Table of content:
- What Is File Handling In C?
- Types Of Files In C
- File Pointer For File Handling In C
- Operations For File Handling In C
- Open A File | Text File Handling In C
- Create A File | Text File Handling In C
- Read & Write | Text File Handling In C
- Close A File | Text File Handling In C
- Binary File Handling In C (Open, Read & Write)
- File Handling In C With fseek()
- File Handling In C With rewind()
- List Of All Functions For File Handling In C
- The Difference Between Text & Binary Files
- Conclusion
- Frequently Asked Questions
File Handling In C (Text & Binary) Explained With Code Examples
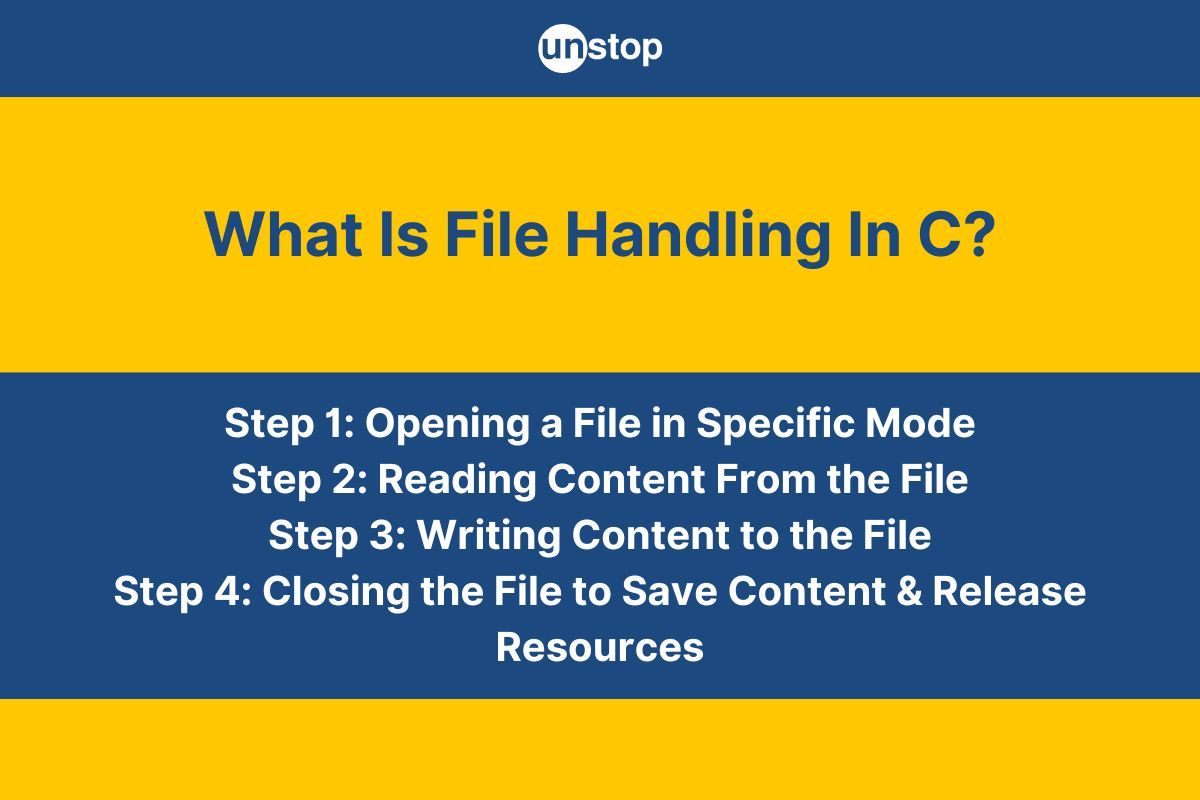
File handling in C bridges the gap between your program and your device's storage. It allows your program to interact with files—whether you're reading data, writing information, or just organizing things. Without file handling, everything your program does would disappear the moment it stops running.
It is safe to say that file handling is a critical aspect of any programming language as it enables the interaction between the program and the file system. In this article, we will walk you through the essentials of file handling in C programming, beginning with what it is, the need, and how to use it. We will also cover all operations (reading, writing, closing, and opening), best practices in file handling, common pitfalls, and more.
What Is File Handling In C?
The process of managing files through various operations like reading, writing, creating, and modifying data stored on your computer is called file handling in C language. Think of a file as a collection of data stored outside your program—file handling allows your C program to interact with this data, so you don't have to reload or re-enter information every time the program runs.
- C provides several standard functions that make file handling possible.
- It allows developers to work with files efficiently and enables the program to persist data beyond its runtime.
- File handling is essential in real-world applications where data storage and retrieval are fundamental, such as when managing databases, logs, or user-generated content.
Now that you have a clear idea of what file handling in C entails, we will look at some key concepts like streams, file pointers, different types of files, operations and more.
Need For File Handling In C
File handling is crucial for system-level development, application programming, and embedded systems. Here are some key reasons why file handling is essential in C programming:
- Persistent Data Storage: Files allow programs to store data persistently on disk, unlike variables and data structures that store data temporarily in memory (RAM) and exist only during program execution. File handling in C allows you to interact with the data. This is essential for cases like storing user preferences, configurations, logging events, maintaining records, etc.
- Data Sharing and Interchange: Files enable the transfer and sharing of data between systems and applications, making them vital for tasks like importing/exporting data or backing up information.
- Handling Large Data Sets: RAM is limited, and holding massive datasets in memory isn’t practical. File handling in C allows a program to work with large amounts of data by reading and writing small portions of it as needed, making memory management more efficient.
- Logging and Debugging: File handling in C programming allows you to maintain logs that track an application’s behavior. This is crucial for storing important events, errors, status updates, and records for future review and writing program traces for analysis.
- Data Security and Recovery: Files can be backed up for safety, ensuring data integrity and recovery in case of system failures.
- Batch Processing: File handling in C allows for batch processing, where programs can process large volumes of data stored in files over time. This helps automate tasks, process historical data, and generate reports from large datasets in files.
- Inter-process Communication: Files can act as intermediaries between processes or programs, allowing them to communicate by reading and writing to shared files. This is handy when more direct communication methods, like sockets or shared memory, aren’t available.
All in all, file handling is essential for efficiently managing data in real-world applications.
Types Of Files In C
In programming, we can classify files into two simplest types: text files and binary files.
1. Text Files
As the name suggests, text files store data in a human-readable format– written text. You can think of them as the regular files you open with a simple text editor. Each line of text is separated by a newline character, making them easy to edit manually.
In other words, text files represent textual information, with files created and manipulated using functions like fprintf(), fscanf(), fgets(), and fputs().
Example:
FILE *filePointer;
filePointer = fopen("example.txt", "w"); // Open file for writing
fprintf(filePointer, "Hello, World!\n"); // Write text
fclose(filePointer); // Close the file
Common uses of text files are:
- Storing Configuration Data: Text files are often used to store program settings, user preferences, and system configurations. Their human-readable nature makes them easy to edit and update.
- Log Files: Programs frequently use text files to record log and error messages during execution, providing a clear record of events.
2. Binary files
Binary files store data in its raw form which is not in human-readable form. Meaning, humans cannot read it without a program to interpret the data. We use functions like fwrite() and fread() to create and manipulate binary files.
Example:
FILE *filePointer;
filePointer = fopen("example.bin", "wb"); // Open file for writing in binary mode
int numbers[] = {1, 2, 3};
fwrite(numbers, sizeof(int), 3, filePointer); // Write binary data
fclose(filePointer); // Close the file
Common uses of binary files are:
- Storing Complex Data: Binary files are commonly used to store structured data like arrays, structures, or objects, ensuring data integrity and more efficient storage and retrieval.
- Storing Multimedia Content: We also use binary files to store images, audio, video, and other multimedia content to retain their exact form and prevent corruption.
File Pointer For File Handling In C
A file pointer is a special pointer variable that holds the reference to a file. In other words, it points to the current position in a file. The file pointer allows you to work with files, enabling your program to read from, write to, or modify the file. Without a file pointer, it would be difficult to perform file operations like opening, reading, or closing a file.
Syntax Of File Pointer:
FILE *filePointer;
Here,
- FILE refers to a predefined structure in the C standard library that stores information about the file being used, such as its current position, end-of-file status, etc.
- The term filePointer is the name/ identifier of the pointer variable that refers to the file we want to access. While you can give it any name, conventionally, programmers use names like fp, file, or filePtr.
- The asterisk symbol (*) before the pointer name indicates that it is a pointer, here pointing to the structure FILE.
Why Is File Pointer Important?
- It allows random access to a file's contents.
- It enables the program to manage multiple files simultaneously.
- It helps in handling large files efficiently by allowing operations on specific parts of a file rather than loading the entire file into memory.
The file pointer is a critical concept of file handling in C, as it ensures that your program can run smoothly and interact with files effectively.
Operations For File Handling In C
File handling operations are essential for working with files efficiently. Here are the various kinds of file operations that facilitate the process of file handling in C:
- Creating a New File: This operation creates a new file in the file system. If a file with the same name already exists, it can be overwritten, depending on how the file is opened.
- Opening an Existing File: Before you can read or write to a file, you need to open it. This basic operation grants access to a file's content for reading, writing, or both.
- Reading from a File: Reading allows you to retrieve data stored in a file. You can read sequentially from the start or use files to access specific portions.
- Writing to a File: The file writing operations allow you to store data in a file. You can append data to the end of the file or overwrite its existing content, depending on how the file is opened.
- Moving to a Specific Location (File Seeking): It involves moving the file pointer to a particular location within the file, allowing random access for reading or writing specific parts of the file.
- Closing a File: After you're done using a file, closing it is important to free system resources and ensure data integrity.
- Deleting a File: This operation permanently removes a file from the file system, freeing up space and removing its data.
- Renaming or Moving a File: It allows you to change the name of a file or move it to a different location within the file system without altering its content.
- Checking File Existence: This operation may be performed before other operations, to verify if a file exists and avoid errors like trying to open a non-existent file.
- Checking File Attributes and Metadata: With this file operation you can gather information about a file, such as its size, modification date, permissions, and more, for better management and control.
- Locking and Unlocking Files: In multi-process environments, locking prevents other processes from modifying a file while it's in use, ensuring data consistency.
- Setting File Permissions: Adjusting read, write, and execute permissions determines who can access or modify a file, which is essential for security and proper file management.
In the following sections, we will explore the primary types of file operations in detail.
Open A File | Text File Handling In C
File opening is a fundamental concept in C programming and serves as a starting point for interacting with files. It establishes a connection between a program and a file stored on the disk, allowing the program to perform file operations like reading from or writing to the file.
We use the fopen() function to open a file in C programs. This function requires two parameters: the name of the file (and optionally its path) and the mode that specifies how the file will be accessed (read, write, append, etc.). The mode determines the type of file access and whether the file will be created if it doesn't exist.
Syntax For Opening A File In C
FILE *fopen(const char *filename, const char *mode);
Here,
- FILE represents the input FILE stream/ structure we want to open.
- The function arguments– filename is a string containing the identifier/ name or path of the file, and mode is a string that defines the file access mode (e.g., read, write, append).
Return Value:
- If the file is successfully opened, the fopen() function returns a pointer to a FILE object, which we can use to perform file operations.
- If the file cannot be opened (due to reasons like an incorrect file path or permission issues), fopen() returns NULL (Null pointer).
Modes Of Opening A File | Text File Handling In C
The table below lists and describes various file access modes we use with the open() function.
File Access Mode | Description | If The File Does Not Exist |
---|---|---|
"r" | Open for reading. The file must already exist. | Returns NULL. |
"w" | Open for writing. Overwrites if file exists or creates a new one if not. | Creates a new file. |
"a" | Open for appending. Adds data to the end of the file. | Creates a new file. |
"r+" | Open for reading and writing. The file must exist. | Returns NULL. |
"w+" | Open for reading and writing. Overwrites if file exists or creates a new one. | Creates a new file. |
"a+" | Open for reading and appending. Adds data at the end. | Creates a new file. |
We have similar reading modes when dealing with binary files. We will discuss these in a later section.
How To Open A File?
- Include the Necessary Header: Add the <stdio.h> header, which provides functions for file handling in C.
- Declare a File Pointer: Create a pointer of type FILE to manage file operations.
- Open the File: Use the fopen() function to open the file in the specified mode.
- Check if the File Opened Successfully: Ensure the file pointer is not NULL to verify success.
- Perform File Operations: Use appropriate functions like fread(), fwrite(), or fprintf() to interact with the file.
Example File Handling Program In C: Opening A File
The opening operation is an integral part of the concept of file handling in C. Look at a basic C program example below to know how this works with various file opening modes.
Code Example:
#include <stdio.h>
int main() {
FILE *file;
file = fopen("example.txt", "r"); // Opening a file for reading
if (file == NULL) {
perror("Error opening file for reading");
return 1;
}
printf("File opened successfully for reading\n");
fclose(file);
file = fopen("example.txt", "w"); // Opening a file for writing
if (file == NULL) {
perror("Error opening file for writing");
return 1;
}
printf("File opened successfully for writing\n");
fclose(file);
file = fopen("example.txt", "a"); // Opening a file for appending
if (file == NULL) {
perror("Error opening file for appending");
return 1;
}
printf("File opened successfully for appending\n");
fclose(file);
file = fopen("example.txt", "r+"); // Opening a file for reading and writing
if (file == NULL) {
perror("Error opening file for reading and writing");
return 1;
}
printf("File opened successfully for reading and writing\n");
fclose(file);
return 0;
}
Output:
File opened successfully for reading
File opened successfully for writing
File opened successfully for appending
File opened successfully for reading and writing
(This is the output you will get, assuming such a file already exists.)
Explanation:
In the basic C code example, we include the header file <stdio.h>, essential for input-output operations.
- We then define the main() function, which is the entry point for the program's execution.
- In main(), we first declare a pointer variable file of type FILE to refer to the file we want to access. We will use it to perform file-handling operations.
- Next, we use the pointer to perform a series of operations as a part of the file handling process.
- We use the function fopen() with the mode "r" to open a file named example.txt (Open the file for reading):
- After that, we use an if statement to check if the file pointer is NULL to verify whether the file was opened successfully.
- If the condition is true, it means the file could be opened. The if-block is executed, where we use the perror() function to print an error message and the program terminates with a return of 1 (error status).
- If the condition is false, it means the file was opened successfully. We skip the if-block and move to the next section of code.
- Then, we use the printf() function to display a success message and use the fclose()function to close the file and release the resources. (Note that this step is crucial, and we must repeat it every time we open a file.)
- Then, we use the fopen() function with the mode "w" to open the same file again (Open the file for writing).
- If the file example.txt does exist, this mode will cause the program to overwrite the existing content or create a new file if it doesn't exist.
- Then, we use an if-statement to verify if the file opened successfully. In case of failure, we print an error message and return 1. And in case of success, we print a success message and close the file using fclose().
- Similarly, we open the file in append mode "a", which allows us to add new data at the end of the file without altering its current content.
- We use the same error-checking method with the if statement and print success or error messages accordingly. After that, we close the file to free up the resources.
- Lastly, we open the file in the read/write mode "r+", which allows both reading from and writing to the file. We use the same logic for error checking and close the file after printing the error/ success messages.
- Finally, the program returns 0, indicating successful execution.
Note: As shown in the output, we have printed various success messages. But this is assuming that such a file does exist. If the file does not exist, and you want to run the code for experimentation, shift the lines of code for the write file opening mode "w" before the read file opening mode "r".
Create A File | Text File Handling In C
Creating a file in C involves opening it in write mode using the fopen() function. If the file doesn’t exist, it will be created; if it already exists, the content will be overwritten. The steps involved in creating a file are given below.
Steps To Create A File In C
- Include the Necessary Header: The first step is to include the essential header file <stdio.h>, which contains file handling functions like fopen() and fclose().
- Declare a File Pointer: Next, you must declare a pointer of type FILE, which is essential for managing file operations.
- Open the File: Use fopen() to open the file in the desired mode, i.e., "w" for write mode. Note that this mode creates a new file if the file doesn’t exist.
- Check if the File is Opened Successfully: You must always check if the file pointer is not NULL to ensure the file was opened correctly.
- Perform File Operations: If the file is opened successfully, you can then use functions like fprintf(), fputs(), or fwrite() to write data to the file.
- Close the File: After completing your file operations, it is important to close the file and release system resources. You can do this using the fclose() function.
Syntax For Creating A File In C
FILE *fopen(const char *filename, const char *mode);
int fclose(FILE *stream);
Here–
- FILE refers to the data type/ structure representing a file stream.
- The fopen() takes two arguments: filename, a string specifying the name we want to give to the file we are creating, and mode, which refers to the access mode we want to use to open the file.
- The fclose() is a library function to close the file with the argument stream is the name of the the pointer of type FILE, indicated by the asterisk symbol (*).
This is the syntax we use to create a file, a concept of file handling in C. Below is a simple C program example illustrating the process.
Code Example:
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *file;
file = fopen("example.txt", "w"); // Open a file for writing
if (file == NULL) {
perror("Error opening file for writing");
return EXIT_FAILURE;
}
// Write some data to the file
fprintf(file, "Hello, World!\n");
fprintf(file, "This is a test file.\n");
fclose(file); // Closing the file
// Open the file again to read and display the content (for verification)
file = fopen("example.txt", "r");
if (file == NULL) {
perror("Error opening file for reading");
return EXIT_FAILURE;
}
// Read and display the content
char buffer[100];
while (fgets(buffer, sizeof(buffer), file) != NULL) {
printf("%s", buffer);
}
// Close the file
fclose(file);
return EXIT_SUCCESS;
}
Output:
Hello, World!
This is a test file.
Code Explanation:
In the simple C code example, we first include the header files <stdio.h> for input/output operations and <stdlib.h> for memory allocation and exit handling.
- In the main() function, we declare a pointer variable, file, which points to the FILE structure, representing the file we’ll work with.
- We then use the fopen() function to open a file, with the file name, example.txt and the access mode "w". This mode either creates a new file or truncates (overwrites) an existing one.
- The open() function returns a pointer, which we assign to the file variable.
- Then, we use an if statement to see if the file pointer is NULL (i.e., file == NULL) to verify if the file was opened successfully.
- If the condition is true, it means the file can't be opened. Then the if-block is executed, printing an error message using the perror() function and the program exits with a failure status (EXIT_FAILURE).
- If the condition is false (i.e., the file was successfully opened), we skip the if block and proceed with the code.
- Next, we use the fprintf() function to write two lines of text to the file– "Hello, World!\n" and "This is a test file.\n". Here, the newline escape sequence (\n) ensures each line is printed in a new line.
- Once done writing, we close the file using the fclose() function to ensure that the data is properly saved and release system resources.
- After closing, we re-open the same file in read mode ("r") using fopen(). Again, we check if the file was opened successfully using an if statement.
- If the file can't be opened (statement condition is true), the program prints an error and exits. If the condition is false, it means we can open the file and we move to the next section of the code.
- We declare an array of characters, buffer, to temporarily store data read from the file. In this case, buffer[100] indicates that the buffer array can hold up to 100 characters at a time.
- Then, we use a while loop to read the content of the file one line at a time and print it to the console. Inside:
- We use the fgets() function to read a line from the file and store it in the buffer array. Here, the sizeof() operator ensures that we don't read more than 100 characters in a single iteration.
- The loop condition then checks if the content read into the buffer is not NULL (using the inequality relational operator).
- If the condition is true, the printf() function inside displays the buffer string. Here, the %s format specifier indicates that characters will be printed in string format.
- The loop continues iteration, reading lines until the condition becomes false, i.e., fgets() returns NULL. This means all the lines are read, and the loop terminates.
- After the loop control statement prints the content, we close the file using fclose() again to release resources.
- Finally, the main() function returns a success status (EXIT_SUCCESS) to indicate that the program executed successfully.
Check out this amazing course to take your C programming skills to the next level.
Read & Write | Text File Handling In C
The primary purpose of text file handling in C is to allow you to read from and write to text files. The process begins by opening the file in the appropriate mode, performing the read/write operations, and closing the file afterwards.
Multiple library functions allow us to perform read and write operations as part of file handling in C language. They allow you to work with single characters, strings, or formatted data.
File Handling Functions In C | Read & Write Text Files
The table below summarizes key functions for text file handling in C, including functions to read/write single characters, multiple characters, and in-stream.
Function Name |
Description |
Syntax |
Parameters |
fgetc() |
Reads a single character |
int fgetc(FILE *stream); |
stream – The file stream to read from. |
fputc() |
Writes a single character |
int fputc(int character, FILE *stream); |
character – The character to write. stream – The file stream to write to. |
fgets() |
Reads a line of text |
char *fgets(char *str, int num, FILE *stream); |
str – Buffer to store the line. |
fputs() |
Writes a string |
int fputs(const char *str, FILE *stream); |
str – The string to write. |
fprintf() |
Writes formatted text |
int fprintf(FILE *stream, const char *format, ...); |
stream – The file stream to write to. format – Format specifier for data. |
fscanf() |
Reads formatted text |
int fscanf(FILE *stream, const char *format, ...); |
stream – The file stream to read from. format – Format specifier for data. |
rewind() |
Resets file position indicator |
void rewind(FILE *stream); |
stream – The file stream to reset. |
feof() |
Checks end-of-file indicator |
int feof(FILE *stream); |
stream – The file stream to check. |
Now that we know about the various functions that make the process of file handling in C possible, let's look at a few examples.
File Handling In C Example: Write To A Text File
In the C program example below, we will know how to use the fputc(), fputs(), and fprintf() functions to write to a file. These are three of the most commonly used functions for the read file handling in C operation.
Code Example:
#include <stdio.h>
int main() {
FILE *filePtr;
// Open a file for writing
filePtr = fopen("example.txt", "w");
if (filePtr == NULL) {
printf("Error opening file.");
return 1;
}
// Write data to the file
fputc('H', filePtr);
fputc('e', filePtr);
fputc('l', filePtr);
fputc('l', filePtr);
fputc('o', filePtr);
fputs(", World!\n", filePtr);
fprintf(filePtr, "This is a test.");
// Close the file
fclose(filePtr);
printf("Data written to file successfully.");
return 0;
}
Output:
Data written to file successfully.
Explanation:
In the C code example,
- In the main() function, we first declare a file pointer filePtr to handle file operations.
- Then, we open a file named example.txt using the fopen() function with the mode "w" for writing.
- Next, we use an if-statement to see if the file opened successfully, printing an error message if it failed and skipping the if-block to the next line of code if it is successful.
- We then use the file pointer filePtr with the fputc() function to write characters to the file, one character at a time. Here, we write characters 'H', 'e', 'l', 'l', 'o', to the file.
- After that, we use the fputs() function to write a string of characters to the file, i.e., ", World!" and add a new line character to shift the cursor to the next line.
- Next, we use the fprintf() function to write the formatted string– "This is a test." to the file.
- Following this, we use the fclose() function to close the file after writing to ensure the data is saved.
- We then print a success message to the console using the printf() function to indicate that the data has been written to the file successfully.
After the program is executed, the file content will be as given below.
Hello, World!
This is a test.
File Handling In C Example: Read From A Text File
The example C program below illustrates the use of the fgets(), fgetc(), fscanf(), and rewind() functions to perform the read file handling in C operation.
Code Example:
#include <stdio.h>
int main() {
FILE *filePtr;
char buffer[100];
char ch;
int num;
// Open a file for reading
filePtr = fopen("example.txt", "r");
if (filePtr == NULL) {
printf("Error opening file.");
return 1;
}
// Method 1: Using fgets to read a line of text
fgets(buffer, sizeof(buffer), filePtr);
printf("Data read using fgets: %s\n", buffer);
// Method 2: Using fgetc to read individual characters
printf("Data read using fgetc: ");
while ((ch = fgetc(filePtr)) != EOF) {
putchar(ch); // Print each character read
}
printf("\n");
// Reset file position for fscanf
rewind(filePtr);
// Method 3: Using fscanf to read formatted data
fscanf(filePtr, "%s %d", buffer, &num);
printf("Data read using fscanf: %s %d\n", buffer, num);
// Close the file
fclose(filePtr);
return 0;
}
Output:
Data read using fgets: Hello, World!
This is a test.
Data read using fgetc: This is a test. (or the content of file printed char by char)
Data read using fscanf: Hello 123 (Assuming the file has such formatted content)
Explanation:
In the example C code,
- Inside the main() function, we declare a file pointer, filePtr, for file handling operations, and a character array, buffer, to store the data read from the file with a size of 100 characters.
- We also declare two variables: ch (character type) to store individual characters read from the file (character by character) and num (integer data type) to store an integer value when reading formatted data from the file.
- Next, we use the fopen() function to open the file example.txt in read mode "r". We check if the file was opened successfully using an if statement and print an error message if it is not.
- As mentioned in the code comments, we use the first method, fgets(), to read a string/ line of text from the file, with filePtr referring to the respective file stream. Inside:
- We use the sizeof() operator to ensure the function doesn't read beyond the buffer size. It reads size(buffer) -1 characters or until a newline (\n) or end-of-file (EOF) is encountered, whichever comes first.
- We store the result in the buffer array and add a null terminator (\0) to the end of the string.
- Then, we use the printf() function to print the data that was read from the file and stored in the buffer.
- Next, we use the fgetc() function inside a while loop to read content from the file, one character at a time. Here:
- In every iteration, the fgetc() function reads a single character from the file, which is then assigned to ch.
- If the end of the file isn't reached, the loop prints the character using the putchar() function.
- The loop continues iterations until the end-of-file (EOF) is reached.
- When this happens, the loop returns EOF (an integer constant defined as -1), the condition becomes false, and the loop terminates.
- Since the loop reaches the end of the line, the pointer's position must be adjusted before using fscanf(). For this, we use the rewind() function, which resets the file position indicator to the beginning of the file.
- Then, we fscanf() function to read formatted input from the file. The %s specifier indicates the function to read a string (up to the first whitespace) and is stored in buffer.
- The %d specifier tells the function to read an integer and store in variable num using the address-of operator (&). Then, we use printf() to display the content read to the console.
- Lastly, we use the fclose () function to close the file after reading to ensure resources are released.
Note:
- After fgets() has read part of the file, fgetc() starts from where fgets() left off. Since the whole file is not read here, we do not have to use rewind() at this point.
- For this example to work properly, the file content needs to have a string followed by an integer (e.g., Hello 123). If the file content differs, the format specifiers used in fscanf() should be adjusted accordingly.
Hone your coding skills with the 100-Day Coding Sprint at Unstop and claim bragging rights now!
Close A File | Text File Handling In C
Closing of files is an important concept of file handling in C. It ensures that all resources associated with the file are properly released and any pending data in the file buffer is flushed (i.e., written to the file). Failing to close a file may lead to resource/ memory leaks and corrupted data, especially if buffered data isn't written correctly.
The function used to close a file is fclose(). After closing of files using this function, further operations on that file stream (like reading or writing) are not allowed, and any attempt to do so will result in an error.
Syntax Of fclose() Function
int fclose(FILE *stream);
Here, the *stream refers to the pointer variable of type FILE, pointing to the file stream that needs to be closed.
Return Value:
- On success, fclose() returns 0.
- On failure (e.g., invalid file pointer), it returns EOF (End of File), typically represented by -1.
Now that you know the basic syntax of fclose(), look at the C program sample below to learn about its implementation.
Code Example:
#include <stdio.h>
int main() {
FILE *filePtr;
char data[50] = "Hello, World!";
// Open a file for writing
filePtr = fopen("example.txt", "w");
if (filePtr == NULL) {
printf("Error opening file.");
return 1;
}
// Write data to the file
fprintf(filePtr, "%s", data);
// Close the file
if (fclose(filePtr) == 0) {
printf("File closed successfully.");
} else {
printf("Error closing file.");
}
return 0;
}
Output:
File closed successfully.
Explanation:
In the C code sample,
- We declare a file pointer filePtr to handle file operations inside the main() function.
- Then, we initialize a character array data of size 50, with the string value– "Hello, World!".
- Next, we use the fopen() function with "w" mode to open (or create, if it doesn't exist) a file named example.txt.
- Using an if conditional statement, we verify if the file was opened successfully. If the file fails to open, the program prints an error message and exits.
- We then use the fprintf() function to write the content of the data array to the file.
- After that, we use the fclose() function to close the file inside an if-else statement to verify if it was closed successfully.
- If the statement condition is true, i.e., the file pointer after fclose() is NULL, the if-block is executed, printing the success message to the console.
- If the condition is false, the else block is executed and prints an error message.
Note: After calling fclose() function, no more operations can be performed on filePtr. It is a good practice to always close files after reading or writing to avoid memory or file access issues.
Binary File Handling In C (Open, Read & Write)
As you must know, binary files allow you to store and manipulate non-text data, such as images, audio, video, or binary data, like arrays and structures. The binary file handling in C is similar to that of text files, but the key difference is how data is stored and accessed—binary files store data in a raw, byte-by-byte format, making them more efficient for storing complex data types.
Let's look at the main operations and functions used in binary file handling in C language:
Open Operation | Binary File Handling In C
To open a binary file, we use the same fopen() function, but for the file access modes, you must specify that it is a binary mode. This is done by including a "b" after the initial character, like "rb" for reading binary data, "wb" for writing binary data, etc.
Just like in the case of text files, the fopen() function returns a pointer to a FILE structure, which is used for all subsequent file operations.
Syntax For Opening Binary File:
FILE *fopen(const char *filename, const char *mode);
The function's syntax is the same, as mentioned above. The table below lists the various binary modes used when handling binary files in C language.
Binary Modes | Description | If File Does Not Exist |
---|---|---|
"rb" | Open a binary file for reading. | Returns NULL. |
"wb" | Open a binary file for writing. | Creates a new file. |
"ab" | Open a binary file for appending. | Creates a new file. |
"rb+" | Open a binary file for reading and writing. | Returns NULL. |
"wb+" | Open a binary file for reading and writing. Overwrites the content if the file exists. | Creates a new file. |
"ab+" | Open a binary file to read and append. | Creates a new file. |
Read Operation | Binary File Handling In C
We commonly use the fread() function to read binary data. It reads raw data from the file and stores it in a block of memory, interpreting it based on the size and number of elements specified.
Syntax For Reading From A Binary File:
size_t fread(void *ptr, size_t size, size_t count, FILE *stream);
Here,
- The size_t is an unsigned integer type that represents the size of any object in memory, size refers to the size of each element to be read, and count indicates the number of elements to be read.
- The *ptr is the void pointer referring to block of memory where the data read will be stored and *stream is the file pointer from which to read data.
The function returns the total number of elements successfully read.
Write Operation | Binary File Handling In C
The fwrite() function writes binary data from a block of memory to the file. Like fread(), it operates on raw bytes, making it ideal for writing arrays, structures, or other data types.
Syntax For Writing To A Binary File:
size_t fwrite(const void *ptr, size_t size, size_t count, FILE *stream);
Here, the parameters are the same as in read operation, and the function returns the total number of elements successfully written.
Close Operation | Binary File Handling In C
After performing read or write operations on a file, it is important to close the file using the fclose() function. This releases the resources associated with the file and ensures that any buffered data is properly saved.
Syntax For Closing A Binary File:
int fclose(FILE *stream);
The *stream is the pointer to the FILE structure we want to close. The function returns 0 if the file is closed successfully or EOF (end of file) if an error occurs.
Binary File Handling In C Example: Opening A Binary File To Write Data
The sample C program below illustrates how to open a binary file and write an array of integers into it.
Code Example:
#include <stdio.h>
int main() {
FILE *file;
int data[5] = {10, 20, 30, 40, 50};
// Open a binary file for writing
file = fopen("data.bin", "wb");
if (file == NULL) {
perror("Error opening file");
return 1;
}
// Write an array of integers to the file
size_t elements_written = fwrite(data, sizeof(int), 5, file);
// Close the file
fclose(file);
if (elements_written == 5) {
printf("Data was written to the file.\n");
} else {
printf("Error writing data to the file.\n");
}
return 0;
}
Output:
Data was written to the file.
Explanation:
In the C code sample,
- We first declare a file pointer file to handle file operations.
- Then, we declare an array of integers data (of size 5) and assign the value– {10, 20, 30, 40, 50} to it.
- Next, we use the fopen() function with binary mode "wb" to open a file data.bin for writing. (Here, the file extension .bin represents a binary file, as against file extension .txt for text files.)
- We then use an if-statement to verify if the file opened successfully. If it did, we move to the next line in code; other we print an error message and exit.
- After that, we use the fwrite() function to write the content from data array to the data.bin file. The function returns the number of elements successfully written to the file, which we store in the variable elements_written.
- Then, we use the fclose() function to close the file after writing. This ensures that the data is saved and the file is no longer in use.
- We check if the number of elements written to the file is equal to 5 (the size of the data array). If it is, we print a success message; otherwise, we print an error message indicating an issue with writing the data.
Binary File Handling In C Example: Opening A Binary File To Read Data
In the C example below we will see how to use the fopen() function to open a file and fread() function to read its contents.
Code Example:
#include <stdio.h>
int main() {
FILE *file;
int data[5];
// Open a binary file for reading
file = fopen("data.bin", "rb");
if (file == NULL) {
perror("Error opening file");
return 1;
}
// Read an array of integers from the file
size_t elements_read = fread(data, sizeof(int), 5, file);
// Close the file
fclose(file);
// Display the read data
if (elements_read == 5) {
printf("Read data: ");
for (int i = 0; i < 5; i++) {
printf("%d ", data[i]);
}
printf("\n");
} else {
printf("Error reading data from the file.\n");
}
return 0;
}
Output:
Read data: 10 20 30 40 50
Explanation:
In the example code,
- We declare a file pointer file to handle file operations and an array of integers data (of size 5) to store data read from the file.
- Then, we open a binary file, data.bin, using fopen() with the mode "rb" to read the file. We verify if the file opened successfully. If it did, we skip the if-block; otherwise, we print an error message.
- Next, we use the fread() function to read 5 integers from the file into the data array. The function returns the number of elements read, stored in the variable element_read.
- After reading, we close the file using fclose() to ensure the resources are released.
- We check if the number of elements read from the file is equal to 5. If it is, the for loop in the if-block prints the data to the console. Otherwise, an error message is displayed.
File Handling In C With fseek()
We use the fseek() function to move the file position indicator (often referred to as the file cursor) to a specific byte position within the file stream. This is useful when you need to navigate through a file and read or write data from or to specific locations.
Syntax:
int fseek(FILE *stream, long int offset, int origin);
Here,
- The parameter *stream is the pointer to the file object representing the file you're working with and offset is the number of bytes (+ve or -ve) by which to shift the file position indicator.
- The origin parameter specifies the reference point for the offset. It can be one of the following constants:
SEEK_SET (0): Sets the position relative to the beginning of the file.
SEEK_CUR (1): Sets the position relative to the current file position.
SEEK_END (2): Sets the position relative to the end of the file.
Code Example:
#include <stdio.h>
int main() {
FILE *filePtr;
char data[50] = "Hello, World!";
char buffer[50];
// Open a file for writing
filePtr = fopen("example.txt", "w+");
if (filePtr == NULL) {
printf("Error opening file.");
return 1;
}
// Writing data to the file
fprintf(filePtr, "%s", data);
// Move the file position indicator to the beginning
fseek(filePtr, 0, SEEK_SET);
// Reading data from the file
fgets(buffer, sizeof(buffer), filePtr);
printf("Data read from file: %s", buffer);
// Closing the file
fclose(filePtr);
return 0;
}
Output:
Data read from file: Hello, World!
Explanation:
In the example code,
- In the main() function, we declare a file pointer filePtr to handle file operations and initialize a character array data with the string "Hello, World!".
- We then declare a character array buffer (of size 50) to store data read from the file.
- Then, we open a file named example.txt for both reading and writing using the fopen() function with the mode "w+". We check if the file opened; if it does we skip to after the if-block; other we print an error message and return an error code.
- Next, we use the fprintf() function to write the contents of the data array to the file.
- After that, we use the fseek() function to move the file cursor back to the start of the file (using SEEK_SET parameter) after writing data to allow reading from the beginning.
- We then read the data from the file using fgets(), print it to the console and close the file using fclose() to free resources.
File Handling In C With rewind()
We use the rewind() function to reset the file position indicator to the beginning of the file. Unlike fseek(), which allows more flexibility, rewind() always moves the file cursor to the start. It's useful when you need to reread or overwrite data starting from the file's beginning.
Syntax:
void rewind(FILE *stream);
Here, *stream is the pointer to a file stream that specifies the file whose position indicator needs to be reset.
Code Example:
#include <stdio.h>
int main() {
FILE *filePtr;
char data[50] = "Hello, World!";
char buffer[50];
// Open a file for writing
filePtr = fopen("example.txt", "w+");
if (filePtr == NULL) {
printf("Error opening file.");
return 1;
}
// Writing data to the file
fprintf(filePtr, "%s", data);
// Reset file position indicator to the beginning
rewind(filePtr);
// Reading data from the file
fgets(buffer, sizeof(buffer), filePtr);
printf("Data read from file: %s\n", buffer);
// Closing the file
fclose(filePtr);
return 0;
}
Output:
Data read from file: Hello, World!
Explanation:
Just like in the previous example, we have a file pointer filePtr, a character array data (size 50) initialied with string–"Hello, World!" and another array buffer (size 50).
We open a file example.txt for reading and writing and check for verification, printing an error message if we fail. Then, we write the content of data array to the file using the fprintf() function.
- After that, we reset the file position indicator to the beginning of the file using the rewind() function to prepare for reading.
- Next, we use fgets() to read the data, store it in buffer and print the same to the console.
- We close the file after reading and writing operations are complete, using fclose().
List Of All Functions For File Handling In C
In the table below, we have listed the most important and commonly used functions for file handling in C.
Function Name | Description |
---|---|
fopen() | Opens a file with various reading and wrirting modes. |
fclose() | Closes a file |
fgetc()/ getc() | Reads a single character |
fputc()/ putc() | Writes a single character |
fgets() | Reads a line of text (string) |
fputs() | Writes a string (line of text) |
fprintf() | Writes formatted text |
fscanf() | Reads formatted text |
fread() | Reads a specified number of bytes from a file (binary form) |
fwrite() | Writes a specified number of bytes to a file (binary form) |
fseek() | Sets the file position indicator to a specific location |
rewind() | Resets file position indicator to the beginning |
feof() | Checks end-of-file indicator |
perror() | Prints a descriptive error message |
ferror() | Checks if an error has occurred on the file |
ftell() | Returns the current file position indicator's offset |
rename() | Renames or moves a file to a new location |
fflush() | Flushes the output buffer |
remove() | Deletes a specified file from the file system |
putw() | Writes an integer to a binary file |
getw() | Reads an integer from a binary file |
fsetpos() | Sets the file position indicator using a position structure |
fgetpos() | Gets the current file position |
The Difference Between Text & Binary Files
Text and binary files are two fundamental types of files. The table below highlights the key differences between the two.
Aspect | Text Files | Binary Files |
---|---|---|
Data Representation | Data is stored in human-readable text format. | Data is stored in binary format, not human-readable. |
End-of-Line Character | End-of-line is typically represented by \n or \r\n. | No specific end-of-line character. |
File Size | Larger due to additional formatting characters (e.g., newline) | Smaller as data is stored in raw binary form. |
Reading and Writing | Uses standard library functions like fscanf, fprintf, fgets, fputs. | Uses fread and fwrite for handling binary data. |
Portability | Portable across different platforms due to text encoding. | Less portable, as binary format may vary between systems. |
Performance | Slower for large data due to parsing overhead. | Faster for large data due to direct binary manipulation. |
Use Cases | Configuration files, logs, source code. | Executable files, images, multimedia files, serialized data. |
Error Handling | Easier to spot errors manually due to readability. | Harder to debug as data is not human-readable. |
Looking for mentors? Browse from the list of select experienced coding & software experts here.
Conclusion
File handling in C is a fundamental aspect that enables developers to interact with external files for reading and writing data. The C library provide many function for managing files efficiently, including fopen(), fwrite(), fread(), fprintf(), and fclose().
A solid understanding of file handling is essential for a wide range of applications, be it reading configuration files, logging data, or managing binary data streams. By leveraging the capabilities of file handling in C, developers can design applications that interact seamlessly with external data sources, facilitating data storage, retrieval, and manipulation with precision and efficiency. However, it is vital to approach file operations with care, ensuring proper error checking, resource management, and maintenance of data integrity.
Also Read: 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. Where is file handling used?
The various areas/ reasons for the need for file handling in C language are:
- Data Storage: Storing information in text documents, databases, and configuration files.
- Programming: Reading and writing files for software applications, including logging.
- Database Systems: Managing transaction logs and indexes.
- Web Development: Serving web resources like HTML pages and images.
- Data Import/Export: Importing data from external files/ formats like CSV and exporting for reporting.
Q. What are the five primary functions for file handling in C?
The five fundamental file handling functions in C are:
- fopen(): Opens a file with a specified mode for reading, writing, appending, etc. (like text modes– r, w, a and binary modes – rb, wb).
- fclose(): Closes an open file, saves changes properly, and releases resources.
- fread(): Reads data from a file into a buffer.
- fwrite(): Writes data from a buffer to a file.
- fseek(): Moves the file position indicator to a specified location.
These functions are central to file handling in C and enable operations like opening, reading, writing, and navigating within files.
Q. What is the significance of closing a file in file handing in C?
Closing a file is an integral stage in the process of file handling in C, because:
- Releases system resources tied to the file, preventing resource exhaustion.
- Flushes any buffered data to ensure data integrity.
- Improves system performance by freeing resources for other processes. Neglecting to close files can lead to resource leaks and potential file corruption.
Q. What are the different types of files classified for file handling?
Files can be categorized into different file versions based on their content and format. These include:
- Text Files: Contain human-readable, textual information. They are encoded using character encoding standards such as ASCII, UTF-8, or others. Examples include file extensions– .txt, .html, and .csv files.
- Binary Files: Store data in a non-human-readable format that preserves the exact bit-level representation of values. Examples include image files (.jpg, .png), executable files (.exe), and database files (.sqlite, .mdb).
- Source Files/ Source Code Files: Contain program source code written in programming languages like C, C++, Java, or Python. They are text files in a specific format and structure for computer interpretation. Examples include .c, .cpp, .java, and .py files.
- Configuration Files: Store application settings and parameters that control the behavior of software applications. Examples include .ini, .xml, and .json files.
- Log Files: Record events and activities essential for debugging and monitoring. Examples include .log and .txt files.
Q. What is "a+" in file handling in C?
When opening a file to perform various operations, we need to specify the access mode in which we want to use the file. The "a+" is an access mode specification, which, when used, opens the file in append mode, i.e., opens a file for both reading and appending. It means:
- "a": Appends data to the end of the file. If the file doesn't exist, it is created.
- "+": Allows both reading and writing operations.
This mode is useful when you need to read existing data from a file and then append new data to it without overwriting the existing file content.
Q. How to delete a file in C?
You can delete a file using the remove() function, which is provided by the <stdio.h> header.
Code Example:
#include <stdio.h>
int main() {
char filename[] = "example.txt";
if (remove(filename) == 0) {
printf("File %s deleted successfully.\n", filename);
} else {
printf("Error deleting file %s.\n", filename);
}
return 0;
}
Output:
File example.txt deleted successfully.
Explanation:
In this code we attempt to delete "example.txt". If successful, it prints a success message; otherwise, it prints an error. (The output is achieved assuming such a file exists to begin with. if the file does not exist, you will instead get the error message.)
This compiles our discussion on file handling in C. Here are a few other topics you must explore:
- Typedef In C | Syntax & Use With All Data Types (+ Code Examples)
- Recursion In C | Components, Working, Types & More (+Examples)
- Union In C | Declare, Initialize, Access Member & More (Examples)
- Compilation In C | Detail Explanation Using Diagrams & Examples
- Bitwise Operators In C Programming Explained With Code Examples
- Type Casting In C | Cast Functions, Types & More (+Code Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment