms
- Advantages And Disadvantages Of Selection Sort
- Application Of Selection Sort
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Insertion Sort Algorithm?
- How Does Insertion Sort Work? (Step-by-Step Explanation)
- Implementation of Insertion Sort in C++
- Time And Space Complexity Of Insertion Sort
- Applications Of Insertion Sort Algorithm
- Comparison With Other Sorting Algorithms
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Quick Sort Algorithm
- Step-By-Step Working Of Quick Sort
- Implementation Of Quick Sort Algorithm
- Time Complexity Analysis Of Quick Sort
- Advantages Of Quick Sort Algorithm
- Disadvantages Of Quick Sort Algorithm
- Applications Of Quick Sort Algorithm
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Heap Data Structure
- Working Of Heap Sort Algorithm
- Implementation Of Heap Sort Algorithm
- Advantages Of Heap Sort
- Disadvantages Of Heap Sort
- Real-World Applications Of Heap Sort
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Counting Sort Algorithm
- Conditions For Using Counting Sort Algorithm
- How Counting Sort Algorithm Works?
- Implementation Of Counting Sort Algorithm
- Time And Space Complexity Analysis Of Counting Sort
- Comparison Of Counting Sort With Other Sorting Algorithms
- Advantages Of Counting Sort Algorithm
- Disadvantages Of Counting Sort Algorithm
- Applications Of Counting Sort Algorithm
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Shell Sort Algorithm
- Working Of Shell Sort Algorithm
- Implementation Of Shell Sort Algorithm
- Time Complexity Analysis Of Shell Sort Algorithm
- Advantages Of Shell Sort Algorithm
- Disadvantages Of Shell Sort Algorithm
- Applications Of Shell Sort Algorithm
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Binary Search Algorithm?
- Conditions For Using Binary Search
- Steps For Implementing Binary Search
- Iterative Method For Binary Search With Implementation Examples
- Recursive Method For Binary Search
- Complexity Analysis Of Binary Search Algorithm
- Iterative Vs. Recursive Implementation Of Binary Search
- Advantages & Disadvantages Of Binary Search
- Practical Applications & Real-World Examples Of Binary Search
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Linked List In Data Structures?
- Types Of Linked Lists In Data Structures
- Linked List Operations In Data Structures
- Advantages And Disadvantages Of Linked Lists In Data Structures
- Comparison Of Linked Lists And Arrays
- Applications Of Linked Lists
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Singly Linked List In Data Structure?
- Insertion Operations On Singly Linked Lists
- Deletion Operation On Singly Linked List
- Searching For Elements In Single Linked List
- Calculating Length Of Single Linked List
- Practical Applications Of Singly Linked Lists In Data Structure
- Common Problems With Singly Linked Lists
- Conclusion
- Frequently Asked Questions (FAQ)
Table of content:
- What Is A Linked List?
- Reverse A Linked List
- How To Reverse A Linked List? (Approaches)
- Recursive Approach To Reverse A Linked List
- Iterative Approach To Reverse A Linked List
- Using Stack To Reverse A Linked List
- Complexity Analysis Of Different Approaches To Reverse A Linked List
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Graph Data Structure?
- Importance Of Graph Data Structures
- Types Of Graphs In Data Structure
- Types Of Graph Algorithms
- Application Of Graphs In Data Structures
- Challenges And Complexities In Graphs
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Tree Data Structure?
- Terminologies Of Tree Data Structure:
- Different Types Of Tree Data Structures
- Basic Operations On Tree Data Structure
- Applications Of Tree Data Structures
- Comparison Of Trees, Graphs, And Linear Data Structures
- Advantages Of Tree Data Structure
- Disadvantages Of Tree Data Structure
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Dynamic Programming?
- Real-Life Example: The Jigsaw Puzzle Analogy
- How To Solve A Problem Using Dynamic Programming?
- Dynamic Programming Algorithm Techniques
- Advantages Of Dynamic Programming
- Disadvantages Of Dynamic Programming
- Applications Of Dynamic Programming
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Sliding Window Algorithm
- How Does The Sliding Window Algorithm Works?
- How To Identify Sliding Window Problems?
- Fixed-Size Sliding Window Example: Maximum Sum Subarray Of Size k
- Variable-Size Sliding Window Example: Smallest Subarray With A Given Sum
- Advantages Of Sliding Window Technique
- Disadvantages Of Sliding Window Technique
- Conclusion
- Frequently Asked Questions
Table of content:
- Introduction To Data Structures
- Data Structures Interview Questions: Basics
- Data Structures Interview Questions: Intermediate
- Data Structures Interview Questions: Advanced
- Conclusion
Sliding Window Algorithm - Working Explained With Code Examples
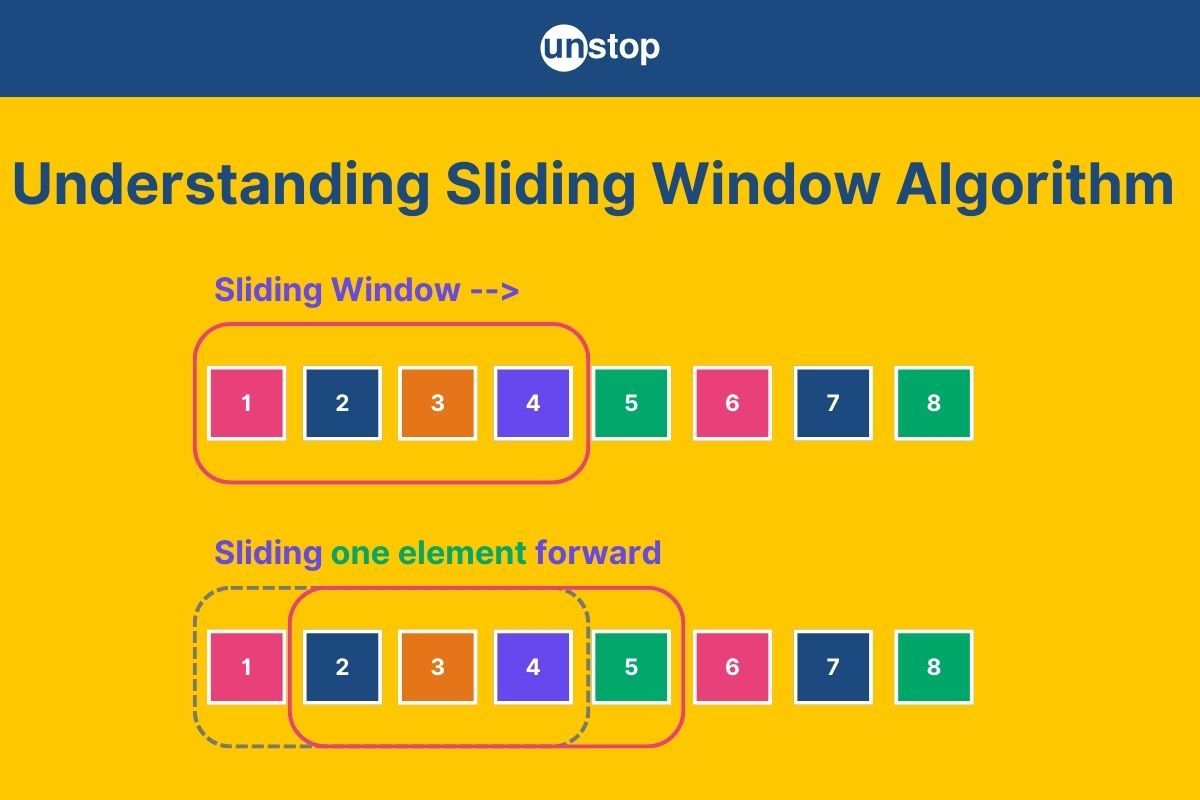
The sliding window algorithm is a powerful and efficient algorithmic approach used to solve problems involving arrays or lists. By maintaining a subset of elements within a "window" that slides over the data, this technique helps us solve complex problems like finding maximums, minimums, or specific patterns in a streamlined way. In this article, we'll explore the sliding window algorithm, understand how it works, and see practical examples to apply it effectively in coding scenarios.
Understanding The Sliding Window Algorithm
The Sliding Window Algorithm is a highly efficient technique used to solve problems involving arrays or lists, especially when the task involves finding a subset of elements that meet certain criteria (e.g., maximum sum, unique elements, etc.). Its key idea is to create a "window" that slides over the data structure to examine only a subset of the elements at a time.
Real-World Analogy
Imagine you're standing in front of a long conveyor belt with boxes of different weights. You need to find the heaviest group of k consecutive boxes. Instead of weighing every possible group, you start with the first k boxes and then "slide" to the next group by adding the weight of the next box and removing the weight of the first box. This saves time and effort compared to re-weighing everything from scratch.
How Does The Sliding Window Algorithm Works?
- Window Definition: The "window" refers to a range of indices in the array or list. The size of the window can either be fixed or dynamic, depending on the problem.
- Sliding the Window:
- Start with the window at the beginning of the array.
- Adjust the window by moving one end at a time:
- If the window size is fixed, move both the start and end of the window simultaneously.
- If the window size is dynamic, adjust the start or end as needed based on conditions.
- Optimize with Each Slide: Compute or update the desired result (like sum, maximum, or unique count) as the window slides, avoiding redundant computations.
When To Use The Sliding Window Technique?
The Sliding Window Algorithm is useful for problems like:
- Finding the maximum or minimum sum of subarrays of a fixed size.
- Counting unique elements or occurrences in a subarray.
- Longest substring with specific properties (e.g., no repeating characters, a certain number of vowels, etc.).
How To Identify Sliding Window Problems?
Recognizing when to apply the sliding window algorithm can save time and effort, especially for problems involving arrays, strings, or lists. Here's how you can identify such problems:
Key Indicators Of Sliding Window Problems
- Subarray or Substring Focus:
If the problem involves finding or processing a subset of consecutive elements in an array or string, sliding window might be applicable. - Example: "Find the maximum sum of any subarray of size k."
- Fixed or Variable Window Size:
The problem requires: - Fixed-size window: A window of constant size (e.g., subarray of size k).
- Variable-size window: The window size adjusts dynamically based on conditions (e.g., longest substring with unique characters).
- Optimize a Metric:
Look for requirements to optimize something (e.g., maximize, minimize, or count): - Maximum sum
- Minimum length
- Longest/shortest substring
- Number of distinct elements
- Sequential Processing:
The solution must process elements in a sequential order, and there is no need to revisit previous elements unnecessarily. - Example: Sliding windows work well for problems where overlapping calculations are required, and only the new and outgoing elements affect the result.
- Constraints and Patterns:
The problem often involves constraints like: - "Find the first k elements meeting a condition."
- "Sum/average of subarrays of size k."
- "Longest/shortest subsequence with specific properties."
A Quick Checklist For Sliding Window
Feature |
Sliding Window Applicable? |
Contiguous elements/subsets |
Yes |
Subset optimization |
Yes |
Sequential iteration |
Yes |
Dynamic adjustments required |
Yes |
Global property (e.g., max/min) |
Yes |
By spotting these patterns, you'll quickly identify when the Sliding Window Algorithm is the right tool for the job!
Fixed-Size Sliding Window Example: Maximum Sum Subarray Of Size k
In this example, we find the maximum sum of any subarray of size k in an array. The Sliding Window Algorithm efficiently computes this by maintaining the sum of the current window and updating it as the window slides over the array. This avoids recalculating the sum from scratch for each subarray.
Code Example:
def max_sum_subarray(arr, k):
n = len(arr)
if n < k:
return "Invalid input: Array size is smaller than k."
# Compute the sum of the first window
max_sum = sum(arr[:k])
current_sum = max_sum
# Slide the window through the array
for i in range(k, n):
current_sum += arr[i] - arr[i - k] # Add next element, remove the first element of the previous window
max_sum = max(max_sum, current_sum)
return max_sum
# Example Usage
arr = [2, 1, 5, 1, 3, 2]
k = 3
result = max_sum_subarray(arr, k)
print(f"Maximum sum of subarray of size {k}: {result}")
Output:
Maximum sum of subarray of size 3: 9
Explanation:
In the above code example-
- We begin by defining a function, max_sum_subarray, that calculates the maximum sum of any subarray of size k in the input array arr.
- First, we determine the length of the array using len(arr) and store it in n.
- If the array size n is smaller than k, we immediately return an error message: "Invalid input: Array size is smaller than k." This ensures we handle edge cases gracefully.
- Next, we compute the sum of the first k elements of the array using sum(arr[:k]). This becomes our initial max_sum and current_sum.
- Using a sliding window approach, we iterate from the kth index to the last index of the array. In each iteration:
- We update current_sum by adding the current element (arr[i]) and removing the first element of the previous window (arr[i - k]).
- We compare the current_sum with max_sum and update max_sum if current_sum is larger. This ensures we keep track of the maximum sum encountered so far.
- After iterating through the array, we return the max_sum, which is the maximum sum of a subarray of size k.
- In the example usage, we pass the array [2, 1, 5, 1, 3, 2] and k = 3 to the function. The function calculates and returns the maximum sum of a subarray of size 3, which is printed as: "Maximum sum of subarray of size 3: 9".
Variable-Size Sliding Window Example: Smallest Subarray With A Given Sum
In this example, we aim to find the smallest subarray whose sum is greater than or equal to a given value target. The Sliding Window Algorithm efficiently adjusts the window size dynamically by expanding and contracting it based on the current sum.
Code Example:
def smallest_subarray_with_sum(arr, target):
n = len(arr)
min_length = float('inf')
current_sum = 0
start = 0
for end in range(n):
current_sum += arr[end] # Expand the window by adding the current element
# Contract the window until the sum is less than the target
while current_sum >= target:
min_length = min(min_length, end - start + 1) # Update the minimum length
current_sum -= arr[start] # Remove the element at the start
start += 1 # Shrink the window from the left
return min_length if min_length != float('inf') else 0
# Example Usage
arr = [2, 3, 1, 2, 4, 3]
target = 7
result = smallest_subarray_with_sum(arr, target)
print(f"Smallest subarray length with sum >= {target}: {result}")
Output:
Smallest subarray length with sum >= 7: 2
Explanation:
In the above code example-
- We define the function smallest_subarray_with_sum to find the smallest contiguous subarray in the input array arr whose sum is greater than or equal to the given target.
- We calculate the array length using len(arr) and initialize variables: min_length to infinity (to track the smallest subarray length), current_sum to 0 (to track the current subarray sum), and start to 0 (to represent the start of the sliding window).
- Using a for loop, we iterate through the array with end representing the current index. For each end:
- We expand the window by adding arr[end] to current_sum.
- While the current_sum is greater than or equal to the target, we contract the window from the left:
- We update min_length to the smaller of its current value or the current subarray length (end - start + 1).
- We subtract arr[start] from current_sum to remove the leftmost element and then increment start to shrink the window.
- After completing the loop, we check if min_length was updated from infinity. If not, it means no valid subarray was found, so we return 0. Otherwise, we return min_length.
- In the example usage, we pass the array [2, 3, 1, 2, 4, 3] and target = 7. The function calculates and returns the smallest subarray length, which is printed as: "Smallest subarray length with sum >= 7: 2".
Advantages Of Sliding Window Technique
Some of the advantages are as follows:
- Optimized Time Complexity: Reduces time complexity from O(n²) to O(n), especially useful for large datasets.
- Reduced Redundant Computations: Avoids recalculating results for every subarray by reusing previous computations.
- Memory Efficiency: Works in-place, requiring minimal additional memory.
- Scalability: Handles large inputs efficiently, ideal for real-time or streaming data.
- Simplicity and Easy Implementation: Easy to implement with two pointers to track the window.
- Works Well for Contiguous Subarrays/Substrings: Best suited for problems with contiguous data.
- Dynamic Adjustment: Can handle problems where window size adjusts based on conditions.
Disadvantages Of Sliding Window Technique
Some of the disadvantages are as follows:
- Limited to Contiguous Subarrays/Substrings: Only works for problems where data must be contiguous, not applicable for non-contiguous subsets.
- May Not Be Ideal for All Problems: For problems involving complex conditions or dependencies across distant elements, sliding window might not be the best approach.
- Requires Precise Window Conditions: The algorithm relies on knowing the exact conditions for sliding the window, which can be challenging for some problems.
- Not Always Memory Efficient: In cases where extra space is needed for auxiliary data structures (e.g., hashmaps), memory efficiency might be compromised.
- Harder to Visualize for Complex Scenarios: For problems with dynamic window sizes or complex constraints, understanding and visualizing the window's behavior can be tricky.
Conclusion
The Sliding Window Technique is a highly efficient and versatile approach for solving problems involving contiguous subarrays or substrings. By optimizing time and space complexity, it allows us to handle large datasets and dynamic conditions without unnecessary recalculations. Whether you're working with fixed or variable window sizes, this technique ensures that the solution is both scalable and easy to implement.
However, it's essential to recognize its limitations, as it is best suited for problems where elements are processed sequentially and conditions can be checked as the window slides. With its ability to significantly reduce computational overhead, the Sliding Window technique is an invaluable tool in the problem-solving toolkit for many algorithmic challenges.
Frequently Asked Questions
Q. What is the Sliding Window Technique?
The Sliding Window Technique is an optimization method used to solve problems involving contiguous subarrays or substrings. It involves maintaining a "window" that slides over the data, updating the result incrementally without recalculating everything for every subarray.
Q. When should I use the Sliding Window Technique?
Use the Sliding Window when working with contiguous sequences, such as subarrays or substrings, where you need to optimize performance by avoiding redundant calculations. It's especially useful for problems with fixed or variable window sizes.
Q. How does the Sliding Window technique improve time complexity?
The Sliding Window reduces time complexity by avoiding recalculating values for every possible subarray. Instead of computing the sum or other properties from scratch for each window, it maintains the result and updates it incrementally as the window slides, reducing the time complexity from O(n²) to O(n).
Q. What are the limitations of the Sliding Window Technique?
The technique is best suited for problems involving contiguous elements. It may not be applicable for problems where elements are non-contiguous or when complex conditions span large sections of the array. Additionally, handling dynamic window sizes and certain edge cases can be tricky.
Q. Can the Sliding Window technique be used with variable window sizes?
Yes, the Sliding Window can handle variable window sizes. For example, when solving problems like finding the smallest subarray with a sum greater than a given target, the window size adjusts dynamically based on the current sum.
You may also like to read:
- Difference Between Hashing And Encryption Decoded
- 53 Frequently Asked Linked List Interview Questions With Answers 2024
- Data Structure Interview Questions For 2024 [With Detailed Answers]
- Tree Topology | Advantages & Disadvantages In Computer Network
- Decoding Data Redundancy In DBMS| Causes, Advantages, Solutions
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Siddhi Raney 17 hours ago
K. Mahammad Asif 2 days ago
Divyansh Shrivastava 2 days ago
Adarsh Kumar 4 days ago
Archana Ba Parmar 6 days ago
Jayant Issar 1 week ago