Array Of Structures In C | Declare, Initialize & More (+Examples)
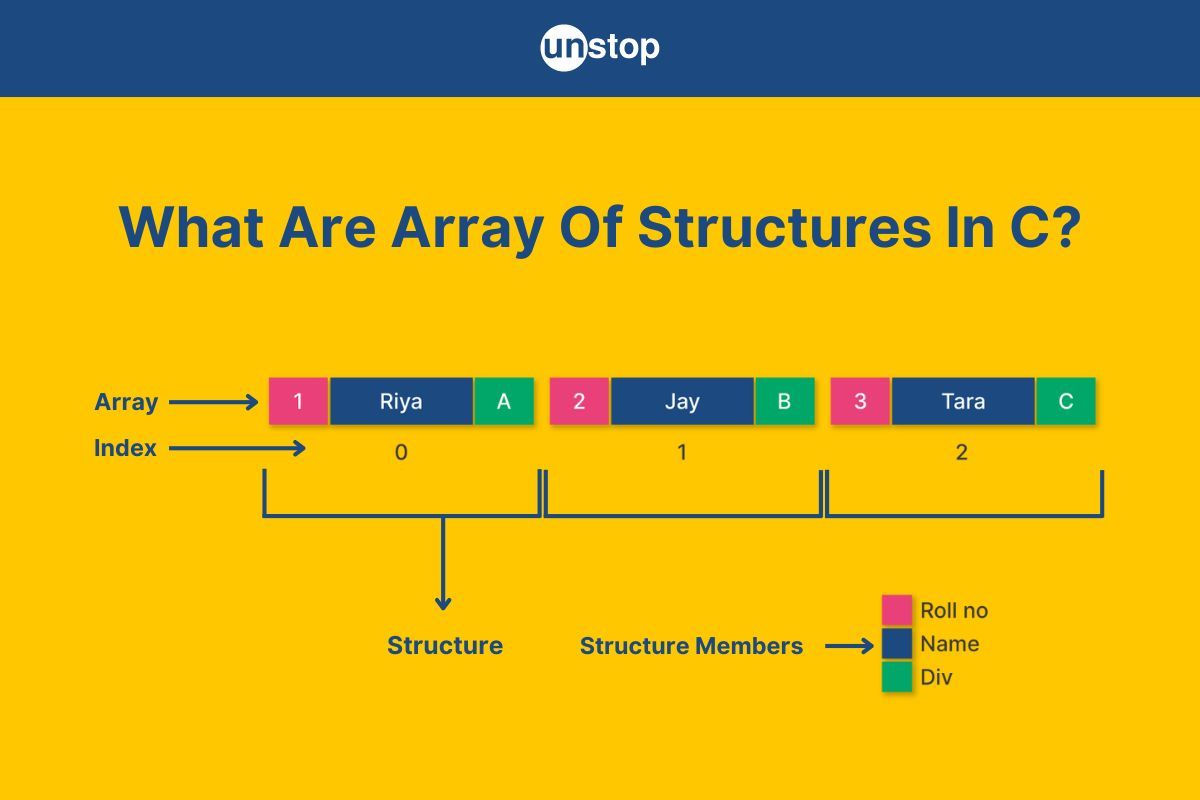
Table of content:
- What Is A Structure?
- What Is An Array Of Structure In C?
- Declaration Of Array Of Structure In C
- Initialization Of Array Of Structure In C
- Why To Use An Array Of Structures In C?
- Example Of Array Of Structures In C
- Accessing Elements Of An Array Of Structure In C
- Passing An Array Of Structures As Function Parameters
- Sorting An Array Of Structures In C
- Difference Between Array Of Structures And Array Within Structures In C
- Conclusion
- Frequently Asked Questions
As the name suggests, an array of structures in C programming is an array where each element is a structure that itself can contain data of many kinds. This way, a structure array combines the storage & retrieval properties of arrays and the organizational strength of structures. They offer an amazing solution for the effective administration of large data sets involving several entities, such as records of workers in an organization or goods for inventory, etc.
In this article, we will discuss array of structures in C programming, starting with their basic syntax and declaration and then code examples to illustrate their usage and functionality.
What Is A Structure?
A structure in C programming is a user-defined data type that allows us to group variables of different data types under a single unit. This enables the creation of complex data types that mirror real-world entities, such as information about a student, an employee, or a product.
We declare a structure by defining its type with the struct keyword in C, followed by a name and the structure variables/ members enclosed in curly braces. You can read more about structure definitions and declarations here - Structure In C | Create, Access, Modify & More (+Code Examples)
What Is An Array Of Structure In C?
In simple terms, an array of structures in C is a combination of arrays and structures, where each element of the array is a structure. This is particularly useful when we need to manage multiple instances of a complex data type that shares the same structure.
- An array of structures enables you to generate and manage numerous instances of a given structure type as a single contiguous collection.
- As an instance of the defined structure, each element of the array can be accessed and used in the same manner as a variable representing a single structure.
- The arrays of structures in C are especially useful when working with numerous records or entities that share the same set of attributes.
General Example: Say we want to keep track of different products in a store.
- We can define a Product structure to store details like the product ID, name, and price, which will be structure variables/ members.
- Then use an array to manage a list of such products. We can store information about multiple products in an array, where product info is in the form of a structure.
- This not only simplifies the organization of otherwise complex data, but we can also access data of each product conveniently.
Declaration Of Array Of Structure In C
The process of declaring an array of structures in C begins with the structure definition itself. That is, you must first declare a structure using the struct keyword, including the structure name, type, and details of members.
Once you have a declared structure type, you can declare an array of structures for that type.
- For this, use the struct keyword with the type of structure, the array's name, and the number of elements (or structure instances) the array should contain.
- In this sense, one can say that declaring an array of structures is a way to manage multiple instances of a structure type.
- It involves defining a structure type and then creating an array that consists of elements of that structure type.
Array Of Structure Syntax:
struct StructureName {
dataType1 member1;
dataType2 member2;
// Additional members
};// Declaration of an Array of Structures
struct StructureName arrayName[arraySize];
Here,
- struct StructureName { dataType1 member1....; }: It defines the structure with its data members.
- struct StructureName arrayName[arraySize]: It declares an array of structures that stores multiple instances of the structure declaration.
Let’s continue with our previous product and store example. The snippet below shows how we can store each product info, and then combine multiple products into an array.
struct Product {
int productID;
char name[50];
float price;
};struct Product store[10]; // This is an array of 10 structures of type Product
Here, store[] is an array that can hold 10 Product structures, each containing product ID, product name, and product price.
Initialization Of Array Of Structure In C
Initializing an array of structures involves assigning values to the members of each structure in the array. There are two primary ways in which we can initialize an array of structures in C. We will discuss both methods in this section.
Method 1: Individual Initialization Of Array Of Structures In C
In this method, we first declare an array of structures and then set the values of each structure’s members one by one.
Syntax:
struct StructureName ArrayName[Size];
ArrayName[index].member1 = value1; // Initialize the first member of the structure at index
ArrayName[index].member2 = value2;
//Initialize more structure memebers
Here,
- The ArrayName refers to the name of the array with Size indicating the number of elements/ structures it can store.
- Each element in this array is of type struct StructureName.
- We use the array [index] to access a specific element in the array (i.e., structure).
- The dot operator (.) helps us access the member1 of this structure, and we initialize this member with value1 using the assignment operator(=). We follow the same process to initialize more structure members.
Method 2: Inline Initialization Of Array Of Structures In C
In this method, we set the values of the structure members directly within the declaration statement.
Syntax:
struct StructureName ArrayName[Size] = { {value1_member1, value1_member2, ...}
..., {valueN_member1, valueN_member2, ...} };
Here,
- struct StructureName: It specifies the type of structure.
- ArrayName[Size]: It declares an array of structures with a given size.
- { {value1_member1, value1_member2, ...}, ..., {valueN_member1, valueN_member2, ...} }: It refers to the values we assign to each member of the structure, inside the array. Note that the outer curly braces refer to the array and inner braces refer to the structure.
Inline initialization for arrays of structures in C can itself be done in three ways. Though the syntax changes are minor, knowing these approaches might come in handy. These include:
1. Nested Braces Initialization: This is the most commonly used approach for inline initialization of an array of structures in C (as discussed above). It involves using two sets of curly braces, where the outer braces refer to the array (i.e., contain all structures), and each of the inner braces refers to an individual structure within the array.
Each structure’s members are listed within its own set of braces following the order in which they are declared inside the structure definition.
Syntax:
struct StructureName ArrayName[Size] = {
{value1_member1, value1_member2, ...},
{value2_member1, value2_member2, ...},
...
};
2. Flattened Form Initialization: In this, we list the values for all members of all structures in a single, flat sequence (one after the other) without nested braces.
Syntax:
struct StructureName ArrayName[Size] = {
value1_member1, value1_member2, ...,
value2_member1, value2_member2, ...,
...
};
Here, we have eliminated the inner braces, and are initialitizing the structures in the same order in which they exist in the array.
3. Designated Initializers: It allows us to specify values for particular members of the structures using the dot operator, providing more explicit control over the initialization process.
Syntax:
struct StructureName ArrayName[Size] = {
[index1] = {.member1 = value1, .member2 = value2, ...},
[index2] = {.member1 = value3, .member2 = value4, ...},
...
};
Below is a simple C program showcasing how to create an array of structures in C and initializing its members:
Code:
Output:
Point 0: (1, 2)
Point 1: (3, 4)
Point 2: (5, 6)
Explanation:
In the above array of structure examples,
- We start by including the standard input-output header file, which is necessary for functions like printf().
- Then, we define a Point structure with two member variables: x and y, which represent the coordinates of a point.
- In the main() function, we declare a single array of Point structures named points with a size of 3, i.e., it will contain three structures of type Point.
- Next, we then individually initialize each element of the points array using the index notation (to specify the structure) and the dot operator to access the structure member. In that-
- First, we set the first point’s coordinates to (1, 2).
- Next, we set the second point’s coordinates to (3, 4).
- Finally, we set the third point’s coordinates to (5, 6).
- We then use a for loop to iterate over the array and print elements of each structure:
- The control variable i is initialized to 0 and the loop continues iteration until i<3, i.e., it runs 3 times.
- In every iteration, we use printf() to display the coordinates in the formatted string, where we access each coordinate using the dot operator.
- Here, the format specifiers %d is the placeholder for the integer values of coordinates.
- Finally, the main function returns 0, indicating that the program executed successfully.
Why To Use An Array Of Structures In C?
Arrays of structures serve several important purposes, making them essential for organizing and managing related data effectively. Here are the key benefits of using an array of structures in C:
1. Organized Data Management: An array of structures in C allows us to group related data under a single unit/entity. Instead of managing separate entities or array variables for each piece of data, we can handle a collection of data items as a single entity. For example:
struct Student {
int id;
char name[50];
float marks;
};
struct Student students[5];
2. Efficient Data Handling: An array of structures in C simplifies the process of iterating through, accessing, and modifying a collection of similar data items. It’s more efficient than handling individual structures separately. For example:
for (int i = 0; i < 5; i++) {
students[i].marks += 5.0; // Increase marks for all students
}
3. Scalable Code Design: Arrays of structures in C scale well as our data set grows. We can easily increase the size of the array to accommodate more data without changing the underlying code logic. For example:
struct Student students[10]; // Increase size to 10
4. Consistent Data Operations: Performing operations like sorting, searching, and filtering is more straightforward with the implementation of arrays of structures. For example,
qsort(employees, numEmployees, sizeof(struct Employee), compareBySalary);
5. Improved Memory Management: Arrays of structures in C allow for contiguous memory allocation, which can be more efficient in terms of memory access patterns. For example,
struct Order orders[50]; // Contiguous memory allocation for 50 orders
6. Easy Data Initialization: You can initialize all elements of an array of structures at once, which can be more convenient and helps you avoid errors in your program. For example,
struct Car cars[3] = {
{1, "Sedan", 15000.00},
{2, "SUV", 25000.00},
{3, "Hatchback", 18000.00}
};
Example Of Array Of Structures In C
In this section, we will look at multiple examples of an array of structures in C programming language to further cement the concepts of structure definition, initialization of an array of structures, accessing and manipulating elements, and more.
Example 1: Employee Information Using Array Of Structures In C
In this example, we will see how we can create a structure to store Employee information with fields for the employee's ID, name, and salary. Then, we will create an array of Employee structures, initialize them, and print their details.
Code:
Output:
Employee ID: 101
Name: Jahnvi
Salary: INR 55000.00Employee ID: 102
Name: Jia
Salary: INR 60000.00
Explanation:
In the above code example,
- We begin by defining a structure named Employee with three members: an integer id to store the employee’s ID, a character array name of size 50 to store the employee’s name, and a float salary to represent the employee’s salary.
- In the main() function, we declare an array named staff that contains 2 Employee structures. We will now initialize this array with the following data:
- The first employee has an ID of 101, a name "Jahnvi," and a salary of 55000.0.
- The second employee has an ID of 102, a name "Jia," and a salary of 60000.0.
- We then use a for loop to iterate through each element of the staff array.
- The control variable, i, is initialized to 0, and the loop continues iteration until condition i<2, i.e. it will run twice, once for each employee.
- For each employee, we print out their ID, name, and salary using the printf() function.
- We format the salary to show up to two decimal places using %.2f format specifier.
- Finally, the program returns 0, indicating successful completion.
Example 2: Array Of Structures In C For GPS Coordinates
In this example, we will create a structure to store GPS Coordinates, including fields for latitude and longitude. Then, we will define an array of Coordinate structures, populate it with data for various locations, and demonstrate how to access and print each location's latitude and longitude.
Code:
Output:
Location 1:
Latitude: 37.7749
Longitude: -122.4194Location 2:
Latitude: 34.0522
Longitude: -118.2437Location 3:
Latitude: 40.7128
Longitude: -74.0060
Explanation:
In the above code example,
- We begin by defining a macro named NUM_LOCATIONS with a value of 3, which represents the number of locations that we will store.
- Next, we define a structure called Coordinate that has two members: latitude and longitude, both of type double to store GPS coordinates.
- In the main function, we declare an array named locations of type struct Coordinate with a size of NUM_LOCATIONS (which is 3).
- We also initialize this array with three sets of GPS coordinates for different locations:
- The first location is San Francisco, which has a latitude of 37.7749 and a longitude of -122.4194.
- The second location is Los Angeles, which has a latitude of 34.0522 and a longitude of -118.2437.
- The third location is New York, which has a latitude of 40.7128 and a longitude of -74.0060.
- Next, we use a for loop to iterate through the locations array:
- The control variable i is initialized to 0, and the loop continues iteration until condition i<3, i.e. it runs three times.
- In every iteration, we use a printf() statement to display the location number, latitude, and longitude.
- Finally, the main function returns 0, indicating that the program executed successfully.
Accessing Elements Of An Array Of Structure In C
In C, accessing elements of an array of structures is a two-step process:
- First, we use the array name followed by the index to select the structure (record) we want to refer to.
- Next, we use the dot operator to access the members of that selected structure.
Syntax:
arrayName[index].memberName
Here,
- arrayName: It represents the name of an array of structures.
- index: It is the position of the structure in the array (starting from 0).
- memberName: It is the name of the member field within the structure.
- dot operator (.): It provides us with the access to the structure member.
Code:
Output:
Book 1:
Title: The Catcher in the Rye
Author: J.D. Salinger
Year: 1951Book 2:
Title: To Kill a Mockingbird
Author: Harper Lee
Year: 1960
Explanation:
In the above code example,
- We begin by defining a macro named NUM_BOOKS with a value of 2, which specifies the number of books we will manage in our array.
- Next, we define a structure named Book with three members: two character arrays/ strings title and author of size 50 each, and an integer year to record the year of publication.
- In the main() function, we declare an array named books of type struct Book with a size of NUM_BOOKS (which is 2). We initialize this array with data for two books:
- The first book has a title of "The Catcher in the Rye", an author as "J.D. Salinger", and a year of 1951.
- The second book has a title of "To Kill a Mockingbird", an author as "Harper Lee", and a year of 1960.
- We then use a for loop to iterate through the books array:
- The control variable, i is initialized to 0 and the loop continues iteration until condition i<2 i.e it will run twice.
- In each iteration, we use printf() to print the details, such as title, author, and year of the current book.
- Note that we access the members of the Book structure using the array index and the member name.
Passing An Array Of Structures As Function Parameters
In C, when we pass an array of structures to a function, we are actually passing a pointer to the first element of the array. This means that changes made to the array elements in the function will affect the original array in the calling function. There are two main methods for passing an array of structures in C programs as a function parameter:
- Using Array Notation
- Using Pointer Notation
Method 1: Passing An Array Of Structures Using Array Notation
In this method, we declare the function parameters using array notation. We generally use array notation for code reusability and readability.
Syntax:
void functionName(struct StructName arrayName[], int size);
Here,
- functionName: It is the name of the function.
- struct StructName arrayName[ ]: It represents an array of structures passed to the function.
- int size: It is the number of elements present in the array.
Note that the syntax we use to pass the array of structures as a function parameter is the same as that for the declaration of an array of structures in C.
Code:
Output:
Course at index 0: Code = 101, Name = Mathematics
Course at index 1: Code = 102, Name = Physics
Explanation:
In the above code example:
- We begin by defining a Course structure with two members: an integer code to store the course’s code number and a string type name of size 30 to hold the course’s name.
- Next, we declare a function named showCourses() that takes two parameters: an array of Course structures and an integer numCourses representing the number of courses in the array. This function is responsible for displaying the details of each course.
- Inside the function, we use a for loop to iterate through the course array. For each course, we print the code and name of the course, along with the index of the course in the array.
- In the main() function, we declare an array named courseArray of type Course and initialize it with data for two courses:
- The first course has a code of 101 and the name "Mathematics".
- The second course has a code of 102 and the name "Physics".
- We then call the showCourses function, passing the courseArray (by reference) and the number of courses (2) as arguments.
- This function call displays the code and name of each course along with their index in the array.
Method 2: Passing An Array Of Structures Using Pointer Notation
In this approach, we pass a pointer to the array of structures as a function parameter. The function uses the pointer variable to access and manipulate the array's elements.
Syntax:
void functionName(struct StructName *arrayName, int size);
Here,
- functionName: It is the name of the function.
- struct StructName *: It is a pointer to the structure type.
- arrayName[ ]: It is the name of the array parameter.
- int size: It is the number of elements present in the array.
Code:
Output:
Product at index 0: ID = 1, Name = Laptop
Product at index 1: ID = 2, Name = Smartphone
Explanation:
In the above code example,
- We define a Product structure with two members: an integer id to store the product’s ID and a character array name of size 30 to hold the product’s name.
- Next, we declare a function named showProducts() that takes two parameters: a pointer to an array of structures (*products) and an integer numProducts representing the number of products in the array (array dimensions).
- This function displays the details of each product. Inside it, we use a for loop to iterate through the products array using the pointer.
- For each product, we print the id and name of the product, along with the index of the product in the array.
- In the main function, we declare an array named productArray of type Product and initialize it with data for two products:
- The first product has an id of 1 and a name of "Laptop".
- The second product has an id of 2 and a name of "Smartphone".
- We then call the showProducts() function, passing productArray (by pointer) and the number of products (2) as arguments.
- This function call displays the id and name of each product along with their index in the array.
Sorting An Array Of Structures In C
This method involves arranging the elements of the array based on a specific criterion (ascending or descending order). The most common way to achieve the sorting of an array of structures in C is by using a sorting algorithm, such as Bubble Sort, Selection Sort, or Quick Sort, and a comparison function to determine the order of the elements.
Approach:
Follow the steps listed below to sort an array of structures in C:
- Define a Structure: We start by creating a structure to hold the data we want to sort. The structure has members to store different pieces of information.
- Create and Initialize the Array: Next, we declare an array of the structure and initialize it with data.
- Write a Comparison Function: We now write a comparison function to tell the sorting algorithm how to compare two elements. This function helps decide the order of the elements.
- Call the qsort() Function: We use the qsort() function to sort the element array. We pass the array, the number of elements, the size of each element, and the comparison function as arguments.
- Print the Sorted Array: After sorting, you can iterate over the array to display the sorted results.
Let's look at a simple code example that sorts an array of structures using the qsort() function.
Code:
Output:
Products sorted by price (ascending):
ID: 3, Name: Headphones, Price: $89.99
ID: 2, Name: Smartphone, Price: $499.49
ID: 1, Name: Laptop, Price: $999.99
Explanation:
In the above code example,
- We define a Product structure with three members: an integer id to store the product’s ID, a character array name of size 50 for the product’s name, and a float price to represent the product’s price.
- Next, we create a comparison function named compareByPrice() that takes two const void * pointers as parameters, which are the items to be compared.
- Inside the function, we first typecast these pointers to type struct Product * so that we can access the price members.
- Then, we compare the price of the two structures pointed to by productA and productB as follows:
- If the price of productA is less than the price of productB, we return -1.
- If the price of productA is greater than the price of productB, we return 1.
- If the prices of productA and productB are equal, we return 0.
- Note that we use the arrow operator/ this pointer (->) to access the price member of the Product structures through the pointers productA and productB.
- In the main function(), we declare and initialize an array of structure (type Product) named products. This array contains three products with different IDs, names, and prices.
- We then call the qsort() function to sort the products array passing the array, array dimension/ size (3), the size of each element (using the sizeof() operator), and the comparison function compareByPrice as arguments.
- This function sorts the products by their price in ascending order by default.
- After sorting, we print the details of the products to the console using printf():
- We first use a for loop to go through the sorted array.
- For every iteration, we print each product’s ID, name, and price, formatting the price to two decimal places.
- Finally, the main function returns 0, indicating that the program executed successfully.
Difference Between Array Of Structures And Array Within Structures In C
Given below is a table that lists the key differences between an array of structures and an array within structures in C.
Aspect | Array Of Structures | Array Within Structures |
---|---|---|
Definition | It is an array where each element is a separate instance of a structure type. | It is a structure that contains an array as one of its members. |
Data Organization | It organizes data by creating multiple instances of a structure. Each instance holds distinct data. | It organizes data by embedding array components as a member within a single structure. This allows the structure to handle a homogeneous collection of data. |
Usage | Best used when you need to handle multiple distinct entities of the same type, like a list of products or employees. It increases code readability and code efficiency. | Ideal for scenarios where a single record contains an array of integers or related values, such as a company with a list of employee IDs. |
Declaration Syntax | struct StructureName arrayName[arraySize]; | struct StructureName { dataType arrayName[arraySize]; }; |
Accessing Elements | Access individual structures using array indexing to specify the structure and access structure members with dot notation. | Access the array within the structure using dot notation to specify the member and array indexing to specify the array element. |
Initialization | Each structure in the array can be initialized individually or collectively in a single statement. | The array within the structure is initialized as part of the structure instance. |
Code Example: Array Within Structures
Output:
Team Name: Dev Team
Member IDs:
Member 1 ID: 1
Member 2 ID: 2
Member 3 ID: 3
Explanation:
In the above code example,
- We begin by defining a structure called Team with two members: a character array name of size 50 to store the team’s name and an array of integers members of size 3 to hold the IDs of the team members.
- In the main() function, we declare an instance of the Team structure named myTeam and initialize it with the name "Dev Team" and member IDs 1, 2, and 3, respectively.
- Next, we use printf() to display the team’s name and member IDs. We print the team name using %s to format the string.
- Then we use a for loop to go through each of the three-member IDs:
- The control variable, i, is initialized to 0, and the loop continues iteration until condition i<3, i.e. it runs three times.
- Inside the loop, we use the printf() function to print each member’s ID with a label like "Member 1 ID:", "Member 2 ID:", and so on.
- Finally, the main function returns 0, which signals that the program finished successfully.
Conclusion
The array of structures in the C programming language is a powerful tool that offers a structured approach to organizing complex data types. It makes it easier to store, access, and manipulate information efficiently. By defining a structure, we can group various data types into a single logical unit. By creating an array of these structures, we can efficiently handle multiple instances of this unit. Mastering these techniques allows us to create more organized and maintainable code for various applications.
Frequently Asked Questions
Q. What is the difference between an array and an array of structures in C?
Here's a table explaining the key differences between a standard array and an array of structures in C:
Aspect | Array | Array Of Structures |
---|---|---|
Definition | A collection of elements of the same data type. | A collection of structures, where each structure can have different data types. |
Data Type | Single data type (e.g., int, float, char). | Composite data type consisting of multiple fields of different types. |
Purpose | To store a list of constant values of the same type. | To store a list of records, each containing different types of data. |
Access Method | array[index] (e.g., numbers[0]) | array[index].field (e.g., people[0].name) |
Usage | For simple lists like integers, characters, or floating-point values. | For complex data like student records, employee details, etc. |
Example Usage | int scores[3] = {90, 85, 88}; | struct Person people[2] = {{"Alia", 30}, {"Bhaskar", 25}}; |
Initialization | All elements must be of the same type. | Each structure in the array can have multiple fields of different types. |
Memory Layout | Contiguous memory for elements of the same type. | Contiguous memory, where each element is a structure with multiple fields. |
Q. Can I initialize an array of structures at the time of declaration?
Yes, you can initialize an array of structures at the time of declaration. This process is called inline initialization of an array of structures in C programming. You can do this by providing a list of initializers for each structure in the array. For example,
struct Employee staff[2] = {
{101, "John Doe", 55000.0},
{102, "Jane Smith", 60000.0}
};
In the above code fragment, we initialize staff with two Employee structures, setting the id, name, and salary for each employee.
Q. Can you resize an array of structures in C?
In C, the size of a static array of structures is fixed at compile time and cannot be changed during runtime. Therefore you cannot directly resize an array of structures in C once it has been created. However, you can use dynamic memory allocation input functions like malloc(), calloc(), and realloc() to manage the size of the array at runtime. For example:
#include <stdlib.h>
struct Employee *staff = malloc(initialSize * sizeof(struct Employee)); // Allocate memory
staff = realloc(staff, newSize * sizeof(struct Employee)); // Resize array
free(staff); // Free memory when done
In the above code, we first start by allocating memory for the initial size of the array, then resize it as needed, and finally deallocate the memory.
Q. List three common use cases of an array of structures in C.
Here are the three common and important use cases of an array of structures in the C programming language:
- Storing Student Records: An array of structures can be used to store information about multiple students, including details like name, ID, age, and grades. This is a classic example where arrays of structures help organize and display student data efficiently.
- Tracking Products in a Store: An array of structures in C can be used to store product details and perform operations such as listing products, calculating total inventory value, or applying discounts.
- Managing Game Entities: An array of structures can represent multiple game entities, which can be used for tasks like rendering connected data collection of objects in game, handling collisions, or updating game states to boost memory performance.
Q. Is it possible to pass an entire structure or array of structures to a function by value, and what are the implications?
Yes, you can pass an entire structure or array of structures to a function by value, but it is not recommended for large arrays due to cache memory and performance concerns. Passing by value copies the entire data structure or array, which can be inefficient. For example:
void modifyBook(struct Book book) {
book.year = 2024; // This modification does not affect the original book
}int main() {
struct Book myBook = {"1984", "George Orwell", 1949};
modifyBook(myBook);
printf("Year: %d\n", myBook.year); // Prints: 1949, not 2024
}
In the above input code, when myBook is passed to modifyBook by value, the function operates on a copy, so changes do not affect the original structure.
Here are a few other topics you might be interested in:
- Nested Loop In C | For, While, Do-While, Break & Continue (+Codes)
- Arithmetic Operations In C | Types & Precedence Explained (+Examples)
- 100+ Top C Interview Questions With Answers (2024)
- Ternary (Conditional) Operator In C Explained With Code Examples
- 6 Relational Operators In C & Precedence Explained (+Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment