Table of content:
- Text Segment Of Memory Layout In C
- Initialized Data Segment Of Memory Layout In C
- Uninitialized Data Segment Of Memory Layout In C
- Stack Segment Of Memory Layout In C
- Heap Segment Of Memory Layout In C
- Command-line Arguments Segment Of Memory Layout In C
- Dynamic Memory Layout Of C Program
- Static Memory Layout Of C Program
- Check the Size of Code, Data, and BSS Segments
- Example Of Memory Layout In C
- Conclusion
- Frequently Asked Questions
Memory Layout In C | A Complete Guide With Detailed Code Examples
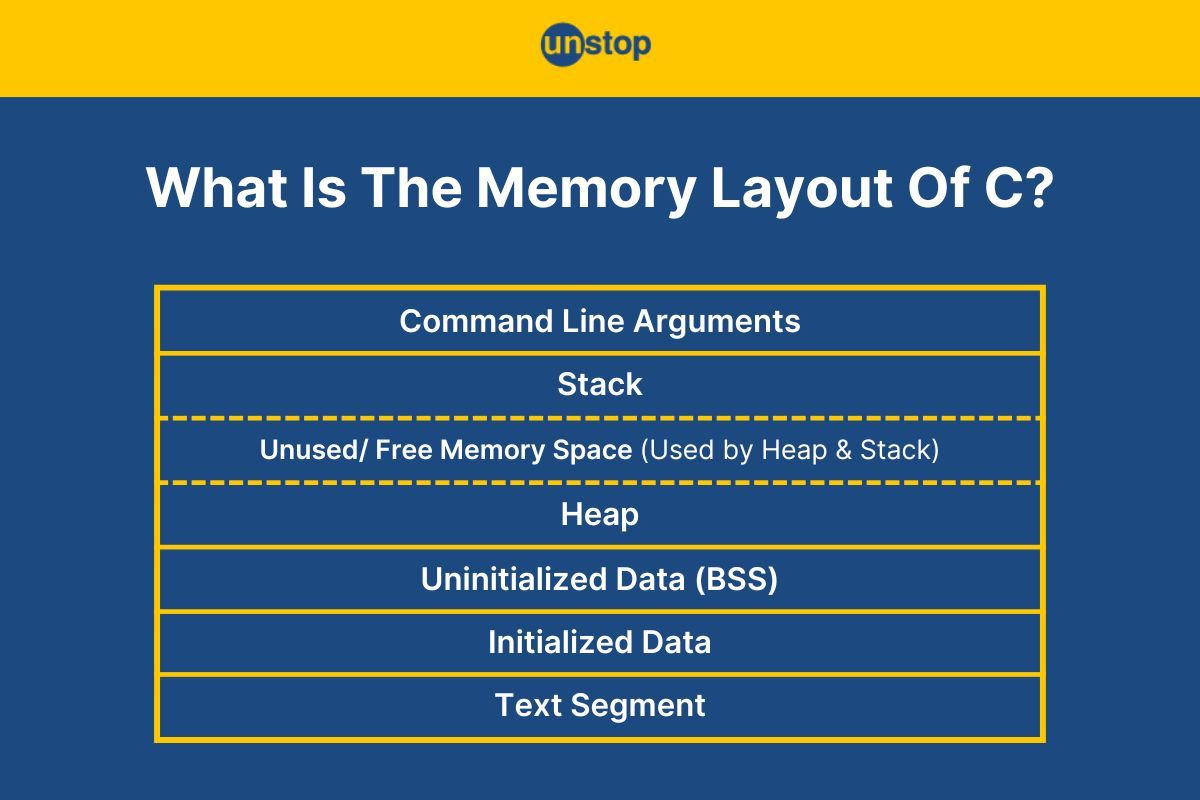
Have you ever wondered how your computer keeps track of the information it processes? The answer lies in the memory layout of C programs. It is like an organizational chart for your code, determining how data is stored and accessed. In this article, we’ll explore the memory layout in C programming in detail.
Understanding this layout/ structure is crucial for writing efficient C programs. Imagine your computer's memory as a series of neatly labelled storage boxes, each holding specific pieces of information. The memory layout acts as a roadmap, guiding where each box is located and how your program retrieves and uses the data inside.
Diagram For Memory Structure Of C Programs
This diagram illustrates the key components of the memory layout in C programming, which include:
- Command Line Arguments: Located at the top of the memory structure, these are inputs provided by users (in the shell/command line) when the program starts, allowing for customization of the program's behavior.
- Stack: A data structure that manages function calls and local variables, maintaining the execution flow of the program.
- Heap: A dynamically allocated memory space that allows the program to request and release memory during runtime as needed.
- Uninitialized Data Segment (BSS): Reserved for uninitialized global and static variables, providing space for future data storage.
- Initialized Data Segment: Contains global and static variables that are initialized with specific values at the start of the program.
- Text/Code Segment: The section where the executable code resides, guiding the program's execution.
Each segment plays a vital role in how your program functions and manages memory. Now that we know the basics of memory layout in C let’s explore each of these segments in greater detail.
Text Segment Of Memory Layout In C
The Text Segment is a critical part of the memory layout in C, as it contains the actual executable code of your program. Below is a breakdown of the Text Segment, followed by a code example and its corresponding memory layout.
Details of the Text Segment Of Memory Layout in C:
- Function Code: It includes the compiled code for your functions, such as the main() function.
- Constants and Strings: Any constants or string literals used in your program are stored in this segment.
- Read-Only Data: Some compilers store read-only data, like constant global variables, in the Text Segment.
The simple C program example below, followed by the layout, shows how the creation of a function affects the memory layout.
Code Example:
#include <stdio.h>
void greetUser() {
printf("Hello, User!\n");
}
int main() {
greetUser();
return 0;
}
Output:
Hello, User!
Code Explanation:
In the simple C code example,
- We first include the header file <stdio.h> for input/output operations, which tells the compiler about functions like printf().
- Next, we define the function greetUser(), which prints a message to the console using printf(). Memory is allocated for this function’s code during execution, and space is set aside for any local variables it might have (none in this case).
- The program’s execution begins with the main() function. When the program runs, memory is allocated for main(), including space for any local variables.
- Inside main(), we call the greetUser() function, at which point printf() retrieves the string "Hello, User!" from memory and instructs the operating system to display it on the console.
- While calling greetUser(), the return address is pushed onto the stack to ensure control returns to main() after the function finishes execution.
- As the program runs, control flows through various segments of memory: the Text Segment stores the machine code for the functions, while the Stack Segment manages function calls and local variables.
- After greetUser() completes, control returns to main(), and the program exits with a return status of 0, indicating successful execution
Memory Layout In C As Per Above Code:
Segment | Contents |
---|---|
Text Segment | greetUser() Function, printf() Instructions, "Hello, User!" String |
Data Segment | Empty (no global/static variables) |
Heap Segment | Empty (no dynamic memory allocated) |
Stack Segment | Return address of main(), local variables for greetUser() (if any) |
This simplified representation illustrates how the Text Segment stores the machine code for functions and any associated constants or strings. As the program executes, control flows through this segment, ensuring proper execution of the code.
Initialized Data Segment Of Memory Layout In C
The Initialized Data Segment stores global and static variables that are explicitly initialized with values before the program starts. This segment of memory layout in C is crucial for handling variables that need to retain their values throughout the program’s execution. Below are the components/ details of the Initialized Data Segment:
Details of the Initialized Data Segment of Memory Layout in C:
- Global Variables: All global variables that are initialized with explicit values are stored here.
- Static Variables: Similar to global, static variables with explicit initial values are also stored in this segment.
- Constants: This segment may also store constants with predefined values, such as literals or constant global variables.
The basic C program example below, along with the memory layout table afterwards, illustrates what happens in the memory structure when we define variables and use them in the main() function.
Code Example:
#include <stdio.h>
int globalVar = 42; // Initialized global variable
static float staticVar = 3.14; // Initialized static variable
int main() {
printf("Global Variable: %d\n", globalVar);
printf("Static Variable: %.2f\n", staticVar);
return 0;
}
Output:
Global Variable: 42
Static Variable: 3.14
Code Explanation:
In this basic C code example,
- We declare and initialize a global variable globalVar with the value 42 to it. After which we initialize a static variable staticVar with the value 3.14. Both these variables are allocated memory in the Initialized Data Segment.
- Inside the main() function, we use printf() statements to display the values of globalVar and staticVar on the console. Here, the %d and %2f format specifiers indicate integer and floating values, and the newline escape sequence (\n) shifts the cursor to the next line after printing the output.
- When the program is executed, the initialized variables are accessed from the Initialized Data Segment, and the values are printed to the console.
Memory Layout As Per The Code Above:
Segment | Contents |
---|---|
Text Segment | main() function |
Initialized Data Segment | Global Variable (42), Static Variable (3.14) |
Data Segment | Empty (no uninitialized variables) |
Heap Segment | Empty (no dynamic memory allocated) |
Stack Segment | Function call stack for main() |
The table above represents how the flow moves through the Text and Initialized Data segments. The global and static integer variables are stored in the Initialized data segment because we assign them a value at the beginning of the program.
Initialized Read-Only Area
This section of the Initialized Data Segment in memory layout in C language is reserved for variables and constants that are initialized with specific values but marked as read-only. It means that these values cannot be changed during program execution.
Example:
const int MAX_VALUE = 100; // Read-only initialized constant
const char GREETING[] = "Hello, World!"; // Read-only initialized string
Explanation:
In this example, MAX_VALUE and GREETING are constants explicitly initialized with specific values. Since they are in the area marked as read-only, their values cannot be altered during the program's execution.
Initialized Read-Write Area
This area of the Initialized Data Segment stores initialized global and static variables that can be modified during program execution. Unlike the read-only section, variables here are flexible and can be updated as needed.
Example:
int globalCounter = 0; // Initialized global variable (modifiable)
static double staticTemperature = 25.5; // Initialized static variable (modifiable)
Explanation:
In this instance, globalCounter is a global variable initialized with the value of 0, and staticTemperature is a static variable initialized with the value of 25.5. Both can be modified during the program's execution.
Check out this amazing course and grasp all the C programming concepts with ease!
Uninitialized Data Segment Of Memory Layout In C
The Uninitialized Data Segment, also referred to as the BSS (Block Started by Symbol) segment, is responsible for holding global and static variables that do not have explicit initial values when declared. Let’s dive into the details and how this segment functions in a C program.
Details Of The Uninitialized Data Segment of Memory Layout in C:
- Global and Static Variables: This segment stores global and static variables that have not been explicitly initialized during declaration.
- Default Values: Variables in this segment are automatically assigned a default value of zero during runtime. For integers, this is 0, and for floating-point numbers, this is 0.0.
In the C program example below, we illustrate the use of uninitialized global and static integer variables. Then, in the table after, we show how this translates to the memory layout in C.
Code Example:
#include <stdio.h>
int globalVar; // Uninitialized global variable
static double staticVar; // Uninitialized static variable
void displayValues() {
printf("Global Variable: %d\n", globalVar);
printf("Static Variable: %.2f\n", staticVar);
}
int main() {
displayValues();
return 0;
}
Output:
Global Variable: 0
Static Variable: 0.00
Code Explanation:
In the C code example,
- As mentioned in the code comments, we declare two uninitialized variables—globalVar (a global integer) and staticVar (a static double). Since they are uninitialized, they are allocated memory in the Uninitialized Data Segment (BSS).
- We then define the displayValue() function, which uses printf() to display the default values of these uninitialized variables.
- During runtime, globalVar is automatically assigned the default value of 0, and staticVar is assigned 0.00 (for floating-point numbers).
- When we run the C program, the values of globalVar and staticVar are printed, showing their default initialized values: 0 and 0.00, respectively.
Memory Layout After The Code Above Is Executed:
Segment | Contents |
---|---|
Text Segment | main() function, displayValues() function |
Uninitialized Data Segment (BSS) | Global Variable (globalVar: 0), Static Variable (staticVar: 0.00) |
Data Segment | Empty (no initialized variables) |
Heap Segment | Empty (no dynamic memory allocated) |
Stack Segment | Function call stack for main() and displayValues() |
The representation above highlights how the Uninitialized Data Segment stores global and static variables that are not explicitly initialized. When the program runs, default values are assigned to these variables, ensuring the program operates correctly even without explicit initial values.
Stack Segment Of Memory Layout In C
The Stack memory segment is a dynamic and organized area that plays a crucial role in managing function calls and local variables and maintaining the execution flow of your program. The details of the stack segment of the memory layout in C are given below, followed by its syntax and comprehensive use cases.
Details Of The Stack Segment of Memory Layout in C:
- Function Calls: The Stack memory section tracks the execution flow by managing function calls. A new stack frame is created each time a function is invoked, containing local variables and related data.
- Local Variables: The stack segment stores local variables declared within functions, temporarily. Once the function's execution is complete, its corresponding stack frame is popped off, reclaiming the allocated space.
- Execution Flow Control: The stack functions like a structured to-do list, ensuring functions are called and executed systematically while managing local variables efficiently.
Syntax:
// Define the maximum size of the stack
#define MAX_SIZE 100// Define the stack structure
struct Stack {
int arr[MAX_SIZE];
int top;
};
Here,
- The #define preprocessor directive sets a constant value/ macro named MAX_SIZE to 100. It represents the maximum number of elements that the stack can hold.
- The struct keyword indicates that we are defining a structure named Stack, allowing bundling different types of variables under a single name inside curly braces {}.
- The array arr[] is the name/ identifier of the integer array (int data type) with MAX_SIZE specifying the size/ number of elements in the array. This array is where the actual elements of the stack will be stored.
- Integer variable top track of the top element of the stack, indicating the position where the next element will be added or removed.
Comprehensive Use Cases Of Stack Section Of Memory Layout In C
- Function Calls: The stack manages function calls effectively. When a function is called, a new stack frame is created to store local variables, return addresses, and other necessary information. As functions complete execution, their corresponding stack frames are popped off, maintaining a streamlined execution flow.
- Local Variables: Local variables declared within a function are stored on the stack, preventing conflicts with other parts of the program. This isolation ensures that functions can operate independently and reliably.
- Parameter Passing: Parameters passed to functions are often stored on the stack. This approach efficiently handles the passing of values between functions, ensuring a clean and organized management of parameters.
- Execution Flow Control: The stack serves as a roadmap for the program's execution flow, keeping track of where the program should return after completing a function and ensuring local variables are appropriately handled.
- Recursion: The stack is essential for recursion. A new stack frame is created for each recursive call, allowing the program to maintain multiple copies of the same function with different local variables.
- Temporary Storage: The stack is often used for temporary data storage during program execution, ensuring efficient memory management as stack frames are reclaimed once their purpose is fulfilled.
Understanding the stack's role in managing function calls and local variables provides foundational insight into how C programs navigate and organize their execution flow. Each segment of the memory layout contributes to the seamless functioning of C programs.
Common Stack Error Conditions
When working with stacks, it is important to be aware of potential errors, as they can lead to program failures if not handled properly. Two significant types of stack errors are Stack Overflow and Stack Corruption, which we will discuss in this section.
Error Condition 1: Stack Overflow
- Definition: Stack Overflow occurs when the stack exceeds its predefined size, resulting in a collision with adjacent memory.
- Causes: Recursive function calls, excessive local variable usage, or an insufficiently sized stack can lead to overflow.
- Consequences: Overwriting adjacent memory can cause unpredictable behavior, crashes, and security vulnerabilities.
In the example C program, we illustrate how stack overflow occurs. Note that when executed, it will result in an endless loop.
Code Example:
#include <stdio.h>
void recursiveFunction(int counter) {
int localVariable;
printf("Stack Depth: %d\n", counter);
recursiveFunction(counter + 1); // Note: This will cause a stack overflow
}
int main() {
recursiveFunction(1);
return 0;
}
Code Explanation:
In the example C code, we define a function recursiveFunction(), which takes an integer variable counter as input and continuously calls itself. There is no termination condition (base case), due to which the calls and localVariable consume stack space with each iteration. This results in a stack overflow once the stack limit is reached.
How To Avoid:
- Optimize Recursion: Ensure recursive functions have appropriate termination conditions to avoid infinite recursion.
- Adjust Stack Size: Increase the stack size if your program involves deep recursion or extensive local variable usage.
Error Condition 2: Stack Corruption
- Definition: Stack Corruption occurs when data in the stack is unintentionally overwritten or modified.
- Causes: Improper pointer usage, buffer overflows, or coding bugs can lead to corruption.
- Consequences: Altered control flow, incorrect data, and program crashes can result from stack corruption.
The C program sample below illustrates this concept, after which we describe how to avoid stack corruption.
Code Example:
#include <stdio.h>
void corruptStack() {
int array[5];
for (int i = 0; i <= 5; i++) { // Note: This will cause stack corruption
array[i] = i; // Writing beyond the bounds of the array
}
}
int main() {
corruptStack();
return 0;
}
Output:
No output because writing beyond the bounds of the array causes stack corruption.
Code Explanation:
In the C code sample, we define the corruptStack() function containing an array of integers with 5 elements. We then use a for loop to iterate through the array and write values to indices 0 through 5. We increment the loop control variable i by 1 after every iteration, and the loop should ideally terminate after i becomes equal to or greater than 5. However, since the array only has 5 elements, accessing index 5 (6th element) leads to stack corruption.
How To Avoid:
- Bounds Checking: Always perform proper bounds checking when using arrays to prevent buffer overflows.
- Pointer Safety: Be cautious when using pointers, avoiding invalid memory accesses.
Other Stack Error Conditions
In addition to Stack Overflow and Stack Corruption, several other stack error conditions can arise:
-
Accessing Invalid Memory:
- Definition: This occurs when trying to read or modify data beyond the boundaries of the allocated stack space.
- Consequences: Accessing invalid memory can lead to data corruption, unpredictable behavior, or program crashes. Proper bounds checking is essential to prevent such errors.
-
Unbalanced Push and Pop Operations:
- Definition: This occurs when the number of elements pushed onto the stack does not match the number of elements popped off.
- Consequences: Unbalanced operations can lead to memory leaks, data corruption, or incorrect program output.
-
Missing Initialization:
- Definition: This happens when using a stack variable without initializing it first.
- Consequences: Using uninitialized variables can result in unpredictable values being pushed onto the stack, leading to program bugs or incorrect results.
Heap Segment Of Memory Layout In C
The Heap segment of memory, in contrast to the stack, provides dynamic memory allocation to accommodate data structures whose size is not known at compile time. The details of the Heap segment of memory layout in C is as follows:
Details of the Heap Segment of Memory Layout in C:
- Dynamic Memory Allocation: The Heap segment enables dynamic memory allocation during the program's execution, allowing for the creation of data structures whose size can be determined at runtime. This flexibility is crucial for applications requiring variable-sized data, such as arrays and linked lists.
- Explicit Memory Management: Unlike the stack, the Heap segment requires explicit memory management. Developers are responsible for allocating memory when needed and releasing it when no longer required. This responsibility helps prevent memory leaks and fragmentation.
- Data Structures and Objects: Complex data structures such as arrays, linked lists, trees, and objects can be dynamically created and manipulated in the Heap segment. This capability is essential for applications that require dynamic resizing or modification of data structures.
The sample C program below illustrates how the flow moves through the Heap segment when we dynamically allocate memory for a variable.
Code Example:
#include <stdio.h>
#include <stdlib.h>
int main() {
// Dynamic memory allocation for an integer
int *dynamicInt = (int*)malloc(sizeof(int));
// Check if memory allocation was successful
if (dynamicInt == NULL) {
printf("Memory allocation failed.\n");
return 1; // Return an error code
}
// Assign a value to the dynamically allocated integer
*dynamicInt = 42;
printf("Value of dynamically allocated integer: %d\n", *dynamicInt);
// Free the allocated memory when done
free(dynamicInt);
return 0;
}
Output:
Value of dynamically allocated integer: 42
Code Explanation:
In this sample C code, we include the <stdlib.h> header, which provides functions for dynamic memory allocation.
- Then, in the main() function, we dynamically allocate memory for an integer on the heap using the malloc() function. Here, we use the sizeof() operator to determine the size of memory to allocate.
- Before proceeding, we use an if-statement to check if the memory allocation was successful by comparing the returned pointer, dynamicInt, with a NULL pointer. If malloc fails to allocate memory, the if-block prints an error message, and the program returns 1 to indicate an error.
- If malloc is successful, we assign the value 42 to the memory location pointed to by dynamicInt, and print it using the printf() function.
- Then, we use the free() function to release the dynamically allocated memory to prevent memory leaks.
Memory Layout After The Above Code Is Executed:
Segment | Contents |
---|---|
Text Segment | Program code, constants, and string literals |
Data Segment | Global and static variables (initialized/uninitialized) |
Heap Segment | Dynamically allocated memory (e.g., dynamicInt) |
Stack Segment | Function call information and local variables. |
The table shows how the memory layout changes when the program is executed. The Heap Segment contains dynamically allocated memory, specifically for dynamicInt, which points to the integer allocated with malloc(). The Stack Segment manages the function call information for main() and any local variables (such as the pointer dynamicInt). The Text Segment stores the compiled code and any constants or string literals, while the Data Segment contains global and static variables (though none are declared in this example).
Hone your coding skills with the 100-Day Coding Sprint at Unstop and compete to rank on the leader board.
Command-line Arguments Segment Of Memory Layout In C
In C programming, command-line arguments provide a means for external inputs to influence the behavior of a program during execution. These arguments are parameters passed to a C program when it is invoked but from the command line. In other words,
- Command-line arguments are strings or parameters provided to a program when it is run from the command line or terminal.
- These arguments are passed to the main() function, allowing the program to receive external input and adapt its behavior accordingly.
How Command-Line Arguments Work In C?
The main() function in C can be defined with two parameters: argc and argv.
- argc (argument count): Represents the number of command-line arguments passed to the program.
- argv (argument vector): An array of strings where each element is a command-line argument. The first element (argv[0]) is the name of the program itself.
Importance Of Command-line Argument In Memory Layout In C
Memory for command-line arguments is managed in the stack segment of the program's memory layout.
- The array of strings (argv) and the count of arguments (argc) are typically stored in the stack.
- The strings themselves (individual command-line arguments) are stored in the data segment.
Dynamic Memory Allocation for Command-Line Arguments
- While the argv array itself is typically managed on the stack, the strings it points to are often dynamically allocated on the heap.
- Dynamic allocation allows for flexibility in handling arguments of varying lengths. Developers need to manage memory for these strings explicitly.
Code Example:
#include <stdio.h>
int main() {
// Simulated command-line arguments
char *fakeArgs[] = {"program_name", "arg1", "arg2"};
int fakeArgCount = sizeof(fakeArgs) / sizeof(fakeArgs[0]);
printf("Welcome to the Unstop blog!\n");
// Check if command-line arguments are provided
if (fakeArgCount > 1) {
printf("You provided %d command-line argument(s):\n", fakeArgCount - 1);
// Print each command-line argument
for (int i = 1; i < fakeArgCount; i++) {
printf("Argument %d: %s\n", i, fakeArgs[i]);
}
} else {
printf("No command-line arguments provided.\n");
}
return 0;
}
Output:
Welcome to the Unstop blog!
You provided 2 command-line argument(s):
Argument 1: arg1
Argument 2: arg2
Code Explanation:
In this C example, we are simulating command-line arguments, which are usually given in the shell itself.
- In the main() function, we define an array of character pointers fakeArgs[] and assign three string values to it, each of which represents the command-line arguments.
- Then, we declare an integer variable, fakeArgCount, to represent the number of arguments, which we calculate by dividing the size memory of all elements by that of the first element.
- Next, we use an if-else statement to check if any command-line arguments are provided by verifying if fakeArgCount is greater than 1.
- If there’s only one argument, it’s the program name, so no additional arguments are considered.
- If command-line arguments are provided, we print the total number of arguments provided (excluding the program name) and iterate over each argument using a loop to print them individually.
- If no command-line arguments are provided (i.e., fakeArgCount is 1), the else-block prints a message indicating the absence of arguments.
Dynamic Memory Layout Of C Program
Dynamic memory offers a flexible, runtime-managed approach to memory allocation, allowing programs to allocate and deallocate memory as needed during execution. Unlike static memory (allocated at compile-time), dynamic memory is allocated at runtime, enabling the creation of data structures with sizes determined at execution time, such as arrays and linked lists.
Dynamic Memory Layout In C
Dynamic memory allocation in C is akin to having a flexible storage system. It allows programs to request memory on the fly rather than predefining memory requirements during compilation. Two key memory segments are involved in the dynamic memory layout in C: the Heap and the Stack.
Heap Segment:
The Heap is dedicated to dynamic memory allocation and is crucial for managing data structures whose size is unknown at compile-time. We use the following functions to allocate memory at runtime:
- malloc(size): Allocates a specified amount of memory.
- calloc(num, size): Allocates memory for an array of elements, initializing them to zero.
- realloc(ptr, new_size): Resizes an existing memory block.
Note that managing memory in the Heap is the programmer's responsibility, and they must explicitly deallocate memory using free(). Failure to do this can lead to memory leaks or inefficient memory usage.
Stack Segment:
The Stack handles local variables and function calls and also stores pointers to dynamically allocated memory in the Heap, enabling efficient access to Heap memory. While the actual data resides in the Heap, the Stack tracks these pointers for efficient access.
Let's look at an example we've already seen in the Heap segment section. Here, we will discuss what happens in the Heap and the Stack segments when memory is allocated dynamically.
Code Example:
#include <stdio.h>
#include <stdlib.h>
int main() {
// Dynamic memory allocation for an integer
int *dynamicInt = (int*)malloc(sizeof(int));
// Check if memory allocation was successful
if (dynamicInt != NULL) {
// Assign a value to the dynamically allocated integer
*dynamicInt = 42;
// Print the value of the dynamically allocated integer
printf("The dynamically allocated integer value is: %d\n", *dynamicInt);
// Free the allocated memory when done
free(dynamicInt);
}
return 0;
}
Output:
The dynamically allocated integer value is: 42
Code Explanation:
In the example above, when we call the malloc() function, memory is allocated dynamically on the heap. The size of the allocated memory is determined at runtime, which, in this case, is large enough to store an integer (sizeof(int)).
- The memory location for this dynamically allocated integer exists in the heap, which allows flexible memory allocation that isn't determined at compile time.
- The actual integer value (42) resides in this heap-allocated memory space, which persists until the programmer explicitly releases it using free(dynamicInt).
Stack Component: The pointer dynamicInt, which holds the address of the dynamically allocated memory, is stored on the stack.
- The stack keeps track of the pointer to the heap memory. Whenever the program needs to access the value stored on the heap, it uses this pointer in the stack to locate the heap's memory address.
- The local variables, like dynamicInt itself, are stored in the stack, and when the function completes execution, the stack frame is cleaned up.
Memory Layout When The Above Code is Executed:
Segment | Contents |
---|---|
Heap Segment | Dynamically Allocated Integer (dynamicInt) |
Stack Segment | Pointer to Dynamically Allocated Memory |
This illustrates how pointers in the Stack direct access to dynamically allocated memory in the Heap, a fundamental concept in C’s dynamic memory management.
Static Memory Layout Of C Program
Static memory in C is allocated at compile-time and persists throughout the program’s execution. The key components of static memory are the Text Segment, Data Segment, and BSS Segment, which we’ve discussed in detail earlier.
In short, the static memory layout in C comprises:
- Text Segment: Stores executable code and read-only data.
- Data Segment: Holds initialized global and static variables.
- BSS Segment: Contains uninitialized static variables, initialized to zero.
Code Example:
#include <stdio.h>
// Initialized global variable in Data Segment
int globalVar = 10;
// Uninitialized global variable in BSS Segment
static int uninitializedVar;
int main() {
// Local static variable in Data Segment
static int localStaticVar = 5;
// Print values to demonstrate static memory layout
printf("Value of globalVar: %d\n", globalVar);
printf("Value of uninitializedVar: %d\n", uninitializedVar);
printf("Value of localStaticVar: %d\n", localStaticVar);
return 0;
}
Output:
Value of globalVar: 10
Value of uninitializedVar: 0
Value of localStaticVar: 5
Explanation:
- Data Segment: The globalVar and localStaticVar are stored here. Both are initialized before the program starts (globalVar = 10 and localStaticVar = 5).
- BSS Segment: The variable uninitializedVar resides in the BSS segment and is automatically initialized to 0, as it was declared without an initial value.
- Text Segment: The compiled code, including the main() function and the printf() calls, is stored here.
Check the Size of Code, Data, and BSS Segments
Understanding the size of memory segments, such as Code, Data, and BSS, is crucial for optimizing a C program's memory usage. This section will explore how to check the size of these segments using the size command.
- Code Segment (Text): Contains the compiled machine code of the program. Checking its size helps assess the overall memory footprint of your code.
- Data Segment: Stores initialized global and static variables. Understanding its size helps manage the memory used by initialized variables.
- BSS Segment: Holds uninitialized global and static variables. Knowing its size is important for estimating memory consumption from these variables.
Let's take a code and then run the size command to determine the size of the various segments.
Code Example:
#include <stdio.h>
// Initialized global variable in the Data Segment
int globalVar = 10;
// Uninitialized global variable in the BSS Segment
static int uninitializedVar;
int main() {
printf("Hello, world!\n");
return 0;
}
Once you compile this program above, you can use the size command to check the memory layout. Here what you will have to type in the Bash terminal:
$ size myProgram
Output:
text data bss dec hex filename
1024 256 32 1312 520 myProgram
Code Explanation:
- The size command provides a direct breakdown of how much memory each segment of your program occupies.
- In the output above, the text segment is 1024 bytes, the data segment is 256 bytes, and the BSS segment is 32 bytes.
Example Of Memory Layout In C
Let’s walk through a step-by-step example of a basic C program and see how incremental changes affect the memory layout, specifically focusing on the Code, Data, and BSS segments.
Step 1: Basic Program
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
Memory Layout Impact: We begin with a simple C program that prints "Hello, World!" to the console. At this stage, the executable instructions/ code resides in the Text Segment, which holds the compiled instructions for the printf function.
Step 2: Adding Initialized Data
#include <stdio.h>
int globalVar = 42;
int main() {
printf("Hello, World! GlobalVar: %d\n", globalVar);
return 0;
}
Memory Layout Impact: We introduce an initialized global variable globalVar and print its value alongside our greeting message. With this addition, the initialized global variable occupies space in memory in the Data segment.
Step 3: Adding Uninitialized Data
#include <stdio.h>
int globalVar = 42;
int uninitializedVar;
int main() {
printf("Hello, World! GlobalVar: %d\n", globalVar);
return 0;
}
Memory Layout Impact: We include an uninitialized global variable, uninitializedVar, without assigning it a value. This variable uninitializedVar is allocated space in the BSS Segment.
Step 4: Adding Local Static Variable
#include <stdio.h>
int globalVar = 42;
int uninitializedVar;
int main() {
static int localStaticVar = 7;
printf("Hello, World! GlobalVar: %d LocalStaticVar: %d\n", globalVar, localStaticVar);
return 0;
}
Memory Layout Impact: We introduce a local static variable, localStaticVar, which, unlike automatic variables, persists throughout the program's execution. The local static integer variable joins other initialized variables in the Data Segment.
Step 5: Adding Function with Local Variable
#include <stdio.h>
int globalVar = 42;
int uninitializedVar;
void myFunction() {
int localVar = 3;
// Some code...
}
int main() {
static int localStaticVar = 7;
printf("Hello, World! GlobalVar: %d LocalStaticVar: %d\n", globalVar, localStaticVar);
return 0;
}
Memory Layout Impact: Here, we define a function myFunction with a local variable localVar. The code for the function, along with its local variable localVar, is stored in the Text Segment.
Step 6: Allocating Dynamic Memory
#include <stdio.h>
#include <stdlib.h>
int globalVar = 42;
int uninitializedVar;
void myFunction() {
int localVar = 3;
// Some code...
}
int main() {
static int localStaticVar = 7;
// Allocating dynamic memory
int* dynamicInt = (int*)malloc(sizeof(int));
printf("Hello, World! GlobalVar: %d LocalStaticVar: %d\n", globalVar, localStaticVar);
// Freeing dynamic memory
free(dynamicInt);
return 0;
}
Memory Layout Impact: Finally, we dynamically allocate memory for an integer pointer dynamicInt within the main function using the malloc function. The dynamic memory allocation for dynamicInt occurs in the Heap Segment.
This example illustrates how different modifications to the program affect the memory layout across various segments, providing insights crucial for optimizing memory management in C programs. Understanding these changes will help you write more efficient code and manage memory effectively.
Need guidance? Find the perfect mentor for yourself from experienced coding & software experts here.
Conclusion
The memory layout in C comprises five major segments. The Text Segment contains the executable code, serving as the foundation for program execution. The Data Segment holds initialized global and static variables, while the BSS Segment is reserved for uninitialized variables, ensuring efficient memory allocation. Also, the Heap Segment allows dynamic memory management during runtime, and the Stack Segment manages function calls and local variables.
Understanding these segments is crucial for writing efficient C programs and optimizing memory usage, ultimately leading to better resource management in software development.
Also read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q1. What is the memory format of C?
The memory format in C outlines how memory is organized during program execution, dividing it into several segments, each having its own purpose. The segments are:
- Code Segment (Text Segment): Contains compiled program code, functions, and constants.
- Data Segment: Stores initialized global and static variables with explicit starting values.
- BSS Segment: Reserved for uninitialized global and static variables, defaulting to zero.
- Heap Segment: Facilitates dynamic memory allocation during runtime, managed by functions like malloc() and free().
- Stack Segment: Manages function calls, function parameters and local variables in a last-in, first-out (LIFO) structure.
Q2. What is the memory layout of arrays?
In C, arrays are stored in memory as contiguous blocks of memory, meaning that all elements of the array are located one after the other in a linear sequence. The layout follows the order of declaration, with each element occupying a specific size determined by the array's data type. For example, if you declare an array of integers, each integer (typically 4 bytes) will be placed in adjacent memory locations.
The address of each element can be calculated using the formula:
Address of array[i] = Base address of array + (i * size of each element)
This contiguous storage ensures efficient access to array elements, as their addresses can be computed quickly during program execution.
Q3. What are the types of memory used in dynamic allocation in C?
In C, dynamic memory allocation primarily utilizes the Heap Segment. This segment allows programs to request memory at runtime using functions like malloc(), calloc(), and realloc(). Unlike stack memory, which is automatically managed, heap memory must be explicitly allocated and freed by the programmer using free to prevent memory leaks. Therefore, the types of memory associated with dynamic allocation in C can be summarized as follows:
- Heap Memory: Used for dynamic memory allocation during runtime, enabling flexible memory management.
- Stack Memory: While not typically used for dynamic allocation, local variables and function parameters are stored here, which can interact with dynamically allocated memory.
Q4. What are the 4 types of memory in C?
The four main types of memory in C are categorized based on the role they play in storing program elements:
- Code Segment (Text Segment): Contains the compiled code of the program, including functions and constants.
- Data Segment: Stores initialized global and static variables with explicit starting values.
- BSS Segment: Reserved for uninitialized global and static variables, initializing them to zero.
- Heap Segment: Facilitates dynamic memory allocation during runtime, allowing flexible memory management.
Q5. How is a structure stored in memory in C?
In C, a structure is stored in memory as a contiguous block, where each member of the structure occupies a specific offset within that block. The layout of the structure follows the order in which its members are declared. The total size of the structure is determined by the sum of the sizes of its individual members, which may be adjusted for padding to ensure proper memory alignment.
When a structure is declared, memory is allocated for each member based on its data type. The members are accessed using the structure's base address, with their individual offsets calculated based on their order in the declaration. For instance, if a structure has an integer followed by a double, the memory layout will reflect these types, potentially introducing padding bytes between members to align them correctly in memory.
By now, you must be familiar with the memory layout in C programs. Here are a few other articles you must read:
- Infinite Loop In C | Types, Causes, Prevention (+Code Examples)
- Void Pointer In C | Referencing, Dereferencing & More (+Examples)
- Union In C | Declare, Initialize, Access Member & More (Examples)
- Nested Loop In C | For, While, Do-While, Break & Continue (+Codes)
- Type Casting In C | Cast Functions, Types & More (+Code Examples)
- Control Statements In C | The Beginner's Guide (With Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment