Python Programming Table of content:
Python List index() Method | Use Cases Explained (+Code Examples)
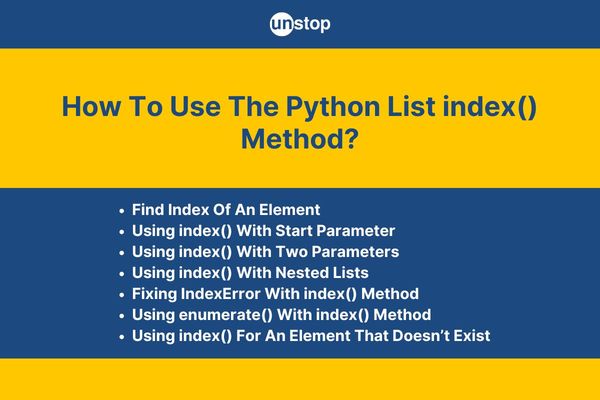
Python lists are versatile data structures that allow you to store, retrieve, and manipulate collections of elements. Python uses indexing–a powerful way to pinpoint the exact position of an item, to access or locate specific elements within a list.
In this article, we will focus on the list index() method, which helps find the position of a given element in a list. We'll look at its syntax, parameters, return values, and practical use cases, ensuring you understand both the fundamentals and nuances of this function in Python programming.
What Is Indexing In Python?
Indexing in Python refers to accessing individual elements of a sequence (like a list, tuple, or string) using their position or index. Python uses zero-based indexing, meaning the first element of a list is at position 0, the second at 1, and so on.
Code Example:
Here, the index 0 retrieves the first element, "Python". Indexing simplifies data retrieval, making it an essential concept when working with Python lists or other sequences.
The Python List index() Function
The index() method is a built-in function in Python used to find the first occurrence of an element in a list and return its index position. If the element appears multiple times in the list, the method will only return the index of its first occurrence. This method is very useful when you need to determine the position of an item without manually searching through the list.
Syntax Of Python List index() Method
list.index(element, start, stop)
Parameters Of Python List index() Method
As shown in the syntax above, the index() method takes the following parameters:
- element: The item whose index you want to find.
- start (optional): The position from which the search should start (default is 0).
- stop (optional): The position where the search should end (default is the end of the list).
Return Value Of Python List index() Method
The method returns the index of the first occurrence of the specified element. If the element is not found, it raises a ValueError.
What Does Return Lowest Index Value Mean?
When using the index() method, if the element occurs multiple times in the list, Python will return the lowest index value—which means it returns the index of the first appearance of the element. This behavior is crucial to understand when working with lists that may contain duplicate items.
Code Example:
Output:
1
In the basic Python program example, even though element 3 appears twice in the list (at indices 1 and 3), the index() method returns 1 because it is the first occurrence of the element.
How To Use Python List index() To Find Index Of A List Element
The index() function in Python is straightforward to use when you need to find the position of a specific element in a list. You simply pass the element as the argument, and it will return its first occurrence index. This method is ideal when you're sure the element exists in the list, and you only need its position.
In the basic Python code example below, we show how to use index() method to find the index of a string element, from a list of strings.
Code Example:
Output:
2
Code Explanation:
In the example,
- We create a list of strings Unstop containing four string elements.
- Then, we call the index() method on Unstop list and pass ‘compete’ as the argument.
- The method returns the index 2, where ‘compete’ is located.
This method is a quick way to retrieve an element's index when you’re dealing with known data.
Check out this amazing course to become the best version of the Python programmer you can be.
The Python List index() Method With Single Parameter (Start)
As mentioned before, the index() method takes two optional parameters: start and end. It can be used with just the optional start parameter that defines the position from which to begin searching for the element. When you provide this start index, the search will only consider the portion of the list from that index onward. The simple Python program example below illustrates this scenario.
Code Example:
Output:
3
Code Explanation:
In this example, we begin with the list Unstop with five string elements.
- Note that there is one duplicate element in the list, i.e., practice.
- Then, we call the index() method on the Unstop list, passing the element ‘practice’ and the 2 for start as arguments.
- When we specify 2 as the start index, it means Python will start the search from index 2 onward, skipping the first occurrence at index 1.
- As a result, the Python function finds the element at index 3, which it returns.
This shows how using the start parameter with index() allows you to search a list starting from a specific point. This can be useful when you know the element appears later in the list.
The Python List index() Method With Start & Stop Parameters
In addition to the start parameter, the index() method also allows you to specify a stop parameter. This defines the point where the search should end. When both are specified, the built-in Python method will search for the element between the start and stop indexes, making it useful when you want to restrict the search to a particular range of the list.
Code Example:
Output:
1
Code Explanation:
In the simple Python code example,
- We begin with the same Unstop list with one duplicate element.
- Then, we call the index() method on Unstop, passing three parameters– ‘practice’ element, 1 for start and 4 for end.
- So, the search begins at index 1 and stops at index 4 (exclusive).
- The first occurrence of ‘practice’ within this range is at index 1, so that's the value returned.
- We store the value in the variable index_value and then use the print() function to display it.
Using both parameters allows you to fine-tune your search and avoid unnecessary parts of the list.
What Happens When We Use Python List index() For An Element That Doesn't Exist
If the element you're trying to find does not exist in the list, Python will raise a ValueError. This is a built-in exception that signals the absence of the specified element in the list. The Python program example below illustrates this scenario.
Code Example:
Output:
Error: 'pro' is not in list
Code Explanation:
In the Python code example,
- We have a list of string, Unstop, with four elements.
- Then, we set a try-except block:
- We call the index() method on the Unstop list inside the try block passing element ‘pro’ as an argument.
- Since 'pro' is not found in the Unstop list, a ValueError is raised, which we catch using the except block.
- It prints an error message to the console.
- Ideally, when we look for an element that does not exist in the list, it raises a ValueError. But here, we use try-except to handle such situations and prevent the program from crashing.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and get the bragging rights now!
Python List index() With Nested Lists
When working with nested lists (lists containing other lists as elements), the index() method works in the same way as it does with flat lists. However, keep in mind that the method will search for an element within the outer list and not inside the inner lists. This means if you want to find an index of an element within a nested list, it will only return the position of that element in the outer list.
Code Example:
Output:
1
Code Explanation:
In the example Python program,
- We begin with a nested list, Unstop, that contains three lists with 2 string elements each.
- Then, we call the index() method on Unstop nested list, passing the list [‘learn’, ‘compete’] as the argument.
- The inside list is at index 1 in the outer list, so the index() method returns 1, which we store in the variable index_value.
- We then print the index value to the console.
Note that if you want to search for an element inside the inner lists, you will need to flatten the list or loop through each sublist to search.
Fixing IndexError Using The Python List index() Method
The IndexError: list index out of range occurs when you attempt to access an index that is outside the valid range of indices for a list. While the index() method itself doesn't raise an IndexError directly, it can help you fix scenarios where improper index usage might lead to this error. By ensuring the element exists and retrieving its index dynamically, you can prevent such errors.
In the example Python code below, we illustrate how to use the index() method to prevent the IndexError.
Code Example:
Output:
The index of 'get hired' is: 3
Code Explanation:
In the example,
- We begin with the Unstop list and initialize variable element_to_find with the string value ‘get hired’.
- Then, we use an if-statement to check if the element exists in the list using the in keyword.
- If the element exists, Unstop.index(element_to_find) retrieves its index.
- If the element doesn’t exist, a custom message is displayed, preventing any attempts to access an invalid index and thus avoiding an IndexError.
Python List index() With Enumerate()
When working with lists, sometimes you might need to find both the index and the value of the element you're searching for. While the index() method can give you the index, it doesn't provide the element itself.
This is where enumerate() comes in handy. It allows you to loop over the list and get both the index and the value at the same time. Using enumerate() in combination with index() can simplify scenarios where you need to perform a more complex search across the list.
Code Example:
Output:
Index of practice is: 1
Code Explanation:
In the sample Python program,
- We use a for loop to iterate over list Unstop. In the loop condition, we use enumerate() to generate pairs of (index, value) and use it as looping range.
- The loop checks if the current value is 'practice'.
- As soon as it finds the first occurrence of the list element, it prints the index and stops further searching after encountring break.
This method is efficient, especially when you need to handle more than just finding the index, such as comparing values or performing additional operations within the loop.
Real-world Examples Of Python List index() Method
The index() method can be very useful in real-world applications where you need to find the position of an element in a list for various purposes, such as data analysis, searching, or processing user input. Given below are few practical examples of where index() can be handy.
Example: Finding the Position of a User in a List of Names
Imagine you're working on a project where you have a list of users, and you need to find the position of a specific user to display their information.
Code Example:
Output:
The index of Shivani is 2
In the sample Python code, index() helps you locate the position of a user in the list of names so you can fetch their details from a database or perform other actions based on their position.
Example: Inventory Management
Let's say you're managing an inventory list of items, and you want to find the position of a specific item to update its stock quantity or price.
Code Example:
Output:
The index of tablet is 2
This is particularly useful in inventory management systems where you need to update or track products by their position in the list.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Difference Between find() And index() Method In Python
Both the find() method (available for strings) and the index() method (available for lists) are commonly used for searching an element. While they share similarities, they differ significantly in how they handle errors and return values. Here's a comparison to clarify the distinctions:
Feature |
index() |
find() |
Usage |
Used to find the index of an element in a list. |
Used to find the index of a substring in a string. |
Return Value |
Returns the index of the first occurrence. |
Returns the index of the first occurrence. |
Error Handling |
Raises a ValueError if the element is not found. |
Returns -1 if the substring is not found. |
Data Types |
Works with lists. |
Works with strings. |
Example of Error |
ValueError: 'apple' is not in list |
find() will return -1 when the substring is not found. |
Return on Absence |
Throws an error. |
Returns -1. |
Example: index() vs find()
Let's compare both methods with an example. Here, we will use the index() method to find elements in a list and find() to get substrings from a string.
Code Example:
Output:
Using index() - 'orange' not found
6
-1
Code Explanation:
In the Python program sample,
- For the list, we use index() to search for 'orange', which raises a ValueError because the element is not in the list.
- For the hello, world string, find() searches for 'world', returning its index 6, but for 'orange', it returns -1 as it doesn’t exist in the string.
As you can see, find() is safer for strings when you're unsure if the element exists, while index() is ideal when you want to handle the exception and make sure the element is present.
Conclusion
The Python list index() method is an essential tool when you need to find the position of an element in a list.
- It allows you to pinpoint the first occurrence of a value, and with the ability to use optional parameters like start and stop, you can fine-tune your search within specific ranges.
- Handling exceptions with try-except blocks ensures that your program doesn’t crash when the element isn't found, making it more robust.
- Whether you're working with simple lists, nested lists, or integrating with other functions like enumerate(), the index() method proves to be versatile.
- Its key differences with find() (especially for strings) should be kept in mind to choose the best tool for your specific needs.
By mastering the index() method, you can effectively search through lists and handle errors gracefully, a skill that will come in handy in various real-world applications like data processing, inventory management, and beyond.
Frequently Asked Questions
Q1. What is a list index in Python?
A list index in Python refers to the position of an element within a list. It starts at 0 for the first element and increases by 1 for each subsequent element.
Q2. What does the index() method do in Python lists?
The index() method in Python returns the index of the first occurrence of a specified element in a list. If the element is not found, it raises a ValueError.
Q3. Can I specify a range to search within using the index() method?
Yes, you can specify an optional start and stop parameter when using the Python list index() method. These parameters allow you to search within a specific portion of the list, instead of the entire list.
Q4. How do I use the index() method to find the index of a specific value in a list?
You can use the index() method by calling it on the list, passing the value you're looking for as an argument. For example:
my_list.index('banana')
Q5. What is the "list index out of range" error?
The "list index out of range" error occurs when you try to access an index that is beyond the valid range of indices for a list. This error typically happens when the index is either negative (and there aren’t enough elements to account for it) or exceeds the list's length.
Think You Know Python List Indexing? Take A Quiz!
QUIZZ SNIPPET IS HERE
QUIZZ SNIPPET IS HERE
QUIZZ SNIPPET IS HERE
This compiles our discussion on the Python list index() method. Do check the following out:
- Find Length Of List In Python | 8 Ways (+Examples) & Analysis
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- Python input() Function (+Input Casting & Handling With Examples)
- Python Libraries | Standard, Third-Party & More (Lists + Examples)
- Python Assert Keyword | Types, Uses, Best Practices (+Code Examples)