- What Is Data Structure?
- Key Features Of Data Structures
- Basic Terminologies Related To Data Structures
- Types Of Data Structures
- What Are Linear Data Structures & Its Types?
- What Are Non-Linear Data Structures & Its Types?
- Importance Of Data Structure In Programming
- Basic Operations On Data Structures
- Applications Of Data Structures
- Real-Life Applications Of Data Structures
- Linear Vs. Non-linear Data Structures
- What Are Algorithms? The Difference Between Data Structures & Algorithms
- Conclusion
- Frequently Asked Questions
- What Is Asymptotic Notation?
- How Asymptotic Notation Helps In Analyzing Performance
- Types Of Asymptotic Notation
- Big-O Notation (O)
- Omega Notation (Ω)
- Theta Notation (Θ)
- Little-O Notation (o)
- Little-Omega Notation (ω)
- Summary Of Asymptotic Notations
- Real-World Applications Of Asymptotic Notation
- Conclusion
- Frequently Asked Questions
- Understanding Big O Notation
- Types Of Time Complexity
- Space Complexity In Big O Notation
- How To Determine Big O Complexity
- Best, Worst, And Average Case Complexity
- Applications Of Big O Notation
- Conclusion
- Frequently Asked Questions
- What Is Time Complexity?
- Why Do You Need To Calculate Time Complexity?
- The Time Complexity Algorithm Cases
- Time Complexity: Different Types Of Asymptotic Notations
- How To Calculate Time Complexity?
- Time Complexity Of Sorting Algorithms
- Time Complexity Of Searching Algorithms
- Writing And optimizing An algorithm
- What Is Space Complexity And Its Significance?
- Relation Between Time And Space Complexity
- Conclusion
- What Is Linear Data Structure?
- Key Characteristics Of Linear Data Structures
- What Are The Types Of Linear Data Structures?
- What Is An Array Linear Data Structure?
- What Are Linked Lists Linear Data Structure?
- What Is A Stack Linear Data Structure?
- What Is A Queue Linear Data Structure?
- Most Common Operations Performed in Linear Data Structures
- Advantages Of Linear Data Structures
- What Is Nonlinear Data Structure?
- Difference Between Linear & Non-Linear Data Structures
- Who Uses Linear Data Structures?
- Conclusion
- Frequently Asked Questions
- What is a linear data structure?
- What is a non-linear data structure?
- Difference between linear data structure and non-linear data structure
- FAQs about linear and non-linear data structures
- What Is Search?
- What Is Linear Search In Data Structure?
- What Is Linear Search Algorithm?
- Working Of Linear Search Algorithm
- Complexity Of Linear Search Algorithm In Data Structures
- Implementations Of Linear Search Algorithm In Different Programming Languages
- Real-World Applications Of Linear Search In Data Structure
- Advantages & Disadvantages Of Linear Search
- Best Practices For Using Linear Search Algorithm
- Conclusion
- Frequently Asked Questions
- What Is The Binary Search Algorithm?
- Conditions For Using Binary Search
- Steps For Implementing Binary Search
- Iterative Method For Binary Search With Implementation Examples
- Recursive Method For Binary Search
- Complexity Analysis Of Binary Search Algorithm
- Iterative Vs. Recursive Implementation Of Binary Search
- Advantages & Disadvantages Of Binary Search
- Practical Applications & Real-World Examples Of Binary Search
- Conclusion
- Frequently Asked Questions
- Understanding The Jump Search Algorithm
- How Jump Search Works?
- Code Implementation Of Jump Search Algorithm
- Time And Space Complexity Analysis
- Advantages Of Jump Search
- Disadvantages Of Jump Search
- Applications Of Jump Search
- Conclusion
- Frequently Asked Questions
- What Is Sorting In Data Structures?
- What Is Bubble Sort?
- What Is Selection Sort?
- What Is Insertion Sort?
- What Is Merge Sort?
- What Is Quick Sort?
- What Is Heap Sort?
- What Is Radix Sort?
- What Is Bucket Sort?
- What Is Counting Sort?
- What Is Shell Sort?
- Choosing The Right Sorting Algorithm
- Applications Of Sorting
- Conclusion
- Frequently Asked Questions
- Understanding Bubble Sort Algorithm
- Bubble Sort Algorithm
- Implementation Of Bubble Sort In C++
- Time And Space Complexity Analysis Of Bubble Sort Algorithm
- Advantages Of Bubble Sort Algorithm
- Disadvantages Of Bubble Sort Algorithm
- Applications of Bubble Sort Algorithms
- Conclusion
- Frequently Asked Questions
- Understanding The Merge Sort Algorithm
- Algorithm For Merge Sort
- Implementation Of Merge Sort In C++
- Time And Space Complexity Analysis Of Merge Sort
- Advantages And Disadvantages Of Merge Sort
- Applications Of Merge Sort
- Conclusion
- Frequently Asked Questions
- Understanding The Selection Sort Algorithm
- Algorithmic Approach To Selection Sort
- Implementation Of Selection Sort Algorithm
- Complexity Analysis Of Selection Sort
- Comparison Of Selection Sort With Other Sorting Algorithms
- Advantages And Disadvantages Of Selection Sort
- Application Of Selection Sort
- Conclusion
- Frequently Asked Questions
- What Is Insertion Sort Algorithm?
- How Does Insertion Sort Work? (Step-by-Step Explanation)
- Implementation of Insertion Sort in C++
- Time And Space Complexity Of Insertion Sort
- Applications Of Insertion Sort Algorithm
- Comparison With Other Sorting Algorithms
- Conclusion
- Frequently Asked Questions
- Understanding Quick Sort Algorithm
- Step-By-Step Working Of Quick Sort
- Implementation Of Quick Sort Algorithm
- Time Complexity Analysis Of Quick Sort
- Advantages Of Quick Sort Algorithm
- Disadvantages Of Quick Sort Algorithm
- Applications Of Quick Sort Algorithm
- Conclusion
- Frequently Asked Questions
- Understanding The Heap Data Structure
- Working Of Heap Sort Algorithm
- Implementation Of Heap Sort Algorithm
- Advantages Of Heap Sort
- Disadvantages Of Heap Sort
- Real-World Applications Of Heap Sort
- Conclusion
- Frequently Asked Questions
- Understanding The Counting Sort Algorithm
- Conditions For Using Counting Sort Algorithm
- How Counting Sort Algorithm Works?
- Implementation Of Counting Sort Algorithm
- Time And Space Complexity Analysis Of Counting Sort
- Comparison Of Counting Sort With Other Sorting Algorithms
- Advantages Of Counting Sort Algorithm
- Disadvantages Of Counting Sort Algorithm
- Applications Of Counting Sort Algorithm
- Conclusion
- Frequently Asked Questions
- Understanding Shell Sort Algorithm
- Working Of Shell Sort Algorithm
- Implementation Of Shell Sort Algorithm
- Time Complexity Analysis Of Shell Sort Algorithm
- Advantages Of Shell Sort Algorithm
- Disadvantages Of Shell Sort Algorithm
- Applications Of Shell Sort Algorithm
- Conclusion
- Frequently Asked Questions
- What Is Linked List In Data Structures?
- Types Of Linked Lists In Data Structures
- Linked List Operations In Data Structures
- Advantages And Disadvantages Of Linked Lists In Data Structures
- Comparison Of Linked Lists And Arrays
- Applications Of Linked Lists
- Conclusion
- Frequently Asked Questions
- What Is A Singly Linked List In Data Structure?
- Insertion Operations On Singly Linked Lists
- Deletion Operation On Singly Linked List
- Searching For Elements In Single Linked List
- Calculating Length Of Single Linked List
- Practical Applications Of Singly Linked Lists In Data Structure
- Common Problems With Singly Linked Lists
- Conclusion
- Frequently Asked Questions (FAQ)
- What Is A Linked List?
- Reverse A Linked List
- How To Reverse A Linked List? (Approaches)
- Recursive Approach To Reverse A Linked List
- Iterative Approach To Reverse A Linked List
- Using Stack To Reverse A Linked List
- Complexity Analysis Of Different Approaches To Reverse A Linked List
- Conclusion
- Frequently Asked Questions
- What Is A Stack In Data Structure?
- Understanding Stack Operations
- Stack Implementation In Data Structures
- Stack Implementation Using Arrays
- Stack Implementation Using Linked Lists
- Comparison: Array vs. Linked List Implementation
- Applications Of Stack In Data Structures
- Advantages And Disadvantages Of Stack Data Structure
- Conclusion
- Frequently Asked Questions
- What Is A Graph Data Structure?
- Importance Of Graph Data Structures
- Types Of Graphs In Data Structure
- Types Of Graph Algorithms
- Application Of Graphs In Data Structures
- Challenges And Complexities In Graphs
- Conclusion
- Frequently Asked Questions
- What Is Tree Data Structure?
- Terminologies Of Tree Data Structure:
- Different Types Of Tree Data Structures
- Basic Operations On Tree Data Structure
- Applications Of Tree Data Structures
- Comparison Of Trees, Graphs, And Linear Data Structures
- Advantages Of Tree Data Structure
- Disadvantages Of Tree Data Structure
- Conclusion
- Frequently Asked Questions
- What Is Dynamic Programming?
- Real-Life Example: The Jigsaw Puzzle Analogy
- How To Solve A Problem Using Dynamic Programming?
- Dynamic Programming Algorithm Techniques
- Advantages Of Dynamic Programming
- Disadvantages Of Dynamic Programming
- Applications Of Dynamic Programming
- Conclusion
- Frequently Asked Questions
- Understanding The Sliding Window Algorithm
- How Does The Sliding Window Algorithm Works?
- How To Identify Sliding Window Problems?
- Fixed-Size Sliding Window Example: Maximum Sum Subarray Of Size k
- Variable-Size Sliding Window Example: Smallest Subarray With A Given Sum
- Advantages Of Sliding Window Technique
- Disadvantages Of Sliding Window Technique
- Conclusion
- Frequently Asked Questions
- Introduction To Data Structures
- Data Structures Interview Questions: Basics
- Data Structures Interview Questions: Intermediate
- Data Structures Interview Questions: Advanced
- Conclusion
Stack In Data Structures | Operations, Uses & More (+Examples)
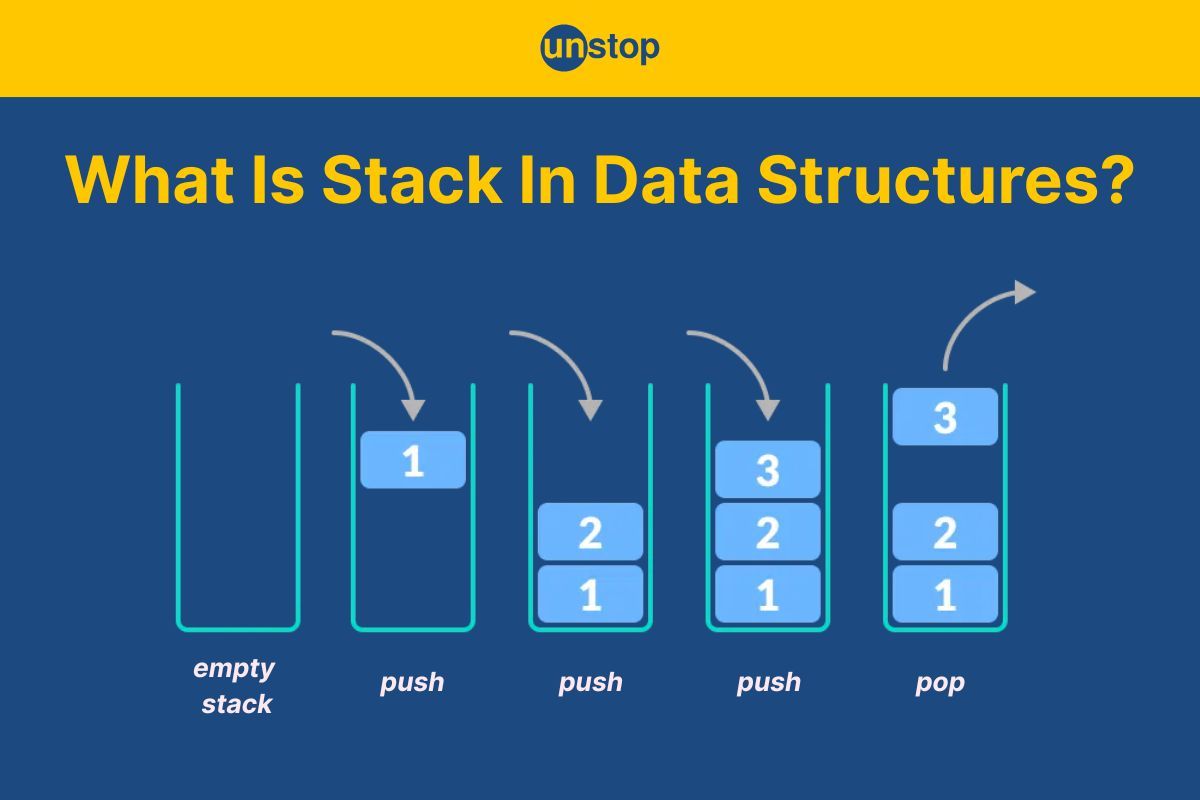
A stack is a fundamental data structure that follows the Last In, First Out (LIFO) principle, meaning the last element added is the first to be removed. Imagine a stack of plates—when you add a new plate, it goes on top, and when you remove one, you take the topmost plate first.
In this article, we'll explore the concept of a stack, its operations (push, pop, peek, and isEmpty), its implementation using arrays and linked lists, and its real-world applications in areas like recursion, expression evaluation, and undo/redo functionality.
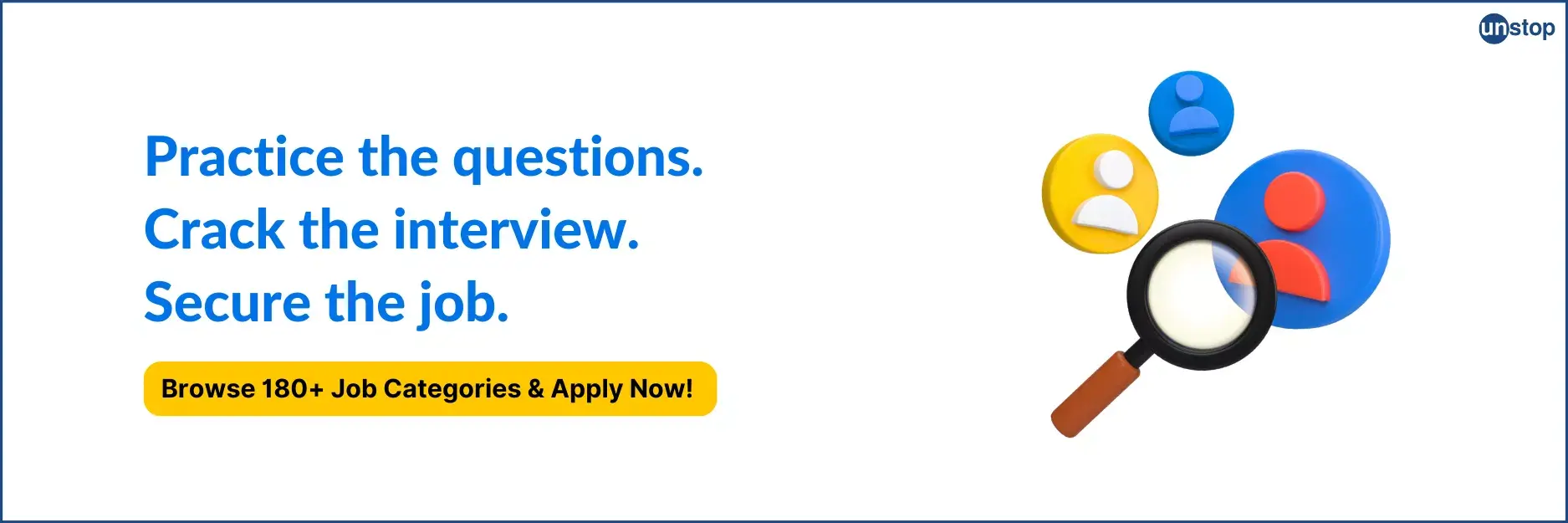
What Is A Stack In Data Structure?
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. This means that the last element added to the stack will be the first one to be removed. Think of it as a collection of elements where insertion and deletion happen only at one end, called the top of the stack.
Basic Stack Operations
A stack primarily supports the following operations:
- Push – Adds an element to the top of the stack.
- Pop – Removes the top element from the stack.
- Peek (or Top) – Returns the top element without removing it.
- isEmpty – Checks if the stack is empty.
Stacks can be implemented using arrays (fixed size) or linked lists (dynamic size). They are widely used in recursion, expression evaluation, and memory management.
Real-Life Analogy Of A Stack
Imagine a stack of plates in a cafeteria. When new plates are washed, they are placed on top of the stack. When someone needs a plate, they take the topmost plate first. You cannot take a plate from the middle or bottom without first removing the ones above it.
This is exactly how a stack works—the last plate placed (pushed) is the first one taken (popped).
Understanding Stack Operations
A stack supports four primary operations: Push, Pop, Peek (Top), and isEmpty. Let's explore each with explanations and examples.
1. Push (Insertion)
The Push operation adds an element to the top of the stack. If the stack is implemented using an array and is full, we call this a stack overflow.
Example:
Imagine we have an empty stack, and we push elements 10, 20, and 30 onto it.
Step |
Operation |
Stack (Top → Bottom) |
1 |
Push(10) |
10 |
2 |
Push(20) |
20 → 10 |
3 |
Push(30) |
30 → 20 → 10 |
Example (C++ using an array):
void push(int value) {
if (top == MAX - 1) {
cout << "Stack Overflow!\n";
return;
}
arr[++top] = value; // Increment top and add element
cout << value << " pushed to stack\n";
}
2. Pop (Removal)
The Pop operation removes the top element from the stack. If the stack is empty, we call this a stack underflow.
Example:
Continuing from the previous stack (30 → 20 → 10):
Step |
Operation |
Stack (Top → Bottom) |
1 |
Pop() |
20 → 10 |
2 |
Pop() |
10 |
3 |
Pop() |
(Empty) |
Example (C++ using an array):
void pop() {
if (top == -1) {
cout << "Stack Underflow!\n";
return;
}
cout << arr[top--] << " popped from stack\n"; // Remove top element
}
3. Peek (Top Element)
The Peek (Top) operation returns the top element without removing it.
Example:
If the stack is 30 → 20 → 10, peek() will return 30.
Example (C++ using an array):
int peek() {
if (top == -1) {
cout << "Stack is empty!\n";
return -1;
}
return arr[top]; // Return top element
}
4. isEmpty (Check if Stack is Empty)
The isEmpty operation checks whether the stack contains any elements.
Example (C++ using an array):
bool isEmpty() {
return (top == -1); // Returns true if stack is empty
}
Stack Implementation In Data Structures
Stacks can be implemented using two approaches:
- Array-based Stack – Uses static memory allocation.
- Linked List-based Stack – Uses dynamic memory allocation.
Let’s explore both implementations in detail.
Stack Implementation Using Arrays
An array-based stack uses a fixed-size array to store elements. It operates efficiently but has a fixed size limitation, meaning it cannot grow dynamically if more space is needed.
Characteristics of Array-based Stack
- Static memory allocation (fixed size).
- Fast access since indexing is direct.
- Risk of Stack Overflow when the array is full.
Code Example:
#include <iostream>
#define MAX 5 // Maximum size of stack
using namespace std;
class Stack {
int top;
int arr[MAX]; // Stack array
public:
Stack() { top = -1; } // Constructor initializes top
// Push operation
void push(int value) {
if (top == MAX - 1) {
cout << "Stack Overflow!\n";
return;
}
arr[++top] = value; // Increment top and add element
cout << value << " pushed to stack\n";
}
// Pop operation
void pop() {
if (top == -1) {
cout << "Stack Underflow!\n";
return;
}
cout << arr[top--] << " popped from stack\n"; // Remove top element
}
// Peek operation
int peek() {
if (top == -1) {
cout << "Stack is empty!\n";
return -1;
}
return arr[top];
}
// Check if stack is empty
bool isEmpty() {
return (top == -1);
}
};
int main() {
Stack s;
s.push(10);
s.push(20);
s.push(30);
cout << "Top element: " << s.peek() << endl;
s.pop();
cout << "Top element after pop: " << s.peek() << endl;
return 0;
}
Output:
10 pushed to stack
20 pushed to stack
30 pushed to stack
Top element: 30
30 popped from stack
Top element after pop: 20
Explanation:
In the above code example-
- We include <iostream> header to enable input and output operations and define MAX as 5, which represents the maximum size of the stack.
- The Stack class has two private members: top, which tracks the top index, and arr[MAX], an array that stores stack elements.
- The constructor initializes top to -1, indicating that the stack is empty initially.
- The push function checks if the stack is full (top == MAX - 1). If it is, we print "Stack Overflow!". Otherwise, we increment top and insert the new value.
- The pop function checks if the stack is empty (top == -1). If so, we print "Stack Underflow!". Otherwise, we remove and display the topmost element.
- The peek function returns the top element if the stack is not empty; otherwise, it prints "Stack is empty!" and returns -1.
- The isEmpty function checks whether the stack is empty by returning true if top == -1.
- In main(), we create a Stack object s, push three values (10, 20, 30), and display the top element using peek().
- After popping an element, we check the top element again, demonstrating the Last-In-First-Out (LIFO) behavior of the stack.
Stack Implementation Using Linked Lists
A linked list-based stack dynamically allocates memory as needed, overcoming the fixed size limitation of arrays.
Characteristics of Linked List-based Stack
- Dynamic memory allocation (no fixed size).
- Efficient memory usage (no wasted space).
- No Stack Overflow unless system memory is exhausted.
- Slower access due to pointer traversal.
Code Example:
#include <iostream>
using namespace std;
// Node structure
class Node {
public:
int data;
Node* next;
Node(int value) {
data = value;
next = nullptr;
}
};
class Stack {
Node* top;
public:
Stack() { top = nullptr; } // Constructor initializes top as NULL
// Push operation
void push(int value) {
Node* newNode = new Node(value);
newNode->next = top; // New node points to old top
top = newNode; // Update top to new node
cout << value << " pushed to stack\n";
}
// Pop operation
void pop() {
if (top == nullptr) {
cout << "Stack Underflow!\n";
return;
}
Node* temp = top;
top = top->next; // Move top pointer to next node
cout << temp->data << " popped from stack\n";
delete temp; // Free memory
}
// Peek operation
int peek() {
if (top == nullptr) {
cout << "Stack is empty!\n";
return -1;
}
return top->data;
}
// Check if stack is empty
bool isEmpty() {
return (top == nullptr);
}
};
int main() {
Stack s;
s.push(10);
s.push(20);
s.push(30);
cout << "Top element: " << s.peek() << endl;
s.pop();
cout << "Top element after pop: " << s.peek() << endl;
return 0;
}
Output:
10 pushed to stack
20 pushed to stack
30 pushed to stack
Top element: 30
30 popped from stack
Top element after pop: 20
Explanation:
In the above code example-
- We include <iostream> to enable input and output operations and use the std namespace for convenience.
- The Node class represents a stack node, containing an integer data and a pointer next that links to the next node.
- The Node constructor initializes data with the given value and sets next to nullptr.
- The Stack class has a private pointer top, which tracks the top node of the stack.
- The constructor initializes top as nullptr, indicating an empty stack.
- The push function creates a new node, sets its next pointer to the current top, and updates top to point to this new node.
- The pop function checks if the stack is empty (top == nullptr). If so, we print "Stack Underflow!". Otherwise, we store top in a temporary pointer, move top to the next node, print the popped value, and free the memory of the removed node.
- The peek function returns the value of top if the stack is not empty; otherwise, it prints "Stack is empty!" and returns -1.
- The isEmpty function checks whether the stack is empty by returning true if top == nullptr.
- In main(), we create a Stack object s, push three values (10, 20, 30), and display the top element using peek().
- After popping an element, we check the top element again, demonstrating the Last-In-First-Out (LIFO) behavior of the stack.
Comparison: Array vs. Linked List Implementation
Feature |
Array-Based Stack |
Linked List-Based Stack |
Memory Allocation |
Static (fixed size) |
Dynamic (grows as needed) |
Memory Usage |
Can waste memory if underutilized |
Efficient, uses only required memory |
Size Limitation |
Yes (defined at creation) |
No (limited only by system memory) |
Insertion/Deletion Speed |
Fast (direct index access) |
Slower (requires pointer traversal) |
Implementation Complexity |
Simpler |
Slightly more complex due to pointers |
Stack Overflow Risk |
Yes (if the array is full) |
No (until system memory is exhausted) |
Best Use Case |
When size is known and fixed |
When size is unpredictable or varies |
Therefore:
- Array-based stacks are simpler and faster but suffer from fixed size limitations.
- Linked list-based stacks offer dynamic memory allocation and avoid overflow but require extra memory for pointers and are slightly slower.
For applications with a known, limited size, array-based stacks are efficient. If the stack size is dynamic, linked list-based stacks are preferred.
Applications Of Stack In Data Structures
Stacks play a vital role in various real-world and computational applications. Let's explore some of the key uses of stacks in programming and everyday scenarios.
1. Function Call Stack (Recursion)
Use Case: Manages function calls in programming languages.
When a function is called, its execution details (such as local variables, return address, etc.) are pushed onto the stack. When the function completes, it is popped from the stack. This mechanism enables recursion to work properly.
2. Expression Evaluation
Use Case: Evaluating infix, prefix, and postfix expressions.
Stacks are used in expression conversion (infix to postfix) and expression evaluation (postfix evaluation).
Example: Postfix Expression Evaluation
A postfix expression like 3 4 + 2 * is evaluated using a stack:
- Push 3, push 4.
- Encounter + → Pop 3 and 4, compute 3 + 4 = 7, push 7.
- Push 2.
- Encounter * → Pop 7 and 2, compute 7 * 2 = 14.
Final result: 14.
3. Undo/Redo Operations
Use Case: Used in text editors and software to track changes.
- Undo: Stores previous states of a document in a stack. Pressing "Undo" pops the last state.
- Redo: Uses another stack to store undone operations. Pressing "Redo" pushes back the popped state.
4. Backtracking (Maze Solving, DFS in Graphs)
Use Case: Used in solving mazes and Depth-First Search (DFS) in graphs.
Backtracking involves exploring a path, and if it fails, reverting to the previous step (LIFO principle).
5. Browser History Navigation
Use Case: Managing web page visits in browsers.
- Back Button: Pops the last visited page from the stack.
- Forward Button: Pushes the popped page onto a redo stack.
Advantages And Disadvantages Of Stack Data Structure
Like every data structure, a stack has its own advantages and limitations. Let's explore them in detail.
Advantages Of Stack Data Structure
- Simple and Efficient – Follows LIFO (Last In, First Out), making operations easy to implement.
- Fast Operations – Push, pop, and peek take O(1) time complexity (very efficient).
- Dynamic Memory Allocation – Linked list implementation avoids fixed size limitations of arrays.
- Function Call Management – Used in recursion to store function execution states.
- Expression Evaluation – Helps in evaluating infix, prefix, and postfix expressions.
- Backtracking Support – Used in maze solving, DFS (Depth-First Search), and undo operations.
- Reversing Data – Helpful in reversing strings, linked lists, and arrays.
Disadvantages Of Stack Data Structure
- Fixed Size (Array Implementation) – If implemented with an array, size is limited and can cause stack overflow.
- No Random Access – Can only access the top element; middle elements are not directly accessible.
- Not Suitable for Frequent Modifications – Inefficient when inserting or deleting elements from the middle.
- Stack Overflow in Recursion – Too many recursive calls can lead to program crashes.
- Extra Memory Usage (Linked List Implementation) – Linked list-based stacks need extra space for pointers.
Conclusion
The Stack data structure is a fundamental concept in computer science, offering a simple yet powerful way to organize and manage data using the LIFO (Last In, First Out) principle. It is widely used in function call management, expression evaluation, backtracking algorithms, and undo/redo operations.
While stacks provide efficient push and pop operations with O(1) time complexity, they also come with limitations like fixed size (in arrays), no random access, and the risk of stack overflow in deep recursion. Choosing between an array-based or linked list-based implementation depends on the specific use case and memory constraints.
Understanding stacks helps in solving complex problems efficiently and is a building block for mastering advanced data structures like queues, trees, and graphs. By leveraging stacks effectively, we can optimize algorithm design and improve system performance.
Frequently Asked Questions
Q. What is a stack in data structures, and how does it work?
A stack is a linear data structure that follows the LIFO (Last In, First Out) principle. This means the last element added is the first one to be removed.
- It supports basic operations like:
- Push – Adds an element to the top.
- Pop – Removes the top element.
- Peek (Top) – Returns the top element without removing it.
- isEmpty – Checks if the stack is empty.
Stacks can be implemented using arrays (fixed size) or linked lists (dynamic size).
Q. How is stack different from other data structures like queues and linked lists?
Stacks are LIFO-based, whereas:
- Queues follow FIFO (First In, First Out) – elements are removed in the order they were added.
- Linked Lists allow insertion/deletion at any position, while stacks allow it only from the top.
Feature |
Stack (LIFO) |
Queue (FIFO) |
Linked List |
Insertion |
Only at the top |
At the rear (enqueue) |
Anywhere |
Deletion |
Only from the top |
From the front (dequeue) |
Anywhere |
Access |
Only top element |
Only front element |
Any element |
Use Cases |
Function calls, recursion, undo/redo, backtracking |
Scheduling tasks, buffering data |
Dynamic memory allocation |
Q. Where are stacks used in real life?
Stacks are used in various applications, including:
- Function Call Stack – Stores function execution order in recursion.
- Undo/Redo Operations – Used in text editors, graphics software, and IDEs.
- Expression Evaluation – Used in infix, prefix, and postfix calculations.
- Browser History Navigation – The "Back" button works using a stack.
- Backtracking Algorithms – Used in DFS (Depth-First Search), maze-solving, and AI pathfinding.
Q. What are the limitations of using a stack?
While stacks are useful, they have some limitations:
- Fixed Size (Array Implementation) – If implemented using an array, the size is fixed, leading to stack overflow if more elements are added.
- No Random Access – Unlike arrays, stacks allow access only to the top element, making searching inefficient.
- Recursive Stack Overflow – Excessive recursive function calls can cause stack overflow, crashing the program.
- Extra Memory in Linked List Implementation – If implemented using a linked list, each node requires extra memory for pointers.
Q. How do array-based and linked list-based stack implementations differ?
Both implementations have their pros and cons:
Aspect |
Array-Based Stack |
Linked List-Based Stack |
Memory Allocation |
Fixed (predefined size) |
Dynamic (grows as needed) |
Memory Usage |
May waste memory if oversized |
Requires extra space for pointers |
Access Speed |
Faster due to contiguous memory |
Slightly slower due to pointer traversal |
Overflow Risk |
Yes, if stack size exceeds array limit |
No, unless memory runs out |
Implementation Complexity |
Simple |
More complex due to pointer handling |
Q. How does a stack handle function calls in programming?
In programming, stacks are used to manage function calls and recursion.
- When a function is called, its execution details (local variables, return address) are pushed onto the stack.
- Once the function completes, its details are popped off the stack, and control returns to the calling function.
- This mechanism helps in handling recursive function calls efficiently.
Example: Function Call Stack in Recursion
void functionA() {
functionB(); // functionB is called and pushed onto the stack
}
void functionB() {
functionC(); // functionC is called and pushed onto the stack
}
void functionC() {
// FunctionC executes and completes
}
int main() {
functionA(); // Function A starts first
}
Stack Execution Order:
- functionA() → functionB() → functionC() (pushed onto stack).
- functionC() completes and is popped, then functionB(), then functionA().
Suggested Reads:
- What Is Selection Sort Algorithm? Explained With Code Examples
- Learn All About Bubble Sort Algorithm (With Code Examples)
- Merge Sort Algorithm | Working, Applications & More (+Examples)
- Quick Sort Algorithm | Working, Applications & More (+Examples)
- Heap Sort Algorithm - Working And Applications (+ Code Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Ujjwal Sharma 3 weeks ago
Mohit kumar Agarwal 3 weeks ago
UTKARSH SINGH 1 month ago
Kartik Deshmukh 1 month ago