Table of content:
- What Is Structured Programming?
- What Are The Components Of Structured Programming?
- Types Of Structured Programming
- Difference Between Structured & Unstructured Programming
- Advantages & Disadvantages Of Structured Programming
- Conclusion
- Frequently Asked Questions
What Is Structured Programming? Components, Types, & Examples
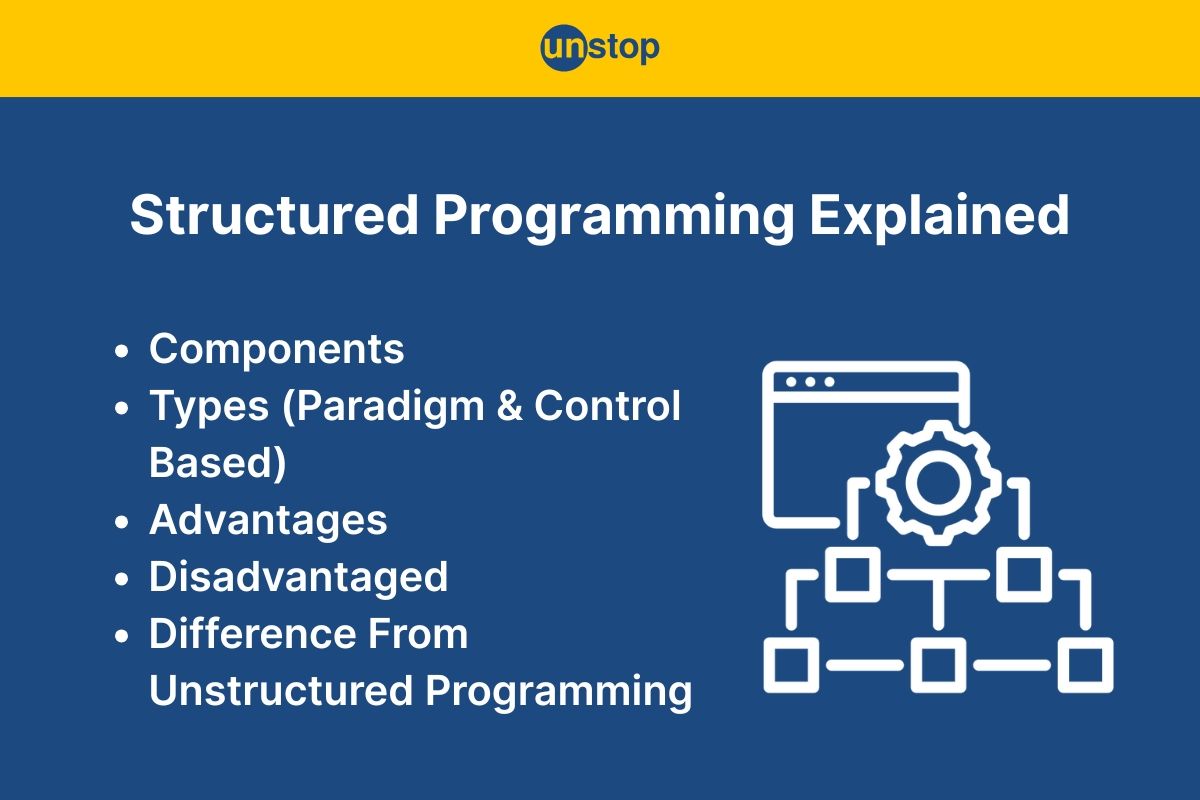
Ever tried untangling a pair of earphones that have been in your bag/pocket for a while? Well, that is what early programming looked like–chaotic, messy, and a bit disastrous. What I mean is that developers wrote code that jumped all over the place, which made debugging a nightmare. This led to the birth of structured programming, a method that brings order and clarity to code, making it easier to read, write, and maintain.
In this article, we’ll break down what structured programming is, its key components, types, advantages, and how it differs from the unstructured approach.
What Is Structured Programming?
At its core, programming is about giving instructions to a computer to perform tasks. But the way these instructions are structured can make all the difference between elegant, maintainable code and a tangled mess.
- Structured programming is a programming paradigm that emphasizes a logical, clear flow of control using well-defined structures such as sequence, selection, and iteration.
- Unlike the free-flowing, jump-heavy approach of unstructured programming, structured programming ensures that every part of the code follows a predictable path, making it easier to debug, test, and modify.
A Brief History
Structured programming emerged in the late 1960s as a response to the increasing complexity of software. A major turning point was Edsger Dijkstra’s 1968 paper, “Go To Statement Considered Harmful,” which criticized the overuse of the goto statement–an infamous culprit behind spaghetti code.
This led to a movement that ultimately led to the adoption of structured control flow mechanisms, laying the foundation for modern programming languages like C, Pascal, and Python.
What Are The Components Of Structured Programming?
Structured programming is built on a few fundamental components that dictate how code should be written and executed. These components ensure that the program follows a logical flow, making it easy to understand and maintain.
The three main components of structured programming are:
- Sequence
- Selection (Decision Making like If-Else statements)
- Iteration (Looping statements)
We will discuss each of these in this section, with the help of code examples.
Sequence: The Foundation Of Structured Code
The sequence structure ensures that instructions execute in a logical, step-by-step manner, just like following a set of directions. Every statement is executed exactly once in the order it appears, without skipping or repeating unless explicitly directed.
Real-World Analogy: Imagine you are following a recipe to bake a cake. You can’t randomly mix ingredients or bake before preparing the batter. The process follows a sequence—step 1, step 2, step 3, and so on.
Code Example:
#include <iostream>
using namespace std;
int main() {
cout << "Step 1: Gather ingredients" << endl;
cout << "Step 2: Mix ingredients" << endl;
cout << "Step 3: Bake at 180°C for 30 minutes" << endl;
return 0;
}
Output:
Step 1: Gather ingredients
Step 2: Mix ingredients
Step 3: Bake at 180°C for 30 minutes
Explanation: The program executes each instruction in the order it is written in, i.e., first gathering ingredients, then mixing, and finally baking. There are no conditionals or loops here, just a straight sequence of steps.
Key takeaway: The sequence structure ensures that instructions execute in the order they appear.
Selection: Making Decisions In Code
We are constantly making some sort of decision (big or small) in our daily lives. For example, should I carry an umbrella, what route to take to and fro work/college, or the most common what to eat (or what not to eat for some of us).
In programming, we use decision-making statements/selection structures to make decisions. These statements help the compiler/interpreter decide between various options to execute, based on specified conditions.
Key Selection Statements In Structured Programming
These help a program choose between different execution paths instead of always following a fixed sequence. Let’s look at an example to see how these statements actually work.
Code Example:
#include <iostream>
using namespace std;
int main() {
int temperature;
cout << "Enter temperature: ";
cin >> temperature;
if (temperature > 30) {
cout << "It's hot outside!" << endl;
} else {
cout << "The weather is pleasant." << endl;
}
return 0;
}
Output (if input is 35):
It's hot outside!
Output (if input is 25):
The weather is pleasant.
Explanation: The program evaluates the input temperature. If it is greater than 30, it prints "It's hot outside!" Otherwise, it prints "The weather is pleasant." This conditional branching allows the program to adapt to different scenarios.
Iteration: Handling Repetitive Tasks Efficiently
Repetition is common in programming. Whether it's processing multiple data points, running a countdown, or displaying a menu, loops help automate repetitive tasks. But what would happen if you had to write a new line of code for every repetitive task? Uh!
Thankfully, we have iteration structures (or loops) that help repeat a block of code until a condition is met, eliminating the need to write the same code multiple times.
Common Loop Types
- for loop: Used when the number of iterations is known.
- while loop: Used when the condition is checked before each iteration.
- do-while loop: Runs at least once before checking the condition.
Code Example:
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 3; i++) {
cout << "Iteration " << i << endl;
}
return 0;
}
Output:
Iteration 1
Iteration 2
Iteration 3
Explanation: The loop starts at i = 1 and runs until i <= 3, incrementing i in each iteration. This automates the repetitive task of printing iteration numbers instead of manually writing three cout statements.
These three components—Sequence, Selection, and Iteration—are the backbone of structured programming. They allow developers to write clear, logical, and maintainable code by ensuring:
- Sequence enforces a logical execution order.
- Selection enables decision-making in programs.
- Iteration simplifies repetitive tasks through loops.
It ultimately ensures that programs are easy to follow, debug, and modify, unlike the chaotic jumps of unstructured code.
Quick Knowledge Check
Types Of Structured Programming
Structured programming isn't a one-size-fits-all approach. It has multiple variations based on how programs are organized and executed. In other words, structured programming isn’t just a singular method; it has multiple variations based on how a program is organized and executed. These variations fall into two broad categories:
- Paradigm-Based Types: These define how programs are designed and structured.
- Control Structures: These dictate the logical flow of execution within a program.
Paradigm-Based Categorization
A paradigm in programming refers to a fundamental style or approach to structuring and solving problems using code. In structured programming, paradigms define how instructions are grouped, reused, and managed to ensure clarity and maintainability. There are three major paradigm-based types of structured programming, as mentioned below:
1. Procedural Programming: The Foundation of Structured Code
Procedural programming is the most fundamental form of structured programming. It follows a step-by-step approach, where programs are broken down into a set of procedures or functions that execute in a logical sequence.
Key Characteristics:
- Focuses on functions (or procedures) that contain reusable blocks of code.
- Uses variables and control structures to manipulate data.
- Follows a top-down approach, where execution flows from the main function downward.
- Commonly used in languages like C, Pascal, and early Python scripts.
Example Scenario: Imagine a library management system where you need to implement book-issuing and returning processes. Instead of writing the same logic multiple times, procedural programming allows you to create dedicated functions for these tasks.
Code Example:
#include <stdio.h>
// Function to issue a book
void issueBook(){
printf("Book issued successfully.\n");
}
// Function to return a book
void returnBook(){
printf("Book returned successfully.\n");
}
int main(){
issueBook();
returnBook();
return 0;
}
Output:
Book issued successfully.
Book returned successfully.
Explanation:
- Here, we first import the <stdio.h> header file.
- Then, we define two functions in the program above, namely, issueBook() and returnBook().
- Both use the printf() function to display a string message to the console.
- In the main() function, we call these functions/procedures sequentially, demonstrating a structured and organized approach.
- This eliminates redundancy and improves code readability and reusability.
2. Modular Programming: Organizing Code into Independent Units
As programs grow larger and more complex, procedural programming can become difficult to manage. Modular programming solves this by dividing a program into independent, self-contained modules, each handling a specific functionality.
Key Characteristics:
- Breaks a program into smaller, manageable modules, improving readability and debugging.
- Each module contains related built-in functions, making it easier to maintain and test.
- Encourages code reuse by allowing modules to be imported into different programs.
- Used in languages like Python (modules), Java (packages), and C (header files & separate files for functions).
Example Scenario: Consider a university student portal where different modules handle student registration, fee payment, and exam results. Modular programming ensures that each feature can be coded separately, reducing complexity.
Code Example (Python with Modules):
student.py (Module File)
def register_student(name):
print(f"{name} registered successfully.")main.py (Main File)
import student
student.register_student("Shivani")
Explanation:
- The function register_student() is defined inside a separate module (student.py).
- In main.py, we import the module and call the function, following the modular approach.
- This separation makes it easy to reuse the module in multiple programs without rewriting the code.
3. Object-Oriented Programming (OOP): Structuring Code With Objects
Object-oriented programming (OOP) builds upon modular programming but introduces objects as the primary way to structure programs. Instead of relying solely on functions, OOP organizes code into classes and objects that encapsulate both data (attributes) and behaviors (methods).
Key Characteristics:
- Uses classes and objects to bundle related data and functionality.
- Encourages encapsulation, inheritance, and polymorphism for better code organization.
- Reduces redundancy by allowing objects to share properties and methods.
- Commonly used in languages like Java, C++, and Python (OOP implementation).
Example Scenario: Imagine an online shopping platform where each product has a name, price, and a method to apply discounts. Instead of maintaining separate variables for each product, OOP lets you create a Product class that handles this data efficiently.
Code Example (C++ Class & Object):
#include <iostream>
using namespace std;
// Class definition
class Product {
public:
string name;
double price;
void applyDiscount(double discount) {
price -= (price * discount / 100);
cout << "New price of " << name << " is: â¹" << price << endl;
}
};
int main() {
Product laptop;
laptop.name = "Gaming Laptop";
laptop.price = 50000;
laptop.applyDiscount(10);
return 0;
}
Output:
New price of Gaming Laptop is: ₹45000
Explanation:
- Here, we define a class called Product with two attributes/data members: name (of tye string) to store the name of the product and price (double data type) to store the price of the product.
- We also define a behavious/member function applyDiscount() which takes an input price and returns the price after applying a specified amount of discount.
- In the main() function, we create an object laptop and then instantiate its attributes with values– “Gaming Laptop” name and 50000 price.
- The, we apply the method to the object. The applyDiscount() method reduces the price dynamically, demonstrating encapsulation and real-world modeling of entities.
Control Structures In Structured Programming
While paradigms define how programs are structured, the control structures define how execution flows within a program. We've already covered these in the Components of Structured Programming section, but to recap:
- Sequence: Ensures that instructions execute in a logical order.
- Selection (Decision-Making): Uses if-else and switch statements to choose between different execution paths.
- Iteration (Loops): Uses for loops, while loops, and do-while loops to handle repetition efficiently.
These structures provide the building blocks that support all paradigm-based approaches, ensuring structured execution flow.
Quick Knowledge Check
Difference Between Structured & Unstructured Programming
Programming has come a long way, evolving from the chaotic world of unstructured programming to the methodical and logical approach of structured programming. But why does this distinction matter? Because the way we organize code directly impacts its readability, maintainability, and efficiency.
Structured programming provides a clear, predictable control flow, making programs easier to debug and scale. Unstructured programming, on the other hand, often leads to spaghetti code–a mess of jumps (goto statements) that make debugging a nightmare. The table below highlights the key differences between the two:
Feature |
Structured Programming |
Unstructured Programming |
Code Organization |
Divides code into functions, procedures, and modules. |
Consists of a single block of code with little or no division. |
Control Flow |
Uses sequence, selection (if-else), and iteration (for, while). |
Relies heavily on goto statements, leading to unpredictable jumps. |
Readability |
Highly readable and easy to understand. |
Difficult to follow due to scattered flow. |
Maintainability |
Easier to debug, modify, and scale. |
Modifications often introduce errors due to complex flow. |
Reusability |
Encourages code reuse with functions and modules. |
Code is often repeated instead of reused. |
Error Handling |
Errors are easier to locate and fix. |
Debugging is challenging due to lack of structure. |
Commonly Used In |
C, Java, Python, C++, etc. |
Early languages like Assembly, old versions of BASIC. |
Advantages & Disadvantages Of Structured Programming
Why Structured Programming Matters? Structured programming has become the foundation of modern software development. By following a well-defined flow using sequences, selections, and iterations, it ensures better readability, maintainability, and efficiency. However, like any approach, it has its own set of strengths and limitations.
Advantages of Structured Programming |
Disadvantages of Structured Programming |
|
|
Quick Knowledge Check
Conclusion
Structured programming transformed the way we write code, replacing chaotic, hard-to-follow logic with a clear, organized approach. By using sequence, selection, and iteration, it ensures that programs are readable, maintainable, and scalable.
From small scripts to large-scale applications, structured programming helps developers create efficient, bug-free software. While it may have some limitations, its advantages make it the backbone of modern programming languages like C, Python, and Java.
Understanding and applying structured programming principles is essential for writing clean, efficient, and professional-grade code. So, whether you're just starting or refining your coding skills, embracing structured programming is a step in the right direction!
Frequently Asked Questions
Q1. What is structured programming in simple terms?
Structured programming is a programming approach that organizes code into logical structures like sequences, selections (decision-making), and iterations (loops). It avoids chaotic jumps (like goto statements) and ensures the code is readable, maintainable, and efficient.
Q2. How is structured programming different from unstructured programming?
Structured programming follows a clear flow of control using functions, loops, and conditionals, making it organized and easy to debug. In contrast, unstructured programming relies on direct jumps (goto statements), making the code harder to read and maintain.
Q3. What are the three key components of structured programming?
The three main components are:
- Sequence: Executes instructions in a specific order.
- Selection: Uses conditional statements (if-else, switch) to make decisions.
- Iteration: Uses for, do-while, and while loops to repeat tasks.
Q4. Which programming languages follow structured programming?
Languages like C, Java, Python, and C++ strongly support structured programming principles. Most modern languages encourage structured programming by default.
Q5. Can structured programming be used in object-oriented languages like Java or Python?
Yes! While OOP (object-oriented programming) introduces additional concepts like classes and objects, structured programming is still fundamental in writing methods, functions, and loops within those languages.
6. What are the advantages of structured programming?
- Improves readability
- Easier debugging and maintenance
- Enhances code reusability
- Facilitates teamwork
- Reduces complexity
Q7. Is structured programming outdated?
Not at all! Structured programming remains a core principle in modern development. Even in OOP and functional programming, structured logic is crucial for writing clean and efficient code.
Also read:
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
ARUN MISHRA 13 hours ago
Sounak Nandi 19 hours ago
Sunita Bhat 1 day ago
Anshika Kumari 1 day ago
K. Mahammad Asif 1 day ago
Arunima Gupta 1 day ago